ASP.NET Razor HTML Helpers
HtmlHelper is a class which is introduced in MVC 2. It is used to create HTML controls programmatically. It provides built-in methods to generate controls on the view page. In this topic we have tabled constructors, properties and methods of this class. At the end, we have explained an example that creates a form with the help of extension methods.
Note: The HtmlHelper class is designed to generate UI. It should not be used in controllers or models class.
Constructors
Following are the constructors of HtmlHelper class.
Constructor Name |
Description |
HtmlHelper(ViewContext, IViewDataContainer) |
It Initializes a new instance of the HtmlHelper class by using the specified view context and view data container. |
HtmlHelper(ViewContext, IViewDataContainer, RouteCollection) |
It Initializes a new instance of the HtmlHelper class by using the specified view context, view data container, and route collection. |
Properties
Name |
Description |
RouteCollection |
It is used to get or set the collection of routes for the application. |
ViewBag |
It is used to get the view bag. |
ViewContext |
It is used to get or set the context information about the view. |
ViewData |
It is used to get the current view data dictionary. |
ViewDataContainer |
It is used to get or set the view data container. |
HtmlHelper Extension Methods
Name |
Description |
Overloaded Methods |
Action(String) |
It is used to invoke the specified child action method and returns the result as an HTML string. |
Action(String, Object)
Action(String, RouteValueDictionary)
Action(String, String)
Action(String, String, Object)
Action(String, String, RouteValueDictionary)
|
BeginForm() |
It is used to generate an opening <form> tag. The form uses the POST method. |
BeginForm(Object)
BeginForm(RouteValueDictionary)
BeginForm(String, String)
BeginForm(String, String, FormMethod)
BeginForm(String, String, FormMethod, IDictionary<String, Object>)
BeginForm(String, String, FormMethod, Object)
BeginForm(String, String, Object)
BeginForm(String, String, Object, FormMethod)
BeginForm(String, String, Object, FormMethod, Object)
BeginForm(String, String, RouteValueDictionary)
BeginForm(String, String, RouteValueDictionary, FormMethod)
BeginForm(String, String, RouteValueDictionary, FormMethod, IDictionary<String, Object>)
|
CheckBox(String) |
It is used to generate a check box input element by using the specified HTML helper and the name of the form field. |
CheckBox(String, Boolean)
CheckBox(String, Boolean, IDictionary<String, Object>)
CheckBox(String, Boolean, Object)
CheckBox(String, IDictionary<String, Object>)
CheckBox(String, Object)
|
DropDownList(String) |
It generates a single-selection select element using the specified HTML helper and the name of the form field. |
DropDownList(String, IEnumerable<SelectListItem>)
DropDownList(String, IEnumerable<SelectListItem>, IDictionary<String, Object>)
DropDownList(String, IEnumerable<SelectListItem>, Object)
DropDownList(String, IEnumerable<SelectListItem>, String)
DropDownList(String, IEnumerable<SelectListItem>, String, IDictionary<String, Object>)
DropDownList(String, IEnumerable<SelectListItem>, String, Object)
DropDownList(String, String)
|
Editor(String) |
It generates an HTML input element for each property in the object that is represented by the expression. |
Editor(String, Object)
Editor(String, String)
Editor(String, String, Object)
Editor(String, String, String)
Editor(String, String, String, Object)
|
EndForm() |
It renders the closing </form> tag. |
|
Label(String) |
It generates an HTML label element. |
Label(String, IDictionary<String, Object>)
Label(String, Object)
Label(String, String)
Label(String, String, IDictionary<String, Object>)
Label(String, String, Object)
|
ListBox(String) |
It returns a multi-select select element using the specified HTML helper. |
ListBox(String, IEnumerable<SelectListItem>)
ListBox(String, IEnumerable<SelectListItem>, IDictionary<String, Object>)
ListBox(String, IEnumerable<SelectListItem>, Object)
|
Password(String) |
It is used to generate a password input element by using the specified HTML helper. |
Password(String, Object)
Password(String, Object, IDictionary<String, Object>)
Password(String, Object, Object)
|
RadioButton(String, Object) |
It returns a radio button input element. |
RadioButton(String, Object, Boolean)
RadioButton(String, Object, Boolean, IDictionary<String, Object>)
RadioButton(String, Object, Boolean, Object)
RadioButton(String, Object, IDictionary<String, Object>)
RadioButton(String, Object, Object)
|
TextArea(String) |
It is used to create a text area to the web page. |
TextArea(String, IDictionary<String, Object>)
TextArea(String, Object)
TextArea(String, String)
TextArea(String, String, IDictionary<String, Object>)
TextArea(String, String, Int32, Int32, IDictionary<String, Object>)
TextArea(String, String, Int32, Int32, Object)
TextArea(String, String, Object)
|
TextBox(String) |
It is used to return a text input element by using the specified HTML helper. |
TextBox(String, Object)
TextBox(String, Object, IDictionary<String, Object>)
TextBox(String, Object, Object)
TextBox(String, Object, String)
TextBox(String, Object, String, IDictionary<String, Object>)
TextBox(String, Object, String, Object)
|
Example
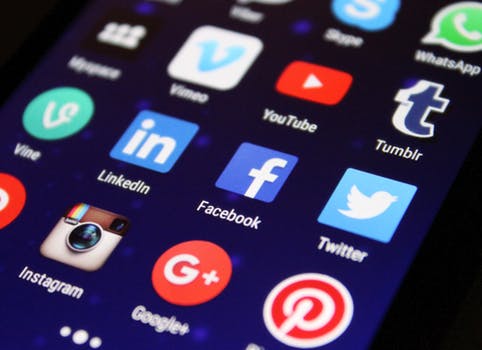
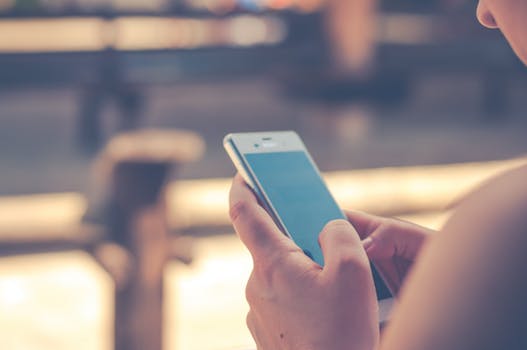
Because this is a MVC application, so, it includes Model, View and Controller. This example includes the following files.
View
// HtmlHelperDemo.cshtml
@{
ViewBag.Title = "HtmlHelperDemo";
}
<hr />
<h3>User Registration Form</h3>
<hr />
<div class="form-horizontal">
@using (Html.BeginForm("UserRegistration", "Students"))
{
<div class="form-group">
@Html.Label("User Name", new { @class = "control-label col-sm-2" })
<div class="col-sm-10">
@Html.TextBox("username", null, new { @class = "form-control" })
</div>
</div>
<div class="form-group">
@Html.Label("Email ID", new { @class = "control-label col-sm-2" })
<div class="col-sm-10">
@Html.TextBox("email", null, new { @class = "form-control" })
</div>
</div>
<div class="form-group">
@Html.Label("Gender", new { @class = "control-label col-sm-2" })
<div>
<div class="col-sm-10">
Male @Html.RadioButton("Gender", "male")
Female @Html.RadioButton("Gender", "female")
</div>
</div>
</div>
<div class="form-group">
@Html.Label("Courses", new { @class = "control-label col-sm-2" })
<div class="col-sm-10">
C# @Html.CheckBox("C#", new { value = "C#" })
ASP.NET @Html.CheckBox("ASP.NET", new { value = "ASP.NET" })
ADO.NET @Html.CheckBox("ADO.NET", new { value = "ADO.NET" })
</div>
</div>
<div class="form-group">
@Html.Label("Contact", new { @class = "control-label col-sm-2" })
<div class="col-sm-10">
@Html.TextBox("contact", null, new { @class = "form-control" })
</div>
</div>
<div class="form-group">
<div class="col-sm-2"></div>
<div class="col-sm-10">
<input type="submit" value="submit" class="btn btn-primary" />
</div>
</div>
}
</div>
Controller
// StudentsController.cs
using System.Web.Mvc;
namespace MvcApplicationDemo.Controllers
{
public class StudentsController : Controller
{
public ActionResult Index()
{
return View();
}
public ActionResult HtmlHelperDemo()
{
return View();
}
[HttpPost]
public ContentResult UserRegistration()
{
return Content(
"User Name = " + Request.Form["username"] + " " +
"Email ID = " + Request.Form["email"] + " " +
"Gender = " + Request.Form["gender"] + " " +
"Courses = " + Request.Form.GetValues("C#")[0] + " " + Request.Form.GetValues("ASP.NET")[0] + " " + Request.Form.GetValues("ADO.NET")[0] + " " +
"Contact = " + Request.Form["contact"] + " "
);
}
}
}
Output:
We are submitting this form with the following details.
After submitting, we are receiving all the values in action method.
|