<< Back to SWIFT
Swift Var Versus Let Keywords
Understand the difference between var and let. Review the cannot assign to value error.Var. X is 10. The identifier "x" has a binding to the value 10. As a var, it can be changed to equal 20. As a constant (specified with let) it can never be changed.
With let, we create immutable things (constants) in our Swift code. Generally constants are more durable and understandable in code. But for loops and objects like arrays, var is needed.
Var example. Let us use the var keyword in a simple example. Here we bind the identifier "color" to the string "blue." We then bind "color" to green with a reassignment.
Next: After this example, we try to rewrite the code with let. We use 2 let constants instead of 1 var.
Swift program that uses var
// Create a variable and assign a string literal to it.
var color = "blue"
print(color)
// Change the assigned value.
color = "green"
print(color)
Output
blue
green
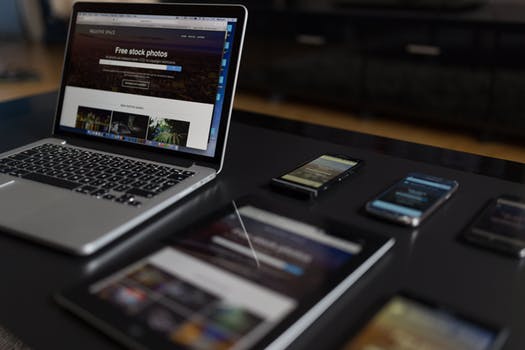
Let error. Now we cause some trouble. We create a constant with the value "blue." But then we try to change its binding to point to "green." This fails and a compile-time error is caused.
Swift program that uses let, causes error
// This part works.
let color = "blue"
print(color)
// But this part does not compile.
// ... A constant cannot be reassigned.
color = "green"
print(color)
Output
Cannot assign to value: 'color' is a 'let' constant
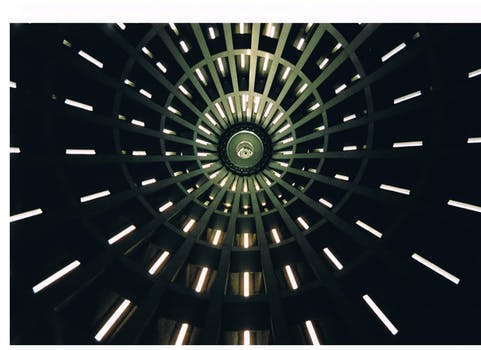
Separate let constants. Here we fix our "cannot assign to value" error. We use two "let" constants. This style of code may lead to better, more durable programs.
Tip: When possible, this style of code is ideal. It means no mistaken assignments to color1 or color2 can occur.
Swift program that uses let, separate bindings
// For constants, we must use separate bindings.
let color1 = "orange"
print(color1)
let color2 = "mauve"
print(color2)
Output
orange
mauve
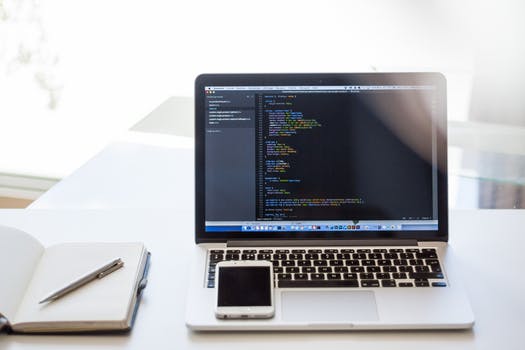
Var argument. A func cannot modify its arguments in Swift (by default). With the var keyword, we specify that an argument can be modified by the method's statements.
Swift program that uses var argument
func incrementId(var id: Int) -> Int {
// Print and increment the var argument.
print(id)
id++
print(id)
return id
}
var id = 10
// Call incrementId with argument of 10.
// ... Print the returned value.
print(incrementId(id))
Output
10
11
11
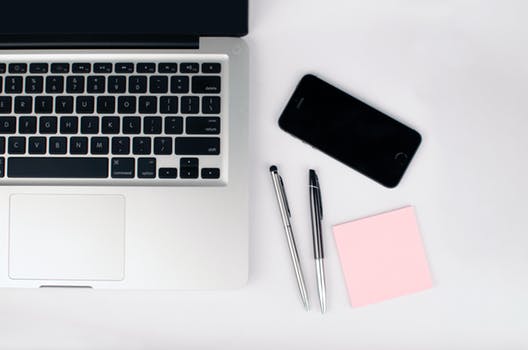
Mutating operator error. Here the incrementId func tries to increment its argument Int. But the argument is not marked with var, so this causes a "mutating operator" error.
Swift program that causes mutating operator error
func incrementId(id: Int) {
// This causes an error.
id++
}
var id = 55
incrementId(id)
Output
Cannot pass immutable value to mutating operator:
'id' is a 'let' constant
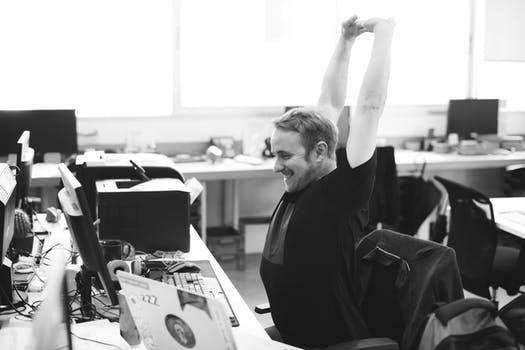
Typically, the let-keyword is best when it can be applied. If a variable is mutated in a program, the var keyword can be used. But immutable things offer advantages and are often preferred.
Related Links:
- Swift String Append Example: reserveCapacity
- Swift File (Read Text File Into String)
- Swift Find Strings: range of Example
- Swift Subscript Example
- Swift hasPrefix and hasSuffix Examples
- Swift Array of Dictionary Elements
- Swift Tutorial
- Swift Odd and Even Numbers
- Swift Operator: Equality, Prefix and Postfix
- Swift Dictionary Examples
- Swift Class Example: init, self
- Swift Combine Arrays: append, contentsOf
- Swift Initialize Array
- Swift Int Examples: Int.max, Int.min
- Swift 2D Array Examples
- Swift Error Handling: try, catch
- Swift Repeat While Loop
- Swift Optional: Nil Examples
- Swift Replace String Example
- Swift Print: Lines, Separator and Terminator
- Swift inout Keyword: Func Example
- Swift Lower, Uppercase and Capitalized Strings
- Swift enum Examples: case, rawValue
- Swift Padding String Example (toLength)
- Swift UIActivityIndicatorView Example
- Swift UIAlertController: Message Box, Dialog Example
- Swift UIButton, iOS Example
- Swift UICollectionView Tutorial
- Swift UIColor iOS Example: backgroundColor, textColor
- Swift UIFont Example: Name and Size
- Swift UIImageView Example
- Swift UIKit (Index)
- Swift UILabel Tutorial: iPhone App, Uses ViewController
- Swift String
- Swift Remove Duplicates From Array
- Swift If, If Else Example
- Swift Caesar Cipher
- Swift UIStepper Usage
- Swift UISwitch: ViewController
- Swift UITableView Example: UITableViewDataSource
- Swift UITextField: iPhone Text Field Example
- Swift UITextView Example
- Swift UIToolbar Tutorial: UIBarButtonItem
- Swift UIWebView: LoadHtmlString Example
- Swift Var Versus Let Keywords
- Swift Math: abs, sqrt and pow
- Swift Reverse String
- Swift Struct (Class Versus Struct)
- Top 22 Swift Interview Questions (2021)
- Swift Substring Examples
- Swift Switch Statements
- Swift Convert Int to Character: UnicodeScalar, utf8
- Swift Property Examples
- Swift isEmpty String (characters.count Is Zero)
- Swift Recursion
- Swift ROT13 Func
- Swift Convert String to Byte Array: utf8 and UInt8
- Swift Keywords
- Swift Convert String to Int Example
- Swift Join Strings: joined, separator Examples
- Swift String Literal, Interpolation
- Swift Extension Methods: String, Int Extensions
- Swift Characters: String Examples
- Swift Func Examples: Var, Return and Static
- Swift Guard Example: Guard Else, Return
- Swift Fibonacci Numbers
- Swift Trim Strings
- Swift Set Collection: Insert and Contains
- Swift Tuple Examples
- Swift Loops: for, repeat and while Examples
- Swift Split Strings Example (components)
- Swift Array Examples, String Arrays
- Swift UIPickerView Example: UIPickerViewDataSource
- Swift UIProgressView Example: Progress
- Swift UISegmentedControl
- Swift UISlider Example
- Swift Random Numbers: arc4random
- Swift ASCII Table
- Swift Range Examples (index, offsetBy)
- Swift String Length
- Swift Sort String Arrays: Closure Examples
Related Links
Adjectives
Ado
Ai
Android
Angular
Antonyms
Apache
Articles
Asp
Autocad
Automata
Aws
Azure
Basic
Binary
Bitcoin
Blockchain
C
Cassandra
Change
Coa
Computer
Control
Cpp
Create
Creating
C-Sharp
Cyber
Daa
Data
Dbms
Deletion
Devops
Difference
Discrete
Es6
Ethical
Examples
Features
Firebase
Flutter
Fs
Git
Go
Hbase
History
Hive
Hiveql
How
Html
Idioms
Insertion
Installing
Ios
Java
Joomla
Js
Kafka
Kali
Laravel
Logical
Machine
Matlab
Matrix
Mongodb
Mysql
One
Opencv
Oracle
Ordering
Os
Pandas
Php
Pig
Pl
Postgresql
Powershell
Prepositions
Program
Python
React
Ruby
Scala
Selecting
Selenium
Sentence
Seo
Sharepoint
Software
Spellings
Spotting
Spring
Sql
Sqlite
Sqoop
Svn
Swift
Synonyms
Talend
Testng
Types
Uml
Unity
Vbnet
Verbal
Webdriver
What
Wpf