<< Back to SWIFT
Swift Range Examples (index, offsetBy)
Use ranges with startIndex and endIndex. Call the index func and specify offsetBy.Range. The numbers 0, 1 and 2 are sequential—this is a range. In Swift we can create a range with special syntax. We can even loop over a range.
Bounds. With startIndex and endIndex, we can test the bounds of a range. Count returns the number of elements. And index() with offsetBy changes the start or end.
Loop example. Here we create a constant range of the values 0, 1 and 2. With this syntax, the 0 and the 2 are both included. We use a for-in loop over the range.
Here: The term "range" is just an identifier for the range of values. This syntax includes 0 and 2.
Let: A range can be specified directly in the loop expression (after in). In this usually a clearer way to write a loop.
Swift program that uses range syntax
// Create a range.
let range = 0...2
// Use a for-in loop over the range.
for value in range {
print(value)
}
Output
0
1
2
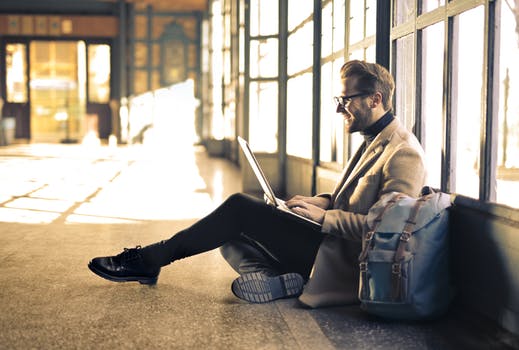
StartIndex, endIndex. This example shows the startIndex and endIndex properties. These return the first and last indexes in the range.
Note: There is some complexity here. EndIndex here is one greater than the last included value.
Count: With count, we get an Int that is equal to the number of values within the range. Here that is 6.
IntSwift program that uses startIndex, endIndex, count
let range = 5...10
// A range has a startIndex and an endIndex.
// ... The endIndex is one more than the last included index.
print(range.startIndex)
print(range.endIndex)
// Count returns the number of elements in the range.
print(range.count)
Output
ClosedRangeIndex<Int>(_value:
Swift._ClosedRangeIndexRepresentation<Swift.Int>.inRange(5))
ClosedRangeIndex<Int>(_value:
Swift._ClosedRangeIndexRepresentation<Swift.Int>.pastEnd)
6
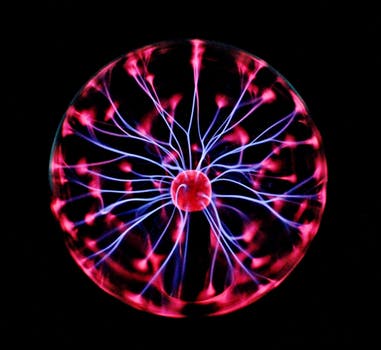
Half-open ranges. In Swift we can create ranges that do not include the second (end) number. These use a "less than" sign. These are called half-open ranges.
Tip: With this operator, we can get a range of all the indexes in an array without subtracting one from the array's count.
Quote: [A range] is said to be half-open because it contains its first value, but not its final value (Swift Programming Language).
Swift program that uses half-open range operator
// This range does not include the last number.
let values = 0..<5
// Loop over values in the exclusive-end range.
for value in values {
print(value)
}
Output
0
1
2
3
4
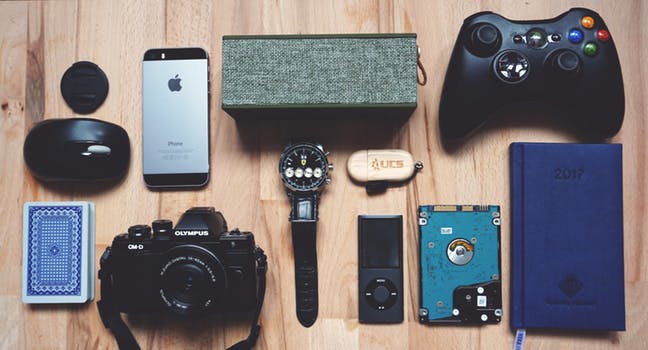
Negative numbers. Usually ranges have positive starts and ends. But we can have negative numbers in our ranges. The start (even if negative) must be less than the end.
Note: The range here is specified directly within the for-in loop. This makes for simpler code.
Swift program that uses negative number in range
// A range can start at a negative number.
for id in -4...2 {
print(id)
}
Output
-4
-3
-2
-1
0
1
2
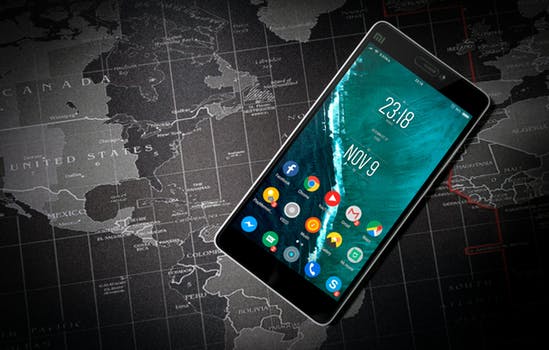
Index, offsetBy. Sometimes we want to create a range based on an existing one. We can use index (with offsetBy) on the startIndex or endIndex. Here we increment the range's start by 2.
Tip: With index() we can reduce an index by passing a negative Int as the second argument.
Note: When we print a range, we see information about the range, not just a start and end integer pair.
Swift program that advances range
var range = 0...3
// Create a new range based on an existing range.
// ... Advance the startIndex by 2.
var x = range.index(range.startIndex, offsetBy: 2)..<range.endIndex
// Display ranges.
print(range)
print(x)
Output
0...3
ClosedRangeIndex<Int>(_value:
Swift._ClosedRangeIndexRepresentation<Swift.Int>.inRange(
2))..<ClosedRangeIndex<Int>(_value:
Swift._ClosedRangeIndexRepresentation<Swift.Int>.pastEnd)
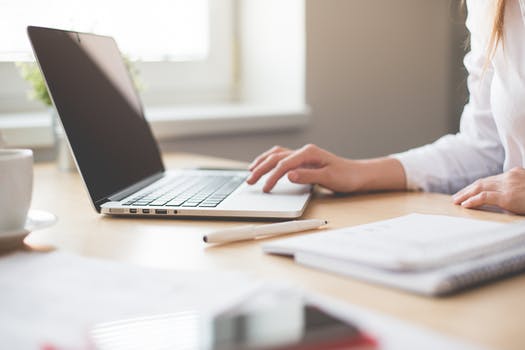
Invalid range. A range's start must be less than or equal to its end. It cannot be larger. Here we encounter the "can't form Range" error in a compiled Swift program.
Swift program that has invalid range
// This will cause an error.
var range = 100...0
// Not reached.
print(range)
Output
fatal error: Can't form Range with end < start
(lldb)
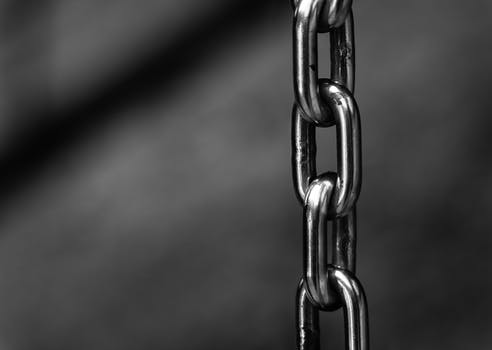
Range of array indexes. A half-open range can return all valid indexes for an array. The end is one less than the count. Here we use for-in on all array indexes.
ArraySwift program that uses range of indexes, array
// This string array has three elements.
let animals: [String] = ["bird", "cat", "fox"]
// Loop through all indexes in the array.
for index in 0..<animals.count {
print(index)
print(animals[index])
}
Output
0
bird
1
cat
2
fox
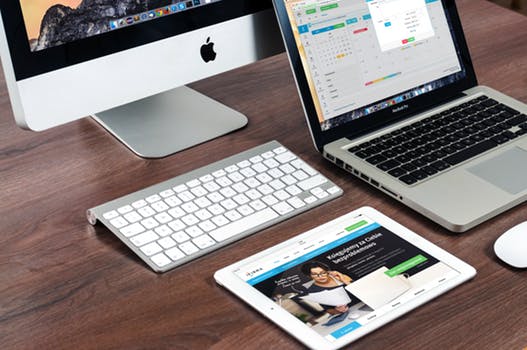
Substring note. Ranges are useful when taking substrings. The index() offsetBy method helps us index into a string. And then we can access the string by a range.
Substring
A history lesson. Range syntax has been changed in Swift 2 and Swift 3. This is confusing. But in many ways the newer syntax forms are clearer and easier to use.
Ranges are used with arrays and strings. They can create a sequence for a for-in loop. Closed ranges, and half-open ones, are common in Swift programs.
Related Links:
- Swift String Append Example: reserveCapacity
- Swift File (Read Text File Into String)
- Swift Find Strings: range of Example
- Swift Subscript Example
- Swift hasPrefix and hasSuffix Examples
- Swift Array of Dictionary Elements
- Swift Tutorial
- Swift Odd and Even Numbers
- Swift Operator: Equality, Prefix and Postfix
- Swift Dictionary Examples
- Swift Class Example: init, self
- Swift Combine Arrays: append, contentsOf
- Swift Initialize Array
- Swift Int Examples: Int.max, Int.min
- Swift 2D Array Examples
- Swift Error Handling: try, catch
- Swift Repeat While Loop
- Swift Optional: Nil Examples
- Swift Replace String Example
- Swift Print: Lines, Separator and Terminator
- Swift inout Keyword: Func Example
- Swift Lower, Uppercase and Capitalized Strings
- Swift enum Examples: case, rawValue
- Swift Padding String Example (toLength)
- Swift UIActivityIndicatorView Example
- Swift UIAlertController: Message Box, Dialog Example
- Swift UIButton, iOS Example
- Swift UICollectionView Tutorial
- Swift UIColor iOS Example: backgroundColor, textColor
- Swift UIFont Example: Name and Size
- Swift UIImageView Example
- Swift UIKit (Index)
- Swift UILabel Tutorial: iPhone App, Uses ViewController
- Swift String
- Swift Remove Duplicates From Array
- Swift If, If Else Example
- Swift Caesar Cipher
- Swift UIStepper Usage
- Swift UISwitch: ViewController
- Swift UITableView Example: UITableViewDataSource
- Swift UITextField: iPhone Text Field Example
- Swift UITextView Example
- Swift UIToolbar Tutorial: UIBarButtonItem
- Swift UIWebView: LoadHtmlString Example
- Swift Var Versus Let Keywords
- Swift Math: abs, sqrt and pow
- Swift Reverse String
- Swift Struct (Class Versus Struct)
- Top 22 Swift Interview Questions (2021)
- Swift Substring Examples
- Swift Switch Statements
- Swift Convert Int to Character: UnicodeScalar, utf8
- Swift Property Examples
- Swift isEmpty String (characters.count Is Zero)
- Swift Recursion
- Swift ROT13 Func
- Swift Convert String to Byte Array: utf8 and UInt8
- Swift Keywords
- Swift Convert String to Int Example
- Swift Join Strings: joined, separator Examples
- Swift String Literal, Interpolation
- Swift Extension Methods: String, Int Extensions
- Swift Characters: String Examples
- Swift Func Examples: Var, Return and Static
- Swift Guard Example: Guard Else, Return
- Swift Fibonacci Numbers
- Swift Trim Strings
- Swift Set Collection: Insert and Contains
- Swift Tuple Examples
- Swift Loops: for, repeat and while Examples
- Swift Split Strings Example (components)
- Swift Array Examples, String Arrays
- Swift UIPickerView Example: UIPickerViewDataSource
- Swift UIProgressView Example: Progress
- Swift UISegmentedControl
- Swift UISlider Example
- Swift Random Numbers: arc4random
- Swift ASCII Table
- Swift Range Examples (index, offsetBy)
- Swift String Length
- Swift Sort String Arrays: Closure Examples
Related Links
Adjectives
Ado
Ai
Android
Angular
Antonyms
Apache
Articles
Asp
Autocad
Automata
Aws
Azure
Basic
Binary
Bitcoin
Blockchain
C
Cassandra
Change
Coa
Computer
Control
Cpp
Create
Creating
C-Sharp
Cyber
Daa
Data
Dbms
Deletion
Devops
Difference
Discrete
Es6
Ethical
Examples
Features
Firebase
Flutter
Fs
Git
Go
Hbase
History
Hive
Hiveql
How
Html
Idioms
Insertion
Installing
Ios
Java
Joomla
Js
Kafka
Kali
Laravel
Logical
Machine
Matlab
Matrix
Mongodb
Mysql
One
Opencv
Oracle
Ordering
Os
Pandas
Php
Pig
Pl
Postgresql
Powershell
Prepositions
Program
Python
React
Ruby
Scala
Selecting
Selenium
Sentence
Seo
Sharepoint
Software
Spellings
Spotting
Spring
Sql
Sqlite
Sqoop
Svn
Swift
Synonyms
Talend
Testng
Types
Uml
Unity
Vbnet
Verbal
Webdriver
What
Wpf