<< Back to RUBY
Ruby Format String Examples
Use the format string syntax. See examples for formatting values in many ways.Format. A string can be created with concatenation. This sometimes requires many statements. It can be complex. With a format string we build more complex strings.
A syntax form. In Ruby we apply the string format syntax (the "%" operator) to ease this creation of formatted string data. After the "%" we specify arguments.
A first example. We use the percentage sign ("%") to specify a format code within a string. And we also apply the "%" sign after the string, to indicate the arguments.
One argument: In the first format example, we use one argument. We insert the 12 as a decimal number into the "%d" code.
Two arguments: We next use two arguments. We use a "%d" and the "%s" string code. We specify the two values in an array.
Ruby program that formats string
# Use digit format.
format = "Number is %d" % 12
puts format
# Use two formatting codes.
format = "Number is %d, type is %s" % [13, "cat"]
puts format
Output
Number is 12
Number is 13, type is cat
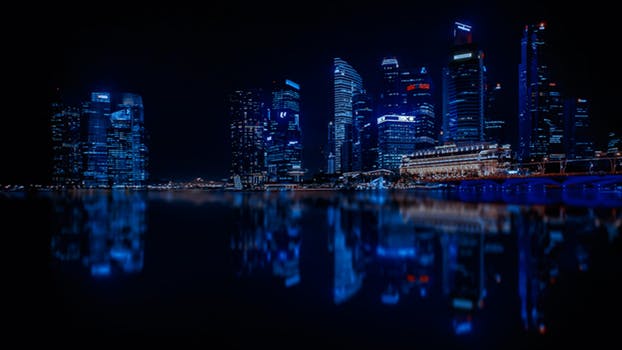
Padding. A format string can pad strings. We specify the total width of the desired string. And an amount of padding (space characters) is added on the left or right side to fit the size.
Positive: With a positive padding, the string is aligned to the right. To have a 10 char string, use the code "%10s."
Negative: A negative padding adds whitespace to the right. Try the code "%-10s" to pad to ten characters.
Ruby program that pads string
# Align string to the right (pad the left).
right = "[%10s]" % "carrot"
puts right
# Align to the left.
left = "[%-10s]" % "carrot"
puts left
Output
[ carrot]
[carrot ]
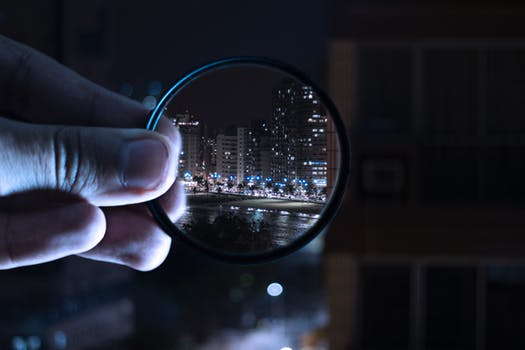
Floating-point. Often when representing floating-point numbers as strings, we want to control the number of digits past the decimal.
And: With format syntax, we can use a "precision" like ".2" after the percentage mark and before an "f."
Tip: The precision, like 2, indicates the number of post-decimal digits—so we get "12.35."
Rounding: This operation rounds numbers, so 12.345 is rounded to 12.34. We can specify greater precisions.
Ruby program that formats floating-point numbers
# Use two digits after the decimal place for floating-point.
result = "Result is %.2f" % 12.3456
p result
# Use three digits.
result = "Result is %.3f" % 12.3456
p result
Output
"Result is 12.35"
"Result is 12.346"
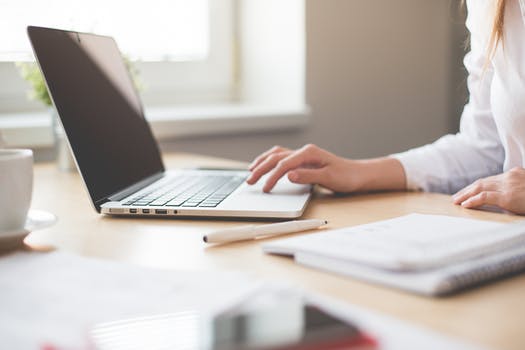
String interpolation. This is another kind of format syntax. When we use the "#" and curly-brackets in a string literal, we can capture variables and format them as strings.
Tip: For this syntax form, we must use the "#{identifier}" pattern. Ruby then inserts a value.
Ruby program that uses string interpolation
name = "Plato"
id = 65
# Use string interpolation to format variables.
# ... The names specified in brackets must match exactly.
result = "Name is #{name}, ID is #{id}"
p result
Output
"Name is Plato, ID is 65"
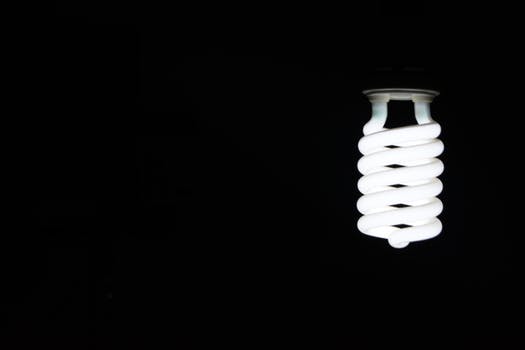
Format syntax, names. We can specify a mapping from string format identifiers to actual program variables. Here we specify "b" means the breed variable and "z" means size.
Tip: This syntax makes it possible to map variables to a string format pattern.
And: Suppose were name variables in a program. The string does not need changing, just the mapping.
Ruby program that uses format syntax with names
breed = "Spaniel"
size = 65
# Use names in format syntax.
# ... We use "b" as the name for breed and "z" for size.
result = "Breed %{b} size %{z}" % {b: breed, z: size}
p result
Output
"Breed Spaniel size 65"
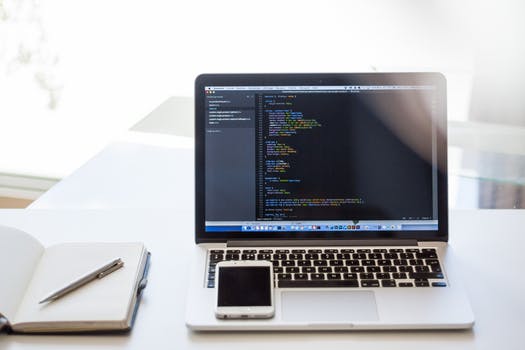
Kernel::sprintf. The string format syntax, which uses the "%" symbol, calls into the Kernel::sprintf method. We can use sprintf or format() explicitly, but there is no benefit to this.
Ruby program that uses Kernel, sprintf, format
# String format syntax.
result = "There are %d units." % 10
puts result
# Call sprintf for equivalent functionality.
result = Kernel::sprintf("There are %d units.", 10)
puts result
# Call format.
result = Kernel::format("There are %d units.", 10)
puts result
Output
There are 10 units.
There are 10 units.
There are 10 units.
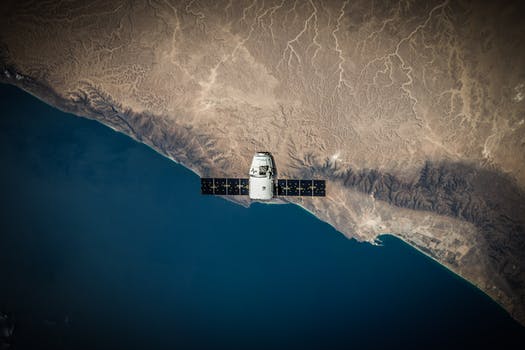
Interpolation benchmark. Is string interpolation fast? I compared this syntax to a concatenation of 4 values. I found interpolation was faster.
Version 1: This version of the code uses string interpolation syntax to combine 4 values together.
Version 2: This code uses string concatenation with the plus operator to merge 4 values into 1 string. This version was slower.
Result: String interpolation is a clear performance winner in these simple tests. I recommend it for most Ruby programs.
Further: I tested string format syntax, with "%s" and "%d" and found it to be even slower than concat.
Ruby program that times string interpolation, concat
n1 = Time.now.usec
# Version 1: use string interpolation syntax.
90000.times do
cat = "Felix"
size = 100
result = "Name: #{cat}, size: #{size}"
end
n2 = Time.now.usec
# Version 2: use string concatenation.
90000.times do
cat = "Felix"
size = 100
result = "Name: " + cat + ", size: " + String(size)
end
n3 = Time.now.usec
# Total milliseconds.
puts ((n2 - n1) / 1000)
puts ((n3 - n2) / 1000)
Output
44 ms: String interpolation syntax
71 ms: String concat (plus)
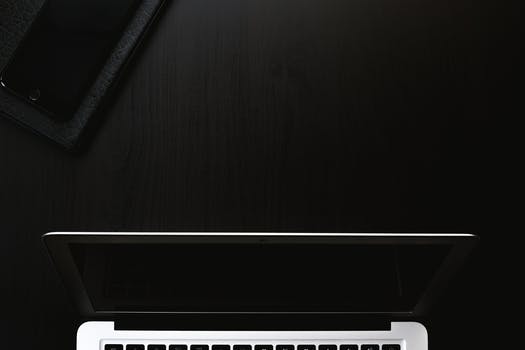
A perspective. Most requirements in programming are not glamorous. They involve no inventive algorithms. Instead, we often format strings.
We process text. In Ruby the string format syntax helps make this mundane stuff easier. A method (sprintf) can be used instead of the "%" symbol.
Related Links:
- Ruby Convert Types: Arrays and Strings
- Ruby File Handling: File and IO Classes
- Ruby Regexp Match Method
- Ruby String Array Examples
- Ruby include: Array Contains Method
- Ruby Class Examples: Self, Super and Module
- Ruby DateTime Examples: require date
- Ruby 2D Array Examples
- Ruby Number Examples: Integer, Float, zero and eql
- Ruby Exception Examples: Begin and Rescue
- Top 65 Ruby Interview Questions (2021)
- Ruby on Rails Interview Questions (2021)
- Ruby String Examples (each char, each line)
- Ruby if Examples: elsif, else and unless
- Ruby Math Examples: floor, ceil, round and truncate
- Ruby Sub, gsub: Replace String
- Ruby Substring Examples
- Ruby Console: Puts, Print and stdin
- Ruby Remove Duplicates From Array
- Ruby Random Number Generator: rand, srand
- Ruby Recursion Example
- Ruby ROT13 Method
- Ruby Iterator: times, step Loops
- Ruby String Length, For Loop Over Chars
- Ruby Join Example (Convert Array to String)
- Ruby Format String Examples
- Ruby Copy Array Example
- Ruby Keywords
- Ruby Nil Value Examples: NoMethodError
- Learn Ruby Tutorial
- Ruby Method: Def, Arguments and Return Values
- Ruby Fibonacci Sequence Example
- Ruby Hash Examples
- Ruby While, Until and For Loop Examples
- Learn Ruby on Rails Tutorial
- Ruby Word Count: Split Method
- Ruby Sort Arrays (Use Block Syntax)
- Ruby Case Examples: Ranges, Strings and Regexp
- Ruby Array Examples
- Ruby Split String Examples
Related Links
Adjectives
Ado
Ai
Android
Angular
Antonyms
Apache
Articles
Asp
Autocad
Automata
Aws
Azure
Basic
Binary
Bitcoin
Blockchain
C
Cassandra
Change
Coa
Computer
Control
Cpp
Create
Creating
C-Sharp
Cyber
Daa
Data
Dbms
Deletion
Devops
Difference
Discrete
Es6
Ethical
Examples
Features
Firebase
Flutter
Fs
Git
Go
Hbase
History
Hive
Hiveql
How
Html
Idioms
Insertion
Installing
Ios
Java
Joomla
Js
Kafka
Kali
Laravel
Logical
Machine
Matlab
Matrix
Mongodb
Mysql
One
Opencv
Oracle
Ordering
Os
Pandas
Php
Pig
Pl
Postgresql
Powershell
Prepositions
Program
Python
React
Ruby
Scala
Selecting
Selenium
Sentence
Seo
Sharepoint
Software
Spellings
Spotting
Spring
Sql
Sqlite
Sqoop
Svn
Swift
Synonyms
Talend
Testng
Types
Uml
Unity
Vbnet
Verbal
Webdriver
What
Wpf