<< Back to GO
Golang Const, Var Examples: Iota
Use the const and var keywords. Generate constants with the iota enumerator.Consts. Some things do not change. Over the years, a mountain remains mostly the same. Consider a value like 155. This is a constant value: we can use a const block for it.
Constant creation. With iota we gain a way to generate groups of constants based on expressions. With it, we can create an entire group of constants. This reduces bugs.
An example. Here we introduce the const keyword in a block of enumerated constants. We do not use all the features of const here. We declare three names and give them values.
Tip: The equals signs should line up vertically in consts. This is achieved with the Gofmt program.
Tip 2: We can use these constants as values in a Go program by directly inserting the names.
Golang program that uses const
package main
import "fmt"
const (
Cat = 10
Dog = 20
Bird = 30
)
func main() {
// Use our constants.
fmt.Println(Cat)
fmt.Println(Dog)
fmt.Println(Bird)
}
Output
10
20
30
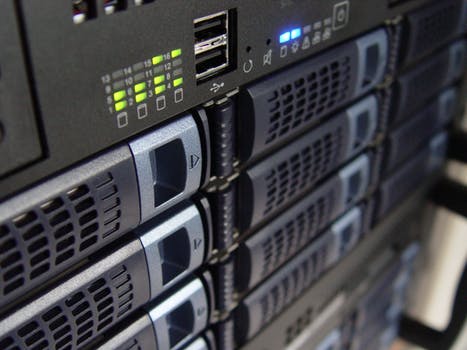
Iota. This is an enumerator for const creation. The Go compiler starts iota at 0 and increments it by one for each following constant. We can use it in expressions.
Caution: We cannot use iota in an expression that must be evaluated at runtime—a const is determined at compile-time.
Tip: The term iota is like beta: it stands for the letter "I" in Greek, just as beta stands for B.
Quote: In some programming languages iota is used to represent an array of consecutive integers.
Iota: WikipediaGolang program that uses iota in const
package main
import "fmt"
const (
Low = 5 * iota
Medium
High
)
func main() {
// Use our iota constants.
fmt.Println(Low)
fmt.Println(Medium)
fmt.Println(High)
}
Output
0
5
10
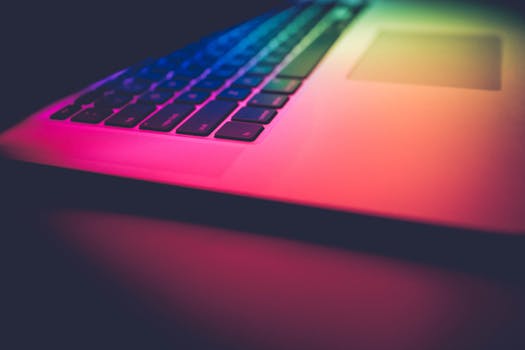
Cannot assign const. A constant name cannot be assigned to another value. It is not a variable. The Go compiler will report an error here. With var, the program will compile.
Golang program that causes assignment error
package main
const (
Normal = 1
Medium = 2
)
func main() {
Medium = 3
}
Output
C:\programs\file.go:13: cannot assign to Medium
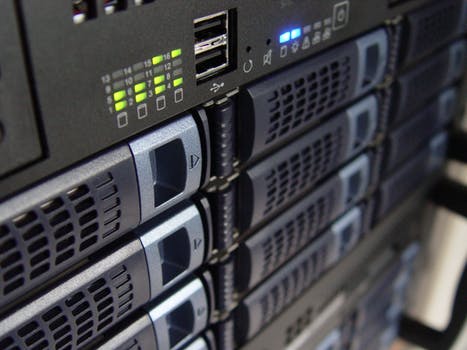
One const syntax. A constant can be declared in a single-line statement. We can also specify constants within func bodies. Here we create a constant string of value "Blue."
Golang program that uses constant in func
package main
import "fmt"
func main() {
// Use a constant string in a func.
const color = "Blue"
// Test the constant.
if color == "Blue" {
fmt.Println(color)
}
}
Output
Blue
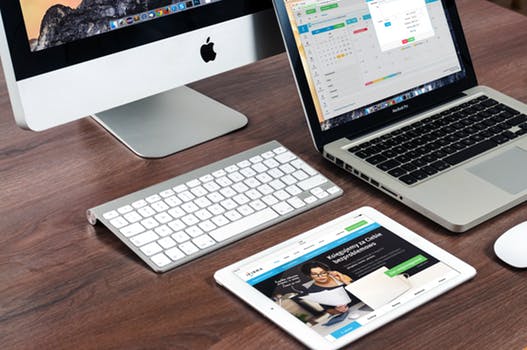
Multiple consts syntax. We can declare multiple consts on one line. No parentheses are required. Here we have a const string and a const untyped integer.
Note: By default, a const integer value is untyped. A type like int64 can be specified.
Golang program that uses multiple consts on one line
package main
import "fmt"
func main() {
// Declare multiple consts on one line.
const name, size = "carrot", 100
// Display values.
fmt.Println(name)
fmt.Println(size)
}
Output
carrot
100
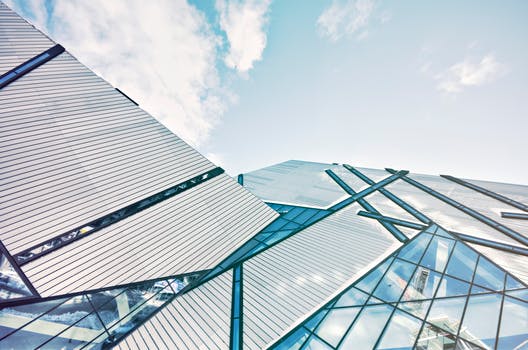
Type int16. A const can be typed. Here we specify the type int16 for the const "v." We add the int16 to a slice of int16 elements.
Tip: By default, integer consts are untyped integers. These can be used as int16 or other integral values.
Golang program that uses const with int16 type
package main
import "fmt"
func main() {
// A const can have an explicit type.
const v int16 = 10
// Create a slice with the constant int16.
slice := []int16{v, 10}
fmt.Println(slice)
}
Output
[10 10]
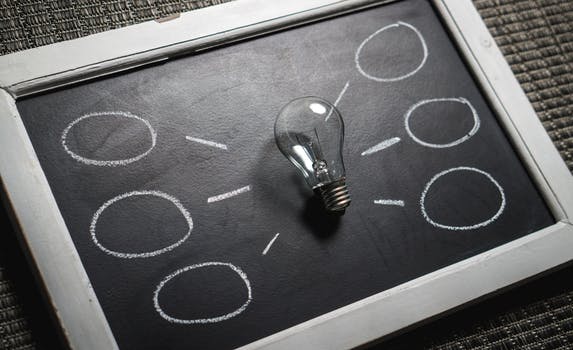
Type error. Sometimes using a type on an integer constant will cause an error. The type must be correct for the rest of the program. Otherwise the program will not compile.
Golang program that causes error with typed const
package main
import "fmt"
func main() {
const v int32 = 1000
// The const int32 cannot be used in an int16 slice.
slice := []int16{v, 10}
fmt.Println(slice)
}
Output
cannot use v (type int32) as type int16 in array element
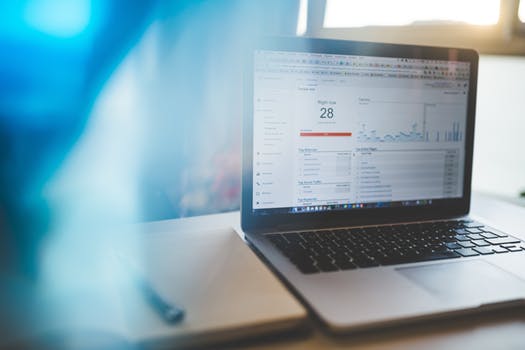
Overflow. With const typed integers, overflow is detected at compile-time. Here we try to use an invalid number in an int16. The program never reaches execution.
Golang program that uses invalid const int16
package main
import "fmt"
func main() {
// We cannot have an int16 with this value.
const v int16 = 1000000
// Not reached.
fmt.Println(v)
}
Output
constant 1000000 overflows int16
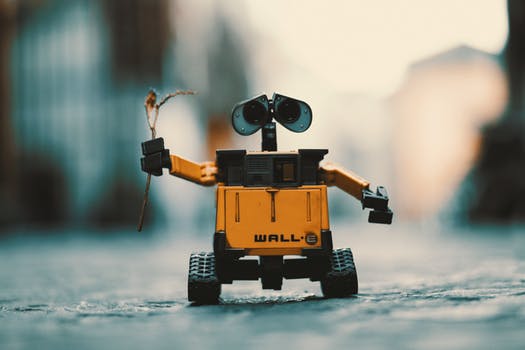
Var, variables. We can declare variables in a var block. Here variables are initialized at runtime, and they can be reassigned in methods. They can be used throughout the program.
Golang program that uses var
package main
import (
"fmt"
"time"
)
var (
Month = time.Now().Month()
Year = time.Now().Year()
)
func main() {
// Use variables declared in var.
fmt.Println(Month)
fmt.Println(Year)
}
Output
January
2015
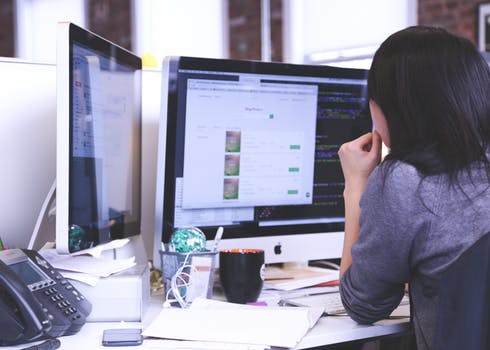
Var, short syntax. In Go we can use the var keyword with a type or without a type. We can declare variables with var. But we can also use a short declaration syntax form.
Version 1: This code uses a var declaration with no type specified. The string "animal" is assigned to different values and printed.
Version 2: This version uses a short variable declaration with the ":=" characters. It does the same thing as program version 1.
Golang program that uses variable declaration, var keyword
package main
import "fmt"
func Test1() {
// Version 1: use a local variable string.
var animal = "Cat"
fmt.Println("TEST1: " + animal)
// Reassign the local variable.
animal = "Dog"
fmt.Println("TEST1: " + animal)
}
func Test2() {
// Version 2: use short variable declaration.
animal := "Cat"
fmt.Println("TEST2: " + animal)
// Reassign it.
animal = "Dog"
fmt.Println("TEST2: " + animal)
}
func main() {
Test1()
Test2()
}
Output
TEST1: Cat
TEST1: Dog
TEST2: Cat
TEST2: Dog
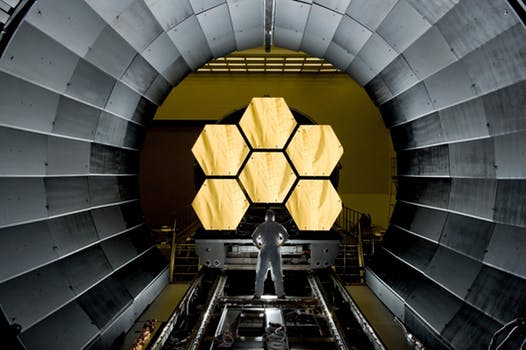
Typed vars. A var declaration can have more than one variable in it, and each can have a separate value. But only one type can be used. All variables use the specified type.
Quote: If a type is present, each variable is given that type (Go Specification).
Golang program that uses two typed vars, one line
package main
import "fmt"
func main() {
// Create two vars of type int32 on one line.
var n, y int32 = 100, 200
// Display variables.
fmt.Println(n)
fmt.Println(y)
}
Output
100
200
With const and iota, Go provides compile-time code generation features. We can specify many constants with just an expression. With var we separate variables into a block.
Built-Ins
Related Links:
- Golang strconv, Convert Int to String
- Golang Odd and Even Numbers
- Golang Recover Built In: Handle Errors, Panics
- Learn Go Language Tutorial
- Golang html template Example
- Golang http.Get Examples: Download Web Pages
- Golang container list Example (Linked List)
- Golang base64 Encoding Example: EncodeToString
- Golang os exec Examples: Command Start and Run
- Golang String Between, Before and After
- Golang os.Remove: Delete All Files in Directory
- Golang First Words in String
- Golang flag Examples
- Golang Regexp Find Examples: FindAllString
- Golang Regexp Examples: MatchString, MustCompile
- Golang Index, LastIndex: strings Funcs
- Golang Compress GZIP Examples
- Golang Interface Example
- Golang 2D Slices and Arrays
- Golang Sscan, Sscanf Examples (fmt)
- Top 41 Go Programming (Golang) Interview Questions (2021)
- Golang Padding String Example (Right or Left Align)
- Golang Equal String, EqualFold (If Strings Are the Same)
- Golang map Examples
- Golang Map With String Slice Values
- Golang Array Examples
- Golang Remove Duplicates From Slice
- Golang If, Else Statements
- Golang ParseInt Examples: Convert String to Int
- Golang Strings
- Golang strings.Map func
- Golang bufio.ScanBytes, NewScanner (Read Bytes in File)
- Golang Built In Functions
- Golang bytes.Buffer Examples (WriteString, Fprintf)
- Golang Bytes: Slices and Methods
- Golang Caesar Cipher Method
- Golang Chan: Channels, Make Examples
- Golang Math Module: math.Abs, Pow
- Golang Reverse String
- Golang Struct Examples: Types and Pointers
- Golang path and filepath Examples (Base, Dir)
- Golang Substring Examples (Rune Slices)
- Golang Suffixarray Examples: New, Lookup Benchmark
- Golang switch Examples
- Golang Convert Map to Slice
- Golang Convert Slice to String: int, string Slices
- Golang Const, Var Examples: Iota
- Golang ROT13 Method
- Golang strings.Contains and ContainsAny
- Golang rand, crypto: Random Number Generators
- Golang String Literal Examples (Repeat Method)
- Golang ToLower, ToUpper String Examples
- Golang Trim, TrimSpace and TrimFunc Examples
- Golang Join Examples (strings.Join)
- Golang Len (String Length)
- Golang Convert String to Rune Slice (append)
- Golang JSON Example: Marshal, Unmarshal
- Golang Replace String Examples: Replacer, NewReplacer
- Golang nil (Cannot Use nil as Type)
- Golang Slice Examples
- Golang ListenAndServe Examples (HandleFunc)
- Golang Fibonacci Sequence Example
- Golang Time: Now, Parse and Duration
- Golang bits, OnesCount (Get Bitcount From Int)
- Golang Fprint, Fprintf and Fprintln Examples (fmt)
- Golang Func Examples
- Golang csv Examples
- Golang Fields and FieldsFunc
- Golang unicode.IsSpace (If Char Is Whitespace)
- Golang fmt.Println Examples
- Golang for Loop Examples: Foreach and While
- Golang ioutil.WriteFile, os.Create (Write File to Disk)
- Golang File Handling
- Golang range: Slice, String and Map
- Golang Readdir Example (Get All Files in Directory)
- Golang Sort Slice: Len, Less, Swap in Interface
- Golang Get Lines in File (String Slice)
- Golang Split Examples (SplitAfter, SplitN)
Related Links
Adjectives
Ado
Ai
Android
Angular
Antonyms
Apache
Articles
Asp
Autocad
Automata
Aws
Azure
Basic
Binary
Bitcoin
Blockchain
C
Cassandra
Change
Coa
Computer
Control
Cpp
Create
Creating
C-Sharp
Cyber
Daa
Data
Dbms
Deletion
Devops
Difference
Discrete
Es6
Ethical
Examples
Features
Firebase
Flutter
Fs
Git
Go
Hbase
History
Hive
Hiveql
How
Html
Idioms
Insertion
Installing
Ios
Java
Joomla
Js
Kafka
Kali
Laravel
Logical
Machine
Matlab
Matrix
Mongodb
Mysql
One
Opencv
Oracle
Ordering
Os
Pandas
Php
Pig
Pl
Postgresql
Powershell
Prepositions
Program
Python
React
Ruby
Scala
Selecting
Selenium
Sentence
Seo
Sharepoint
Software
Spellings
Spotting
Spring
Sql
Sqlite
Sqoop
Svn
Swift
Synonyms
Talend
Testng
Types
Uml
Unity
Vbnet
Verbal
Webdriver
What
Wpf