<< Back to GO
Golang fmt.Println Examples
Use the fmt package to write values to the console. Call Println and Sprintf.Fmt, printing. A program keeps some of its memory private. It does not need to tell us everything it does. But sometimes output needs to be printed and reported.
With fmt, a package included with Go, we display data to the console or another location. With classic methods like Printf and Println, fmt is easy to use.
Println. Let us begin with the Println method. This method is one of the easiest and simplest ones in fmt. We first must use an import statement with the argument "fmt."
Then: We invoke methods, like Println, on the fmt package. Println is versatile and can accept many arguments.
Tip: We can print values like strings or ints, or more complex things like slices. No loop is needed to print elements of a slice or array.
Golang program that uses fmt, Println
package main
import "fmt"
func main() {
// The Println method can handle one or more arguments.
fmt.Println("cat")
fmt.Println("cat", 900)
// Use Println on a slice.
items := []int{10, 20, 30}
fmt.Println(items)
}
Output
cat
cat 900
[10 20 30]
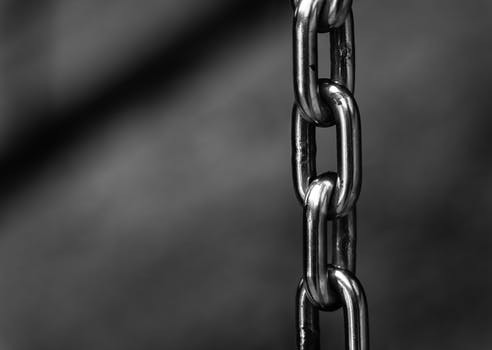
Print, for-loop. The Println always inserts a newline after we use it. But Print does not: it just writes the data to the console with no trailing newline.
Tip: For loops where we want to display many things on a single line, Print is ideal.
Golang program that uses Print on slice
package main
import "fmt"
func main() {
elements := []int{999, 99, 9}
// Loop over the int slice and Print its elements.
// ... No newline is inserted after Print.
for i := 0; i < len(elements); i++ {
fmt.Print(elements[i] + 1)
fmt.Print(" ")
}
fmt.Println("... DONE!")
}
Output
1000 100 10 ... DONE!
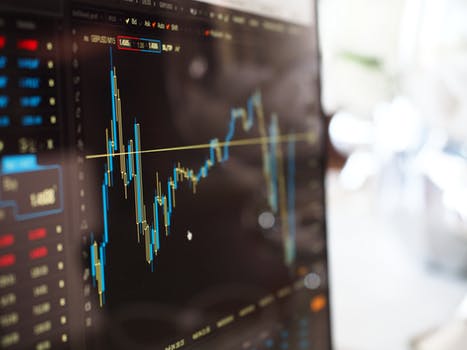
Printf. This method accepts a format string. We use codes like "%s" and "%d" in this string to indicate insertion points for values. Those values are also passed as arguments.
Golang program that uses Printf
package main
import "fmt"
func main() {
name := "Mittens"
weight := 12
// Use %s to mean string.
// ... Use an explicit newline.
fmt.Printf("The cat is named %s.\n", name)
// Use %d to mean integer.
fmt.Printf("Its weight is %d.\n", weight)
}
Output
The cat is named Mittens.
Its weight is 12.
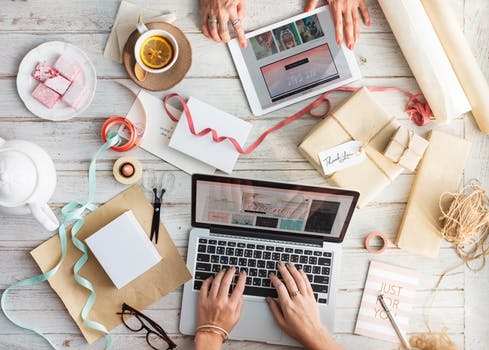
Printf, V format. With Printf many format codes are available. But to make programs simpler, we can just use the "%v" format code to insert values.
And: The v code handles ints, bools, strings and other values. It makes Printf calls easier to write and read.
Golang program that uses Printf, V code
package main
import "fmt"
func main() {
result := true
name := "Spark"
size := 2000
// Print line with v format codes.
fmt.Printf("Result = %v, Name = %v, Size = %v",
result, name, size)
}
Output
Result = true, Name = Spark, Size = 2000
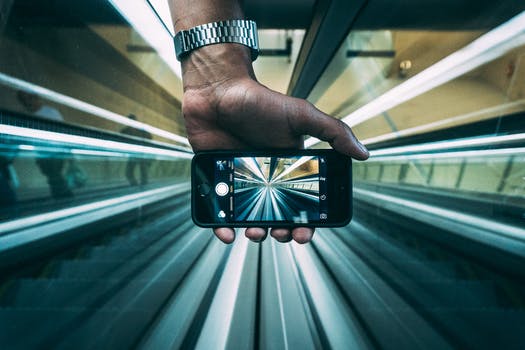
Print, println. Print and println are universal functions. We can call these without referencing the "fmt" package. But their functionality is not identical to the fmt methods.
Built-InsWarning: Idiomatic Go tends to use fmt.Println not just println. The fmt package is thus preferred.
Golang program that uses print, println
package main
func main() {
value := 10
// Use println.
println(value)
// Use print.
// ... Use println with no arguments to write a newline.
print(value)
println()
// Done.
println("DONE")
}
Output
10
10
DONE
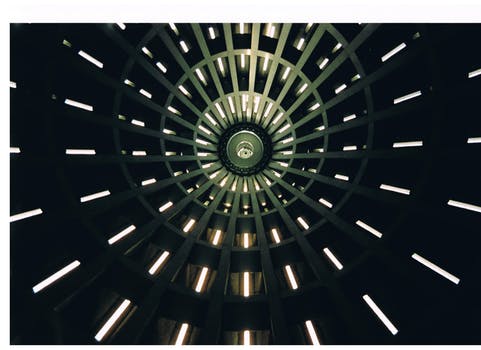
Println differences. The println universal function has different output than the fmt.Println method. They are not the interchangeable.
Here: Consider a slice of two ints. Fmt.Println displays the elements, but "println" displays a reference value.
Golang program that shows println differences
package main
import "fmt"
func main() {
items := []int{10, 20}
// These two println methods have different output.
fmt.Println(items)
println(items)
}
Output
[10 20]
[2/2]0xc08200a250
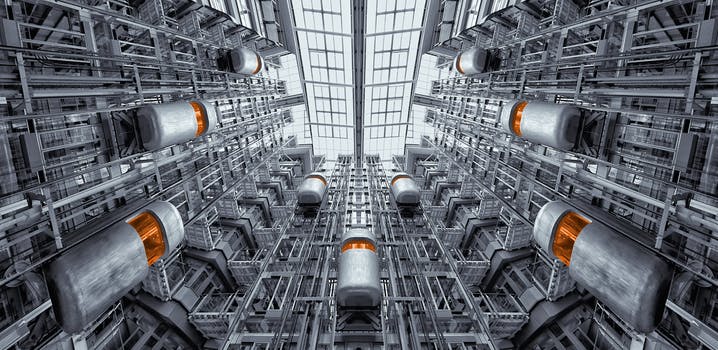
Sprintf. This method is a string-based form of Printf. The "S" stands for "string." So we use Printf and it returns a string—the value is not written to the console.
Here: We use 2 formats (the "%v" handles any value) to compose 1 string. We then print its length, and its contents with fmt.Prinln.
Tip: If we want to store the results of Printf in a string (for later use or processing) then Sprintf is ideal.
Golang program that uses fmt.Sprintf
import "fmt"
func main() {
value1 := "a tree";
value2 := "the park";
// Use format string to generate string.
result := fmt.Sprintf("I saw %v at %v.\n", value1, value2)
// Write length of string, and string itself.
fmt.Println("Length:", len(result))
fmt.Println(result)
}
Output
Length: 26
I saw a tree at the park.
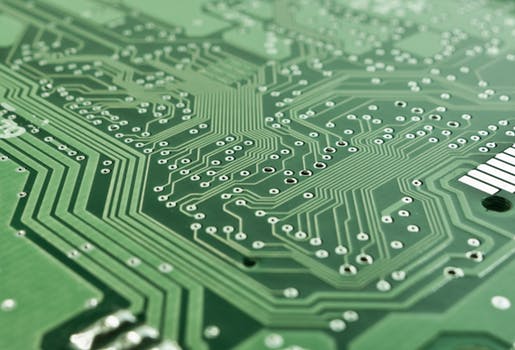
Sprintln. This func takes any number or arguments—it works the same way as fmt.Println, but returns a string. It does not support format codes.
Golang program that uses Sprintln
package main
import "fmt"
func main() {
// Use Sprintln, no format strings are supported.
// ... A newline is added.
// A string is returned.
result := fmt.Sprintln("Hey friend", 100)
fmt.Print("[" + result + "]")
}
Output
[Hey friend 100
]
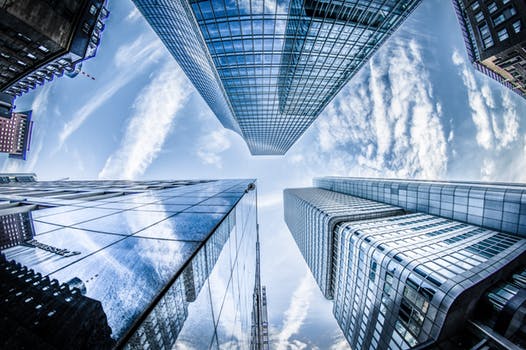
Fprint, Fprintf. These methods are just like fmt.Print but target a file in the first argument. They write to files—nothing is written to the console.
Fprint
Sscan, Sscanf. We can parse in space-separated values with fmt.Sscan. And with Sscanf we use a format string to parse in values. Sometimes this is easier than using split() or fields().
Sscan, Sscanf
Padding. We can apply padding with fmt.Printf to ensure strings are a certain number of characters long. Spaces are added before or after the value.
PaddingNotes on fmt. Most programs in this Go tutorial use the import "fmt" statement. They are console programs, designed to be compiled and run directly.
Quote: Package fmt implements formatted IO with functions analogous to C's printf and scanf. The format 'verbs' are derived from C's but are simpler.
Fmt: golang.orgWith methods prefixed by s, we can output the results to a string. We can write those strings to a file, or use them in any way we can use a string.
Related Links:
- Golang strconv, Convert Int to String
- Golang Odd and Even Numbers
- Golang Recover Built In: Handle Errors, Panics
- Learn Go Language Tutorial
- Golang html template Example
- Golang http.Get Examples: Download Web Pages
- Golang container list Example (Linked List)
- Golang base64 Encoding Example: EncodeToString
- Golang os exec Examples: Command Start and Run
- Golang String Between, Before and After
- Golang os.Remove: Delete All Files in Directory
- Golang First Words in String
- Golang flag Examples
- Golang Regexp Find Examples: FindAllString
- Golang Regexp Examples: MatchString, MustCompile
- Golang Index, LastIndex: strings Funcs
- Golang Compress GZIP Examples
- Golang Interface Example
- Golang 2D Slices and Arrays
- Golang Sscan, Sscanf Examples (fmt)
- Top 41 Go Programming (Golang) Interview Questions (2021)
- Golang Padding String Example (Right or Left Align)
- Golang Equal String, EqualFold (If Strings Are the Same)
- Golang map Examples
- Golang Map With String Slice Values
- Golang Array Examples
- Golang Remove Duplicates From Slice
- Golang If, Else Statements
- Golang ParseInt Examples: Convert String to Int
- Golang Strings
- Golang strings.Map func
- Golang bufio.ScanBytes, NewScanner (Read Bytes in File)
- Golang Built In Functions
- Golang bytes.Buffer Examples (WriteString, Fprintf)
- Golang Bytes: Slices and Methods
- Golang Caesar Cipher Method
- Golang Chan: Channels, Make Examples
- Golang Math Module: math.Abs, Pow
- Golang Reverse String
- Golang Struct Examples: Types and Pointers
- Golang path and filepath Examples (Base, Dir)
- Golang Substring Examples (Rune Slices)
- Golang Suffixarray Examples: New, Lookup Benchmark
- Golang switch Examples
- Golang Convert Map to Slice
- Golang Convert Slice to String: int, string Slices
- Golang Const, Var Examples: Iota
- Golang ROT13 Method
- Golang strings.Contains and ContainsAny
- Golang rand, crypto: Random Number Generators
- Golang String Literal Examples (Repeat Method)
- Golang ToLower, ToUpper String Examples
- Golang Trim, TrimSpace and TrimFunc Examples
- Golang Join Examples (strings.Join)
- Golang Len (String Length)
- Golang Convert String to Rune Slice (append)
- Golang JSON Example: Marshal, Unmarshal
- Golang Replace String Examples: Replacer, NewReplacer
- Golang nil (Cannot Use nil as Type)
- Golang Slice Examples
- Golang ListenAndServe Examples (HandleFunc)
- Golang Fibonacci Sequence Example
- Golang Time: Now, Parse and Duration
- Golang bits, OnesCount (Get Bitcount From Int)
- Golang Fprint, Fprintf and Fprintln Examples (fmt)
- Golang Func Examples
- Golang csv Examples
- Golang Fields and FieldsFunc
- Golang unicode.IsSpace (If Char Is Whitespace)
- Golang fmt.Println Examples
- Golang for Loop Examples: Foreach and While
- Golang ioutil.WriteFile, os.Create (Write File to Disk)
- Golang File Handling
- Golang range: Slice, String and Map
- Golang Readdir Example (Get All Files in Directory)
- Golang Sort Slice: Len, Less, Swap in Interface
- Golang Get Lines in File (String Slice)
- Golang Split Examples (SplitAfter, SplitN)
Related Links
Adjectives
Ado
Ai
Android
Angular
Antonyms
Apache
Articles
Asp
Autocad
Automata
Aws
Azure
Basic
Binary
Bitcoin
Blockchain
C
Cassandra
Change
Coa
Computer
Control
Cpp
Create
Creating
C-Sharp
Cyber
Daa
Data
Dbms
Deletion
Devops
Difference
Discrete
Es6
Ethical
Examples
Features
Firebase
Flutter
Fs
Git
Go
Hbase
History
Hive
Hiveql
How
Html
Idioms
Insertion
Installing
Ios
Java
Joomla
Js
Kafka
Kali
Laravel
Logical
Machine
Matlab
Matrix
Mongodb
Mysql
One
Opencv
Oracle
Ordering
Os
Pandas
Php
Pig
Pl
Postgresql
Powershell
Prepositions
Program
Python
React
Ruby
Scala
Selecting
Selenium
Sentence
Seo
Sharepoint
Software
Spellings
Spotting
Spring
Sql
Sqlite
Sqoop
Svn
Swift
Synonyms
Talend
Testng
Types
Uml
Unity
Vbnet
Verbal
Webdriver
What
Wpf