<< Back to RUBY
Ruby Split String Examples
Call the split method to separate strings. Use string and regular expression delimiters.Split. Strings often contain blocks of data. With split, we separate these blocks based on a delimiter. In Ruby, a string, or a regular expression, is used as the separator.
Split is powerful. This method is widely used. When we omit an argument, it separates a string on spaces. This is the default behavior.
First example. Please consider the input string here: it contains three words. Each one is separated with a single space character.
Then: We call split(), specifying a space as the delimiter character. This separates those three words.
Array: The resulting array has three string elements. We loop over these with the each iterator.
Ruby program that uses split
# Split this string on a space.
input = "one two three"
values = input.split(" ")
# Display each value to the console.
values.each do |value|
puts value
end
Output
one
two
three
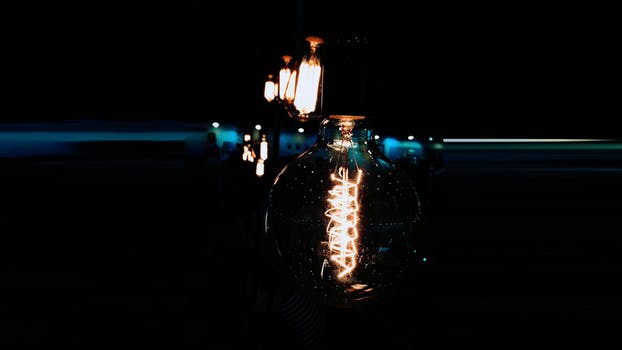
No arguments. No arguments are required to split on a space character. The space delimiter is implicit: you do not need to specify it. This can make some programs easier to read.
But: Perhaps a comment would help in this case. Usually split() is called with a delimiter, so this may not be as expected.
Ruby program that uses split, no arguments
input = "a b c"
# We do not specify an argument: space is implicit.
values = input.split()
puts values
Output
a
b
c
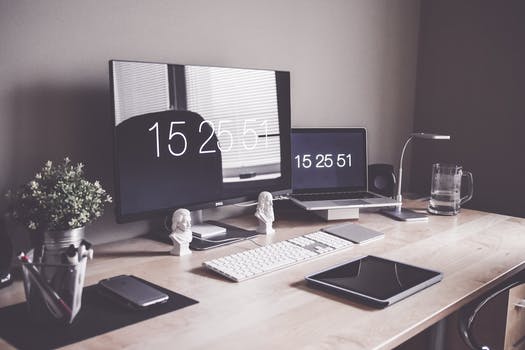
Regexp. Split does not require a simple string argument. It can act also upon a regular expression (regexp). In Ruby we specify these with forward slashes.
Here: We specify a delimiter of one or more non-word characters. The Kleene closure + indicates "one or more."
Tip: The delimiter here matches one or two characters. It includes both the comma and the following space.
So: The resulting string array has no empty values. It contains just the four words stored within the text.
Ruby program that uses Regexp to split
value = "one, two three: four"
# Split on one or more non-word characters.
a = value.split(/\W+/)
# Display result.
puts a
Output
one
two
three
four
Regexp pattern
\W+ One or more non-word characters.
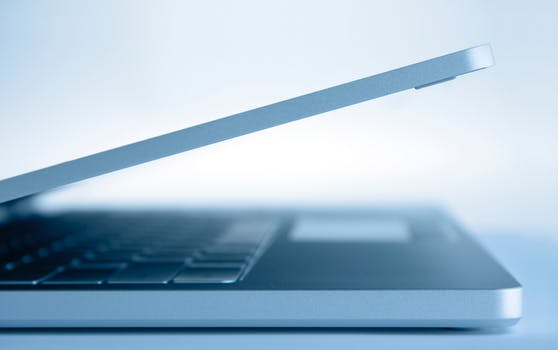
Limit. This is the maximum number of array elements that are returned. If more elements are found than are allowed by the limit, the excess ones are grouped in the final array element.
Tip: Limiting the number of array elements can be useful if you only need the first several parts from a string.
Ruby program that splits with limit
# Contains five vegetable names.
value = "carrot,squash,corn,broccoli,spinach"
# Split with limit of 3.
vegetables = value.split(",", 3)
puts vegetables
Output
carrot
squash
corn,broccoli,spinach
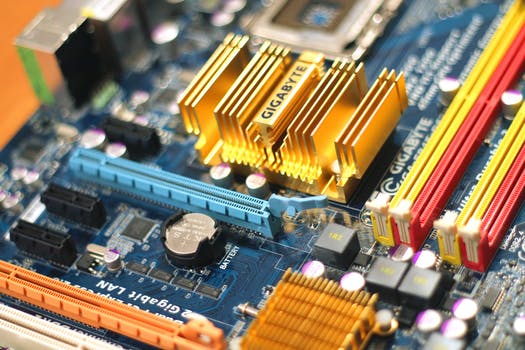
Empty. Often the split method will return empty entries. These are caused by having two delimiters with no interior content. We can invoke delete_if to remove these empty elements.
So: We use an iterator block. We delete all elements that are zero chars. The empty entry, between "cat" and "dog," is removed.
Ruby program that removes empty entries
# Split on a comma.
value = "cat,,dog,bird"
elements = value.split(",")
print elements, "\n"
# Remove empty elements from the array.
elements.delete_if{|e| e.length == 0}
print elements
Output
["cat", "", "dog", "bird"]
["cat", "dog", "bird"]
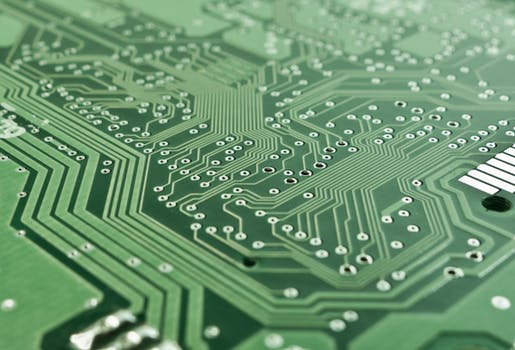
Characters. With split we can get the characters from a string. Pass an empty string literal ("") to the split method. The length of the array equals the length of the string.
Ruby program that splits characters
value = "xyz 1"
# Separate chars.
array = value.split ""
# Write length.
puts array.length
# Write elements.
print array
Output
5
["x", "y", "z", " ", "1"]
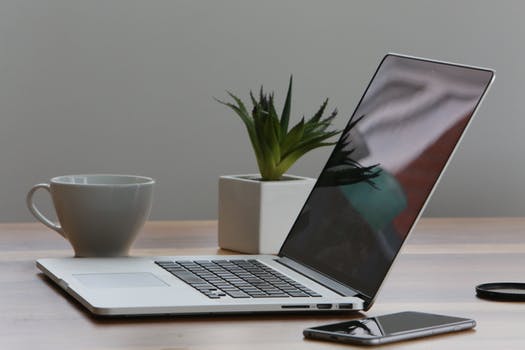
File. Often we need to handle CSV files. We first use the IO.foreach iterator to easily loop over the lines in a text file. Each line must be chomped to remove the trailing newline.
Then: We use split() on the commas. The parts between the comma chars are returned in an array.
Output: The program writes the contents of the Array returned by split. It also prints the length of that Array.
Example file: csv.txt
cat,tiger,meow,100
airplane,bird,200
tree,grove,400
sand,beach,fish,50
Ruby program that splits lines in file
# Open this file (change file name for your program).
IO.foreach("/files/csv.txt") do |line|
# Remove trailing whitespace.
line.chomp!
# Split on comma.
values = line.split(",")
# Write results.
print values.join("+") << "... " << String(values.length) << "\n"
end
Output
cat+tiger+meow+100... 4
airplane+bird+200... 3
tree+grove+400... 3
sand+beach+fish+50... 4
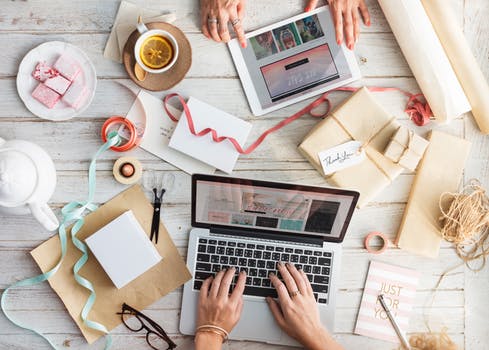
Parse integers. Often we need to parse integer values that are in a CSV format. We first split the line, and then use Integer to convert each string.
Here: We display each number in the string that is equal to or greater than 200. The value 100 is not displayed.
Ruby program that uses split, parses Integers
line = "100,200,300"
# Split on the comma char.
values = line.split(",")
# Parse each number in the result array.
values.each do |v|
number = Integer(v)
# Display number if it is greater than or equal to 200.
if number >= 200
puts number
end
end
Output
200
300
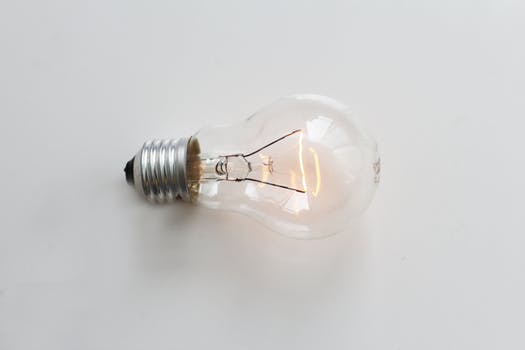
Join. This is the opposite of split. It merges together values in an array. With join and split we can parse a string, modify the values, and return the string to its original state.
Join
In CSV files, input lines contain separating characters. We do not need a special parsing method to extract the inner strings. Split(), with a special delimiter, works well.
Delimiters. We learned how to split based on a string delimiter. A regular expression offers more power. And finally we used join to combine strings in an Array.
Related Links:
- Ruby Convert Types: Arrays and Strings
- Ruby File Handling: File and IO Classes
- Ruby Regexp Match Method
- Ruby String Array Examples
- Ruby include: Array Contains Method
- Ruby Class Examples: Self, Super and Module
- Ruby DateTime Examples: require date
- Ruby 2D Array Examples
- Ruby Number Examples: Integer, Float, zero and eql
- Ruby Exception Examples: Begin and Rescue
- Top 65 Ruby Interview Questions (2021)
- Ruby on Rails Interview Questions (2021)
- Ruby String Examples (each char, each line)
- Ruby if Examples: elsif, else and unless
- Ruby Math Examples: floor, ceil, round and truncate
- Ruby Sub, gsub: Replace String
- Ruby Substring Examples
- Ruby Console: Puts, Print and stdin
- Ruby Remove Duplicates From Array
- Ruby Random Number Generator: rand, srand
- Ruby Recursion Example
- Ruby ROT13 Method
- Ruby Iterator: times, step Loops
- Ruby String Length, For Loop Over Chars
- Ruby Join Example (Convert Array to String)
- Ruby Format String Examples
- Ruby Copy Array Example
- Ruby Keywords
- Ruby Nil Value Examples: NoMethodError
- Learn Ruby Tutorial
- Ruby Method: Def, Arguments and Return Values
- Ruby Fibonacci Sequence Example
- Ruby Hash Examples
- Ruby While, Until and For Loop Examples
- Learn Ruby on Rails Tutorial
- Ruby Word Count: Split Method
- Ruby Sort Arrays (Use Block Syntax)
- Ruby Case Examples: Ranges, Strings and Regexp
- Ruby Array Examples
- Ruby Split String Examples
Related Links
Adjectives
Ado
Ai
Android
Angular
Antonyms
Apache
Articles
Asp
Autocad
Automata
Aws
Azure
Basic
Binary
Bitcoin
Blockchain
C
Cassandra
Change
Coa
Computer
Control
Cpp
Create
Creating
C-Sharp
Cyber
Daa
Data
Dbms
Deletion
Devops
Difference
Discrete
Es6
Ethical
Examples
Features
Firebase
Flutter
Fs
Git
Go
Hbase
History
Hive
Hiveql
How
Html
Idioms
Insertion
Installing
Ios
Java
Joomla
Js
Kafka
Kali
Laravel
Logical
Machine
Matlab
Matrix
Mongodb
Mysql
One
Opencv
Oracle
Ordering
Os
Pandas
Php
Pig
Pl
Postgresql
Powershell
Prepositions
Program
Python
React
Ruby
Scala
Selecting
Selenium
Sentence
Seo
Sharepoint
Software
Spellings
Spotting
Spring
Sql
Sqlite
Sqoop
Svn
Swift
Synonyms
Talend
Testng
Types
Uml
Unity
Vbnet
Verbal
Webdriver
What
Wpf