<< Back to RUBY
Ruby Console: Puts, Print and stdin
Use the console window. Call the puts, p, print and stdin.readline methods.Console. Programs are often run with console input and output. We use the puts, print and p methods in Ruby to display text (or other data types).
With stdin, we handle input from the console. Operators like << are helpful. And often a loop is used to create an interactive console program with a prompt.
An example. We begin with a simple program. Here the puts method writes a line to the console window. It writes each argument to a separate line.
Tip: To combine multiple parts on a single line, we can concatenate strings. We use << or + for this.
String: It sometimes is necessary to use the String constructor to convert integers to strings.
Array: When displaying an array, puts will place each element onto a separate line.
Ruby program that uses puts
value = 100
# Print values on separate lines.
# ... Parentheses are optional.
puts value
puts("FINISHED")
# Use << to append a string.
puts "VALUE " << String(value)
# Use + to append.
puts "VALUE " + String(value)
# Print all Array elements on separate lines.
elements = [10, 100, 1000]
puts elements
Output
100
FINISHED
VALUE 100
VALUE 100
10
100
1000
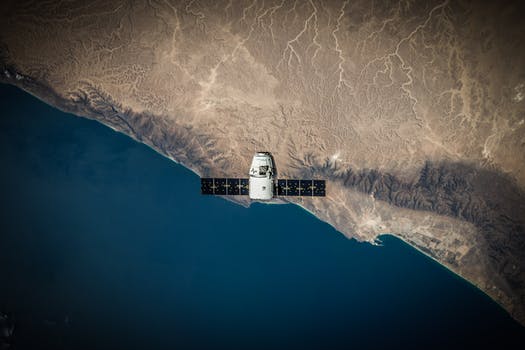
P method. Let us continue with the P method. This one is good for lazy programmers. It displays data in a literal way—it includes quotes around a string, for example.
And: P displays an Array or Hash on a single line. This is convenient and often a good choice.
Tip: As with other methods in Ruby, parentheses are optional. Usually "p" is used without parentheses.
Ruby program that uses p method
# Use p method.
p "cat"
# Write an array.
items = [5, 55, 555]
p items
# Write a hash.
lookup = {"cat" => 4, "bird" => 2}
p lookup
# Use p to write two strings on one line.
part1 = "HELLO"
part2 = "WORLD"
p part1 << "... " << part2
# Nil is displayed as nil.
p(nil)
Output
"cat"
[5, 55, 555]
{"cat"=>4, "bird"=>2}
"HELLO... WORLD"
nil
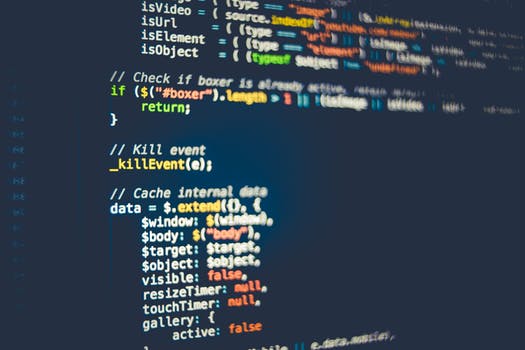
Print. This method appends no newlines to text. We can thus use many print statements, one after another, on a single line. But we must also print a newline manually.
Tip: Print() is a good choice for lines that are built up in many steps. We can avoid concatenating strings ourselves.
Ruby program that uses print
# Print statements on the same line.
print "dog"
print " is cute"
print "\n"
# Print entire-line statements.
print "There are " << String(4) << " apples.\n"
print "I ate a lemon.\n"
# Print a multiline statement.
print "***\nYou are a winner!\n***\n"
Output
dog is cute
There are 4 apples.
I ate a lemon.
***
You are a winner!
***
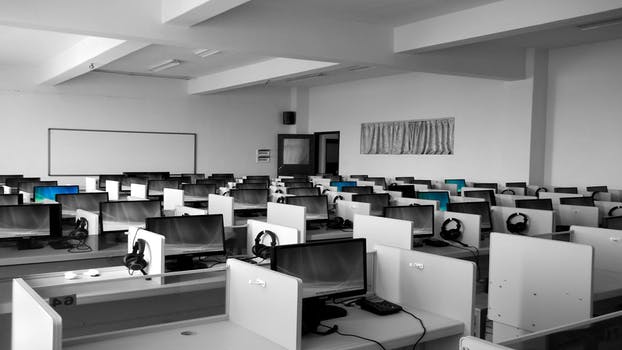
Stdin. We can read a line from the console with $stdin.readline. This method returns a string. The stdin source can be configured but by default it is set to the keyboard on the console.
Ruby program that uses stdin
# Read line from console window.
line = $stdin.readline()
# Display the string.
puts "You typed: " << line
Output
cat
You typed: cat
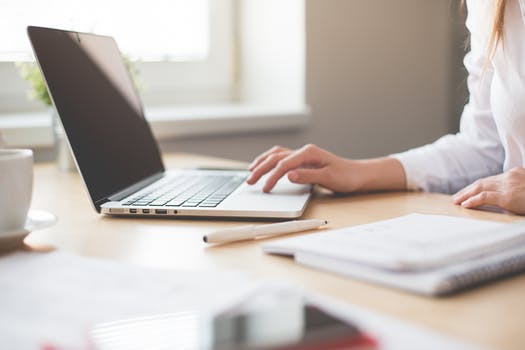
Interactive. An interactive console program can be developed with $stdin.readline. First we enter a while-true loop—this continues indefinitely.
Begin: We use a begin, rescue, ensure construct to handle errors and run some code after the selection is made.
Integer: We convert the text entered by the user into an Integer. This makes it easier to test for values.
IntegerCase: The case statement tests the integer we received. We handle the values 0 through 3 in special ways.
CaseRuby program that uses stdin, interactive loop
while true
print "Type a number: "
line = $stdin.readline()
begin
# Convert string to integer.
number = Integer(line)
# Handle various cases.
case number
when 0
puts "Zero"
when 1, 2, 3
puts "One to three"
else
puts "Other"
end
rescue
# Let the loop continue.
puts "Invalid"
ensure
puts "Done"
end
end
Output
Type a number: 0
Zero
Done
Type a number: 2
One to three
Done
Type a number: X
Invalid
Done
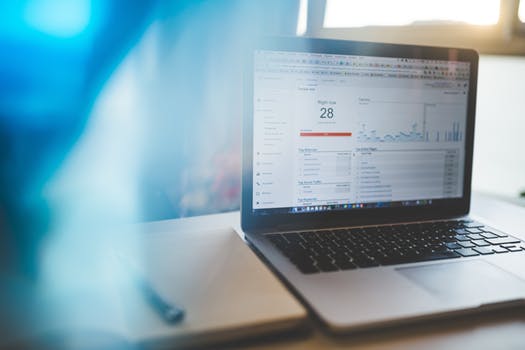
A summary. Ruby, as an interpreted language, is well-suited to developer or server tasks. For user programs, a compiled language is often a better choice.
But for developers, console programs are easy-to-maintain and efficient. With print(), puts() and the concise P method, we easily output text to an output stream.
Related Links:
- Ruby Convert Types: Arrays and Strings
- Ruby File Handling: File and IO Classes
- Ruby Regexp Match Method
- Ruby String Array Examples
- Ruby include: Array Contains Method
- Ruby Class Examples: Self, Super and Module
- Ruby DateTime Examples: require date
- Ruby 2D Array Examples
- Ruby Number Examples: Integer, Float, zero and eql
- Ruby Exception Examples: Begin and Rescue
- Top 65 Ruby Interview Questions (2021)
- Ruby on Rails Interview Questions (2021)
- Ruby String Examples (each char, each line)
- Ruby if Examples: elsif, else and unless
- Ruby Math Examples: floor, ceil, round and truncate
- Ruby Sub, gsub: Replace String
- Ruby Substring Examples
- Ruby Console: Puts, Print and stdin
- Ruby Remove Duplicates From Array
- Ruby Random Number Generator: rand, srand
- Ruby Recursion Example
- Ruby ROT13 Method
- Ruby Iterator: times, step Loops
- Ruby String Length, For Loop Over Chars
- Ruby Join Example (Convert Array to String)
- Ruby Format String Examples
- Ruby Copy Array Example
- Ruby Keywords
- Ruby Nil Value Examples: NoMethodError
- Learn Ruby Tutorial
- Ruby Method: Def, Arguments and Return Values
- Ruby Fibonacci Sequence Example
- Ruby Hash Examples
- Ruby While, Until and For Loop Examples
- Learn Ruby on Rails Tutorial
- Ruby Word Count: Split Method
- Ruby Sort Arrays (Use Block Syntax)
- Ruby Case Examples: Ranges, Strings and Regexp
- Ruby Array Examples
- Ruby Split String Examples
Related Links
Adjectives
Ado
Ai
Android
Angular
Antonyms
Apache
Articles
Asp
Autocad
Automata
Aws
Azure
Basic
Binary
Bitcoin
Blockchain
C
Cassandra
Change
Coa
Computer
Control
Cpp
Create
Creating
C-Sharp
Cyber
Daa
Data
Dbms
Deletion
Devops
Difference
Discrete
Es6
Ethical
Examples
Features
Firebase
Flutter
Fs
Git
Go
Hbase
History
Hive
Hiveql
How
Html
Idioms
Insertion
Installing
Ios
Java
Joomla
Js
Kafka
Kali
Laravel
Logical
Machine
Matlab
Matrix
Mongodb
Mysql
One
Opencv
Oracle
Ordering
Os
Pandas
Php
Pig
Pl
Postgresql
Powershell
Prepositions
Program
Python
React
Ruby
Scala
Selecting
Selenium
Sentence
Seo
Sharepoint
Software
Spellings
Spotting
Spring
Sql
Sqlite
Sqoop
Svn
Swift
Synonyms
Talend
Testng
Types
Uml
Unity
Vbnet
Verbal
Webdriver
What
Wpf