<< Back to SWIFT
Swift Characters: String Examples
Use the characters property on strings to access individual chars. Loop over characters with a for-loop.Characters. Strings in Swift are complicated. We cannot access characters just by integers. So a for-loop over a range of Ints cannot access a string's characters.
Instead, we use the characters property on a String. This allows us to access each individual character. We can test or count or these characters.
Simple example. Let us begin with a simple for-loop. The "c" variable here is the current iteration's character. We call print() on the characters.
Count: To count the characters in a string, we can access the count property. To count specific chars, we use for-loop with if-statements.
Note: With this kind of for-in loop, we cannot access adjacent characters in a string. We must use an index loop for this.
Swift program that uses characters property
let value = "cat"
// Loop over characters in string.
for c in value.characters {
print(c)
}
Output
c
a
t
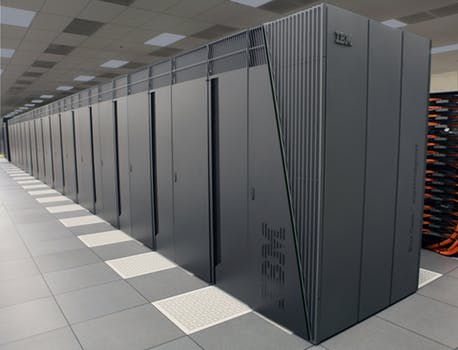
Count. This property returns the number of characters in the string. So with the string "carrot" it will return 6. The property cannot be assigned.
Swift program that uses count to get string length
var vegetable = "carrot"
// Get count of chars in string.
let c = vegetable.characters.count
print(c)
Output
6
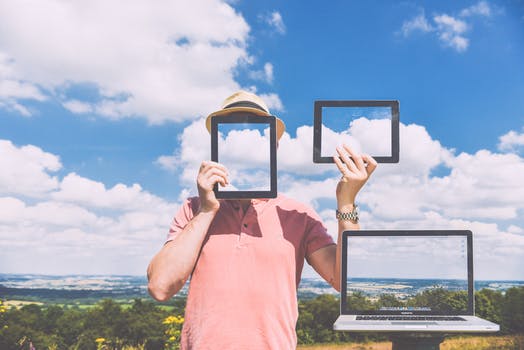
Contains. This method receives a Character, which can be specified as a one-char string. It returns true if the Character exists in the string, and false otherwise.
Swift program that uses contains to search for chars
var vegetable = "turnip"
// The string contains this letter.
let n = vegetable.characters.contains("n")
print(n)
// There is no "x" in the string.
let x = vegetable.characters.contains("x")
print(x)
Output
true
false
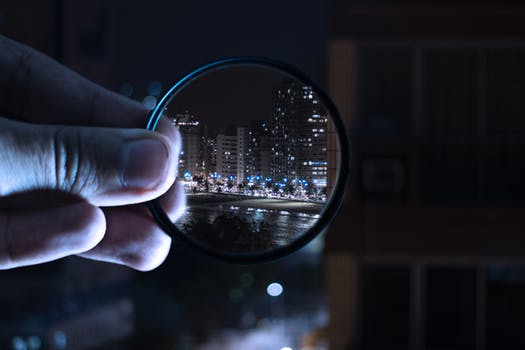
Count chars. To get the number of characters in a string, we can use count. All characters, including spaces and punctuation, are counted by this property.
Tip: Please see also the endIndex property. This is an index so it can be used to access and manipulate other parts of the string data.
endIndex: LengthSwift program that uses count, gets string length
// This string has four characters in it.
var value = "bird"
var length = value.characters.count
print(length)
// This string has six characters.
// ... Spaces and other chars are all counted.
value = "x y z "
length = value.characters.count
print(length)
Output
4
6
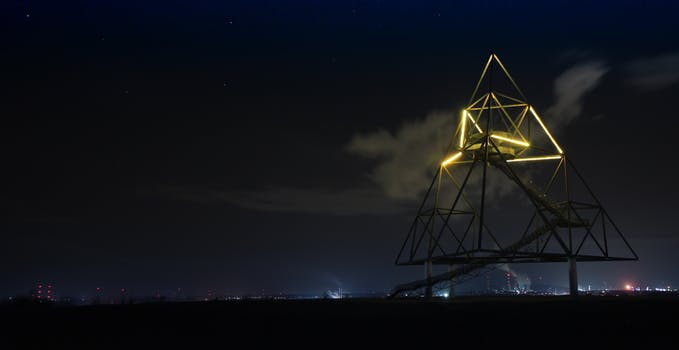
Convert to String. A string may be created from a Character array. Here we create a Character array of six characters. When we convert it to a string, it equals the name "Sancho."
Swift program that converts Character array to string
// Create a character array.
let chars: [Character] = ["S", "a", "n", "c", "h", "o"]
// Create a string from the character array.
let result = String(chars)
print(result)
// Test the string value.
if result == "Sancho" {
print(true)
}
Output
Sancho
true
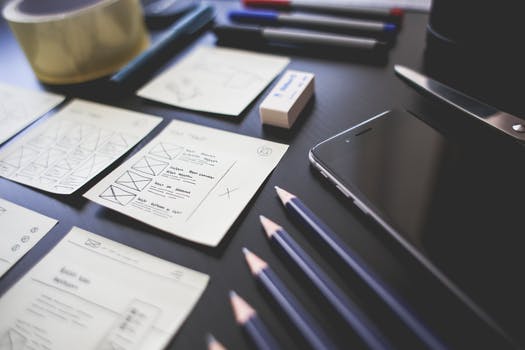
Convert characters. We can apply UnicodeScalar to convert Ints into Characters. And with utf8 and utf16 we can access integral values in a string's character data.
Convert Int to Character
With characters, we can combine multiple string operations. We can search for multiple things at once in a string. In one loop, we can determine if a string contains "X" or "Y."
A note. The characters property returns logical characters. In Swift, due to graphemes, some characters use more space than others. The characters property treats all characters the same.
A review. With characters, we access a property that returns all char values in a string. We can use this in a for-loop to test a string's text data.
Related Links:
- Swift String Append Example: reserveCapacity
- Swift File (Read Text File Into String)
- Swift Find Strings: range of Example
- Swift Subscript Example
- Swift hasPrefix and hasSuffix Examples
- Swift Array of Dictionary Elements
- Swift Tutorial
- Swift Odd and Even Numbers
- Swift Operator: Equality, Prefix and Postfix
- Swift Dictionary Examples
- Swift Class Example: init, self
- Swift Combine Arrays: append, contentsOf
- Swift Initialize Array
- Swift Int Examples: Int.max, Int.min
- Swift 2D Array Examples
- Swift Error Handling: try, catch
- Swift Repeat While Loop
- Swift Optional: Nil Examples
- Swift Replace String Example
- Swift Print: Lines, Separator and Terminator
- Swift inout Keyword: Func Example
- Swift Lower, Uppercase and Capitalized Strings
- Swift enum Examples: case, rawValue
- Swift Padding String Example (toLength)
- Swift UIActivityIndicatorView Example
- Swift UIAlertController: Message Box, Dialog Example
- Swift UIButton, iOS Example
- Swift UICollectionView Tutorial
- Swift UIColor iOS Example: backgroundColor, textColor
- Swift UIFont Example: Name and Size
- Swift UIImageView Example
- Swift UIKit (Index)
- Swift UILabel Tutorial: iPhone App, Uses ViewController
- Swift String
- Swift Remove Duplicates From Array
- Swift If, If Else Example
- Swift Caesar Cipher
- Swift UIStepper Usage
- Swift UISwitch: ViewController
- Swift UITableView Example: UITableViewDataSource
- Swift UITextField: iPhone Text Field Example
- Swift UITextView Example
- Swift UIToolbar Tutorial: UIBarButtonItem
- Swift UIWebView: LoadHtmlString Example
- Swift Var Versus Let Keywords
- Swift Math: abs, sqrt and pow
- Swift Reverse String
- Swift Struct (Class Versus Struct)
- Top 22 Swift Interview Questions (2021)
- Swift Substring Examples
- Swift Switch Statements
- Swift Convert Int to Character: UnicodeScalar, utf8
- Swift Property Examples
- Swift isEmpty String (characters.count Is Zero)
- Swift Recursion
- Swift ROT13 Func
- Swift Convert String to Byte Array: utf8 and UInt8
- Swift Keywords
- Swift Convert String to Int Example
- Swift Join Strings: joined, separator Examples
- Swift String Literal, Interpolation
- Swift Extension Methods: String, Int Extensions
- Swift Characters: String Examples
- Swift Func Examples: Var, Return and Static
- Swift Guard Example: Guard Else, Return
- Swift Fibonacci Numbers
- Swift Trim Strings
- Swift Set Collection: Insert and Contains
- Swift Tuple Examples
- Swift Loops: for, repeat and while Examples
- Swift Split Strings Example (components)
- Swift Array Examples, String Arrays
- Swift UIPickerView Example: UIPickerViewDataSource
- Swift UIProgressView Example: Progress
- Swift UISegmentedControl
- Swift UISlider Example
- Swift Random Numbers: arc4random
- Swift ASCII Table
- Swift Range Examples (index, offsetBy)
- Swift String Length
- Swift Sort String Arrays: Closure Examples
Related Links
Adjectives
Ado
Ai
Android
Angular
Antonyms
Apache
Articles
Asp
Autocad
Automata
Aws
Azure
Basic
Binary
Bitcoin
Blockchain
C
Cassandra
Change
Coa
Computer
Control
Cpp
Create
Creating
C-Sharp
Cyber
Daa
Data
Dbms
Deletion
Devops
Difference
Discrete
Es6
Ethical
Examples
Features
Firebase
Flutter
Fs
Git
Go
Hbase
History
Hive
Hiveql
How
Html
Idioms
Insertion
Installing
Ios
Java
Joomla
Js
Kafka
Kali
Laravel
Logical
Machine
Matlab
Matrix
Mongodb
Mysql
One
Opencv
Oracle
Ordering
Os
Pandas
Php
Pig
Pl
Postgresql
Powershell
Prepositions
Program
Python
React
Ruby
Scala
Selecting
Selenium
Sentence
Seo
Sharepoint
Software
Spellings
Spotting
Spring
Sql
Sqlite
Sqoop
Svn
Swift
Synonyms
Talend
Testng
Types
Uml
Unity
Vbnet
Verbal
Webdriver
What
Wpf