<< Back to VBNET
VB.NET Regex.Match Examples: Regular Expressions
Use Regex from System.Text.RegularExpressions. Match Strings based on patterns.Regex. For testing and manipulating text, the Regex class is useful. With Regex, we use a text-processing language. This language easily handles string data.
Functions. With Match, we search strings. And with Replace, we change those we find. And often RegexOptions are used to change how these functions are evaluated.
Match example. This program uses Regex. Please notice the System.Text.RegularExpressions namespace—this is important to get started with regular expressions.
Step 1: We create a Regex object. The Regex pattern "\d+" matches one or more digit characters together.
Step 2: We invoke the Match Function on the Regex instance. This returns a Match (which we test next).
Step 3: We test if the match is successful. If it is, we print (with Console.WriteLine) its value—the string "77."
VB.NET program that uses Regex
Imports System.Text.RegularExpressions
Module Module1
Sub Main()
' Step 1: create Regex.
Dim regex As Regex = New Regex("\d+")
' Step 2: call Match on Regex.
Dim match As Match = regex.Match("Dot 77 Perls")
' Step 3: test the Success bool.
' ... If we have Success, write the Value.
If match.Success Then
Console.WriteLine(match.Value)
End If
End Sub
End Module
Output
77
Pattern details:
\d Match digit char.
+ Match 1 or more chars.
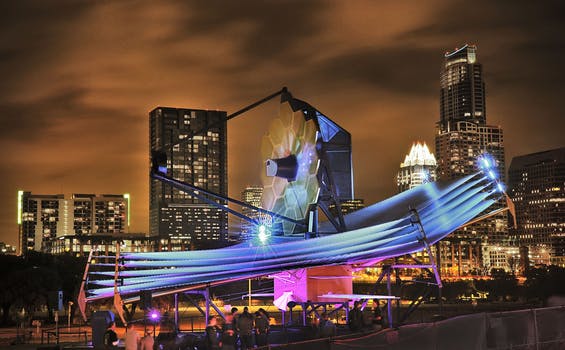
IgnoreCase. Next, we use different syntax, and an option, for Match. We call the Regex.Match shared Function—no Regex object is needed. We then specify an option, RegexOptions.IgnoreCase.
IgnoreCase: This enum value, a constant, specifies that lower and uppercase letters are equal.
VB.NET program that uses RegexOptions.IgnoreCase
Imports System.Text.RegularExpressions
Module Module1
Sub Main()
' Match ignoring case of letters.
Dim match As Match = Regex.Match("I like that cat",
"C.T",
RegexOptions.IgnoreCase)
If match.Success Then
' Write value.
Console.WriteLine(match.Value)
End If
End Sub
End Module
Output
cat
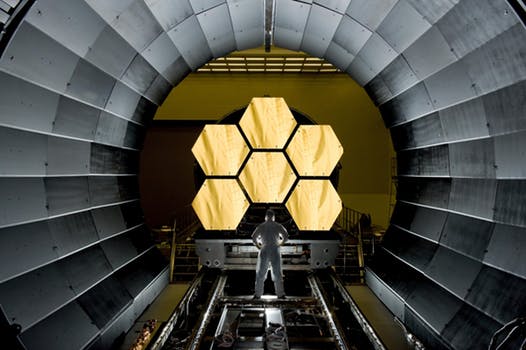
Groups. This example uses Match and Groups. We specify the case of letters is unimportant with RegexOptions.IgnoreCase. And finally we test for Success on the Match object received.
Info: When we execute this program, we see the target text was successfully extracted from the input.
Groups index: We use the value 1 to get the first group from the Match. With Regex, indexing starts at 1 not 0 (don't ask why).
VB.NET program that uses Regex.Match
Imports System.Text.RegularExpressions
Module Module1
Sub Main()
' The input string.
Dim value As String = "/content/alternate-1.aspx"
' Invoke the Match method.
Dim m As Match = Regex.Match(value, _
"content/([A-Za-z0-9\-]+)\.aspx$", _
RegexOptions.IgnoreCase)
' If successful, write the group.
If (m.Success) Then
Dim key As String = m.Groups(1).Value
Console.WriteLine(key)
End If
End Sub
End Module
Output
alternate-1
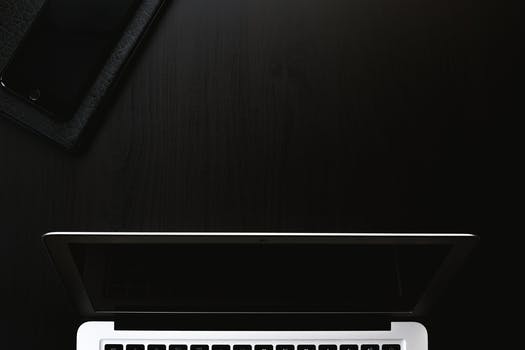
Shared. A Regex object requires time to be created. We can instead share Regex objects, with the shared keyword. A shared Regex object is faster than shared Regex Functions.
SharedTherefore: Storing a Regex as a field in a module or class often results in a speed boost, when Match is called more than once.
Function: The Match function is an instance function on a Regex object. This program has the same result as the previous program.
VB.NET program that uses Match on Regex field
Imports System.Text.RegularExpressions
Module Module1
''' <summary>
''' Member field regular expression.
''' </summary>
Private _reg As Regex = New Regex("content/([A-Za-z0-9\-]+)\.aspx$", _
RegexOptions.IgnoreCase)
Sub Main()
' The input string.
Dim value As String = "/content/alternate-1.aspx"
' Invoke the Match method.
' ... Use the regex field.
Dim m As Match = _reg.Match(value)
' If successful, write the group.
If (m.Success) Then
Dim key As String = m.Groups(1).Value
Console.WriteLine(key)
End If
End Sub
End Module
Output
alternate-1
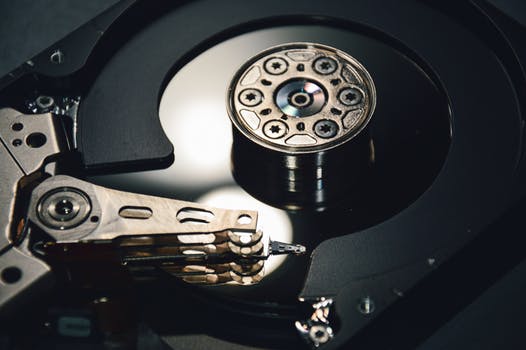
Match, NextMatch. The Match() Function returns the first match only. But we can call NextMatch() on that returned Match object. This is a match that is found in the text, further on.
Tip: NextMatch can be called in a loop. This results in behavior similar to the Matches method (which may be easier to use).
For Each, ForVB.NET program that uses Match, NextMatch
Imports System.Text.RegularExpressions
Module Module1
Sub Main()
' Get first match.
Dim match As Match = Regex.Match("4 and 5", "\d")
If match.Success Then
Console.WriteLine(match.Value)
End If
' Get next match.
match = match.NextMatch()
If match.Success Then
Console.WriteLine(match.Value)
End If
End Sub
End Module
Output
4
5
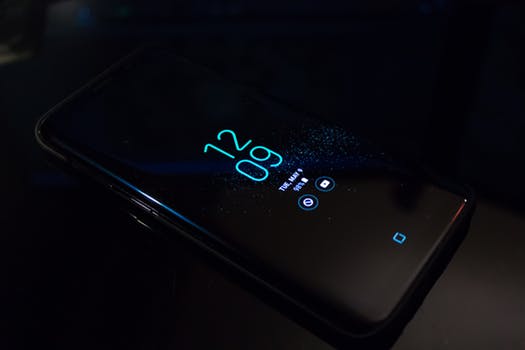
IsMatch. This returns true if a String matches the regular expression. We get a Boolean that tells us whether a pattern matches. If no other results are needed, IsMatch is useful.
Here: This program introduces the IsValid Boolean function, which computes the result of the Regex.IsMatch function on its parameter.
Note: The regular expression pattern indicates any string of lowercase ASCII letters, uppercase ASCII letters, or digits.
VB.NET program that uses Regex.IsMatch function
Imports System.Text.RegularExpressions
Module Module1
Function IsValid(ByRef value As String) As Boolean
Return Regex.IsMatch(value, "^[a-zA-Z0-9]*$")
End Function
Sub Main()
Console.WriteLine(IsValid("dotnetCodex0123"))
Console.WriteLine(IsValid("DotNetPerls"))
Console.WriteLine(IsValid(":-)"))
End Sub
End Module
Output
True
True
False
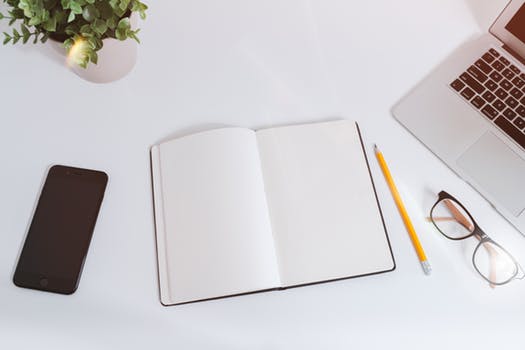
Start and ends. Matching the start and end of a String is commonly-needed. We use the metacharacters "^" and "$" to match the starts and ends of a string.
Note: IsMatch() evaluates these metacharacters in the same way that Match (or Matches) can—the result is different for each function.
VB.NET program that uses IsMatch, tests start and end
Imports System.Text.RegularExpressions
Module Module1
Sub Main()
Dim value As String = "xxyy"
' Match the start with a "^" char.
If Regex.IsMatch(value, "^xx") Then
Console.WriteLine("ISMATCH START")
End If
' Match the end with a "$" char.
If Regex.IsMatch(value, "yy$") Then
Console.WriteLine("ISMATCH END")
End If
End Sub
End Module
Output
ISMATCH START
ISMATCH END
Pattern details:
^ Start of string.
xx 2 x chars.
yy 2 y chars.
$ End of string.
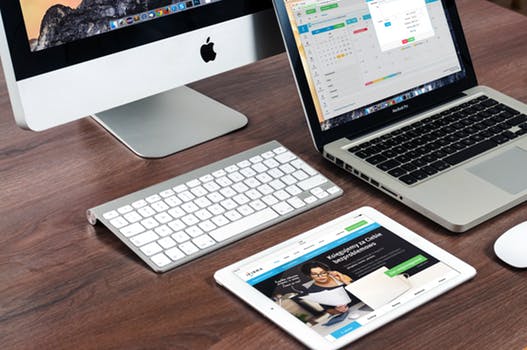
Benchmark, Compiled Regex. To optimize Regex performance in VB.NET, we can use the RegexOptions.Compiled enum and store the Regex in a field. Here we test Compiled Regexes.
Version 1: This code uses a Regex field in the Module, and calls IsMatch on the field instance.
Version 2: Uses Regex.IsMatch directly with no stored Regex instance. This code does the same thing as version 1.
Result: The optimizations applied (a field, and RegexOptions.Compiled) makes regular expression testing much faster.
VB.NET program that times RegexOptions.Compiled
Imports System.Text.RegularExpressions
Module Module1
Dim _regex As Regex = New Regex("X.+0", RegexOptions.Compiled)
Sub Version1()
' Use compiled regular expression stored as field.
If _regex.IsMatch("X12340") = False Then
Throw New Exception
End If
End Sub
Sub Version2()
' Do not use compiled Regex.
If Regex.IsMatch("X12340", "X.+0") = False Then
Throw New Exception
End If
End Sub
Sub Main()
Dim m As Integer = 100000
Dim s1 As Stopwatch = Stopwatch.StartNew
' Version 1: use RegexOptions.Compiled.
For i As Integer = 0 To m - 1
Version1()
Next
s1.Stop()
Dim s2 As Stopwatch = Stopwatch.StartNew
' Version 2: do not compile the Regex.
For i As Integer = 0 To m - 1
Version2()
Next
s2.Stop()
Dim u As Integer = 1000000
Console.WriteLine(((s1.Elapsed.TotalMilliseconds * u) / m).ToString("0.00 ns"))
Console.WriteLine(((s2.Elapsed.TotalMilliseconds * u) / m).ToString("0.00 ns"))
End Sub
End Module
Output
131.78 ns IsMatch, RegexOptions.Compiled
484.66 ns IsMatch
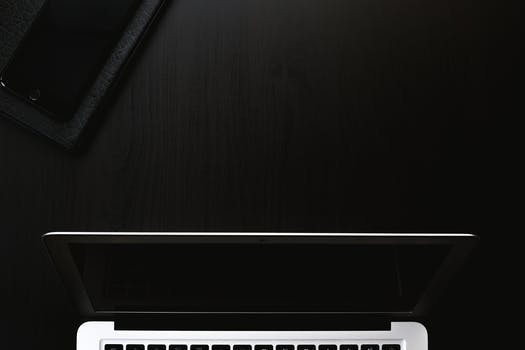
Matches. This function is used to locate and return parts of the source String in separate variables. To capture groups, we use parentheses in a Regex pattern.
Regex.MatchesRegex.Matches Quote
Some examples. In these programs, I remove HTML tags. Be careful—this does not work on all HTML. I also count words in English text (this is also not perfect).
HTML TagsWord Counts
Replace. This function takes an optional MatchEvaluator. It will perform both a matching operation and a replacement of the matching parts.
Regex.ReplaceSplit. Sometimes the String Split function is just not enough for our splitting needs. For these times, try the Regex.Split function.
Regex.SplitFiles. See how you can read in the lines of a file, and parse each line, accessing only a part of the text from each line. The StreamReader type and Regex.Match Function are used.
Regex FileIn some programs, a Regex function is the easiest way to process text. In others, it adds complexity for little gain. As developers, we must decide when to use Regex.
At its core, the Regex type exposes a text-processing language, one built upon finite deterministic automata. Tiny programs efficiently manipulate text.
Related Links:
- VB.NET Nullable
- VB.NET Convert Char Array to String
- VB.NET Object Array
- VB.NET File.ReadAllText, Get String From File
- VB.NET Compress File: GZipStream Example
- VB.NET Console.WriteLine (Print)
- VB.NET File.ReadLines Example
- VB.NET AddressOf Operator
- VB.NET Recursion Example
- VB.NET Recursive File Directory Function
- VB.NET Regex, Read and Match File Lines
- VB.NET Regex.Matches Quote Example
- VB.NET Regex.Matches: For Each Match, Capture
- VB.NET Convert String, Byte Array
- VB.NET File Size: FileInfo Example
- VB.NET File Handling
- VB.NET String.Format Examples: String and Integer
- VB.NET SyncLock Statement
- VB.NET TextInfo Examples
- VB.NET Array.Copy Example
- VB.NET HtmlEncode, HtmlDecode Examples
- VB.NET HtmlTextWriter Example
- VB.NET Stack Type
- VB.NET Func, Action and Predicate Examples
- VB.NET Function Examples
- VB.NET GoTo Example: Labels, Nested Loops
- VB.NET Array.Find Function, FindAll
- VB.NET HttpClient Example: System.Net.Http
- VB.NET DataColumn Class
- VB.NET DataGridView
- VB.NET DataSet Examples
- VB.NET DataTable Select Function
- VB.NET DataTable Examples
- VB.NET Attribute Examples
- VB.NET OpenFileDialog Example
- VB.NET Benchmark
- VB.NET BinaryReader Example
- VB.NET BinarySearch List
- VB.NET BinaryWriter Example
- VB.NET Regex.Replace Function
- VB.NET Regex.Split Examples
- VB.NET Regex.Match Examples: Regular Expressions
- VB.NET Convert ArrayList to Array
- VB.NET Array Examples, String Arrays
- VB.NET ArrayList Examples
- VB.NET Boolean, True, False and Not (Return True)
- VB.NET Nothing, IsNothing (Null)
- VB.NET Directive Examples: Const, If and Region
- VB.NET Do Until Loops
- VB.NET Do While Loop Examples (While)
- VB.NET Array.Resize Subroutine
- VB.NET Chr Function: Get Char From Integer
- VB.NET Class Examples
- VB.NET IndexOf Function
- VB.NET Insert String
- VB.NET Interface Examples (Implements)
- VB.NET 2D, 3D and Jagged Array Examples
- VB.NET Enum.Parse, TryParse: Convert String to Enum
- VB.NET Remove HTML Tags
- VB.NET Remove String
- VB.NET Event Example: AddHandler, RaiseEvent
- VB.NET Excel Interop Example
- VB.NET StartsWith and EndsWith String Functions
- VB.NET Initialize List
- VB.NET Number Examples
- VB.NET Optional String, Integer: Named Arguments
- VB.NET Replace String Examples
- VB.NET Exception Handling: Try, Catch and Finally
- VB.NET Enum Examples
- VB.NET Enumerable.Range, Repeat and Empty
- VB.NET Dictionary Examples
- VB.NET Double Type
- VB.NET LSet and RSet Functions
- VB.NET LTrim and RTrim Functions
- VB.NET Alphanumeric Sorting
- VB.NET PadLeft and PadRight
- VB.NET String.Concat Examples
- VB.NET String
- VB.NET Math.Abs: Absolute Value
- VB.NET Array.IndexOf, LastIndexOf
- VB.NET Remove Duplicate Chars
- VB.NET If Then, ElseIf, Else Examples
- VB.NET ParamArray (Use varargs Functions)
- VB.NET Integer.Parse: Convert String to Integer
- VB.NET ThreadPool
- VB.NET Process Examples (Process.Start)
- VB.NET TimeZone Example
- VB.NET Path Examples
- VB.NET ToArray Extension Example
- VB.NET ToCharArray Function
- VB.NET Stopwatch Example
- VB.NET Button Example
- VB.NET StreamReader ReadToEnd Function
- VB.NET ByVal Versus ByRef Example
- VB.NET StreamReader Example
- VB.NET StreamWriter Example
- VB.NET String.Compare Examples
- VB.NET Cast: TryCast, DirectCast Examples
- VB.NET String Constructor (New String)
- VB.NET String.Copy and CopyTo
- VB.NET Math.Ceiling and Floor: Double Examples
- VB.NET Math.Max and Math.Min
- VB.NET WebClient: DownloadData, Headers
- VB.NET Math.Round Example
- VB.NET Math.Truncate Method, Cast Double to Integer
- VB.NET Reverse String
- VB.NET Structure Examples
- VB.NET Sub Examples
- VB.NET Substring Examples
- VB.NET Convert Dictionary to List
- VB.NET Convert List and Array
- VB.NET Convert List to String
- VB.NET Convert Miles to Kilometers
- VB.NET Property Examples (Get, Set)
- VB.NET Remove Punctuation From String
- VB.NET Queue Examples
- VB.NET Const Values
- VB.NET Remove Duplicates From List
- VB.NET IComparable Example
- VB.NET ReDim Keyword (Array.Resize)
- VB.NET Contains Example
- VB.NET IEnumerable Examples
- VB.NET IsNot and Is Operators
- VB.NET String.IsNullOrEmpty, IsNullOrWhiteSpace
- VB.NET ROT13 Encode Function
- VB.NET StringBuilder Examples
- VB.NET Image Type
- VB.NET Val, Asc and AscW Functions
- VB.NET String.Empty Example
- VB.NET String.Equals Function
- VB.NET VarType Function (VariantType Enum)
- VB.NET With Statement
- VB.NET WithEvents: Handles and RaiseEvent
- VB.NET String Length Example
- VB.NET ToList Extension Example
- VB.NET ToLower and ToUpper Examples
- VB.NET TextBox Example
- VB.NET ToString Overrides Example
- VB.NET ToTitleCase Function
- VB.NET Convert String Array to String
- VB.NET Iterator Example: Yield Keyword
- VB.NET Mid Statement
- VB.NET Mod Operator (Odd, Even Numbers)
- VB.NET Convert String to Integer
- VB.NET Module Example: Shared Data
- VB.NET Integer
- VB.NET Keywords
- VB.NET Lambda Expressions
- VB.NET LastIndexOf Function
- VB.NET String Join Examples
- VB.NET Multiple Return Values
- VB.NET MustInherit Class: Shadows and Overloads
- VB.NET Namespace Example
- VB.NET KeyValuePair Examples
- VB.NET Environment.NewLine: vbCrLf
- VB.NET Levenshtein Distance Algorithm
- VB.NET Shared Function
- VB.NET Shell Function: Start EXE Program
- VB.NET Sleep Subroutine (Pause)
- VB.NET Sort Dictionary
- VB.NET Exit Statements
- VB.NET LINQ Examples
- VB.NET List Examples
- VB.NET Extension Method
- VB.NET Select Case Examples
- VB.NET MessageBox.Show Examples
- VB.NET Timer Examples
- VB.NET TimeSpan Examples
- VB.NET BackgroundWorker
- VB.NET String Between, Before and After Functions
- VB.NET CStr Function
- VB.NET DataRow Field Extension
- VB.NET DataRow Examples
- VB.NET DateTime Format
- VB.NET Char Examples
- VB.NET DateTime.Now Property (Today)
- VB.NET DateTime.Parse: Convert String to DateTime
- VB.NET DateTime Examples
- VB.NET Decimal Type
- VB.NET HashSet Example
- VB.NET Hashtable Type
- VB.NET Fibonacci Sequence
- VB.NET XmlWriter, Create XML File
- VB.NET File.Copy: Examples, Overwrite
- VB.NET File.Exists: Get Boolean
- VB.NET Path.GetExtension: File Extension
- VB.NET Tuple Examples
- VB.NET Trim Function
- VB.NET TrimEnd and TrimStart Examples
- VB.NET Word Count Function
- VB.NET Word Interop Example
- VB.NET For Loop Examples (For Each)
- VB.NET XElement Example
- VB.NET Truncate String
- VB.NET Sort Number Strings
- VB.NET Sort Examples: Arrays and Lists
- VB.NET SortedList
- VB.NET SortedSet Examples
- VB.NET Split String Examples
- VB.NET Uppercase First Letter
- VB.NET XmlReader, Parse XML File
- VB.NET ZipFile Example
- VB.NET Array.Reverse Example
- VB.NET Random Lowercase Letter
- VB.NET Byte Array: Memory Usage
- VB.NET Byte and Sbyte Types
- VB.NET Char Array
- VB.NET Random String
- VB.NET Random Numbers
- VB.NET Async, Await Example: Task Start and Wait
- VB.NET Choose Function (Get Argument at Index)
- VB.NET Sort by File Size
- VB.NET Sort List (Lambda That Calls CompareTo)
- VB.NET List Find and Exists Examples
- VB.NET Math.Sqrt Function
- VB.NET Loop Over String: For, For Each
Related Links
Adjectives
Ado
Ai
Android
Angular
Antonyms
Apache
Articles
Asp
Autocad
Automata
Aws
Azure
Basic
Binary
Bitcoin
Blockchain
C
Cassandra
Change
Coa
Computer
Control
Cpp
Create
Creating
C-Sharp
Cyber
Daa
Data
Dbms
Deletion
Devops
Difference
Discrete
Es6
Ethical
Examples
Features
Firebase
Flutter
Fs
Git
Go
Hbase
History
Hive
Hiveql
How
Html
Idioms
Insertion
Installing
Ios
Java
Joomla
Js
Kafka
Kali
Laravel
Logical
Machine
Matlab
Matrix
Mongodb
Mysql
One
Opencv
Oracle
Ordering
Os
Pandas
Php
Pig
Pl
Postgresql
Powershell
Prepositions
Program
Python
React
Ruby
Scala
Selecting
Selenium
Sentence
Seo
Sharepoint
Software
Spellings
Spotting
Spring
Sql
Sqlite
Sqoop
Svn
Swift
Synonyms
Talend
Testng
Types
Uml
Unity
Vbnet
Verbal
Webdriver
What
Wpf