<< Back to VBNET
VB.NET Enum Examples
Use Enum values to stores named and magic constants. Use if-statements with enums.Enum. An Enum type stores special values. These are named constants. With an Enum, we replace magic constants throughout a program.
Enum benefits. Enums make code clearer: they organize code and make programs easier to maintain. We use the Enum keyword, and place enums in Select Case and If-statements.
Example. Here we use an Enum named Importance. The Enum represents a constant value. It indicates a value's priority. The names of the constants in an Enum can be any valid identifiers.
Select: In the Main method, we use a Select statement on an enum variable. This enables a fast match for the correct Enum value.
Select CaseResult: The case for Importance.Critical is selected. The output of the program is "True."
VB.NET program that uses Enum with Select
Module Module1
''' <summary>
''' Levels of importance.
''' </summary>
Enum Importance
None = 0
Trivial = 1
Regular = 2
Important = 3
Critical = 4
End Enum
Sub Main()
Dim value As Importance = Importance.Critical
' Select the enum and print a value.
Select Case value
Case Importance.Trivial
Console.WriteLine("Not true")
Return
Case Importance.Critical
Console.WriteLine("True")
Exit Select
End Select
End Sub
End Module
Output
True
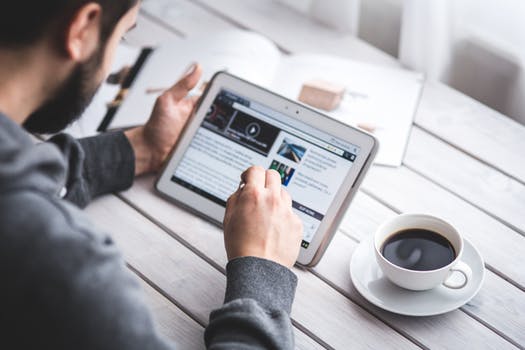
If, ElseIf. Often we use the If and ElseIf constructs to implement checks for certain Enum values. With conditionals, we check an Enum Dim variable against named constants in an Enum type.
If ThenHere: We represent a markup tag, such as HTML, in a conceptual model as an Enum value. We test the tags with the If and ElseIf statements.
VB.NET program that uses enum If and ElseIf
Module Module1
''' <summary>
''' Represents tag type.
''' </summary>
Enum TagType
None = 0
BoldTag = 1
ItalicsTag = 2
HyperlinkTag = 3
End Enum
Sub Main()
' Create enum type variable.
Dim value As TagType = TagType.HyperlinkTag
' Use enum in If-statement.
' ... Also use ElseIf statement.
If value = TagType.BoldTag Then
Console.WriteLine("Bold")
ElseIf value = TagType.HyperlinkTag Then
Console.WriteLine("Not true")
End If
End Sub
End Module
Output
Not true
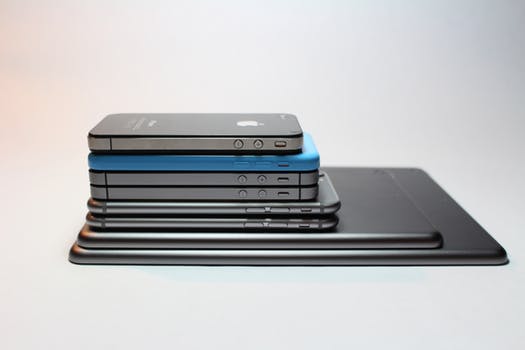
ToString. Suppose we want a string representation. We need to invoke ToString explicitly on an Enum variable when we pass it to the Console.Write or WriteLine subs.
Important: If we do not call ToString, we will receive the numeric value of the enum, not the string representation.
Here: In this example, we show the ToString method on an Enum variable called AnimalType.
Animal: The Enum represents a type of animal, which contains options for cats and dogs. We finally call Console.WriteLine.
ConsoleVB.NET program that uses ToString, Enum
Module Module1
''' <summary>
''' Type of animal.
''' </summary>
Enum AnimalType
None = 0
Cat = 1
Dog = 2
End Enum
''' <summary>
''' Type of visibility.
''' </summary>
Enum VisibilityType
None = 0
Hidden = 2
Visible = 4
End Enum
Sub Main()
Dim dog As AnimalType = AnimalType.Dog
Dim hidden As VisibilityType = VisibilityType.Hidden
' Write to the Console with the ToString method.
Console.WriteLine(dog.ToString)
Console.WriteLine(hidden.ToString)
End Sub
End Module
Output
Dog
Hidden
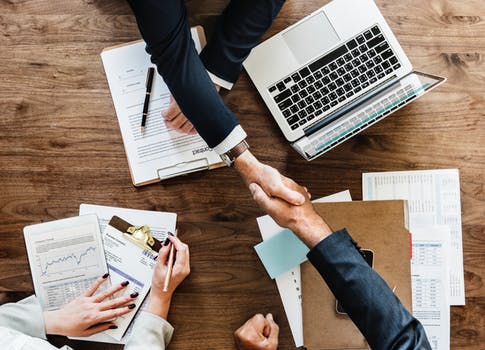
Stack. Here we use a Stack to represent a markup tree. If we have an HTML document with tags, we could use a Stack of Enum values (TagType) to represent this tree.
Note: You could use this functionality for HTML validation as well. Opening and closing tags could be matched.
Tip: To use the Stack collection, we typically use the Push, Pop as well as Peek methods.
StackVB.NET program that uses Stack, Enum
Module Module1
Enum TagType
None = 0
BoldTag = 1
ItalicsTag = 2
HyperlinkTag = 3
End Enum
Sub Main()
' Create a new Stack generic instance.
Dim stack As New Stack(Of TagType)
' Add a BoldTag to it.
stack.Push(TagType.BoldTag)
' Add an ItalicsTag to it.
stack.Push(TagType.ItalicsTag)
' Pop the top element.
' ... Then write it to the Console.
Dim popped As TagType = stack.Pop()
Console.WriteLine(popped)
Console.WriteLine(popped.ToString)
End Sub
End Module
Output
2
ItalicsTag
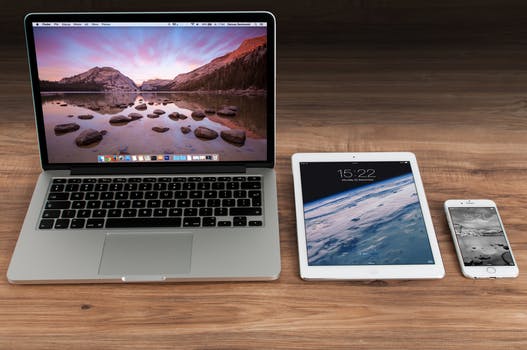
Default. An Enum has a default value. This corresponds to the value that equals 0 within the Enum type, which is by default the first listed one.
Note: The default values of an enum proceed up from 0. So the first is zero, the second is one, and the third is two.
Also: When part of a class, an Enum does not need initialization to this default value. Initializing it to zero is a performance negative.
VB.NET program that uses Enum, default value
Module Module1
Enum Level
Normal
Low
High
End Enum
Class Item
''' <summary>
''' Public enum.
''' </summary>
Public _level As Level
End Class
Sub Main()
' The default value for an enum is the one equal to 0.
Dim v As Item = New Item
Console.WriteLine(v._level.ToString())
End Sub
End Module
Output
Normal
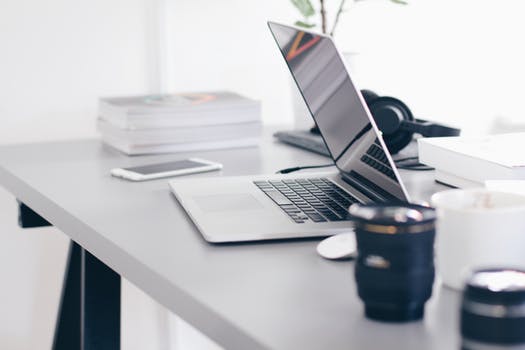
Types. Enums have a backing type—a data representation like Integer or Byte. By default, Enums are represented as Integers. But we can, with the "As" keyword change this.
Here: We create a Byte Enum with the "as Byte" keywords. Each instance of "Code" will only require one byte.
ByteTip: This ability is useful when designing a Class that is instantiated many times in memory.
Tip 2: With smaller types, we can reduce memory usage. Four Bytes are packed into the size occupied by one Integer.
IntegerVB.NET program that uses enum backing type, byte
Module Module1
Enum Code As Byte
Small = 0
Medium = 1
Large = 2
End Enum
Sub Main()
' The value is represented in a byte.
Dim value As Code = Code.Medium
Console.WriteLine(value)
End Sub
End Module
Output
1
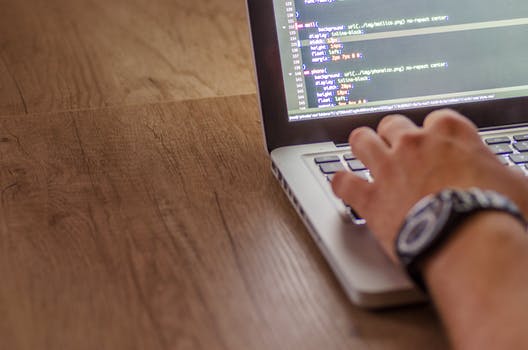
Other values. In VB.NET we can specify an enum variable has a value that is not in the enum. No warning or error is issued. So enums help with, but do not enforce, correct code.
VB.NET program that uses other values in enum
Module Module1
Enum PageColor
None = 0
Red = 1
Blue = 2
Green = 3
End Enum
Sub Main()
' This value does not exist in the enum type.
Dim value As PageColor = 4
' We can still test an enum against any value.
If value = 4 Then
Console.WriteLine("Value is 4")
End If
End Sub
End Module
Output
Value is 4
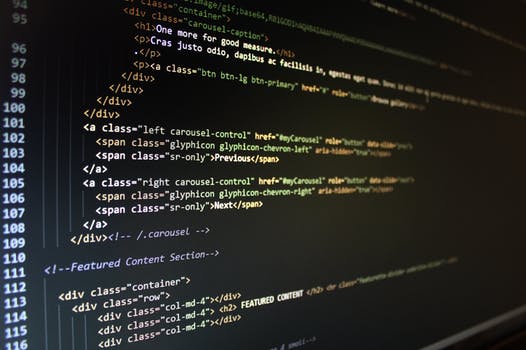
Enum argument. We can pass an enum as an argument to a Sub or Function. We specify the enum name as the type—here we use the name "Position."
VB.NET program that uses enum as argument to Sub
Module Module1
Enum Position
Above
Below
End Enum
Sub Main()
' Pass enum to function.
TestValue(Position.Below)
End Sub
Sub TestValue(ByVal value As Position)
' Test enum argument.
If value = Position.Below Then
Console.WriteLine("POSITION IS BELOW")
End If
End Sub
End Module
Output
POSITION IS BELOW
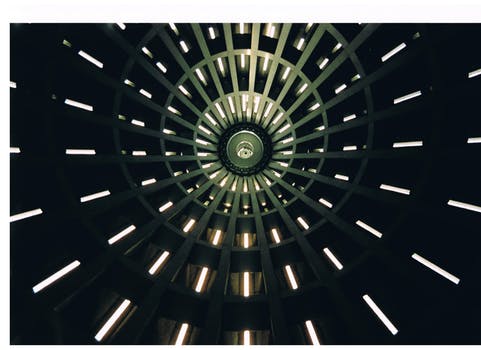
Enum Flags. We can use the Flags attribute on an Enum to enable some bitwise operations. With a Flags enum, we can use the numbers 0, 1, 2 and further to indicate values.
Bitwise Or: The VB.NET language supports Or, Xor, and And for bitwise operators. We can set the Flags value in this way.
Bitwise Operators: Microsoft DocsHasFlag: We can test for the existence of a flag with the HasFlag function. One enum value can thus have many values at once.
Attribute: We specify that an enum is a Flags enum by using an Attribute (named Flags). We surround it with angle brackets.
AttributeVB.NET program that uses Enum Flags
Module Module1
<Flags> Enum FlagData
None = 0
Cached = 1
Current = 2
Obsolete = 4
End Enum
Sub Main()
' The or operator supports bitwise operations.
' ... This is the same as 1 | 2.
Dim attr As FlagData = FlagData.Cached Or FlagData.Current
' Check for flag existence.
If attr.HasFlag(FlagData.Cached) Then
Console.WriteLine("File is cached")
End If
If attr.HasFlag(FlagData.Current) Then
Console.WriteLine("File is current")
End If
If attr.HasFlag(FlagData.Obsolete) Then
Console.WriteLine("Not reached")
End If
End Sub
End Module
Output
File is cached
File is current
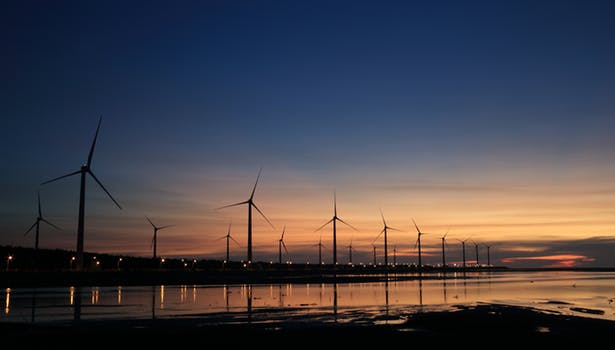
DayOfWeek, enum. Some enums are built into the .NET Framework—we can reuse them in our programs. The DayOfWeek enum stores all 7 days of the week.
So: If we wish to represent a day in a class or collection, we can use the DayOfWeek enum (it works just like any other enum).
VB.NET program that uses DayOfWeek enum
Module Module1
Sub Main()
' Add weekend day enums to list.
Dim list = New List(Of DayOfWeek)()
list.Add(DayOfWeek.Saturday)
list.Add(DayOfWeek.Sunday)
' Loop over enums in list.
For Each value As DayOfWeek In list
Console.WriteLine("WEEKEND ENUM VALUE: {0}", value)
Next
End Sub
End Module
Output
WEEKEND ENUM VALUE: Saturday
WEEKEND ENUM VALUE: Sunday
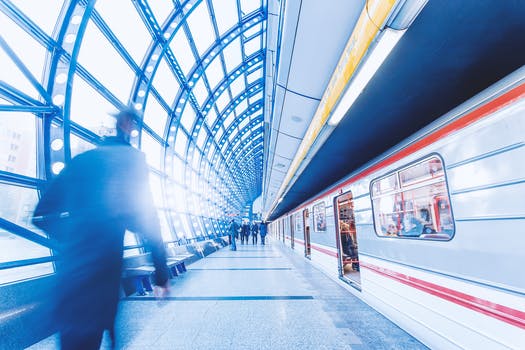
Parse. If we have a String value that contains an Enum name, how can we convert it to an Enum value? The Enum.Parse and Enum.TryParse functions are available for this purpose.
Enum.Parse, TryParse
A summary. Enums are used to represent a set of named constants. Typically, these named, symbolic constants are a selection of options for a specific variable.
Related Links:
- VB.NET Nullable
- VB.NET Convert Char Array to String
- VB.NET Object Array
- VB.NET File.ReadAllText, Get String From File
- VB.NET Compress File: GZipStream Example
- VB.NET Console.WriteLine (Print)
- VB.NET File.ReadLines Example
- VB.NET AddressOf Operator
- VB.NET Recursion Example
- VB.NET Recursive File Directory Function
- VB.NET Regex, Read and Match File Lines
- VB.NET Regex.Matches Quote Example
- VB.NET Regex.Matches: For Each Match, Capture
- VB.NET Convert String, Byte Array
- VB.NET File Size: FileInfo Example
- VB.NET File Handling
- VB.NET String.Format Examples: String and Integer
- VB.NET SyncLock Statement
- VB.NET TextInfo Examples
- VB.NET Array.Copy Example
- VB.NET HtmlEncode, HtmlDecode Examples
- VB.NET HtmlTextWriter Example
- VB.NET Stack Type
- VB.NET Func, Action and Predicate Examples
- VB.NET Function Examples
- VB.NET GoTo Example: Labels, Nested Loops
- VB.NET Array.Find Function, FindAll
- VB.NET HttpClient Example: System.Net.Http
- VB.NET DataColumn Class
- VB.NET DataGridView
- VB.NET DataSet Examples
- VB.NET DataTable Select Function
- VB.NET DataTable Examples
- VB.NET Attribute Examples
- VB.NET OpenFileDialog Example
- VB.NET Benchmark
- VB.NET BinaryReader Example
- VB.NET BinarySearch List
- VB.NET BinaryWriter Example
- VB.NET Regex.Replace Function
- VB.NET Regex.Split Examples
- VB.NET Regex.Match Examples: Regular Expressions
- VB.NET Convert ArrayList to Array
- VB.NET Array Examples, String Arrays
- VB.NET ArrayList Examples
- VB.NET Boolean, True, False and Not (Return True)
- VB.NET Nothing, IsNothing (Null)
- VB.NET Directive Examples: Const, If and Region
- VB.NET Do Until Loops
- VB.NET Do While Loop Examples (While)
- VB.NET Array.Resize Subroutine
- VB.NET Chr Function: Get Char From Integer
- VB.NET Class Examples
- VB.NET IndexOf Function
- VB.NET Insert String
- VB.NET Interface Examples (Implements)
- VB.NET 2D, 3D and Jagged Array Examples
- VB.NET Enum.Parse, TryParse: Convert String to Enum
- VB.NET Remove HTML Tags
- VB.NET Remove String
- VB.NET Event Example: AddHandler, RaiseEvent
- VB.NET Excel Interop Example
- VB.NET StartsWith and EndsWith String Functions
- VB.NET Initialize List
- VB.NET Number Examples
- VB.NET Optional String, Integer: Named Arguments
- VB.NET Replace String Examples
- VB.NET Exception Handling: Try, Catch and Finally
- VB.NET Enum Examples
- VB.NET Enumerable.Range, Repeat and Empty
- VB.NET Dictionary Examples
- VB.NET Double Type
- VB.NET LSet and RSet Functions
- VB.NET LTrim and RTrim Functions
- VB.NET Alphanumeric Sorting
- VB.NET PadLeft and PadRight
- VB.NET String.Concat Examples
- VB.NET String
- VB.NET Math.Abs: Absolute Value
- VB.NET Array.IndexOf, LastIndexOf
- VB.NET Remove Duplicate Chars
- VB.NET If Then, ElseIf, Else Examples
- VB.NET ParamArray (Use varargs Functions)
- VB.NET Integer.Parse: Convert String to Integer
- VB.NET ThreadPool
- VB.NET Process Examples (Process.Start)
- VB.NET TimeZone Example
- VB.NET Path Examples
- VB.NET ToArray Extension Example
- VB.NET ToCharArray Function
- VB.NET Stopwatch Example
- VB.NET Button Example
- VB.NET StreamReader ReadToEnd Function
- VB.NET ByVal Versus ByRef Example
- VB.NET StreamReader Example
- VB.NET StreamWriter Example
- VB.NET String.Compare Examples
- VB.NET Cast: TryCast, DirectCast Examples
- VB.NET String Constructor (New String)
- VB.NET String.Copy and CopyTo
- VB.NET Math.Ceiling and Floor: Double Examples
- VB.NET Math.Max and Math.Min
- VB.NET WebClient: DownloadData, Headers
- VB.NET Math.Round Example
- VB.NET Math.Truncate Method, Cast Double to Integer
- VB.NET Reverse String
- VB.NET Structure Examples
- VB.NET Sub Examples
- VB.NET Substring Examples
- VB.NET Convert Dictionary to List
- VB.NET Convert List and Array
- VB.NET Convert List to String
- VB.NET Convert Miles to Kilometers
- VB.NET Property Examples (Get, Set)
- VB.NET Remove Punctuation From String
- VB.NET Queue Examples
- VB.NET Const Values
- VB.NET Remove Duplicates From List
- VB.NET IComparable Example
- VB.NET ReDim Keyword (Array.Resize)
- VB.NET Contains Example
- VB.NET IEnumerable Examples
- VB.NET IsNot and Is Operators
- VB.NET String.IsNullOrEmpty, IsNullOrWhiteSpace
- VB.NET ROT13 Encode Function
- VB.NET StringBuilder Examples
- VB.NET Image Type
- VB.NET Val, Asc and AscW Functions
- VB.NET String.Empty Example
- VB.NET String.Equals Function
- VB.NET VarType Function (VariantType Enum)
- VB.NET With Statement
- VB.NET WithEvents: Handles and RaiseEvent
- VB.NET String Length Example
- VB.NET ToList Extension Example
- VB.NET ToLower and ToUpper Examples
- VB.NET TextBox Example
- VB.NET ToString Overrides Example
- VB.NET ToTitleCase Function
- VB.NET Convert String Array to String
- VB.NET Iterator Example: Yield Keyword
- VB.NET Mid Statement
- VB.NET Mod Operator (Odd, Even Numbers)
- VB.NET Convert String to Integer
- VB.NET Module Example: Shared Data
- VB.NET Integer
- VB.NET Keywords
- VB.NET Lambda Expressions
- VB.NET LastIndexOf Function
- VB.NET String Join Examples
- VB.NET Multiple Return Values
- VB.NET MustInherit Class: Shadows and Overloads
- VB.NET Namespace Example
- VB.NET KeyValuePair Examples
- VB.NET Environment.NewLine: vbCrLf
- VB.NET Levenshtein Distance Algorithm
- VB.NET Shared Function
- VB.NET Shell Function: Start EXE Program
- VB.NET Sleep Subroutine (Pause)
- VB.NET Sort Dictionary
- VB.NET Exit Statements
- VB.NET LINQ Examples
- VB.NET List Examples
- VB.NET Extension Method
- VB.NET Select Case Examples
- VB.NET MessageBox.Show Examples
- VB.NET Timer Examples
- VB.NET TimeSpan Examples
- VB.NET BackgroundWorker
- VB.NET String Between, Before and After Functions
- VB.NET CStr Function
- VB.NET DataRow Field Extension
- VB.NET DataRow Examples
- VB.NET DateTime Format
- VB.NET Char Examples
- VB.NET DateTime.Now Property (Today)
- VB.NET DateTime.Parse: Convert String to DateTime
- VB.NET DateTime Examples
- VB.NET Decimal Type
- VB.NET HashSet Example
- VB.NET Hashtable Type
- VB.NET Fibonacci Sequence
- VB.NET XmlWriter, Create XML File
- VB.NET File.Copy: Examples, Overwrite
- VB.NET File.Exists: Get Boolean
- VB.NET Path.GetExtension: File Extension
- VB.NET Tuple Examples
- VB.NET Trim Function
- VB.NET TrimEnd and TrimStart Examples
- VB.NET Word Count Function
- VB.NET Word Interop Example
- VB.NET For Loop Examples (For Each)
- VB.NET XElement Example
- VB.NET Truncate String
- VB.NET Sort Number Strings
- VB.NET Sort Examples: Arrays and Lists
- VB.NET SortedList
- VB.NET SortedSet Examples
- VB.NET Split String Examples
- VB.NET Uppercase First Letter
- VB.NET XmlReader, Parse XML File
- VB.NET ZipFile Example
- VB.NET Array.Reverse Example
- VB.NET Random Lowercase Letter
- VB.NET Byte Array: Memory Usage
- VB.NET Byte and Sbyte Types
- VB.NET Char Array
- VB.NET Random String
- VB.NET Random Numbers
- VB.NET Async, Await Example: Task Start and Wait
- VB.NET Choose Function (Get Argument at Index)
- VB.NET Sort by File Size
- VB.NET Sort List (Lambda That Calls CompareTo)
- VB.NET List Find and Exists Examples
- VB.NET Math.Sqrt Function
- VB.NET Loop Over String: For, For Each
Related Links
Adjectives
Ado
Ai
Android
Angular
Antonyms
Apache
Articles
Asp
Autocad
Automata
Aws
Azure
Basic
Binary
Bitcoin
Blockchain
C
Cassandra
Change
Coa
Computer
Control
Cpp
Create
Creating
C-Sharp
Cyber
Daa
Data
Dbms
Deletion
Devops
Difference
Discrete
Es6
Ethical
Examples
Features
Firebase
Flutter
Fs
Git
Go
Hbase
History
Hive
Hiveql
How
Html
Idioms
Insertion
Installing
Ios
Java
Joomla
Js
Kafka
Kali
Laravel
Logical
Machine
Matlab
Matrix
Mongodb
Mysql
One
Opencv
Oracle
Ordering
Os
Pandas
Php
Pig
Pl
Postgresql
Powershell
Prepositions
Program
Python
React
Ruby
Scala
Selecting
Selenium
Sentence
Seo
Sharepoint
Software
Spellings
Spotting
Spring
Sql
Sqlite
Sqoop
Svn
Swift
Synonyms
Talend
Testng
Types
Uml
Unity
Vbnet
Verbal
Webdriver
What
Wpf