<< Back to F#
F# dict: Dictionary Examples, Item and Keys
Create a dictionary with dict. Use Item, Keys and TryGetValue to access the dictionary.Dict. A connection is made. A wolf howls in the dark of night. A cat has soft fur. We link things to values of things: in F# we could use a dict to make these links.
Read-only. A dict is read-only: we create it once and cannot modify its contents. We use the dict keyword. A Dictionary, which can be changed, may also be used when mutability is needed.
Keywords
Syntax. Let us begin with a simple example. We create a "colors" dictionary with string keys and int values. This is a read-only sequence of key-value pairs.
Dict: This keyword allows quick construction of a lookup table. For simple requirements, this is a good choice.
Item: This returns the value for a key in the dict. We use printfn with an "%A" to print the int value returned.
F# program that creates dictionary, gets values
// Create a dictionary with two key-value pairs.
let colors = dict["blue", 40; "red", 700]
// Print value of "blue" key.
printfn "%A" (colors.Item("blue"))
// Print value of "red" key.
printfn "%A" (colors.Item("red"))
Output
40
700
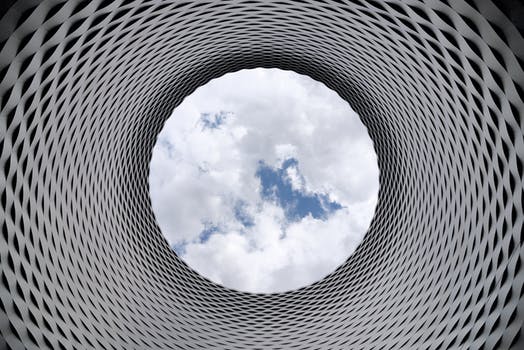
Count, for-loop. A dict has many features—it supports all of the methods from the IDictionary interface. Often we must count, or iterate over, a dict's contents.
Count: This returns an int. Here we see our dict has two key-value pairs. We store this value in the constant "c."
For: We use a for-in loop to print all the values of the dict. We use a do keyword to indicate the start of the loop body.
F# program that uses count, for-loop on dict
// Create a dictionary with dict keyword.
let sizes = dict["small", 0; "large", 10]
// Get count of pairs and display it.
let c = sizes.Count
printfn "%A" c
// Loop over pairs in the dictionary.
// ... Display each KeyValuePair.
for pair in sizes do
printfn "%A" pair
Output
2
[small, 0]
[large, 10]
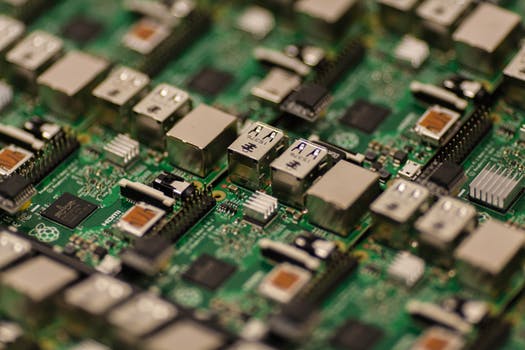
Keys. With this property we get a collection of the keys in the dict. With this collection (which is not a list) we can use a function like Seq.iter. Here we print all the keys.
SeqFunPrintfnF# program that displays keys in dict
let places = dict["Canada", 10; "Germany", 20]
// Get keys in a collection.
let keys = places.Keys
// Display all keys with labels.
Seq.iter (fun key -> printfn "Key = %A" key) keys
Output
Key = "Canada"
Key = "Germany"
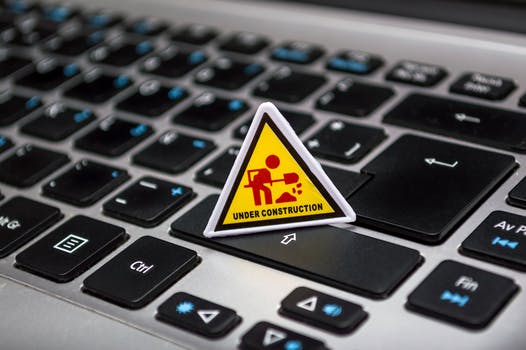
Error. A dict is read-only. It is immutable. This means we cannot set or add items (keys or values) to it. To add keys we must create a new dict.
F# program that causes dict exception
// Create a lookup table.
let lookup = dict["100", false; "200", true]
// This causes an exception.
lookup.Item("300") <- false
Output
Unhandled Exception: System.NotSupportedException:
This value cannot be mutated
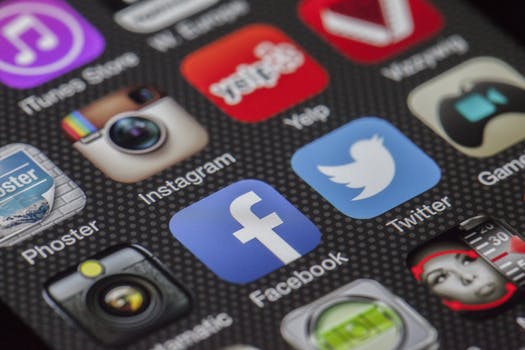
Key-value pairs. We can use the dict keyword with existing key-value pairs. We can create these pairs with tuple syntax. Then we just pass them into the dict expression.
TupleF# program that uses Key-Value pairs, dict
[<EntryPoint>]
let main argv =
let pair = ("carrot", "orange")
let pair2 = ("apple", "red")
// Use dict with Key-Value pairs already created.
let fruit = dict[pair; pair2]
// Write the Count.
printfn "COUNT: %d" fruit.Count
0
Output
COUNT: 2
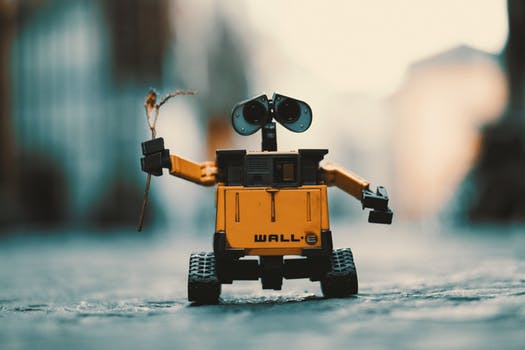
A review. A dict is immutable in F#. This helps with functional programming, but sometimes we want a more versatile lookup table. We use a Dictionary for this purpose.
In this language, special F# constructs like dict can be used with other constructs like the tuple syntax. We can use a 2-item tuple to create an entry in a dict. Immutability is retained.
Related Links:
- F# Files: open System.IO, use StreamReader
- F# Odd, Even Numbers
- F# Record Examples: Type, With Keywords
- F# Recursion Example: rec Keyword
- F# Option int Example: IsNone, IsSome and Value
- F# Array Examples
- F# dict: Dictionary Examples, Item and Keys
- F# IndexOf String Examples: LastIndexOf and Contains
- F# Replace String Examples
- F# Failwith: Exception Handling
- F# Type Example: member, get and set
- F# Match Keyword
- F# Remove Duplicates From List (Seq.distinct)
- F# If, elif and else Examples
- F# Printfn Examples: printf, Formats
- F# Math Operators: abs, floor and ceil
- F# Convert String, Int, Array and Seq
- F# Downcast and upcast Example
- F# ROT13 Cipher (String.map)
- F# String Examples: String.map
- F# Keywords
- F# Seq Examples: Seq.sum, Seq.where
- F# Measure Example: Units of Measurement
- F# Fun Keyword: Lambda Expressions, Functions
- F# Tuple Examples
- F# For and While Loop Examples: For To, For In
- F# Let Keyword: let mutable, Errors
- F# Sort List Examples: sortBy, Lambdas
- F# List Examples, String Lists
- F# Split Strings
Related Links
Adjectives
Ado
Ai
Android
Angular
Antonyms
Apache
Articles
Asp
Autocad
Automata
Aws
Azure
Basic
Binary
Bitcoin
Blockchain
C
Cassandra
Change
Coa
Computer
Control
Cpp
Create
Creating
C-Sharp
Cyber
Daa
Data
Dbms
Deletion
Devops
Difference
Discrete
Es6
Ethical
Examples
Features
Firebase
Flutter
Fs
Git
Go
Hbase
History
Hive
Hiveql
How
Html
Idioms
Insertion
Installing
Ios
Java
Joomla
Js
Kafka
Kali
Laravel
Logical
Machine
Matlab
Matrix
Mongodb
Mysql
One
Opencv
Oracle
Ordering
Os
Pandas
Php
Pig
Pl
Postgresql
Powershell
Prepositions
Program
Python
React
Ruby
Scala
Selecting
Selenium
Sentence
Seo
Sharepoint
Software
Spellings
Spotting
Spring
Sql
Sqlite
Sqoop
Svn
Swift
Synonyms
Talend
Testng
Types
Uml
Unity
Vbnet
Verbal
Webdriver
What
Wpf