<< Back to SWIFT
Swift Func Examples: Var, Return and Static
Use funcs. Specify the var keyword to create local variables and return values.Funcs. A method does something. It computes the area of a circle. It calculates pi. In Swift we specify funcs with zero or more arguments and return values.
Func features. With InOut we have output arguments—changes are retained at the caller. We can return tuples and optionals. We can apply lambda expressions.
Initial example. Here we introduce a simple function: computeArea. It receives two arguments, the values "x" and "y." It returns an Int.
Return: ComputeArea returns the product of the two parameters. The expression is computed and the value is returned.
Var: We store the result of computeArea in a variable we declare with var. We then use print() to display it.
Var, LetSwift program that uses func
func computeArea(x: Int, y: Int) -> Int {
return x * y
}
// Call the example func.
var result = computeArea(10, y: 3)
print(result)
Output
30
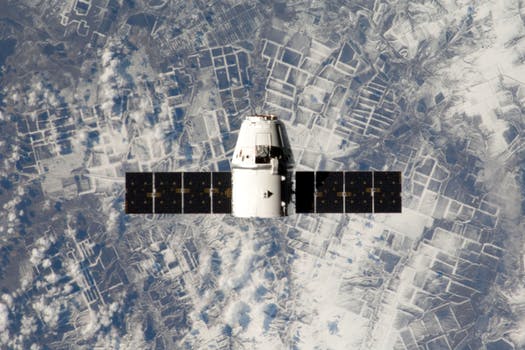
External parameter names. A parameter can have two names in Swift. The first name specified is optional—this is an external parameter name.
Note: An external parameter name is used when calling the func. It can make function calls more readable.
Here: The "printMessage" func uses an external parameter name. We "print a message about" something.
Swift program that uses external parameter name
func printMessage(about value: String) {
// The identifier "about" is used for external labels.
// The identifier "value" is used in the func.
print("Your message is about \(value)")
}
// Test our external parameter name.
printMessage(about: "Swift")
printMessage(about: "turtles")
printMessage(about: "cats")
Output
Your message is about Swift
Your message is about turtles
Your message is about cats
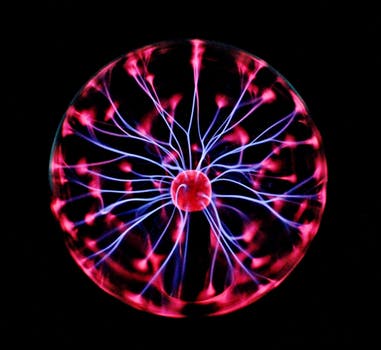
Default parameters. A parameter can have a default value. We use an assignment expression in the formal parameter list. Here the value parameter is 100 when not specified in the caller.
Swift program that uses default parameter value
func printValue(value: Int = 100) {
// Write value to the console.
print("Value is \(value)")
}
// The default parameter value is used.
printValue()
printValue(200)
Output
Value is 100
Value is 200
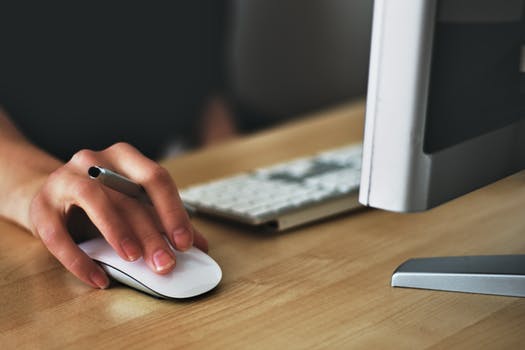
Variable argument list. Sometimes we want a method that receives any number of arguments. This is a variadic func. We use an ellipsis to indicate a variadic method.
Then: We can use the argument as an array in the method body. Here we use a for-in loop to iterate the array.
Swift program that uses variadic func
func sumStringLengths(values: String...) -> Int {
// Loop over string array and sum String lengths.
var sum = 0
for value in values {
sum += value.characters.count
}
return sum
}
// Call the method with 2 arguments.
let result = sumStringLengths("bird", "cat")
print(result)
Output
7
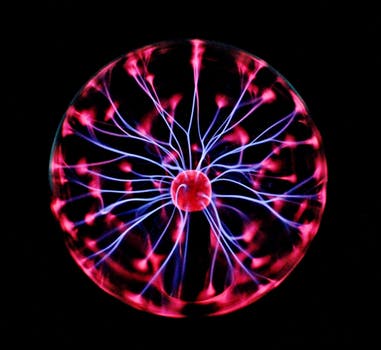
Constant arguments. In Swift, arguments are by default constant. We cannot reassign an argument in the func body. This helps prevent mistakes in coding.
Tip: Please use the var keyword to have a non-constant argument. Or rewrite the method to use a local variable.
Swift program that causes error, assigns to argument
func printExample(argument: Int) {
// This will not compile.
argument = 10
}
printExample(20)
Output
main.swift:3:14: Cannot assign to 'let' value 'argument'
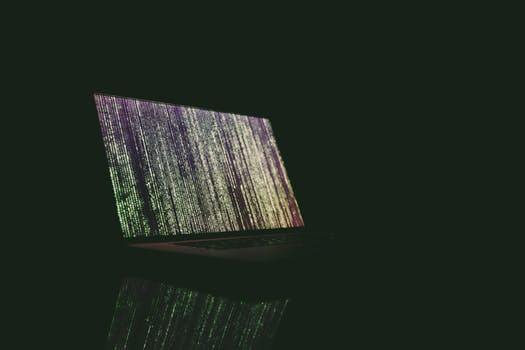
Static. This program uses a static func. We introduce the Music class, and provide a static play() method. Play() changes the static field named "count" when called.
ClassNote: Static things are tied to types, not instances of those types. So only one "count" static field can exist in this program.
Swift program that uses static method, var
class Music {
static var count = 0
static func play() {
print("Music.play called: \(count)")
// Increment static var.
count++
}
}
// Call static method.
Music.play()
Music.play()
Output
Music.play called: 0
Music.play called: 1
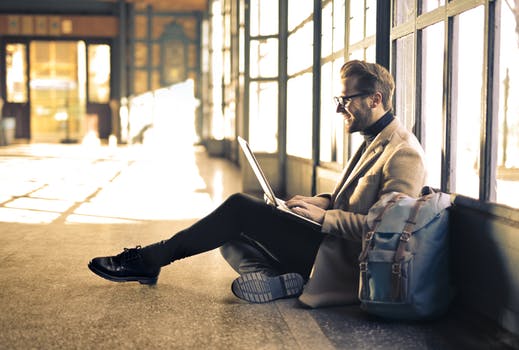
Inout. This keyword is used on a parameter. When modified, an inout parameter affects the variable at the calling location. One memory region is shared.
inout
Multiple return values. A func can return more than one value. We specify a tuple as its return value. Two or more values (of any type) can then be returned.
Tuple
Extensions. An extension may add funcs to a class. Even a type like String or Int can have methods added with an extension block.
ExtensionRecursion. Sometimes a problem requires a search to be solved. With recursion, we can exhaustively search. We implement a recursive algorithm to count coins in Swift.
RecursionA summary. A key part of a modern programming language is its function call syntax. In Swift we find powerful, new features like multiple return values and optional tuples.
Related Links:
- Swift String Append Example: reserveCapacity
- Swift File (Read Text File Into String)
- Swift Find Strings: range of Example
- Swift Subscript Example
- Swift hasPrefix and hasSuffix Examples
- Swift Array of Dictionary Elements
- Swift Tutorial
- Swift Odd and Even Numbers
- Swift Operator: Equality, Prefix and Postfix
- Swift Dictionary Examples
- Swift Class Example: init, self
- Swift Combine Arrays: append, contentsOf
- Swift Initialize Array
- Swift Int Examples: Int.max, Int.min
- Swift 2D Array Examples
- Swift Error Handling: try, catch
- Swift Repeat While Loop
- Swift Optional: Nil Examples
- Swift Replace String Example
- Swift Print: Lines, Separator and Terminator
- Swift inout Keyword: Func Example
- Swift Lower, Uppercase and Capitalized Strings
- Swift enum Examples: case, rawValue
- Swift Padding String Example (toLength)
- Swift UIActivityIndicatorView Example
- Swift UIAlertController: Message Box, Dialog Example
- Swift UIButton, iOS Example
- Swift UICollectionView Tutorial
- Swift UIColor iOS Example: backgroundColor, textColor
- Swift UIFont Example: Name and Size
- Swift UIImageView Example
- Swift UIKit (Index)
- Swift UILabel Tutorial: iPhone App, Uses ViewController
- Swift String
- Swift Remove Duplicates From Array
- Swift If, If Else Example
- Swift Caesar Cipher
- Swift UIStepper Usage
- Swift UISwitch: ViewController
- Swift UITableView Example: UITableViewDataSource
- Swift UITextField: iPhone Text Field Example
- Swift UITextView Example
- Swift UIToolbar Tutorial: UIBarButtonItem
- Swift UIWebView: LoadHtmlString Example
- Swift Var Versus Let Keywords
- Swift Math: abs, sqrt and pow
- Swift Reverse String
- Swift Struct (Class Versus Struct)
- Top 22 Swift Interview Questions (2021)
- Swift Substring Examples
- Swift Switch Statements
- Swift Convert Int to Character: UnicodeScalar, utf8
- Swift Property Examples
- Swift isEmpty String (characters.count Is Zero)
- Swift Recursion
- Swift ROT13 Func
- Swift Convert String to Byte Array: utf8 and UInt8
- Swift Keywords
- Swift Convert String to Int Example
- Swift Join Strings: joined, separator Examples
- Swift String Literal, Interpolation
- Swift Extension Methods: String, Int Extensions
- Swift Characters: String Examples
- Swift Func Examples: Var, Return and Static
- Swift Guard Example: Guard Else, Return
- Swift Fibonacci Numbers
- Swift Trim Strings
- Swift Set Collection: Insert and Contains
- Swift Tuple Examples
- Swift Loops: for, repeat and while Examples
- Swift Split Strings Example (components)
- Swift Array Examples, String Arrays
- Swift UIPickerView Example: UIPickerViewDataSource
- Swift UIProgressView Example: Progress
- Swift UISegmentedControl
- Swift UISlider Example
- Swift Random Numbers: arc4random
- Swift ASCII Table
- Swift Range Examples (index, offsetBy)
- Swift String Length
- Swift Sort String Arrays: Closure Examples
Related Links
Adjectives
Ado
Ai
Android
Angular
Antonyms
Apache
Articles
Asp
Autocad
Automata
Aws
Azure
Basic
Binary
Bitcoin
Blockchain
C
Cassandra
Change
Coa
Computer
Control
Cpp
Create
Creating
C-Sharp
Cyber
Daa
Data
Dbms
Deletion
Devops
Difference
Discrete
Es6
Ethical
Examples
Features
Firebase
Flutter
Fs
Git
Go
Hbase
History
Hive
Hiveql
How
Html
Idioms
Insertion
Installing
Ios
Java
Joomla
Js
Kafka
Kali
Laravel
Logical
Machine
Matlab
Matrix
Mongodb
Mysql
One
Opencv
Oracle
Ordering
Os
Pandas
Php
Pig
Pl
Postgresql
Powershell
Prepositions
Program
Python
React
Ruby
Scala
Selecting
Selenium
Sentence
Seo
Sharepoint
Software
Spellings
Spotting
Spring
Sql
Sqlite
Sqoop
Svn
Swift
Synonyms
Talend
Testng
Types
Uml
Unity
Vbnet
Verbal
Webdriver
What
Wpf