<< Back to SWIFT
Swift Optional: Nil Examples
Use optional values. An optional may be nil, which means it has no value.Optional. Sometimes a func fails. It has no valid value (like an Int) to return. In Swift, this method can return an optional Int, not an Int.
No special codes. When a func has no value to return, we do not need to know a special "failure" code. Instead we test the returned optional value. We see if any value exists.
An example. This program shows a func that returns an optional String. This is specified as a "String?" return value. The func returns a String or nil.
If: The program calls the computeName() method and tests the optional String in an if-statement.
And: The exclamation mark is used to get the value from an optional that is not nil.
Swift program that uses optionals
func computeName(id: Int) -> String? {
// This returns an optional string.
switch id {
case 0:
return "Car"
case 1:
return "Fish"
case 2:
return "House"
default:
return nil
}
}
// Get optional string from this func.
let name1 = computeName(id: 1)
// See if optional string is not nil.
if name1 != nil {
// Use exclamation point to get string from optional.
let value = name1!
print(value)
}
Output
Fish
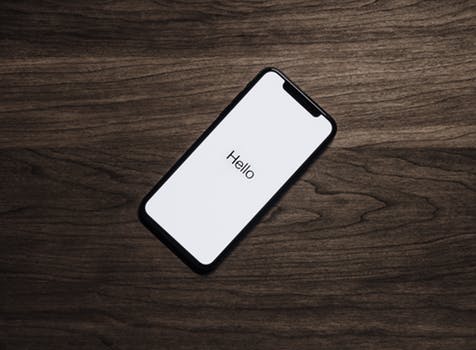
Optional binding. This feature lets us assign a name to an optional in an if-statement. Inside the if-block, we can access the value (like a String) with no special syntax.
Here: The "id" constant is assigned to the String value, not an optional. So we directly use it.
Swift program that uses optional binding
func computeId(id: Int) -> String? {
// Return an optional string.
if id == 0 {
return nil
}
else {
return "1"
}
}
if let id = computeId(id: 10) {
// Use id as a string, not an optional string.
// ... No exclamation point is needed.
print(id)
}
if let id = computeId(id: 0) {
// This is not reached.
print(false)
}
Output
1
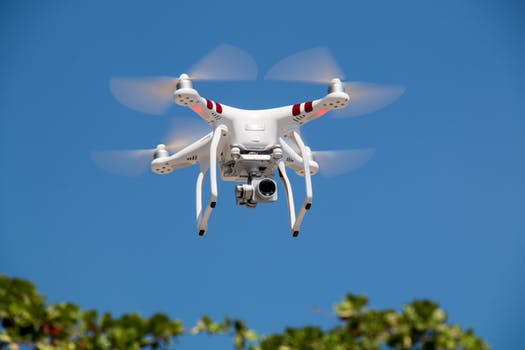
Field. A class can have an optional field. No special init method is required to initialize an optional field. These fields begin as nil values.
Class: initHowever: The color field on Box is an optional String. It begins life as nil, but we change it to "Blue" and then test its value.
Swift program that uses optional field
class Box {
var color: String?
}
// Create Box instance.
var box = Box()
// Optional is nil.
if let c = box.color {
print(0)
}
// Assign optional to String value.
box.color = "Blue"
// Use "if let" statement.
if let c = box.color {
print("Box has color \(c)")
// The optional can be accessed with an exclamation mark.
print(box.color!)
}
Output
Box has color Blue
Blue
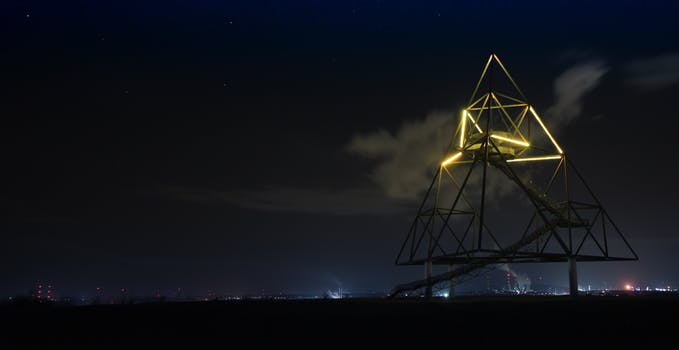
Chaining. With optional chaining, we can safely access nested optionals. If an early access is nil, further accesses will not be done. The end result is nil unless a valid result is found.
Box: This class contains an optional Side class instance. It requires no init method because its only field is optional.
Side: This has an optional pixels Int. We can access a Side's pixels through a special expression.
Swift program that uses optional chains, question mark
class Box
{
var side: Side?
}
class Side
{
var pixels: Int?
}
// Create instance of class.
var example = Box()
// Access a field on a nil optional.
let pixels = example.side?.pixels
print(pixels) // Does not exist.
// Initialize the optional field.
example.side = Side()
// Assign field on the instance.
example.side!.pixels = 10
// Access field through nested optionals.
print(example.side!.pixels!)
Output
nil
10
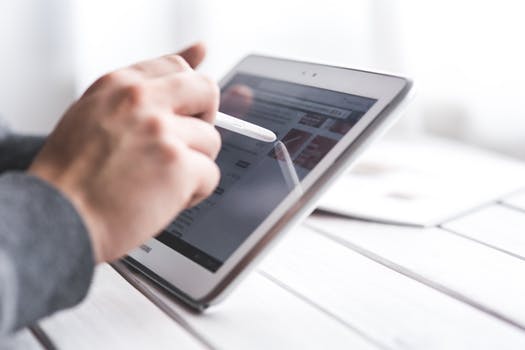
Subscripts. A subscript (like the lookup method of a dictionary) can return an optional value. Here we use optional syntax to increment and decrement values in a dictionary.
Result: No errors occur even if the value does not exist. Instead the expression does nothing.
Swift program that uses optionals with dictionary values
var colors = ["Blue": 140, "Red": 30]
// Increment and decrement optional values.
colors["Blue"]? += 1
colors["Red"]? -= 1
// This has no effect.
colors["Hello"]? += 1
// Display dictionary.
print(colors)
Output
["Blue": 141, "Red": 29]
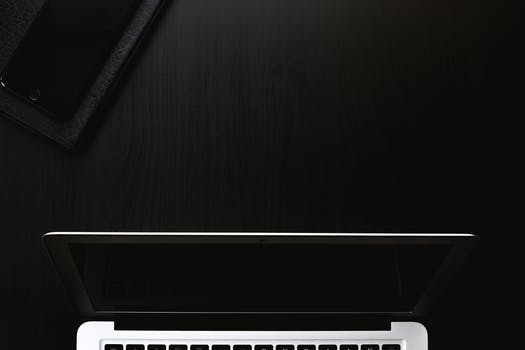
Convert errors. With the "try!" and "try?" syntax, we can convert an error thrown by a method into an optional. This makes calling exception-throwing funcs easier.
Try: convert errors
An optional review. With optionals, Swift makes failure values consistent. It means we have a unified, reliable way to test for "no value." This makes programs clearer.
Related Links:
- Swift String Append Example: reserveCapacity
- Swift File (Read Text File Into String)
- Swift Find Strings: range of Example
- Swift Subscript Example
- Swift hasPrefix and hasSuffix Examples
- Swift Array of Dictionary Elements
- Swift Tutorial
- Swift Odd and Even Numbers
- Swift Operator: Equality, Prefix and Postfix
- Swift Dictionary Examples
- Swift Class Example: init, self
- Swift Combine Arrays: append, contentsOf
- Swift Initialize Array
- Swift Int Examples: Int.max, Int.min
- Swift 2D Array Examples
- Swift Error Handling: try, catch
- Swift Repeat While Loop
- Swift Optional: Nil Examples
- Swift Replace String Example
- Swift Print: Lines, Separator and Terminator
- Swift inout Keyword: Func Example
- Swift Lower, Uppercase and Capitalized Strings
- Swift enum Examples: case, rawValue
- Swift Padding String Example (toLength)
- Swift UIActivityIndicatorView Example
- Swift UIAlertController: Message Box, Dialog Example
- Swift UIButton, iOS Example
- Swift UICollectionView Tutorial
- Swift UIColor iOS Example: backgroundColor, textColor
- Swift UIFont Example: Name and Size
- Swift UIImageView Example
- Swift UIKit (Index)
- Swift UILabel Tutorial: iPhone App, Uses ViewController
- Swift String
- Swift Remove Duplicates From Array
- Swift If, If Else Example
- Swift Caesar Cipher
- Swift UIStepper Usage
- Swift UISwitch: ViewController
- Swift UITableView Example: UITableViewDataSource
- Swift UITextField: iPhone Text Field Example
- Swift UITextView Example
- Swift UIToolbar Tutorial: UIBarButtonItem
- Swift UIWebView: LoadHtmlString Example
- Swift Var Versus Let Keywords
- Swift Math: abs, sqrt and pow
- Swift Reverse String
- Swift Struct (Class Versus Struct)
- Top 22 Swift Interview Questions (2021)
- Swift Substring Examples
- Swift Switch Statements
- Swift Convert Int to Character: UnicodeScalar, utf8
- Swift Property Examples
- Swift isEmpty String (characters.count Is Zero)
- Swift Recursion
- Swift ROT13 Func
- Swift Convert String to Byte Array: utf8 and UInt8
- Swift Keywords
- Swift Convert String to Int Example
- Swift Join Strings: joined, separator Examples
- Swift String Literal, Interpolation
- Swift Extension Methods: String, Int Extensions
- Swift Characters: String Examples
- Swift Func Examples: Var, Return and Static
- Swift Guard Example: Guard Else, Return
- Swift Fibonacci Numbers
- Swift Trim Strings
- Swift Set Collection: Insert and Contains
- Swift Tuple Examples
- Swift Loops: for, repeat and while Examples
- Swift Split Strings Example (components)
- Swift Array Examples, String Arrays
- Swift UIPickerView Example: UIPickerViewDataSource
- Swift UIProgressView Example: Progress
- Swift UISegmentedControl
- Swift UISlider Example
- Swift Random Numbers: arc4random
- Swift ASCII Table
- Swift Range Examples (index, offsetBy)
- Swift String Length
- Swift Sort String Arrays: Closure Examples
Related Links
Adjectives
Ado
Ai
Android
Angular
Antonyms
Apache
Articles
Asp
Autocad
Automata
Aws
Azure
Basic
Binary
Bitcoin
Blockchain
C
Cassandra
Change
Coa
Computer
Control
Cpp
Create
Creating
C-Sharp
Cyber
Daa
Data
Dbms
Deletion
Devops
Difference
Discrete
Es6
Ethical
Examples
Features
Firebase
Flutter
Fs
Git
Go
Hbase
History
Hive
Hiveql
How
Html
Idioms
Insertion
Installing
Ios
Java
Joomla
Js
Kafka
Kali
Laravel
Logical
Machine
Matlab
Matrix
Mongodb
Mysql
One
Opencv
Oracle
Ordering
Os
Pandas
Php
Pig
Pl
Postgresql
Powershell
Prepositions
Program
Python
React
Ruby
Scala
Selecting
Selenium
Sentence
Seo
Sharepoint
Software
Spellings
Spotting
Spring
Sql
Sqlite
Sqoop
Svn
Swift
Synonyms
Talend
Testng
Types
Uml
Unity
Vbnet
Verbal
Webdriver
What
Wpf