<< Back to C-SHARP
C# for Loop Examples
Iterate over numbers with for. Increment or decrement an index int from a start to an end value.For loop. You feel a gust of wind. A branch on a nearby tree rustles. In nature these phenomena are all around us. Our world is full of motion.
Loop details. In a for-loop, we iterate through a series of numbers. We progress from one point to another. Like the wind we travel in a path, over a range.
First example. The name of the variable "i" is a convention. It is easier for other programmers to understand than unusual variable names.
Start: The loop starts at the value 0. In C# most collections are zero-based, so the first element is at 0.
End: The loop continues running through the body until "i" equals 10 (or some other value if it is set elsewhere).
Increment: Please note the third clause in the for-loop, the i++ part. This is the iteration statement—it occurs after each pass.
C# program that uses increment loop
using System;
class Program
{
static void Main()
{
for (int i = 0; i < 10; i++)
{
Console.WriteLine(i);
}
}
}
Output
0
1
2
3
4
5
6
7
8
9
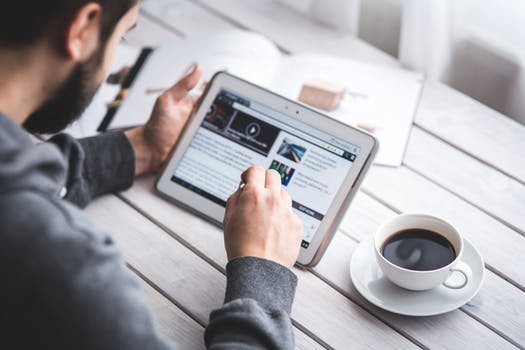
Decrement loop. This is a simple example. A for-loop can decrement in the step condition. Here we start at 10, and continue until 0 is reached.
Tip: Make sure to use ">= 0" in decrementing for-loops to ensure you reach the index 0 and can process it.
C# program that uses decrement loop
using System;
class Program
{
static void Main()
{
for (int i = 10 - 1; i >= 0; i--)
{
Console.WriteLine(i);
}
}
}
Output
9
8
7
6
5
4
3
2
1
0
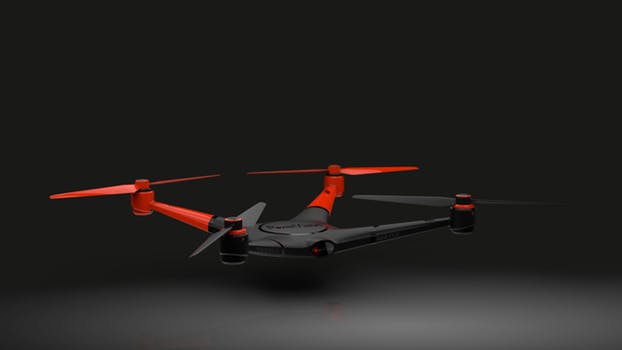
Iteration step. The third clause in the for-loop is the step. This can change the variable (or any variable) by any amount—a constant is not even needed.
Two: Here we add two after each pass through the loop. So we skip odd number indexes.
C# program that uses step increment loop
using System;
class Program
{
static void Main()
{
for (int i = 0; i < 10; i += 2)
{
Console.WriteLine(i);
}
}
}
Output
0
2
4
6
8
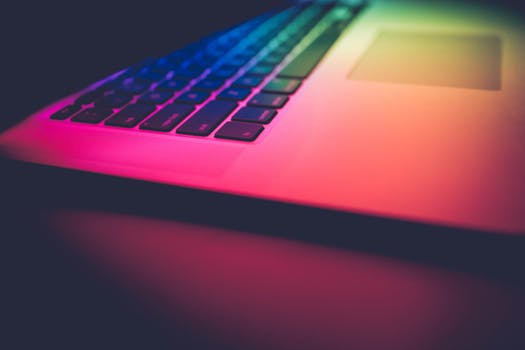
Decrement, step. This program revisits loop decrementing. It decreases the iteration variable by two each time. The example is simple and straightforward.
C# program that uses step decrement loop
using System;
class Program
{
static void Main()
{
for (int i = 10 - 1; i >= 0; i -= 2)
{
Console.WriteLine(i);
}
}
}
Output
9
7
5
3
1
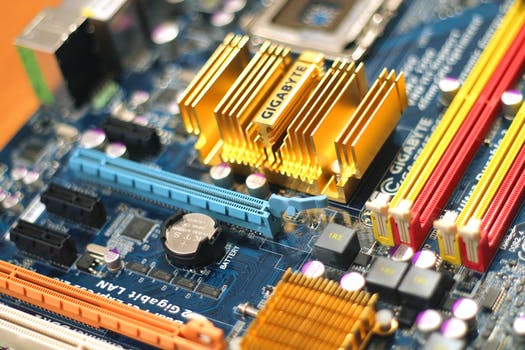
Expression, maximum bound. Complex expressions, even method calls, can be used in the conditions of a for-loop. This can help simplify code.
However: Be careful not to call an expensive function too much. Unneeded operations can end up executing.
C# program that uses expression in maximum bounds
using System;
class Program
{
static void Main()
{
for (int i = 0; i < (20 / 2); i += 2)
{
Console.WriteLine(i);
}
}
}
Output
0
2
4
6
8
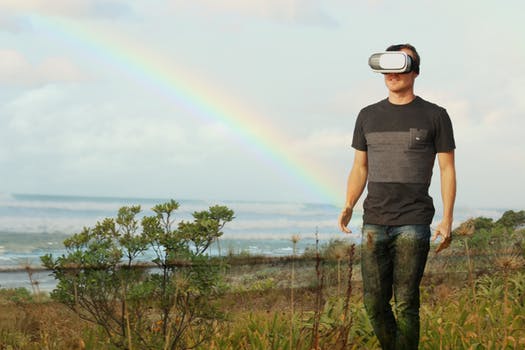
For parts. When a for-loop is encountered, the first of the three statements is executed. The for-loop shown first has its "int i = 0" statement executed.
Part 1: We can start a for-loop with any value for the iteration variable. The value does not need to be a constant.
Part 2: Evaluated before the loop body is entered. If this is true, the loop proceeds. If false, it stops (the body is not entered).
True, FalsePart 3: Executed after each successful iteration. Specifies how the iteration variable (i) changes.
C# program that shows 3 parts of for-loop
using System;
class Program
{
static int FirstPart()
{
Console.WriteLine("[1] PART 1");
return 0;
}
static int SecondPart()
{
Console.WriteLine("[2] PART 2");
return 3;
}
static int ThirdPart()
{
Console.WriteLine("[3] PART 3");
return 1;
}
static void Main()
{
// Carefully understand how the 3 parts are called.
for (int i = FirstPart();
i < SecondPart();
i += ThirdPart())
{
Console.WriteLine("[ ] BODY");
}
}
}
Output
[1] PART 1
[2] PART 2
[ ] BODY
[3] PART 3
[2] PART 2
[ ] BODY
[3] PART 3
[2] PART 2
[ ] BODY
[3] PART 3
[2] PART 2
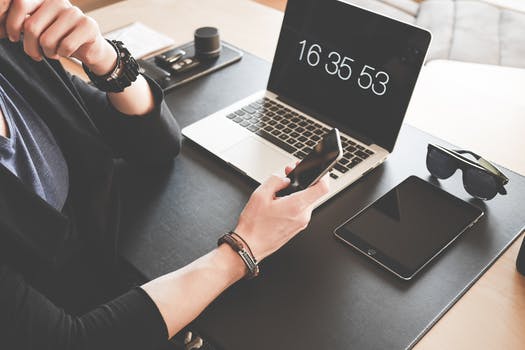
Empty statements. We can omit statements in the for-loop. With empty statements, we just see some semicolons. No action is taken on each iteration—this is like a while true loop.
C# program that shows empty for-statements
using System;
class Program
{
static void Main()
{
int i = 0;
// Use for-loop with empty statements.
for (; ; )
{
if (i > 4)
{
break;
}
Console.WriteLine("EMPTY FOR-LOOP: " + i);
i++;
}
}
}
Output
EMPTY FOR-LOOP: 0
EMPTY FOR-LOOP: 1
EMPTY FOR-LOOP: 2
EMPTY FOR-LOOP: 3
EMPTY FOR-LOOP: 4
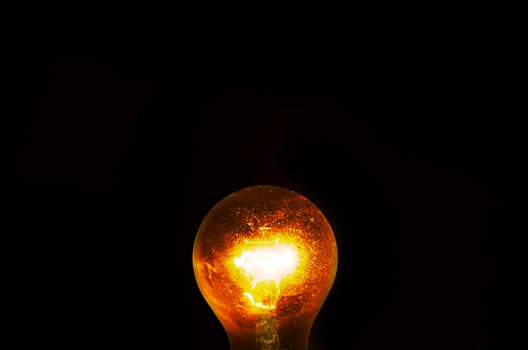
For, 2 variables. We can use 2 variables in a for-loop statement. Here we initialize "i" and "x" to zero, and increment "i" and decrement "x."
Tip: We use commas to separate statements. For the termination condition, we can use 1 expression with the "&&" operator.
C# program that loops over 2 variables
class Program
{
static void Main()
{
// Loop over 2 variables at once.
for (int i = 0, x = 0; i < 10 && x >= -2; i++, x--)
{
System.Console.WriteLine("FOR: i={0}, x={1}", i, x);
}
}
}
Output
FOR: i=0, x=0
FOR: i=1, x=-1
FOR: i=2, x=-2
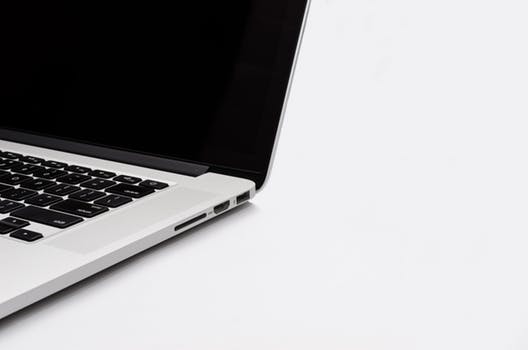
Chars. A for-loop often uses an int index. But other index types are possible. Here I use a char variable and loop over all the lowercase letters.
Tip: Often a for-loop over chars is useful for initializing a lookup table. Each char is accessed separately.
ROT13C# program that uses for, char range
using System;
class Program
{
static void Main()
{
// Loop over character range.
for (char c = 'a'; c <= 'z'; c++)
{
Console.WriteLine(c);
}
}
}
Output
a
b
c
d
e....
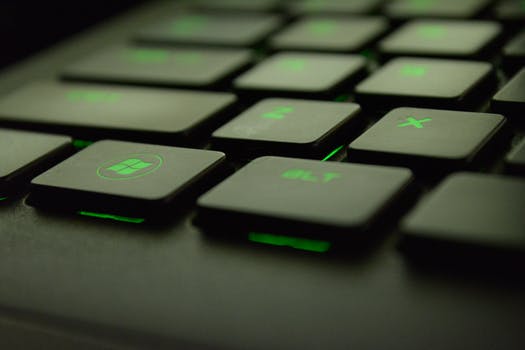
Strings. All kinds of loops work with strings. But the for-loop is often preferable for its syntax and index variable. Testing chars directly is fastest.
For, Foreach: An example shows a string being looped over with for and foreach in the same way. For gives us an index to use.
Loop, String CharsPerformance: Make sure not to use ToString in a loop if you do not need it. Improve performance by not using ToString in a for-loop.
Loop, ToStringNext: We see a simple loop through the characters in the constant string literal "Rome." All 4 are printed to the console.
C# program that uses for-loop over string chars
class Program
{
static void Main()
{
string value = "Rome";
// Use for-loop from 0 through Length of string (goes to last index).
for (int i = 0; i < value.Length; i++)
{
char current = value[i];
System.Console.WriteLine(current);
}
}
}
Output
R
o
m
e
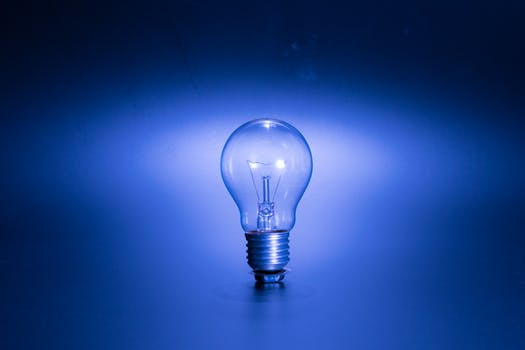
Local function, max. Suppose we need some logic to determine the max bound of a for-loop. Instead of repeating it, we can place it in a local function.
Math.Min: The Math.Min function is sometimes useful in this situation too—it can prevent us from going off the end of an array.
Math.Max, MinC# program that uses local function for loop max
using System;
class Program
{
static void Main()
{
// Use this local function to safely get a top bound for a for-loop.
int Limit(int max, int[] array)
{
return Math.Min(max, array.Length);
}
int[] values = { 10, 20, 30, 40 };
// Continue to index 2.
for (int i = 0; i < Limit(2, values); i++)
{
Console.WriteLine("LIMIT 2: " + values[i]);
}
// Continue to index 10 (or array length).
for (int i = 0; i < Limit(10, values); i++)
{
Console.WriteLine("LIMIT 10: " + values[i]);
}
}
}
Output
LIMIT 2: 10
LIMIT 2: 20
LIMIT 10: 10
LIMIT 10: 20
LIMIT 10: 30
LIMIT 10: 40
Array, for. Loop constructs can be used upon arrays. We can iterate in forward or reverse order, or access the elements in any other order we can come up with.
Loop, ArrayLoop, String ArrayC# program that loops over array
using System;
class Program
{
static void Main()
{
int[] values = { 20, -20, 30 };
for (int i = 0; i < values.Length; i++)
{
int element = values[i];
Console.WriteLine("ARRAY: {0}, {1}", i, element);
}
}
}
Output
ARRAY: 0, 20
ARRAY: 1, -20
ARRAY: 2, 30
Benchmark, jammed. Suppose we have 2 loops that iterate over the same range. If the latter ones do not depend on changes made in the earlier loops, we can merge or "jam" them.
Info: We want to set 3 values in an array repeatedly. There is no data dependence between the 3 operations.
Version 1: Here we see the "jammed" loops merged into 1 loop. Each iteration acts upon 3 indexes before continuing.
Version 2: This version is the unoptimized code. We perform 3 loops to perform the operation, instead of just 1.
Result: The "jammed" loop, where we make a single pass from start to end, is measurably (and consistently) faster.
C# program that uses jammed loop optimization
using System;
using System.Diagnostics;
class Program
{
const int _max = 1000000;
static void Main()
{
int[] data = new int[10];
// Version 1: use jammed loop method.
var s1 = Stopwatch.StartNew();
for (int i = 0; i < _max; i++)
{
Method1(data);
}
s1.Stop();
// Version 2: use separate loops.
var s2 = Stopwatch.StartNew();
for (int i = 0; i < _max; i++)
{
Method2(data);
}
s2.Stop();
Console.WriteLine(((double)(s1.Elapsed.TotalMilliseconds * 1000000) /
_max).ToString("0.00 ns"));
Console.WriteLine(((double)(s2.Elapsed.TotalMilliseconds * 1000000) /
_max).ToString("0.00 ns"));
}
static void Method1(int[] array)
{
// Use "jammed" loop.
for (int i = 0; i < array.Length; i++)
{
array[0] = i;
array[1] = i;
array[2] = i;
}
}
static void Method2(int[] array)
{
// Use 3 separate loops.
for (int i = 0; i < array.Length; i++)
{
array[0] = i;
}
for (int i = 0; i < array.Length; i++)
{
array[1] = i;
}
for (int i = 0; i < array.Length; i++)
{
array[2] = i;
}
}
}
Output
9.11 ns Jammed for-loop (1)
13.39 ns Separate for-loops (3)
Benchmark, unwinding. A loop can process just one operation at a time. But sometimes we can place 2 or more operations in an iteration. This is called loop unrolling or unwinding.
Important: We must be sure to only use this when we know that the size of the loop is divisible by the number of operations.
Version 1: Here we test 1 array element per iteration. This is the simplest way to write many loops.
Version 2: In the second method, we test 2 array elements per iteration—and increment by 2. We unwind the loop.
Result: Acting on 2 array elements per iteration is faster. For small arrays, or arrays of uncertain size, this optimization may not work.
C# program that uses loop unwinding optimization
using System;
using System.Diagnostics;
class Program
{
const int _max = 100000;
static void Main()
{
int[] data = new int[100];
// Version 1: loop over each element.
var s1 = Stopwatch.StartNew();
for (int i = 0; i < _max; i++)
{
Method1(data);
}
s1.Stop();
// Version 2: loop over 2 elements at a time (use loop unwinding).
var s2 = Stopwatch.StartNew();
for (int i = 0; i < _max; i++)
{
Method2(data);
}
s2.Stop();
Console.WriteLine(((double)(s1.Elapsed.TotalMilliseconds * 1000000) /
_max).ToString("0.00 ns"));
Console.WriteLine(((double)(s2.Elapsed.TotalMilliseconds * 1000000) /
_max).ToString("0.00 ns"));
}
static void Method1(int[] array)
{
// If element is 3, change it to 0.
for (int i = 0; i < array.Length; i++)
{
if (array[i] == 3)
{
array[i] = 0;
}
}
}
static void Method2(int[] array)
{
// Handle 2 elements in each iteration, and increment by 2.
// ... Uses loop unwinding.
for (int i = 0; i < array.Length; i += 2)
{
if (array[i] == 3)
{
array[i] = 0;
}
if (array[i + 1] == 3)
{
array[i + 1] = 0;
}
}
}
}
Output
112.84 ns Test 1 element per iteration
65.97 ns Test 2 elements, i += 2, loop unwinding
Benchmark, decrement. Many CPUs can compare against 0 faster. We can use this to optimize a for-loop. We start at the max, decrement, and then compare against zero each iteration.
Version 1: Consider the inner loop of Method 1. We start at the max, decrement by 1, and compare against 0.
Version 2: In this method we start at 0, and continue until we reach the max—so we compare against a non-zero number each time.
Result: The test-against-zero optimization makes the loop a tiny bit faster. Even when tested on a newer PC (in late 2018) this is true.
C# program that uses decrement to zero optimization
using System;
using System.Diagnostics;
class Program
{
const int _max = 1;
static void Main()
{
// Version 1: decrement to zero.
var s1 = Stopwatch.StartNew();
for (int i = 0; i < _max; i++)
{
Method1(10, 100000000);
}
s1.Stop();
// Version 2: increment to max.
var s2 = Stopwatch.StartNew();
for (int i = 0; i < _max; i++)
{
Method2(10, 100000000);
}
s2.Stop();
Console.WriteLine(s1.Elapsed.TotalMilliseconds.ToString("0.00 ms"));
Console.WriteLine(s2.Elapsed.TotalMilliseconds.ToString("0.00 ms"));
}
static int Method1(int max1, int max2)
{
// Inner loop compares against 0 and decrements.
int result = 0;
for (int i = 0; i < max1; i++)
{
for (int a = max2 - 1; a >= 0; --a)
{
if (result++ > 1000)
{
result = 0;
}
}
}
return result;
}
static int Method2(int max1, int max2)
{
// Inner loop compares against max int and increments.
int result = 0;
for (int i = 0; i < max1; i++)
{
for (int a = 0; a < max2; a++)
{
if (result++ > 1000)
{
result = 0;
}
}
}
return result;
}
}
Output
556.88 ms Decrement, test against 0
558.37 ms Increment, test against max int
Performance, review. The for-loop is often the fastest way to loop over a series of numbers. It does not require allocations. It is easy for the compiler to optimize.
Array: The JIT compiler can detect array accesses in a for-loop. It can determine the iteration variable will never be out-of-bounds.
Then: It can automatically remove array bounds checking. Because of this, the simplest for-loops are sometimes fastest.
Variable names. In isolated, small loops, a simple name such as the famous "i" is a good choice. It is standard. It is short. It is easy to type and remember.
But: If the variable is used to access an array, you can change the name. Use a more descriptive word to indicate its purpose.
Letter i: This identifier has no importance. It is simply a tradition. The book Code Complete by Steve McConnell has great detail here.
A name mistake. Single-letter loop iteration variables seem convenient. But what happens when we have nested loops, and mistake these letters? Sometimes more descriptive names are better.
Compilers. Programs commonly spend most of their time in tight loops such as for-loops. Compilers put considerable effort into speeding up these constructs. This is worthwhile.
Note: Fields of mathematics (linear algebra) can analyze data reuse and data dependence in for-loops.
And: Compilers use techniques (like strength reduction) to improve performance of affine expressions based on iteration variables.
Keywords, notes. With break we can stop a loop—no more iterations are run. Continue ends the current iteration only. Goto allows us to transfer control—but there are limitations here.
BreakContinueGotoParallel.For. This is a loop we write as a function. This can perform a task many times faster, but we must use a separate method, and avoid data dependencies.
Parallel.ForWhile versus for. Is the end point known? In a for-loop we usually go from a known point to another known point. A while-loop is better when no end is yet known.
WhileA summary. The for-loop is powerful and easy to write. It is many developers' loop of choice. It makes a C# program feel more like the old times when developers were writing C code.
Related Links:
- C# Array Examples, String Arrays
- C# ArrayList Examples
- C# ArraySegment: Get Array Range, Offset and Count
- C# break Statement
- C# Buffer BlockCopy Example
- C# BufferedStream: Optimize Read and Write
- 404 Not Found
- C# 24 Hour Time Formats
- C# 2D Array Examples
- 7 Zip Command Line Examples
- C# 7 Zip Executable (Process.Start)
- C# All Method: All Elements Match a Condition
- C# Alphabetize String
- C# Alphanumeric Sorting
- C# Arithmetic Expression Optimization
- C# Array.AsReadOnly Method (ObjectModel)
- C# Array.BinarySearch Method
- C# Array.Clear Examples
- C# Array.IndexOf, LastIndexOf: Search Arrays
- C# async, await Examples
- C# Attribute Examples
- C# Average Method
- C# BackgroundWorker
- C# base Keyword
- C# String Between, Before, After
- C# Binary Representation int (Convert, toBase 2)
- C# BinarySearch List
- C# bool Array (Memory Usage, One Byte Per Element)
- C# bool.Parse, TryParse: Convert String to Bool
- C# bool Type
- C# Array Length Property, Get Size of Array
- C# Button Example
- C# Byte Array: Memory Usage, Read All Bytes
- C# Byte and sbyte Types
- C# Capacity for List, Dictionary
- C# Case Insensitive Dictionary
- C# case Example (Switch Case)
- C# Char Array
- C# Checked and Unchecked Keywords
- C# CheckedListBox: Windows Forms
- C# Color Table
- C# Color Examples: FromKnownColor, FromName
- C# ColorDialog Example
- C# Comment: Single Line and Multiline
- C# Concat Extension: Combine Lists and Arrays
- C# Conditional Attribute
- C# Console Color, Text and BackgroundColor
- C# String Clone() method
- C# Constructor Examples
- C# Contains Extension Method
- C# String GetTypeCode() method
- C# String ToLowerInvariant() method
- C# Customized Dialog Box
- C# DataColumn Example: Columns.Add
- C# DataGridView Add Rows
- DataGridView Columns, Edit Columns Dialog
- C# DataGridView Row Colors (Alternating)
- C# DataGridView Tutorial
- C# DataGridView
- C# DataRow Examples
- C# DataSet Examples
- C# DataSource Example
- C# DataTable Compare Rows
- C# DataTable foreach Loop
- C# DataTable RowChanged Example: AcceptChanges
- C# DataTable Select Example
- C# DataTable Examples
- C# DataView Examples
- C# String ToString() method
- C# String ToUpper() method
- C# Digit Separator
- C# DateTime.MinValue (Null DateTime)
- C# DateTime.Month Property
- C# DateTime.Parse: Convert String to DateTime
- C# DateTime Subtract Method
- C# Decompress GZIP
- C# Remove Duplicates From List
- C# dynamic Keyword
- C# ElementAt, ElementAtOrDefault Use
- C# Encapsulate Field
- C# Enum Array Example, Use Enum as Array Index
- C# enum Flags Attribute Examples
- C# Enum ToString: Convert Enum to String
- C# enum Examples
- C# Enumerable.Range, Repeat and Empty
- C# Environment Type
- C# EventLog Example
- C# Exception Handling
- C# explicit and implicit Keywords
- C# Factory Design Pattern
- C# File.Copy Examples
- C# typeof and nameof Operators
- C# String TrimEnd() method
- C# var Examples
- C# virtual Keyword
- C# void Method, Return No Value
- C# volatile Example
- C# WebBrowser Control (Navigate Method)
- C# WebClient: DownloadData, Headers
- C# Where Method and Keyword
- C# String TrimStart() method
- C# delegate Keyword
- C# descending, ascending Keywords
- C# while Loop Examples
- C# Whitespace Methods: Convert UNIX, Windows Newlines
- C# XmlReader, Parse XML File
- C# XmlTextReader
- C# XmlTextWriter
- C# XmlWriter, Create XML File
- C# XOR Operator (Bitwise)
- C# yield Example
- C# float Numbers
- FlowLayoutPanel Control
- C# Focused Property
- C# FolderBrowserDialog Control
- C# Font Type: FontFamily and FontStyle
- C# FontDialog Example
- C# for Loop Examples
- C# foreach Loop Examples
- ForeColor, BackColor: Windows Forms
- C# Form: Event Handlers
- C# Contains String Method
- C# ContainsValue Method (Value Exists in Dictionary)
- C# ContextMenuStrip Example
- C# continue Keyword
- C# Control: Windows Forms
- C# Windows Forms Controls
- C# Convert Char Array to String
- C# Convert Char to String
- C# Convert Days to Months
- C# Convert String to Byte Array
- C# String Format
- C# Func Object (Lambda That Returns a Value)
- C# GC.Collect Examples: CollectionCount, GetTotalMemory
- C# Path.GetDirectoryName (Remove File From Path)
- C# goto Examples
- C# HttpClient Example: System.Net.Http
- ASP.NET HttpContext Request Property
- IL Disassembler Tutorial
- C# Intermediate Language (IL)
- C# IndexOf Examples
- C# IndexOfAny Examples
- C# Initialize Array
- C# Initialize List
- C# InitializeComponent Method: Windows Forms
- C# Inline Optimization
- C# Dictionary Equals: If Contents Are the Same
- C# Dictionary Versus List Loop
- C# Dictionary Order, Use Keys Added Last
- C# Dictionary Size and Performance
- C# Dictionary Versus List Lookup Time
- C# Dictionary Examples
- C# Get Directory Size (Total Bytes in All Files)
- C# Directory Type
- C# Distinct Method, Get Unique Elements Only
- C# Divide by Powers of Two (Bitwise Shift)
- C# Divide Numbers (Cast Ints)
- C# DomainUpDown Control Example
- C# Double Type: double.MaxValue, double.Parse
- C# Remove Duplicate Chars
- C# IEqualityComparer
- C# If Preprocessing Directive: Elif and Endif
- C# If Versus Switch Performance
- C# if Statement
- C# int.MaxValue, MinValue (Get Lowest Number)
- C# Program to reverse number
- C# Int and uint Types
- C# Integer Append Optimization
- C# Keywords
- C# Label Example: Windows Forms
- C# Lambda Expressions
- C# LastIndexOf Examples
- C# Last, LastOrDefault Methods
- C# Mutex Example (OpenExisting)
- C# Named Parameters
- C# Let Keyword (Use Variable in Query Expression)
- C# Levenshtein Distance
- C# LinkLabel Example: Windows Forms
- C# LINQ
- C# List Add Method, Append Element to List
- C# List AddRange, InsertRange (Append Array to List)
- C# List Clear Example
- C# List Contains Method
- C# List Remove Examples
- C# List Examples
- C# ListBox Tutorial (DataSource, SelectedIndex)
- C# ListView Tutorial: Windows Forms
- C# Maze Pathfinding Algorithm
- C# Memoization
- C# Memory Usage for Arrays of Objects
- C# MessageBox.Show Examples
- C# Method Call Depth Performance
- C# Method Parameter Performance
- C# Method Size Optimization
- C# Multidimensional Array
- C# MultiMap Class (Dictionary of Lists)
- C# Optimization
- C# new Keyword
- C# NotifyIcon: Windows Forms
- C# NotImplementedException
- C# Null Array
- C# String GetType() method
- C# Null Coalescing and Null Conditional Operators
- C# Null List (NullReferenceException)
- C# Numeric Casts
- C# NumericUpDown Control: Windows Forms
- C# object.ReferenceEquals Method
- C# Object Examples
- C# Optional Parameters
- C# Prime Number
- C# OrderBy, OrderByDescending Examples
- C# Process Examples (Process.Start)
- Panel, Windows Forms (Create Group of Controls)
- C# Path Examples
- C# Get Percentage From Number With Format String
- ASP.NET PhysicalApplicationPath
- C# PictureBox: Windows Forms
- C# PNG Optimization
- C# Position Windows: Use WorkingArea and Location
- Visual Studio Post Build, Pre Build Macros
- C# ProfileOptimization
- C# ProgressBar Example
- C# Property Examples
- C# PropertyGrid: Windows Forms
- C# Protected and internal Keywords
- C# Public and private Methods
- C# Remove Punctuation From String
- C# Query Windows Forms (Controls.OfType)
- C# Queryable: IQueryable Interface and AsQueryable
- ASP.NET QueryString Examples
- C# Queue Collection: Enqueue
- C# RadioButton Use: Windows Forms
- C# ReadOnlyCollection Use (ObjectModel)
- C# Recursion Optimization
- C# Recursive File List: GetFiles With AllDirectories
- C# ref Keyword
- C# Reflection Examples
- C# Regex.Escape and Unescape Methods
- C# StringBuilder Examples
- C# StringComparison and StringComparer
- C# StringReader Class (Get Parts of Strings)
- C# String GetEnumerator() method
- C# String GetHashCode() method
- C# Regex Versus Loop: Use For Loop Instead of Regex
- C# Regex.Match Examples: Regular Expressions
- C# RemoveAll: Use Lambda to Delete From List
- C# Replace String Examples
- ASP.NET Response.BinaryWrite
- ASP.NET Response.Write
- C# Return Optimization: out Performance
- C# SaveFileDialog: Use ShowDialog and FileName
- C# Scraping HTML Links
- C# sealed Keyword
- C# Seek File Examples: ReadBytes
- C# select new Example: LINQ
- C# Select Method (Use Lambda to Modify Elements)
- C# Serialize List (Write to File With BinaryFormatter)
- C# Settings.settings in Visual Studio
- C# Shuffle Array: KeyValuePair and List
- C# Single and Double Types
- C# Single Instance Windows Form
- C# Snippet Examples
- C# Sort DateTime List
- C# Sort List With Lambda, Comparison Method
- C# Sort Number Strings
- C# Sort Examples: Arrays and Lists
- C# SortedDictionary
- C# SortedList
- C# SortedSet Examples
- C# Split String Examples
- C# String Copy() method
- C# SplitContainer: Windows Forms
- C# SqlClient Tutorial: SqlConnection, SqlCommand
- C# SqlCommand Example: SELECT TOP, ORDER BY
- C# SqlCommandBuilder Example: GetInsertCommand
- C# SqlConnection Example: Using, SqlCommand
- C# SqlDataAdapter Example
- C# SqlDataReader: GetInt32, GetString
- C# SqlParameter Example: Constructor, Add
- C# Stack Collection: Push, Pop
- C# Static List: Global List Variable
- C# Static Regex
- C# Static String
- C# static Keyword
- C# StatusStrip Example: Windows Forms
- C# String Chars (Get Char at Index)
- C# string.Concat Examples
- C# String Interpolation Examples
- C# string.Join Examples
- C# String Performance, Memory Usage Info
- C# String Property
- C# String Slice, Get Substring Between Indexes
- C# String Switch Examples
- C# String
- C# StringBuilder Append Performance
- C# StringBuilder Cache
- C# ToBase64String (Data URI Image)
- C# Struct Versus Class
- C# struct Examples
- C# Substring Examples
- C# Numeric Suffix Examples
- C# switch Examples
- C# String IsNormalized() method
- C# TabControl: Windows Forms
- TableLayoutPanel: Windows Forms
- C# Take and TakeWhile Examples
- C# Task Examples (Task.Run, ContinueWith and Wait)
- C# Ternary Operator
- C# Text Property: Windows Forms
- C# TextBox.AppendText Method
- C# TextBox Example
- C# TextChanged Event
- C# TextFieldParser Examples: Read CSV
- C# ThreadStart and ParameterizedThreadStart
- C# throw Keyword Examples
- C# Timer Examples
- C# TimeSpan Examples
- C# TrimEnd and TrimStart
- C# True and False
- C# Truncate String
- C# String ToLower() method
- C# String ToCharArray() method
- C# String ToUpperInvariant() method
- C# String Trim() method
- C# Assign Variables Optimization
- C# Array.Resize Examples
- C# Array.Sort: Keys, Values and Ranges
- C# Array.Reverse Example
- C# Array Slice, Get Elements Between Indexes
- C# Array.TrueForAll: Use Lambda to Test All Elements
- C# ArrayTypeMismatchException
- C# as: Cast Examples
- C# ASCII Table
- C# ASCII Transformation, Convert Char to Index
- C# AsEnumerable Method
- C# AsParallel Example
- ASP.NET AspLiteral
- C# BaseStream Property
- C# Console.Beep Example
- C# Benchmark
- C# BinaryReader Example (Use ReadInt32)
- C# BinaryWriter Type
- C# BitArray Examples
- C# BitConverter Examples
- C# Bitcount Examples
- C# Bool Methods, Return True and False
- C# bool Sort Examples (True to False)
- C# Caesar Cipher
- C# Cast Extension: System.Linq
- C# Cast to Int (Convert Double to Int)
- C# Cast Examples
- C# catch Examples
- C# Change Characters in String (ToCharArray, For Loop)
- C# Char Combine: Get String From Chars
- C# char.IsDigit (If Char Is Between 0 and 9)
- C# char.IsLower and IsUpper
- C# Character Literal (const char)
- C# Char Lowercase Optimization
- C# Char Test (If Char in String Equals a Value)
- C# char.ToLower and ToUpper
- C# char Examples
- C# abstract Keyword
- C# Action Object (Lambda That Returns Void)
- C# Aggregate: Use Lambda to Accumulate Value
- C# AggressiveInlining: MethodImpl Attribute
- C# Anagram Method
- C# And Bitwise Operator
- C# Anonymous Function (Delegate With No Name)
- C# Any Method, Returns True If Match Exists
- C# StringBuilder Append and AppendLine
- C# StringBuilder AppendFormat
- ASP.NET appSettings Example
- C# ArgumentException: Invalid Arguments
- C# Array.ConvertAll, Change Type of Elements
- C# Array.Copy Examples
- C# Array.CreateInstance Method
- C# Array and Dictionary Test, Integer Lookups
- C# Array.Exists Method, Search Arrays
- C# Array.Find Examples, Search Array With Lambda
- C# Array.ForEach: Use Lambda on Every Element
- C# Array Versus List Memory Usage
- C# Array Property, Return Empty Array
- C# CharEnumerator
- C# Chart, Windows Forms (Series and Points)
- C# CheckBox: Windows Forms
- C# class Examples
- C# Clear Dictionary: Remove All Keys
- C# Clone Examples: ICloneable Interface
- C# Closest Date (Find Dates Nearest in Time)
- C# Combine Arrays: List, Array.Copy and Buffer.BlockCopy
- C# Combine Dictionary Keys
- C# ComboBox: Windows Forms
- C# CompareTo Int Method
- C# Comparison Object, Used With Array.Sort
- C# Compress Data: GZIP
- C# Console.Read Method
- C# Console.ReadKey Example
- C# Console.ReadLine Example (While Loop)
- C# Console.SetOut and Console.SetIn
- C# Console.WindowHeight
- C# Console.Write, Append With No Newline
- C# Console.WriteLine (Print)
- C# const Example
- C# Constraint Puzzle Solver
- C# Count Characters in String
- C# Count, Dictionary (Get Number of Keys)
- C# Count Letter Frequencies
- C# Count Extension Method: Use Lambda to Count
- C# CSV Methods (Parse and Segment)
- C# DataRow Field Method: Cast DataTable Cell
- C# Get Day Count Elapsed From DateTime
- C# DateTime Format
- C# DateTime.Now (Current Time)
- C# DateTime.Today (Current Day With Zero Time)
- C# DateTime.TryParse and TryParseExact
- C# DateTime Examples
- C# DateTimePicker Example
- C# Debug.Write Examples
- C# Visual Studio Debugging Tutorial
- C# decimal Examples
- C# DayOfWeek
- C# Enum.Format Method (typeof Enum)
- C# Enum.GetName, GetNames: Get String Array From Enum
- C# Enum.Parse, TryParse: Convert String to Enum
- C# Error and Warning Directives
- C# ErrorProvider Control: Windows Forms
- C# event Examples
- C# Get Every Nth Element From List (Modulo)
- C# Excel Interop Example
- C# Except (Remove Elements From Collection)
- C# Extension Method
- C# extern alias Example
- C# Convert Feet, Inches
- C# File.Delete
- C# File Equals: Compare Files
- C# File.Exists Method
- C# try Keyword
- C# TryGetValue (Get Value From Dictionary)
- C# Tuple Examples
- C# Type Class: Returned by typeof, GetType
- C# TypeInitializationException
- C# Union: Combine and Remove Duplicate Elements
- C# Unreachable Code Detected
- C# Unsafe Keyword: Fixed, Pointers
- C# Uppercase First Letter
- C# Uri and UriBuilder Classes
- C# Using Alias Example
- C# using Statement: Dispose and IDisposable
- C# value Keyword
- C# ValueTuple Examples (System.ValueTuple, ToTuple)
- C# ValueType Examples
- C# Variable Initializer for Class Field
- C# Word Count
- C# Word Interop: Microsoft.Office.Interop.Word
- C# XElement Example (XElement.Load, XName)
- C# Zip Method (Use Lambda on Two Collections)
- C# File.Move Method, Rename File
- C# File.Open Examples
- C# File.ReadAllBytes, Get Byte Array From File
- C# File.ReadAllLines, Get String Array From File
- C# File.ReadAllText, Get String From File
- C# File.ReadLines, Use foreach Over Strings
- C# File.Replace Method
- C# FileInfo Length, Get File Size
- C# FileInfo Examples
- C# File Handling
- C# Filename With Date Example (DateTime.Now)
- C# FileNotFoundException (catch Example)
- C# FileStream Length, Get Byte Count From File
- C# FileStream Example, File.Create
- C# FileSystemWatcher Tutorial (Changed, e.Name)
- C# finally Keyword
- C# First Sentence
- C# FirstOrDefault (Get First Element If It Exists)
- C# Fisher Yates Shuffle: Generic Method
- C# fixed Keyword (unsafe)
- C# Flatten Array (Convert 2D to 1D)
- C# First Words in String
- C# First (Get Matching Element With Lambda)
- C# ContainsKey Method (Key Exists in Dictionary)
- C# Convert ArrayList to Array (Use ToArray)
- C# Convert ArrayList to List
- C# Convert Bool to Int
- C# Convert Bytes to Megabytes
- C# Convert Degrees Celsius to Fahrenheit
- C# Convert Dictionary to List
- C# Convert Dictionary to String (Write to File)
- C# Convert List to Array
- C# Convert List to DataTable (DataGridView)
- C# Convert List to String
- C# Convert Miles to Kilometers
- C# Convert Milliseconds, Seconds, Minutes
- C# Convert Nanoseconds, Microseconds, Milliseconds
- C# Convert String Array to String
- C# Convert TimeSpan to Long
- C# Convert Types
- C# Copy Dictionary
- C# Count Elements in Array and List
- C# FromOADate and Excel Dates
- C# Generic Class, Generic Method Examples
- C# GetEnumerator: While MoveNext, Get Current
- C# GetHashCode (String Method)
- C# Thumbnail Image With GetThumbnailImage
- C# GetType Method
- C# Global Variable Examples (Public Static Property)
- ASP.NET Global Variables Example
- C# Group By Operator: LINQ
- GroupBox: Windows Forms
- C# GroupBy Method: LINQ
- C# GroupJoin Method
- C# GZipStream Example (DeflateStream)
- C# HashSet Examples
- C# Hashtable Examples
- HelpProvider Control Use
- C# HTML and XML Handling
- C# HtmlEncode and HtmlDecode
- C# HtmlTextWriter Example
- C# HttpUtility.HtmlEncode Methods
- C# HybridDictionary
- C# default Operator
- C# DefaultIfEmpty Method
- C# Define and Undef Directives
- C# Destructor
- C# DialogResult: Windows Forms
- C# Dictionary, Read and Write Binary File
- C# Dictionary Memory
- C# Dictionary Optimization, Increase Capacity
- C# Dictionary Optimization, Test With ContainsKey
- C# DictionaryEntry Example (Hashtable)
- C# Directives
- C# Directory.CreateDirectory, Create New Folder
- C# Directory.GetFiles Example (Get List of Files)
- C# DivideByZeroException
- C# DllImport Attribute
- C# Do While Loop Example
- C# DriveInfo Examples
- C# DropDownItems Control
- C# IComparable Example, CompareTo: Sort Objects
- C# IDictionary Generic Interface
- C# IEnumerable Examples
- C# IList Generic Interface: List and Array
- C# Image Type
- C# ImageList Use: Windows Forms
- C# Increment String That Contains a Number
- C# Increment, Preincrement and Decrement Ints
- Dot Net Perls
- C# Indexer Examples (This Keyword, get and set)
- C# IndexOutOfRangeException
- C# Inheritance
- C# Insert String Examples
- C# int Array
- C# Interface Examples
- C# Interlocked Examples: Add, CompareExchange
- C# Intersect: Get Common Elements
- C# InvalidCastException
- C# InvalidOperationException: Collection Was Modified
- C# IOException Type: File, Directory Not Found
- C# IOrderedEnumerable (Query Expression With orderby)
- C# is: Cast Examples
- C# IsFixedSize, IsReadOnly and IsSynchronized Arrays
- C# string.IsNullOrEmpty, IsNullOrWhiteSpace
- C# IsSorted Method: If Array Is Already Sorted
- C# Jagged Array Examples
- C# join Examples (LINQ)
- C# KeyCode Property and KeyDown
- C# KeyNotFoundException: Key Not Present in Dictionary
- C# KeyValuePair Examples
- C# Line Count for File
- C# Line Directive
- C# LinkedList
- C# List CopyTo (Copy List Elements to Array)
- C# List Equals (If Elements Are the Same)
- C# List Find and Exists Examples
- C# List Insert Performance
- ASP.NET LiteralControl Example
- C# Locality Optimizations (Memory Hierarchy)
- C# lock Keyword
- C# Long and ulong Types
- C# Loop Over String Chars: Foreach, For
- C# Loop Over String Array
- C# Main args Examples
- C# Map Example
- ASP.NET MapPath: Virtual and Physical Paths
- C# Mask Optimization
- C# MaskedTextBox Example
- C# Math.Abs: Absolute Value
- C# Math.Ceiling Usage
- C# Math.Floor Method
- C# Math.Max and Math.Min Examples
- C# Math.Pow Method, Exponents
- C# Math.Round Examples: MidpointRounding
- C# Math Type
- C# Max and Min: Get Highest or Lowest Element
- C# MemoryFailPoint and InsufficientMemoryException
- C# MemoryStream: Use Stream on Byte Array
- C# MenuStrip: Windows Forms
- C# Modulo Operator: Get Remainder From Division
- C# MonthCalendar Control: Windows Forms
- C# Multiple Return Values
- C# Multiply Numbers
- C# namespace Keyword
- C# NameValueCollection Usage
- C# Nested Lists: Create 2D List or Jagged List
- C# Nested Switch Statement
- C# Environment.NewLine
- C# Normalize, IsNormalized Methods
- C# Null String Example
- C# null Keyword
- C# Nullable Examples
- C# NullReferenceException and Null Parameter
- C# Object Array
- C# Obsolete Attribute
- C# OfType Examples
- C# OpenFileDialog Example
- C# operator Keyword
- C# Odd and Even Numbers
- C# Bitwise Or
- C# orderby Query Keyword
- C# out Parameter
- C# OutOfMemoryException
- C# OverflowException
- C# Overload Method
- C# Override Method
- C# PadRight and PadLeft: String Columns
- C# Get Paragraph From HTML With Regex
- C# Parallel.For Example (Benchmark)
- C# Parallel.Invoke: Run Methods on Separate Threads
- C# Parameter Optimization
- C# Parameter Passing, ref and out
- C# params Keyword
- C# int.Parse: Convert Strings to Integers
- C# partial Keyword
- C# Path.ChangeExtension
- C# Path Exists Example
- C# Path.GetExtension: File Extension
- C# Path.GetRandomFileName Method
- C# Pragma Directive
- C# Predicate (Lambda That Returns True or False)
- C# Pretty Date Format (Hours or Minutes Ago)
- C# PreviewKeyDown Event
- C# Random Lowercase Letter
- C# Random Paragraphs and Sentences
- C# Random String
- C# Random Number Examples
- C# StreamReader ReadLine, ReadLineAsync Examples
- C# readonly Keyword
- C# Recursion Example
- C# Regex, Read and Match File Lines
- C# Regex Groups, Named Group Example
- C# Regex.Matches Quote Example
- C# Regex.Matches Method: foreach Match, Capture
- C# Regex.Replace, Matching End of String
- C# Regex.Replace, Remove Numbers From String
- C# Regex.Replace, Merge Multiple Spaces
- C# Regex.Replace Examples: MatchEvaluator
- C# Regex.Split, Get Numbers From String
- C# Regex.Split Examples
- C# Regex Trim, Remove Start and End Spaces
- C# RegexOptions.Compiled
- C# RegexOptions.IgnoreCase Example
- C# RegexOptions.Multiline
- C# Region and endregion
- C# Remove Char From String at Index
- C# Remove Element
- C# Remove HTML Tags
- C# Remove String
- C# Reserved Filenames
- C# return Keyword
- C# Reverse String
- C# Reverse Words
- C# Reverse Extension Method
- C# RichTextBox Example
- C# Right String Part
- C# RNGCryptoServiceProvider Example
- C# ROT13 Method, Char Lookup Table
- C# SelectMany Example: LINQ
- C# Sentinel Optimization
- C# SequenceEqual Method (If Two Arrays Are Equal)
- C# Shift Operators (Bitwise)
- C# Short and ushort Types
- C# Single Method: Get Element If Only One Matches
- C# SingleOrDefault
- C# Singleton Pattern Versus Static Class
- C# Singleton Class
- C# sizeof Keyword
- C# Skip and SkipWhile Examples
- C# Sleep Method (Pause)
- C# Sort Dictionary: Keys and Values
- C# Sort by File Size
- C# Sort, Ignore Leading Chars
- C# Sort KeyValuePair List: CompareTo
- C# Sort Strings by Length
- C# Thread.SpinWait Example
- C# Math.Sqrt Method
- C# stackalloc Operator
- C# StackOverflowException
- C# StartsWith and EndsWith String Methods
- C# Static Array
- C# Static Dictionary
- C# Stopwatch Examples
- C# Stream
- C# StreamReader ReadToEnd Example (Read Entire File)
- C# StreamReader ReadToEndAsync Example (Performance)
- C# StreamReader Examples
- C# StreamWriter Examples
- C# String Append (Add Strings)
- C# String Compare and CompareTo Methods
- C# String Constructor (new string)
- C# string.Copy Method
- C# CopyTo String Method: Put Chars in Array
- C# Empty String Examples
- C# String Equals Examples
- C# String For Loop, Count Spaces in String
- C# string.Intern and IsInterned
- C# String IsUpper, IsLower
- C# String Length Property: Get Character Count
- C# String Literal: Newline and Quote Examples
- C# StringBuilder Capacity
- C# StringBuilder Clear (Set Length to Zero)
- C# StringBuilder Data Types
- C# StringBuilder Performance
- C# StringBuilder Equals (If Chars Are Equal)
- C# StringBuilder Memory
- C# StringBuilder ToString: Get String From StringBuilder
- C# StringWriter Class
- C# Sum Method: Add up All Numbers
- C# Switch Char, Test Chars With Cases
- C# Switch Enum
- C# System (using System namespace)
- C# Tag Property: Windows Forms
- C# TextInfo Examples
- C# TextReader, Returned by File.OpenText
- C# TextWriter, Returned by File.CreateText
- C# this Keyword
- C# ThreadPool
- C# Thread Join Method (Join Array of Threads)
- C# ThreadPool.SetMinThreads Method
- C# TimeZone Examples
- C# Get Title From HTML With Regex
- C# ToArray Extension Method
- C# ToCharArray: Convert String to Array
- C# ToDictionary Method
- C# Token
- C# ToList Extension Method
- C# ToLookup Method (Get ILookup)
- C# ToLower and ToUpper: Uppercase and Lowercase Strings
- ToolStripContainer Control: Dock, Properties
- C# ToolTip: Windows Forms
- C# ToString Integer Optimization
- C# ToString: Get String From Object
- C# ToTitleCase Method
- C# TrackBar: Windows Forms
- C# Tree and Nodes Example: Directed Acyclic Word Graph
- C# TreeView Tutorial
- C# Trim Strings
- C# Thread Methods
- C# History
- C# Features
- C# Variables
- C# Data Types
- C# Operators
- C# Keywords
- C# New Features | C# Version Features
- C# Programs
- C# Program to swap numbers without third variable
- C# Program to convert Decimal to Binary
- C# Program to Convert Number in Characters
- C# Program to Print Alphabet Triangle
- C# Program to print Number Triangle
- C# Program to generate Fibonacci Triangle
- C# String Compare() method
- C# String CompareOrdinal() method
- C# String CompareTo() method
- C# String Concat() method
- C# String Contains() method
- C# String CopyTo() method
- C# String EndsWith() method
- C# String Equals() method
- C# String Format() method
- C# String IndexOf() method
- C# String Insert() method
- C# String Intern(String str) method
- C# String IsInterned() method
- C# String Normalize() method
- C# String IsNullOrEmpty() method
- C# String IsNullOrWhiteSpace() method
- C# String Join() method
- C# String LastIndexOf() method
- C# String LastIndexOfAny() method
- C# String PadLeft() method
- C# String PadRight() method
- C# Nullable
- C# String Remove() method
- C# String Replace() method
- C# String Split() method
- C# String StartsWith() method
- C# String SubString() method
- C# Partial Types
- C# Iterators
- C# Delegate Covariance
- C# Delegate Inference
- C# Anonymous Types
- C# Extension Methods
- C# Query Expression
- C# Partial Method
- C# Implicitly Typed Local Variable
- C# Object and Collection Initializer
- C# Auto Implemented Properties
- C# Dynamic Binding
- C# Named and Optional Arguments
- C# Asynchronous Methods
- C# Caller Info Attributes
- C# Using Static Directive
- C# Exception Filters
- C# Await in Catch Finally Blocks
- C# Default Values for Getter Only Properties
- C# Expression Bodied Members
- C# Null Propagator
- C# String Interpolation
- C# nameof operator
- C# Dictionary Initializer
- C# Pattern Matching
- C# Tuples
- C# Deconstruction
- C# Local Functions
- C# Binary Literals
- C# Ref Returns and Locals
- C# Expression Bodied Constructors and Finalizers
- C# Expression Bodied Getters and Setters
- C# Async Main
- C# Default Expression
Related Links
Adjectives
Ado
Ai
Android
Angular
Antonyms
Apache
Articles
Asp
Autocad
Automata
Aws
Azure
Basic
Binary
Bitcoin
Blockchain
C
Cassandra
Change
Coa
Computer
Control
Cpp
Create
Creating
C-Sharp
Cyber
Daa
Data
Dbms
Deletion
Devops
Difference
Discrete
Es6
Ethical
Examples
Features
Firebase
Flutter
Fs
Git
Go
Hbase
History
Hive
Hiveql
How
Html
Idioms
Insertion
Installing
Ios
Java
Joomla
Js
Kafka
Kali
Laravel
Logical
Machine
Matlab
Matrix
Mongodb
Mysql
One
Opencv
Oracle
Ordering
Os
Pandas
Php
Pig
Pl
Postgresql
Powershell
Prepositions
Program
Python
React
Ruby
Scala
Selecting
Selenium
Sentence
Seo
Sharepoint
Software
Spellings
Spotting
Spring
Sql
Sqlite
Sqoop
Svn
Swift
Synonyms
Talend
Testng
Types
Uml
Unity
Vbnet
Verbal
Webdriver
What
Wpf