PHP Operators
PHP Operator is a symbol i.e used to perform operations on operands. In simple words, operators are used to perform operations on variables or values. For example:
$num=10+20;//+ is the operator and 10,20 are operands
In the above example, + is the binary + operator, 10 and 20 are operands and $num is variable.
PHP Operators can be categorized in following forms:
We can also categorize operators on behalf of operands. They can be categorized in 3 forms:
- Unary Operators: works on single operands such as ++, -- etc.
- Binary Operators: works on two operands such as binary +, -, *, / etc.
- Ternary Operators: works on three operands such as "?:".
Arithmetic Operators
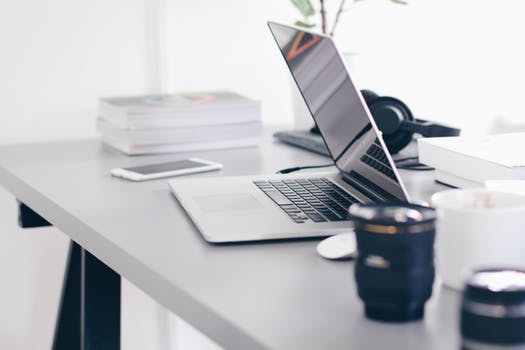
The PHP arithmetic operators are used to perform common arithmetic operations such as addition, subtraction, etc. with numeric values.
Operator |
Name |
Example |
Explanation |
+ |
Addition |
$a + $b |
Sum of operands |
- |
Subtraction |
$a - $b |
Difference of operands |
* |
Multiplication |
$a * $b |
Product of operands |
/ |
Division |
$a / $b |
Quotient of operands |
% |
Modulus |
$a % $b |
Remainder of operands |
** |
Exponentiation |
$a ** $b |
$a raised to the power $b |
The exponentiation (**) operator has been introduced in PHP 5.6.
Assignment Operators
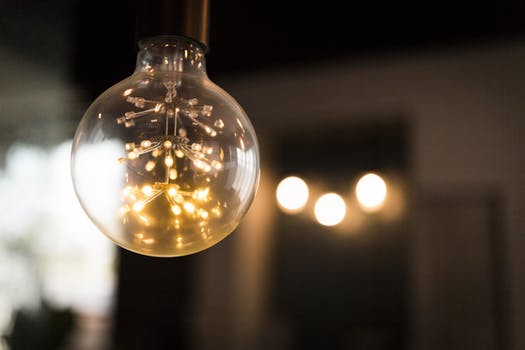
The assignment operators are used to assign value to different variables. The basic assignment operator is "=".
Operator |
Name |
Example |
Explanation |
= |
Assign |
$a = $b |
The value of right operand is assigned to the left operand. |
+= |
Add then Assign |
$a += $b |
Addition same as $a = $a + $b |
-= |
Subtract then Assign |
$a -= $b |
Subtraction same as $a = $a - $b |
*= |
Multiply then Assign |
$a *= $b |
Multiplication same as $a = $a * $b |
/= |
Divide then Assign
(quotient) |
$a /= $b |
Find quotient same as $a = $a / $b |
%= |
Divide then Assign
(remainder) |
$a %= $b |
Find remainder same as $a = $a % $b |
Bitwise Operators
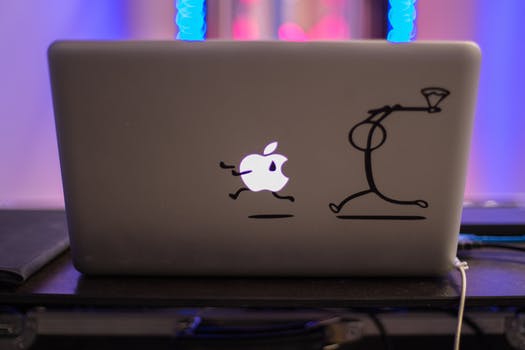
The bitwise operators are used to perform bit-level operations on operands. These operators allow the evaluation and manipulation of specific bits within the integer.
Operator |
Name |
Example |
Explanation |
& |
And |
$a & $b |
Bits that are 1 in both $a and $b are set to 1, otherwise 0. |
| |
Or (Inclusive or) |
$a | $b |
Bits that are 1 in either $a or $b are set to 1 |
^ |
Xor (Exclusive or) |
$a ^ $b |
Bits that are 1 in either $a or $b are set to 0. |
~ |
Not |
~$a |
Bits that are 1 set to 0 and bits that are 0 are set to 1 |
<< |
Shift left |
$a << $b |
Left shift the bits of operand $a $b steps |
>> |
Shift right |
$a >> $b |
Right shift the bits of $a operand by $b number of places |
Comparison Operators
Comparison operators allow comparing two values, such as number or string. Below the list of comparison operators are given:
Operator |
Name |
Example |
Explanation |
== |
Equal |
$a == $b |
Return TRUE if $a is equal to $b |
=== |
Identical |
$a === $b |
Return TRUE if $a is equal to $b, and they are of same data type |
!== |
Not identical |
$a !== $b |
Return TRUE if $a is not equal to $b, and they are not of same data type |
!= |
Not equal |
$a != $b |
Return TRUE if $a is not equal to $b |
<> |
Not equal |
$a <> $b |
Return TRUE if $a is not equal to $b |
< |
Less than |
$a < $b |
Return TRUE if $a is less than $b |
> |
Greater than |
$a > $b |
Return TRUE if $a is greater than $b |
<= |
Less than or equal to |
$a <= $b |
Return TRUE if $a is less than or equal $b |
>= |
Greater than or equal to |
$a >= $b |
Return TRUE if $a is greater than or equal $b |
<=> |
Spaceship |
$a <=>$b |
Return -1 if $a is less than $b
Return 0 if $a is equal $b
Return 1 if $a is greater than $b |
Incrementing/Decrementing Operators
The increment and decrement operators are used to increase and decrease the value of a variable.
Operator |
Name |
Example |
Explanation |
++ |
Increment |
++$a |
Increment the value of $a by one, then return $a |
$a++ |
Return $a, then increment the value of $a by one |
-- |
decrement |
--$a |
Decrement the value of $a by one, then return $a |
$a-- |
Return $a, then decrement the value of $a by one |
Logical Operators
The logical operators are used to perform bit-level operations on operands. These operators allow the evaluation and manipulation of specific bits within the integer.
Operator |
Name |
Example |
Explanation |
and |
And |
$a and $b |
Return TRUE if both $a and $b are true |
Or |
Or |
$a or $b |
Return TRUE if either $a or $b is true |
xor |
Xor |
$a xor $b |
Return TRUE if either $ or $b is true but not both |
! |
Not |
! $a |
Return TRUE if $a is not true |
&& |
And |
$a && $b |
Return TRUE if either $a and $b are true |
|| |
Or |
$a || $b |
Return TRUE if either $a or $b is true |
String Operators
The string operators are used to perform the operation on strings. There are two string operators in PHP, which are given below:
Operator |
Name |
Example |
Explanation |
. |
Concatenation |
$a . $b |
Concatenate both $a and $b |
.= |
Concatenation and Assignment |
$a .= $b |
First concatenate $a and $b, then assign the concatenated string to $a, e.g. $a = $a . $b |
Array Operators
The array operators are used in case of array. Basically, these operators are used to compare the values of arrays.
Operator |
Name |
Example |
Explanation |
+ |
Union |
$a + $y |
Union of $a and $b |
== |
Equality |
$a == $b |
Return TRUE if $a and $b have same key/value pair |
!= |
Inequality |
$a != $b |
Return TRUE if $a is not equal to $b |
=== |
Identity |
$a === $b |
Return TRUE if $a and $b have same key/value pair of same type in same order |
!== |
Non-Identity |
$a !== $b |
Return TRUE if $a is not identical to $b |
<> |
Inequality |
$a <> $b |
Return TRUE if $a is not equal to $b |
Type Operators
The type operator instanceof is used to determine whether an object, its parent and its derived class are the same type or not. Basically, this operator determines which certain class the object belongs to. It is used in object-oriented programming.
<?php
//class declaration
class Developer
{}
class Programmer
{}
//creating an object of type Developer
$charu = new Developer();
//testing the type of object
if( $charu instanceof Developer)
{
echo "Charu is a developer.";
}
else
{
echo "Charu is a programmer.";
}
echo "</br>";
var_dump($charu instanceof Developer); //It will return true.
var_dump($charu instanceof Programmer); //It will return false.
?>
Output:
Charu is a developer.
bool(true) bool(false)
Execution Operators
PHP has an execution operator backticks (``). PHP executes the content of backticks as a shell command. Execution operator and shell_exec() give the same result.
Operator |
Name |
Example |
Explanation |
`` |
backticks |
echo `dir`; |
Execute the shell command and return the result.
Here, it will show the directories available in current folder. |
Note: Note that backticks (``) are not single-quotes.
Error Control Operators
PHP has one error control operator, i.e., at (@) symbol. Whenever it is used with an expression, any error message will be ignored that might be generated by that expression.
Operator |
Name |
Example |
Explanation |
@ |
at |
@file ('non_existent_file') |
Intentional file error |
PHP Operators Precedence
Let's see the precedence of PHP operators with associativity.
Operators | Additional Information | Associativity |
clone new | clone and new | non-associative |
[ | array() | left |
** | arithmetic | right |
++ -- ~ (int) (float) (string) (array) (object) (bool) @ | increment/decrement and types | right |
instanceof | types | non-associative |
! | logical (negation) | right |
* / % | arithmetic | left |
+ - . | arithmetic and string concatenation | left |
<< >> | bitwise (shift) | left |
< <= > >= | comparison | non-associative |
== != === !== <> | comparison | non-associative |
& | bitwise AND | left |
^ | bitwise XOR | left |
| | bitwise OR | left |
&& | logical AND | left |
|| | logical OR | left |
?: | ternary | left |
= += -= *= **= /= .= %= &= |= ^= <<= >>= => | assignment | right |
and | logical | left |
xor | logical | left |
or | logical | left |
, | many uses (comma) | left |
|