<< Back to VBNET
VB.NET DateTime Examples
Represent dates with the DateTime type. Access DateTime constructors and properties.DateTime. This Structure represents a point in time. It stores times, like 12 AM, and dates like yesterday. With TimeSpan we capture time differences.
TimeSpanFeatures. Methods are used to compute relative times such as yesterday and tomorrow. DateTime can be formatted—this changes it to a String.
Format
New instance. A DateTime instance can be created with the New operator. There are many overloads. But the overload shown here accepts 3 arguments.
Arguments: The arguments here are the year, the month, and the day of that month. The value 2 stands for February.
Warning: If you pass an invalid argument (one that no date could have), you will get an ArgumentOutOfRangeException.
VB.NET program that uses constructor
Module Module1
Sub Main()
Dim value As DateTime = New DateTime(2017, 2, 1)
' Display the date.
Console.WriteLine(value)
' Test the year.
If value.Year = 2017 Then
Console.WriteLine("Year is 2017")
End If
End Sub
End Module
Output
2/1/2017 12:00:00 AM
Year is 2017
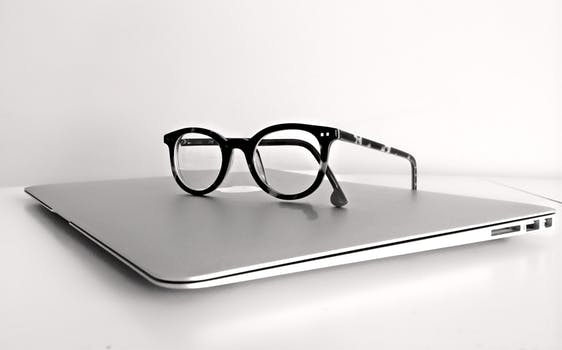
Yesterday. To get yesterday, we can access the Today property and then add negative one days to it. This is equivalent to subtracting one day from the DateTime instance.
VB.NET program that computes yesterday
Module Module1
Sub Main()
' Write the today value.
Console.WriteLine("Today: {0}", DateTime.Today)
' Subtract one day.
Dim yesterday As DateTime = DateTime.Today.AddDays(-1)
' Write the yesterday value.
Console.WriteLine("Yesterday: {0}", yesterday)
End Sub
End Module
Output
Today: 1/17/2010 12:00:00 AM
Yesterday: 1/16/2010 12:00:00 AM
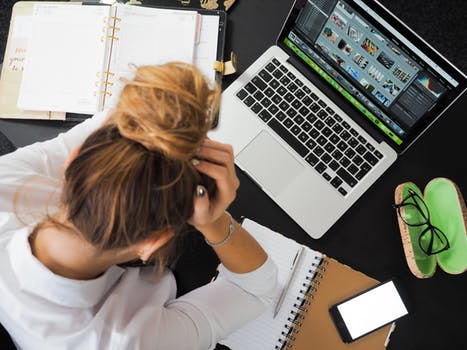
Tomorrow. This is a good day to schedule less pleasurable tasks. To compute tomorrow, we add one day with Add Days() on the value returned by the Today property.
Note: The output of the program will be different depending on when you run it.
VB.NET program that computes tomorrow
Module Module1
Sub Main()
' Write Today.
Console.WriteLine("Today: {0}", DateTime.Today)
' Add one to Today to get tomorrow.
Dim tomorrow As DateTime = DateTime.Today.AddDays(1)
' Write.
Console.WriteLine("Tomorrow: {0}", tomorrow)
End Sub
End Module
Output
Today: 1/17/2010 12:00:00 AM
Tomorrow: 1/18/2010 12:00:00 AM
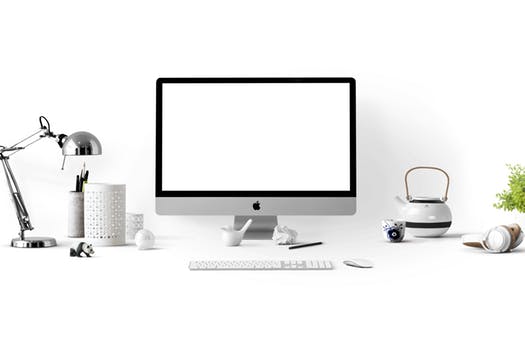
First day. Getting the first day in a year is useful. We defines two new methods to get the first day in a year. When no argument is specified, the current year (for Today) is used.
VB.NET program that finds first day
Module Module1
Sub Main()
' Write first day of current year.
Console.WriteLine("First day: {0}", FirstDayOfYear)
' Write first day of 1999.
Dim y As New DateTime(1999, 6, 1)
Console.WriteLine("First day of 1999: {0}", FirstDayOfYear(y))
End Sub
''' <summary>
''' Get first day of the current year.
''' </summary>
Private Function FirstDayOfYear() As DateTime
Return FirstDayOfYear(DateTime.Today)
End Function
''' <summary>
''' Get first day of the specified year.
''' </summary>
Private Function FirstDayOfYear(ByVal y As DateTime) As DateTime
Return New DateTime(y.Year, 1, 1)
End Function
End Module
Output
First day: 1/1/2010 12:00:00 AM
First day of 1999: 1/1/1999 12:00:00 AM
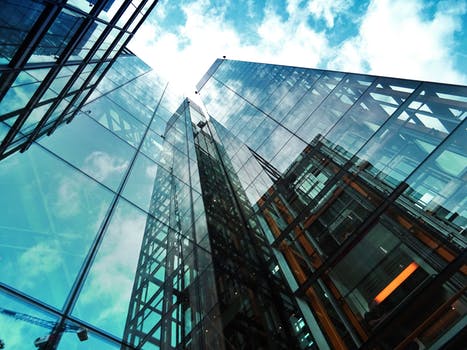
Last day. Here we compute the last day in a year. This may help with year-based record keeping. The method gets the first day of the next year, and then subtracts one day.
Tip: Please see also the IsLeapYear Function, which can tell us whether an additional day is in the year or not.
VB.NET program that computes last day
Module Module1
Sub Main()
' Write current last day.
Console.WriteLine("Last day: {0}", LastDayOfYear)
' Write last day of 1999.
Dim d As New DateTime(1999, 6, 1)
Console.WriteLine("Last day of 1999: {0}", LastDayOfYear(d))
End Sub
''' <summary>
''' Get last day of the current year.
''' </summary>
Private Function LastDayOfYear() As DateTime
Return LastDayOfYear(DateTime.Today)
End Function
''' <summary>
''' Get last day of the specified year.
''' </summary>
Private Function LastDayOfYear(ByVal d As DateTime) As DateTime
Dim time As New DateTime((d.Year + 1), 1, 1)
Return time.AddDays(-1)
End Function
End Module
Output
Last day: 12/31/2010 12:00:00 AM
Last day of 1999: 12/31/1999 12:00:00 AM
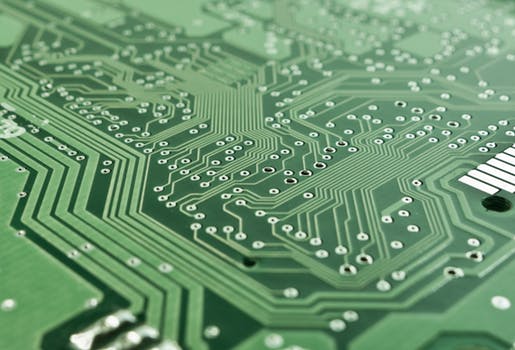
DaysInMonth. Months contain different numbers of days. With the shared DaysInMonth Function, we get day-counts for a month in a specific year. The 2 means February.
VB.NET program that uses DaysInMonth
Module Module1
Sub Main()
' There are 28 days in February.
Dim count As Integer = DateTime.DaysInMonth(2014, 2)
Console.WriteLine(count)
' Count days in March of 2014.
Dim count2 As Integer = DateTime.DaysInMonth(2014, 3)
Console.WriteLine(count2)
End Sub
End Module
Output
28
31
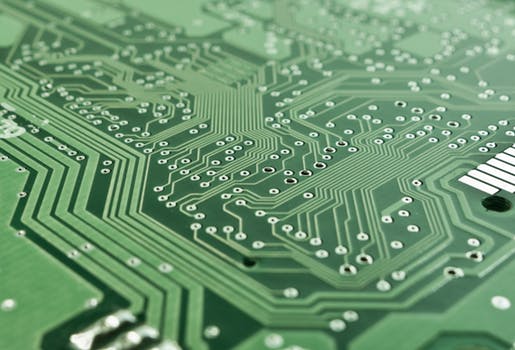
Get DayOfWeek. Every day in the future of our universe will have a day of week—like Monday or Tuesday. If you have a special day you need to test for, use the DayOfWeek property.
Info: This property returns a DayOfWeek Enum value. We can test it against a DayOfWeek enumerated constant.
EnumVB.NET program that gets day of week
Module Module1
Sub Main()
' Get current day of the week.
Dim day As DayOfWeek = DateTime.Today.DayOfWeek
' Write the current day.
Console.WriteLine("TODAY IS {0}", day)
End Sub
End Module
Output
TODAY IS Wednesday
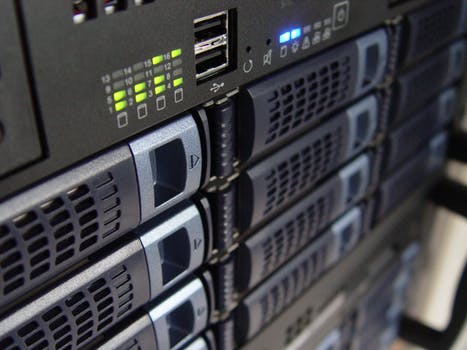
DateString. This property, only offered in VB.NET, returns the current date in String representation. Usually, DateTime-based methods are preferable—they are more standard.
Internals: DateString simply accesses the Today property on DateTime, and then it calls ToString with a format string.
Tip: Many global functions in the VB.NET language are implemented with the standard .NET libraries.
VB.NET program that uses DateString
Module Module1
Sub Main()
Console.WriteLine(DateString)
End Sub
End Module
Output
05-18-2011
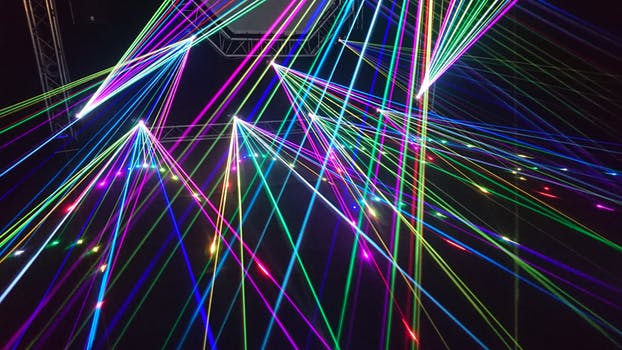
Date. This is another VB.NET special type. It aliases the DateTime type and has all the same methods. We can use Date interchangeably with DateTime.
Note: Usually DateTime is preferred in .NET programs as it is standard—all C# programs use DateTime not Date.
VB.NET program that uses Date type
Module Module1
Sub Main()
' The Date type is the same as the DateTime type.
Dim d As Date = New Date(2014, 10, 6)
Dim d2 As DateTime = New DateTime(2014, 10, 6)
If d = d2 Then
Console.WriteLine("Equal dates")
End If
End Sub
End Module
Output
Equal dates
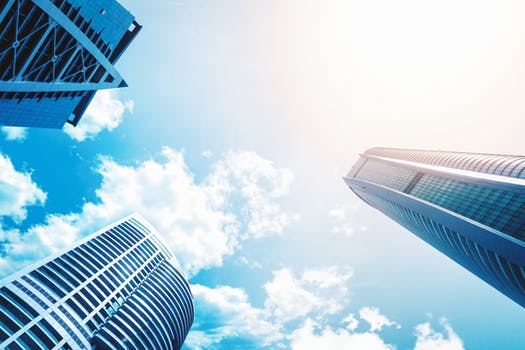
DateTime.MinValue. Suppose we have a DateTime that we are not ready to assign to an actual value. We can use a special constant like MinValue to mean the default, zero value.
Also: We can use a nullable DateTime to mean "no value is set." A nullable may be clearer for this use in programs.
VB.NET program that uses DateTime.MinValue
Module Module1
Sub Main()
Dim value As DateTime = DateTime.MinValue
Console.WriteLine("MINVALUE: {0}", value)
' Test for MinValue.
If value = DateTime.MinValue Then
Console.WriteLine("MIN VALUE IS SET")
End If
End Sub
End Module
Output
MINVALUE: 1/1/0001 12:00:00 AM
MIN VALUE IS SET
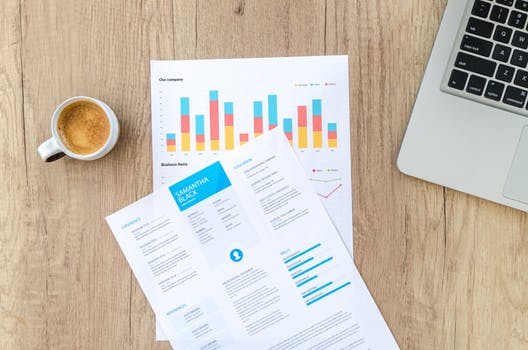
Error, un-representable. We cannot create a DateTime that cannot possibly exist. Here we try to use a month of 20 in the constructor, but get an "un-representable" error.
ExceptionVB.NET program that shows parameter error
Module Module1
Sub Main()
' Cannot have a month of 20, no such month exists.
Dim value As DateTime = New DateTime(2000, 20, 1)
End Sub
End Module
Output
Unhandled Exception: System.ArgumentOutOfRangeException:
Year, Month, and Day parameters describe an un-representable DateTime.
at System.DateTime.DateToTicks(Int32 year, Int32 month, Int32 day)
at System.DateTime..ctor(Int32 year, Int32 month, Int32 day)...
Nothing. We cannot assign a DateTime to Nothing—we must use a special value like MinValue to mean "no date." But we can also use a Nullable DateTime.
Info: A Nullable type is a structure that wraps a value type like the DateTime. We can set a nullable DateTime to Nothing.
NullableIsNothing: In this program we set our nullable DateTime to nothing. And the IsNothing function recognizes our value is nothing.
NothingVB.NET program that uses Nothing, DateTime
Module Module1
Sub Main()
' Use nullable DateTime.
Dim test As DateTime? = New DateTime(2000, 1, 1)
Console.WriteLine("GETVALUEORDEFAULT: {0}", test.GetValueOrDefault())
' The nullable DateTime can be Nothing.
test = Nothing
Console.WriteLine("ISNOTHING: {0}", IsNothing(test))
End Sub
End Module
Output
GETVALUEORDEFAULT: 1/1/2000 12:00:00 AM
ISNOTHING: True
Now property. This property is the same as Today, but it fills in the time. So it represents both the current time and the current date—Today just represents the current date.
DateTime.Now
Parse. DateTime.Parse converts a string to a DateTime. If we read in string data from user input or a database, we can parse it into a DateTime for further processing.
DateTime.Parse
Timing. Timers are excellent for checking up on your program with a diagnostic method. They can execute any code. We can, for example, run a Timer every 30 seconds.
TimerStopwatchBenchmarksTimeZone. Day light changes, and time zones, can be complex. With the TimeZone.CurrentTimeZone property we access information that can help some programs.
TimeZoneA helpful type. We used the DateTime type in the VB.NET language in some specific, useful ways. The examples provide an overall glimpse into the DateTime type but not a comprehensive view.
Related Links:
- VB.NET Nullable
- VB.NET Convert Char Array to String
- VB.NET Object Array
- VB.NET File.ReadAllText, Get String From File
- VB.NET Compress File: GZipStream Example
- VB.NET Console.WriteLine (Print)
- VB.NET File.ReadLines Example
- VB.NET AddressOf Operator
- VB.NET Recursion Example
- VB.NET Recursive File Directory Function
- VB.NET Regex, Read and Match File Lines
- VB.NET Regex.Matches Quote Example
- VB.NET Regex.Matches: For Each Match, Capture
- VB.NET Convert String, Byte Array
- VB.NET File Size: FileInfo Example
- VB.NET File Handling
- VB.NET String.Format Examples: String and Integer
- VB.NET SyncLock Statement
- VB.NET TextInfo Examples
- VB.NET Array.Copy Example
- VB.NET HtmlEncode, HtmlDecode Examples
- VB.NET HtmlTextWriter Example
- VB.NET Stack Type
- VB.NET Func, Action and Predicate Examples
- VB.NET Function Examples
- VB.NET GoTo Example: Labels, Nested Loops
- VB.NET Array.Find Function, FindAll
- VB.NET HttpClient Example: System.Net.Http
- VB.NET DataColumn Class
- VB.NET DataGridView
- VB.NET DataSet Examples
- VB.NET DataTable Select Function
- VB.NET DataTable Examples
- VB.NET Attribute Examples
- VB.NET OpenFileDialog Example
- VB.NET Benchmark
- VB.NET BinaryReader Example
- VB.NET BinarySearch List
- VB.NET BinaryWriter Example
- VB.NET Regex.Replace Function
- VB.NET Regex.Split Examples
- VB.NET Regex.Match Examples: Regular Expressions
- VB.NET Convert ArrayList to Array
- VB.NET Array Examples, String Arrays
- VB.NET ArrayList Examples
- VB.NET Boolean, True, False and Not (Return True)
- VB.NET Nothing, IsNothing (Null)
- VB.NET Directive Examples: Const, If and Region
- VB.NET Do Until Loops
- VB.NET Do While Loop Examples (While)
- VB.NET Array.Resize Subroutine
- VB.NET Chr Function: Get Char From Integer
- VB.NET Class Examples
- VB.NET IndexOf Function
- VB.NET Insert String
- VB.NET Interface Examples (Implements)
- VB.NET 2D, 3D and Jagged Array Examples
- VB.NET Enum.Parse, TryParse: Convert String to Enum
- VB.NET Remove HTML Tags
- VB.NET Remove String
- VB.NET Event Example: AddHandler, RaiseEvent
- VB.NET Excel Interop Example
- VB.NET StartsWith and EndsWith String Functions
- VB.NET Initialize List
- VB.NET Number Examples
- VB.NET Optional String, Integer: Named Arguments
- VB.NET Replace String Examples
- VB.NET Exception Handling: Try, Catch and Finally
- VB.NET Enum Examples
- VB.NET Enumerable.Range, Repeat and Empty
- VB.NET Dictionary Examples
- VB.NET Double Type
- VB.NET LSet and RSet Functions
- VB.NET LTrim and RTrim Functions
- VB.NET Alphanumeric Sorting
- VB.NET PadLeft and PadRight
- VB.NET String.Concat Examples
- VB.NET String
- VB.NET Math.Abs: Absolute Value
- VB.NET Array.IndexOf, LastIndexOf
- VB.NET Remove Duplicate Chars
- VB.NET If Then, ElseIf, Else Examples
- VB.NET ParamArray (Use varargs Functions)
- VB.NET Integer.Parse: Convert String to Integer
- VB.NET ThreadPool
- VB.NET Process Examples (Process.Start)
- VB.NET TimeZone Example
- VB.NET Path Examples
- VB.NET ToArray Extension Example
- VB.NET ToCharArray Function
- VB.NET Stopwatch Example
- VB.NET Button Example
- VB.NET StreamReader ReadToEnd Function
- VB.NET ByVal Versus ByRef Example
- VB.NET StreamReader Example
- VB.NET StreamWriter Example
- VB.NET String.Compare Examples
- VB.NET Cast: TryCast, DirectCast Examples
- VB.NET String Constructor (New String)
- VB.NET String.Copy and CopyTo
- VB.NET Math.Ceiling and Floor: Double Examples
- VB.NET Math.Max and Math.Min
- VB.NET WebClient: DownloadData, Headers
- VB.NET Math.Round Example
- VB.NET Math.Truncate Method, Cast Double to Integer
- VB.NET Reverse String
- VB.NET Structure Examples
- VB.NET Sub Examples
- VB.NET Substring Examples
- VB.NET Convert Dictionary to List
- VB.NET Convert List and Array
- VB.NET Convert List to String
- VB.NET Convert Miles to Kilometers
- VB.NET Property Examples (Get, Set)
- VB.NET Remove Punctuation From String
- VB.NET Queue Examples
- VB.NET Const Values
- VB.NET Remove Duplicates From List
- VB.NET IComparable Example
- VB.NET ReDim Keyword (Array.Resize)
- VB.NET Contains Example
- VB.NET IEnumerable Examples
- VB.NET IsNot and Is Operators
- VB.NET String.IsNullOrEmpty, IsNullOrWhiteSpace
- VB.NET ROT13 Encode Function
- VB.NET StringBuilder Examples
- VB.NET Image Type
- VB.NET Val, Asc and AscW Functions
- VB.NET String.Empty Example
- VB.NET String.Equals Function
- VB.NET VarType Function (VariantType Enum)
- VB.NET With Statement
- VB.NET WithEvents: Handles and RaiseEvent
- VB.NET String Length Example
- VB.NET ToList Extension Example
- VB.NET ToLower and ToUpper Examples
- VB.NET TextBox Example
- VB.NET ToString Overrides Example
- VB.NET ToTitleCase Function
- VB.NET Convert String Array to String
- VB.NET Iterator Example: Yield Keyword
- VB.NET Mid Statement
- VB.NET Mod Operator (Odd, Even Numbers)
- VB.NET Convert String to Integer
- VB.NET Module Example: Shared Data
- VB.NET Integer
- VB.NET Keywords
- VB.NET Lambda Expressions
- VB.NET LastIndexOf Function
- VB.NET String Join Examples
- VB.NET Multiple Return Values
- VB.NET MustInherit Class: Shadows and Overloads
- VB.NET Namespace Example
- VB.NET KeyValuePair Examples
- VB.NET Environment.NewLine: vbCrLf
- VB.NET Levenshtein Distance Algorithm
- VB.NET Shared Function
- VB.NET Shell Function: Start EXE Program
- VB.NET Sleep Subroutine (Pause)
- VB.NET Sort Dictionary
- VB.NET Exit Statements
- VB.NET LINQ Examples
- VB.NET List Examples
- VB.NET Extension Method
- VB.NET Select Case Examples
- VB.NET MessageBox.Show Examples
- VB.NET Timer Examples
- VB.NET TimeSpan Examples
- VB.NET BackgroundWorker
- VB.NET String Between, Before and After Functions
- VB.NET CStr Function
- VB.NET DataRow Field Extension
- VB.NET DataRow Examples
- VB.NET DateTime Format
- VB.NET Char Examples
- VB.NET DateTime.Now Property (Today)
- VB.NET DateTime.Parse: Convert String to DateTime
- VB.NET DateTime Examples
- VB.NET Decimal Type
- VB.NET HashSet Example
- VB.NET Hashtable Type
- VB.NET Fibonacci Sequence
- VB.NET XmlWriter, Create XML File
- VB.NET File.Copy: Examples, Overwrite
- VB.NET File.Exists: Get Boolean
- VB.NET Path.GetExtension: File Extension
- VB.NET Tuple Examples
- VB.NET Trim Function
- VB.NET TrimEnd and TrimStart Examples
- VB.NET Word Count Function
- VB.NET Word Interop Example
- VB.NET For Loop Examples (For Each)
- VB.NET XElement Example
- VB.NET Truncate String
- VB.NET Sort Number Strings
- VB.NET Sort Examples: Arrays and Lists
- VB.NET SortedList
- VB.NET SortedSet Examples
- VB.NET Split String Examples
- VB.NET Uppercase First Letter
- VB.NET XmlReader, Parse XML File
- VB.NET ZipFile Example
- VB.NET Array.Reverse Example
- VB.NET Random Lowercase Letter
- VB.NET Byte Array: Memory Usage
- VB.NET Byte and Sbyte Types
- VB.NET Char Array
- VB.NET Random String
- VB.NET Random Numbers
- VB.NET Async, Await Example: Task Start and Wait
- VB.NET Choose Function (Get Argument at Index)
- VB.NET Sort by File Size
- VB.NET Sort List (Lambda That Calls CompareTo)
- VB.NET List Find and Exists Examples
- VB.NET Math.Sqrt Function
- VB.NET Loop Over String: For, For Each
Related Links
Adjectives
Ado
Ai
Android
Angular
Antonyms
Apache
Articles
Asp
Autocad
Automata
Aws
Azure
Basic
Binary
Bitcoin
Blockchain
C
Cassandra
Change
Coa
Computer
Control
Cpp
Create
Creating
C-Sharp
Cyber
Daa
Data
Dbms
Deletion
Devops
Difference
Discrete
Es6
Ethical
Examples
Features
Firebase
Flutter
Fs
Git
Go
Hbase
History
Hive
Hiveql
How
Html
Idioms
Insertion
Installing
Ios
Java
Joomla
Js
Kafka
Kali
Laravel
Logical
Machine
Matlab
Matrix
Mongodb
Mysql
One
Opencv
Oracle
Ordering
Os
Pandas
Php
Pig
Pl
Postgresql
Powershell
Prepositions
Program
Python
React
Ruby
Scala
Selecting
Selenium
Sentence
Seo
Sharepoint
Software
Spellings
Spotting
Spring
Sql
Sqlite
Sqoop
Svn
Swift
Synonyms
Talend
Testng
Types
Uml
Unity
Vbnet
Verbal
Webdriver
What
Wpf