<< Back to VBNET
VB.NET StringBuilder Examples
Improve performance when appending Strings with the StringBuilder class. Replace and insert data.StringBuilder. This is an optimization. A class, StringBuilder improves common String operations. Appending, replacing and inserting are faster. It is easy to use.
With ToString, we can convert our data back into a String. This operation is optimized to avoid copies. With StringBuilder we have many built-in optimizations.
Strings
First example. We see a program that declares a new StringBuilder. We append Strings and newlines to it. Finally the StringBuilder is converted to a String and written to the Console.
Imports: The first directive "Imports System.Text" lets us reference StringBuilder more directly in the program.
ToString: With this method, we convert the internal data of a StringBuilder into an actual String object.
VB.NET program that uses StringBuilder
Imports System.Text
Module Module1
Sub Main()
' Declare new StringBuilder Dim.
Dim builder As New StringBuilder
' Append a string to the StringBuilder.
builder.Append("Here is the list:")
' Append a line break.
builder.AppendLine()
' Append a string and then another line break.
builder.Append("1 dog").AppendLine()
' Get internal String value from StringBuilder.
Dim s As String = builder.ToString
' Write output.
Console.WriteLine(s)
Console.ReadLine()
End Sub
End Module
Output
Here is the list:
1 dog
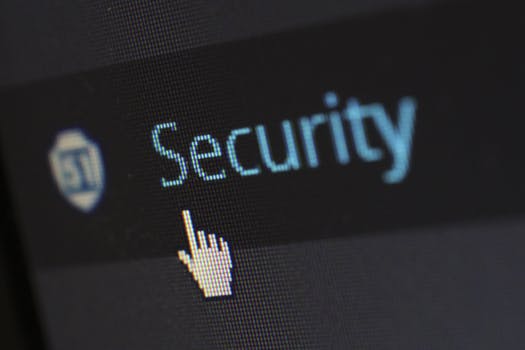
Replace. This example creates a StringBuilder with the constructor. Then it calls Replace() to replace one substring with another. Performance is good. No string temporaries are created.
Here: The starting buffer for the StringBuilder has the word "the" in it. After the Replace method is run, it instead has the word "my."
Return: Replace() returns a reference to the StringBuilder. We can ignore this return value, or call another method on it.
VB.NET program that uses Replace
Imports System.Text
Module Module1
Sub Main()
Dim builder As New StringBuilder("Initialize the StringBuilder.")
builder.Replace("the", "my")
Console.WriteLine(builder.ToString)
Console.ReadLine()
End Sub
End Module
Output
Initialize my StringBuilder.
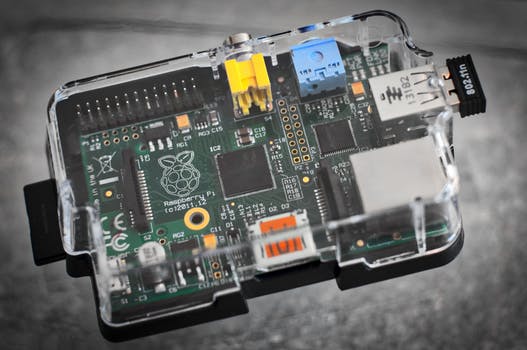
Replace, all instances. The Replace() Function does not just replace the first instance of a string it finds. It changes all of them, no matter how many there are.
VB.NET program that uses Replace, multiple instances
Imports System.Text
Module Module1
Sub Main()
Dim builder As New StringBuilder("I see a cat, and the cat sees me.")
' Replace all instances of substring.
builder.Replace("cat", "dog")
Console.WriteLine("RESULT: {0}", builder)
End Sub
End Module
Output
RESULT: I see a dog, and the dog sees me.
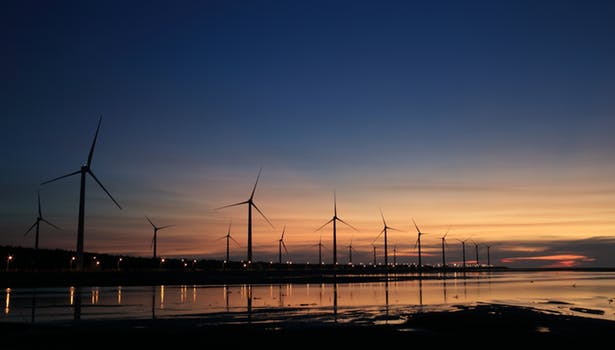
For-each. Here we use StringBuilder in a For-Each loop. Whenever we see Strings being appended in a loop, consider StringBuilder. It can improve runtime performance.
Here: A String array is declared. It contains 3 strings. A new StringBuilder is instantiated with a certain value.
Loop: In the For-Each loop, the Dim String is assigned to each String in the String array.
For Each, ForFinally: The StringBuilder has each string (and a newline) appended to it. After the loop, the results are printed to the Console.
ConsoleVB.NET program that uses loop with StringBuilder
Imports System.Text
Module Module1
Sub Main()
' String array for use in loop.
Dim items As String() = New String() {"Indus", "Danube", "Nile"}
' Initialize new StringBuilder.
Dim builder As StringBuilder =
New StringBuilder("These rivers are cool:").AppendLine
' Loop over each item in Array.
Dim item As String
For Each item In items
builder.Append(item).AppendLine()
Next
' Write result.
Console.WriteLine(builder.ToString)
Console.ReadLine()
End Sub
End Module
Output
These rivers are cool:
Indus
Danube
Nile
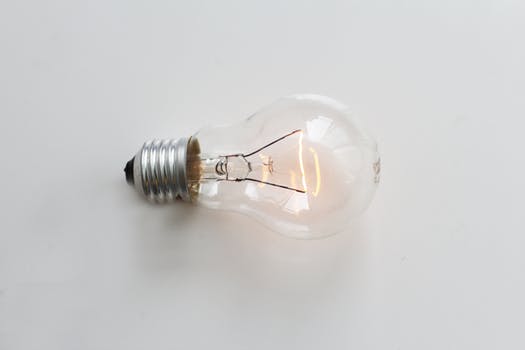
Insert. This modifies a StringBuilder at the specified index. Here I specify the index 1, so the String literal is inserted after the first character in the buffer.
VB.NET program that uses Insert
Imports System.Text
Module Module1
Sub Main()
Dim builder As StringBuilder = New StringBuilder("A cat")
Console.WriteLine(builder)
' Insert this string at index 1.
builder.Insert(1, " fluffy")
Console.WriteLine(builder)
End Sub
End Module
Output
A cat
A fluffy cat
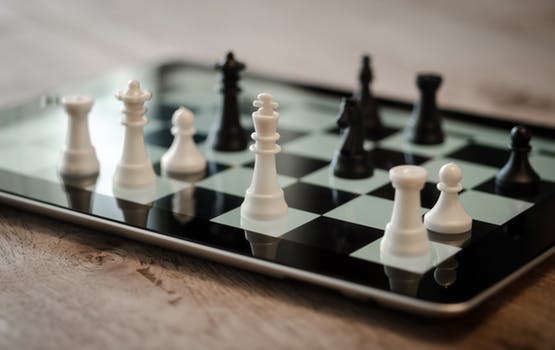
Remove. This erases characters from a StringBuilder and collapse those following it. In this example, I remove four chars starting at index 1.
Result: The final data is missing those four chars, but contains the surrounding ones.
VB.NET program that uses Remove
Imports System.Text
Module Module1
Sub Main()
Dim builder As StringBuilder = New StringBuilder("A big dog")
Console.WriteLine(builder)
' Remove character starting at index 1.
' ... Remove 4 characters.
builder.Remove(1, 4)
Console.WriteLine(builder)
End Sub
End Module
Output
A big dog
A dog
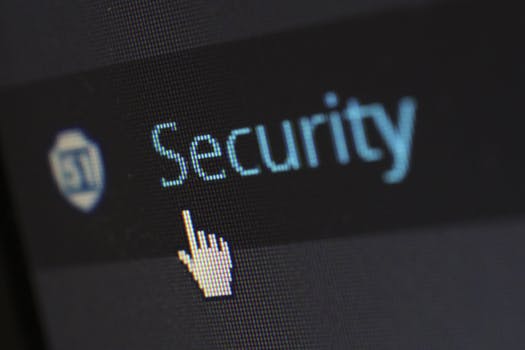
AppendFormat. Let us use the AppendFormat Function. AppendFormat receives a format string, usually with substitutions such as {0}, and parameters to fill those substitutions.
First: The Main entry point declares a StringBuilder. And then each Integer is looped over with For-Each.
Finally: Each integer is appended. Each integer is formatted to have one decimal place.
IntegerTip: Using format strings, as with AppendFormat, often yields code that is easier to read and understand.
VB.NET program that uses AppendFormat
Imports System.Text
Module Module1
''' <summary>
''' Integer array for example
''' </summary>
Private m_values As Integer() = {5, 10, 15, 20}
Sub Main()
' StringBuilder declaration.
Dim b As New StringBuilder
' Declare new loop variable.
Dim v As Integer
For Each v In m_values
' Display with AppendFormat.
b.AppendFormat(("Value: {0:0.0}" & Environment.NewLine), v)
Next
Console.WriteLine(b.ToString)
End Sub
End Module
Output
Value: 5.0
Value: 10.0
Value: 15.0
Value: 20.0
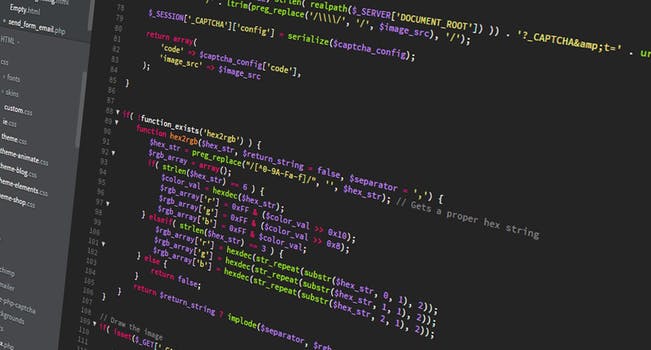
Benchmark. In appending, StringBuilder is faster than String. Consider this benchmark: we want to append 3-character string (abc). We compare StringBuilder and String.
Version 1: This version of the code uses StringBuilder to append a string 100 times.
Version 2: Here we use String and its concatenation operator to append a string 100 times. This creates 100 strings.
Result: When 2 strings are concatenated, a new String object results. Reducing allocations (with StringBuilder) improves performance.
VB.NET program that times StringBuilder, string appends
Imports System.Text
Module Module1
Sub Main()
Dim m As Integer = 100000
' Version 1: use StringBuilder.
Dim s1 As Stopwatch = Stopwatch.StartNew
For i As Integer = 0 To m - 1
Dim builder As StringBuilder = New StringBuilder
For x As Integer = 0 To 99
builder.Append("abc")
Next
Next
s1.Stop()
' Version 2: use String.
Dim s2 As Stopwatch = Stopwatch.StartNew
For i As Integer = 0 To m - 1
Dim str As String = ""
For x As Integer = 0 To 99
str += "abc"
Next
Next
s2.Stop()
Dim u As Integer = 1000000
Console.WriteLine( s1.Elapsed.TotalMilliseconds.ToString("0.00 ms"))
Console.WriteLine( s2.Elapsed.TotalMilliseconds.ToString("0.00 ms"))
End Sub
End Module
Output
156.04 ms, StringBuilder Append
1020.00 ms, String concat
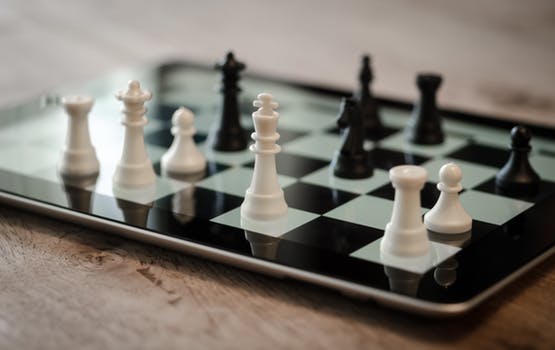
Performance advice. My research shows that appending two or three strings in a loop is faster than using StringBuilder. But using StringBuilder is faster for more than four iterations.
However: It is best to almost always use StringBuilder in loops. It can avert a performance disaster in edge cases.
StringBuilder Performance
Methods. These are my notes on methods you can use with StringBuilder. The documentation here is universal for all .NET languages. We can reference Microsoft Docs for all new types.
Append: Microsoft states that this "appends the string representation of a specified object to the end of this instance."
StringBuilder: Microsoft DocsEnsureCapacity: This rarely is useful for changing the capacity, which is an optimization you can use.
Insert: This is similar to Replace. But we use it to add characters at a specific index.
Remove: This has the same effect as the Remove method on String, but it avoids character buffer copies.
Replace: This exchanges one substring in a StringBuilder with another. All instances (not just one) are changed.
Immutable. This term means that the data pointed at cannot be changed from our code. String is immutable. You cannot assign chars to its contents. But StringBuilder uses mutable buffers.
Important: In programs, we can improve performance by reducing allocations and objects.
So: With StringBuilder, we reuse one buffer to build up a collection of characters. With String, many objects are instead created.
Caution: We can add lots of data to StringBuilder. But we will get an ArgumentOutOfRange exception if we put more data than will fit.
HtmlTextWriter. HTML syntax uses lots of quotes and brackets. We can write HTML markup directly, without dealing with syntax: check out HtmlTextWriter.
HtmlTextWriterNote: Types such as HtmlTextWriter tend to reduce performance but improve code readability.
As developers, we will require StringBuilder in many applications. It is an excellent performance optimization. It can make unusable programs fast.
Often, we use StringBuilder in For-Each loops. For repeated appends, StringBuilder is ideal. Concatenating many strings is a sign that StringBuilder is needed.
Related Links:
- VB.NET Nullable
- VB.NET Convert Char Array to String
- VB.NET Object Array
- VB.NET File.ReadAllText, Get String From File
- VB.NET Compress File: GZipStream Example
- VB.NET Console.WriteLine (Print)
- VB.NET File.ReadLines Example
- VB.NET AddressOf Operator
- VB.NET Recursion Example
- VB.NET Recursive File Directory Function
- VB.NET Regex, Read and Match File Lines
- VB.NET Regex.Matches Quote Example
- VB.NET Regex.Matches: For Each Match, Capture
- VB.NET Convert String, Byte Array
- VB.NET File Size: FileInfo Example
- VB.NET File Handling
- VB.NET String.Format Examples: String and Integer
- VB.NET SyncLock Statement
- VB.NET TextInfo Examples
- VB.NET Array.Copy Example
- VB.NET HtmlEncode, HtmlDecode Examples
- VB.NET HtmlTextWriter Example
- VB.NET Stack Type
- VB.NET Func, Action and Predicate Examples
- VB.NET Function Examples
- VB.NET GoTo Example: Labels, Nested Loops
- VB.NET Array.Find Function, FindAll
- VB.NET HttpClient Example: System.Net.Http
- VB.NET DataColumn Class
- VB.NET DataGridView
- VB.NET DataSet Examples
- VB.NET DataTable Select Function
- VB.NET DataTable Examples
- VB.NET Attribute Examples
- VB.NET OpenFileDialog Example
- VB.NET Benchmark
- VB.NET BinaryReader Example
- VB.NET BinarySearch List
- VB.NET BinaryWriter Example
- VB.NET Regex.Replace Function
- VB.NET Regex.Split Examples
- VB.NET Regex.Match Examples: Regular Expressions
- VB.NET Convert ArrayList to Array
- VB.NET Array Examples, String Arrays
- VB.NET ArrayList Examples
- VB.NET Boolean, True, False and Not (Return True)
- VB.NET Nothing, IsNothing (Null)
- VB.NET Directive Examples: Const, If and Region
- VB.NET Do Until Loops
- VB.NET Do While Loop Examples (While)
- VB.NET Array.Resize Subroutine
- VB.NET Chr Function: Get Char From Integer
- VB.NET Class Examples
- VB.NET IndexOf Function
- VB.NET Insert String
- VB.NET Interface Examples (Implements)
- VB.NET 2D, 3D and Jagged Array Examples
- VB.NET Enum.Parse, TryParse: Convert String to Enum
- VB.NET Remove HTML Tags
- VB.NET Remove String
- VB.NET Event Example: AddHandler, RaiseEvent
- VB.NET Excel Interop Example
- VB.NET StartsWith and EndsWith String Functions
- VB.NET Initialize List
- VB.NET Number Examples
- VB.NET Optional String, Integer: Named Arguments
- VB.NET Replace String Examples
- VB.NET Exception Handling: Try, Catch and Finally
- VB.NET Enum Examples
- VB.NET Enumerable.Range, Repeat and Empty
- VB.NET Dictionary Examples
- VB.NET Double Type
- VB.NET LSet and RSet Functions
- VB.NET LTrim and RTrim Functions
- VB.NET Alphanumeric Sorting
- VB.NET PadLeft and PadRight
- VB.NET String.Concat Examples
- VB.NET String
- VB.NET Math.Abs: Absolute Value
- VB.NET Array.IndexOf, LastIndexOf
- VB.NET Remove Duplicate Chars
- VB.NET If Then, ElseIf, Else Examples
- VB.NET ParamArray (Use varargs Functions)
- VB.NET Integer.Parse: Convert String to Integer
- VB.NET ThreadPool
- VB.NET Process Examples (Process.Start)
- VB.NET TimeZone Example
- VB.NET Path Examples
- VB.NET ToArray Extension Example
- VB.NET ToCharArray Function
- VB.NET Stopwatch Example
- VB.NET Button Example
- VB.NET StreamReader ReadToEnd Function
- VB.NET ByVal Versus ByRef Example
- VB.NET StreamReader Example
- VB.NET StreamWriter Example
- VB.NET String.Compare Examples
- VB.NET Cast: TryCast, DirectCast Examples
- VB.NET String Constructor (New String)
- VB.NET String.Copy and CopyTo
- VB.NET Math.Ceiling and Floor: Double Examples
- VB.NET Math.Max and Math.Min
- VB.NET WebClient: DownloadData, Headers
- VB.NET Math.Round Example
- VB.NET Math.Truncate Method, Cast Double to Integer
- VB.NET Reverse String
- VB.NET Structure Examples
- VB.NET Sub Examples
- VB.NET Substring Examples
- VB.NET Convert Dictionary to List
- VB.NET Convert List and Array
- VB.NET Convert List to String
- VB.NET Convert Miles to Kilometers
- VB.NET Property Examples (Get, Set)
- VB.NET Remove Punctuation From String
- VB.NET Queue Examples
- VB.NET Const Values
- VB.NET Remove Duplicates From List
- VB.NET IComparable Example
- VB.NET ReDim Keyword (Array.Resize)
- VB.NET Contains Example
- VB.NET IEnumerable Examples
- VB.NET IsNot and Is Operators
- VB.NET String.IsNullOrEmpty, IsNullOrWhiteSpace
- VB.NET ROT13 Encode Function
- VB.NET StringBuilder Examples
- VB.NET Image Type
- VB.NET Val, Asc and AscW Functions
- VB.NET String.Empty Example
- VB.NET String.Equals Function
- VB.NET VarType Function (VariantType Enum)
- VB.NET With Statement
- VB.NET WithEvents: Handles and RaiseEvent
- VB.NET String Length Example
- VB.NET ToList Extension Example
- VB.NET ToLower and ToUpper Examples
- VB.NET TextBox Example
- VB.NET ToString Overrides Example
- VB.NET ToTitleCase Function
- VB.NET Convert String Array to String
- VB.NET Iterator Example: Yield Keyword
- VB.NET Mid Statement
- VB.NET Mod Operator (Odd, Even Numbers)
- VB.NET Convert String to Integer
- VB.NET Module Example: Shared Data
- VB.NET Integer
- VB.NET Keywords
- VB.NET Lambda Expressions
- VB.NET LastIndexOf Function
- VB.NET String Join Examples
- VB.NET Multiple Return Values
- VB.NET MustInherit Class: Shadows and Overloads
- VB.NET Namespace Example
- VB.NET KeyValuePair Examples
- VB.NET Environment.NewLine: vbCrLf
- VB.NET Levenshtein Distance Algorithm
- VB.NET Shared Function
- VB.NET Shell Function: Start EXE Program
- VB.NET Sleep Subroutine (Pause)
- VB.NET Sort Dictionary
- VB.NET Exit Statements
- VB.NET LINQ Examples
- VB.NET List Examples
- VB.NET Extension Method
- VB.NET Select Case Examples
- VB.NET MessageBox.Show Examples
- VB.NET Timer Examples
- VB.NET TimeSpan Examples
- VB.NET BackgroundWorker
- VB.NET String Between, Before and After Functions
- VB.NET CStr Function
- VB.NET DataRow Field Extension
- VB.NET DataRow Examples
- VB.NET DateTime Format
- VB.NET Char Examples
- VB.NET DateTime.Now Property (Today)
- VB.NET DateTime.Parse: Convert String to DateTime
- VB.NET DateTime Examples
- VB.NET Decimal Type
- VB.NET HashSet Example
- VB.NET Hashtable Type
- VB.NET Fibonacci Sequence
- VB.NET XmlWriter, Create XML File
- VB.NET File.Copy: Examples, Overwrite
- VB.NET File.Exists: Get Boolean
- VB.NET Path.GetExtension: File Extension
- VB.NET Tuple Examples
- VB.NET Trim Function
- VB.NET TrimEnd and TrimStart Examples
- VB.NET Word Count Function
- VB.NET Word Interop Example
- VB.NET For Loop Examples (For Each)
- VB.NET XElement Example
- VB.NET Truncate String
- VB.NET Sort Number Strings
- VB.NET Sort Examples: Arrays and Lists
- VB.NET SortedList
- VB.NET SortedSet Examples
- VB.NET Split String Examples
- VB.NET Uppercase First Letter
- VB.NET XmlReader, Parse XML File
- VB.NET ZipFile Example
- VB.NET Array.Reverse Example
- VB.NET Random Lowercase Letter
- VB.NET Byte Array: Memory Usage
- VB.NET Byte and Sbyte Types
- VB.NET Char Array
- VB.NET Random String
- VB.NET Random Numbers
- VB.NET Async, Await Example: Task Start and Wait
- VB.NET Choose Function (Get Argument at Index)
- VB.NET Sort by File Size
- VB.NET Sort List (Lambda That Calls CompareTo)
- VB.NET List Find and Exists Examples
- VB.NET Math.Sqrt Function
- VB.NET Loop Over String: For, For Each
Related Links
Adjectives
Ado
Ai
Android
Angular
Antonyms
Apache
Articles
Asp
Autocad
Automata
Aws
Azure
Basic
Binary
Bitcoin
Blockchain
C
Cassandra
Change
Coa
Computer
Control
Cpp
Create
Creating
C-Sharp
Cyber
Daa
Data
Dbms
Deletion
Devops
Difference
Discrete
Es6
Ethical
Examples
Features
Firebase
Flutter
Fs
Git
Go
Hbase
History
Hive
Hiveql
How
Html
Idioms
Insertion
Installing
Ios
Java
Joomla
Js
Kafka
Kali
Laravel
Logical
Machine
Matlab
Matrix
Mongodb
Mysql
One
Opencv
Oracle
Ordering
Os
Pandas
Php
Pig
Pl
Postgresql
Powershell
Prepositions
Program
Python
React
Ruby
Scala
Selecting
Selenium
Sentence
Seo
Sharepoint
Software
Spellings
Spotting
Spring
Sql
Sqlite
Sqoop
Svn
Swift
Synonyms
Talend
Testng
Types
Uml
Unity
Vbnet
Verbal
Webdriver
What
Wpf