<< Back to F#
F# If, elif and else Examples
Add branches with the if, elif and else keywords. Test conditions in programs.If, elif. The forest has many trees, a pond, and some frogs in the pond. Are there 2 frogs, or 3 frogs? With a conditional statement we could test this information.
In our programs we usually use ifs to execute statements inside the blocks. But in F# we can use an if-construct to return a value. It is part of an expression.
An example. This program uses an if, elif, else construct. It first creates a string. Then it tests the length of this string in the if-statement.
If then: We must use the "then" keyword after the if-condition. A "then" is also required for an elif, but not an else.
Equals: We use a single equals sign to test for equality in an expression. The expression of an if-statement must be in parentheses.
F# program that uses if, elif and else
let animal = "bird"
// Test the length of the string.
if (animal.Length = 1) then
// Not reached.
printfn "A"
elif (animal.Length = 2) then
// Not reached.
printfn "B"
else
// This statement is reached.
printfn "C"
Output
C
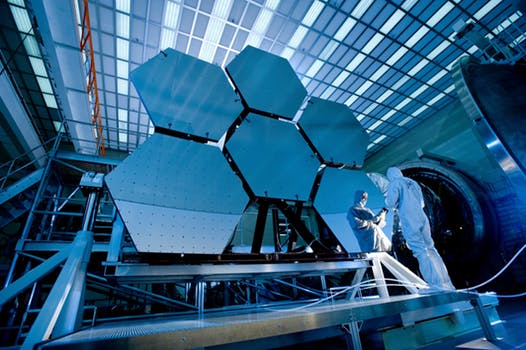
If inside let. An if-statement can be used to return a value (as an expression). We can embed an if in more complex statements. Here we assign the value of "result" with an if.
Info: The program sets the value of result to 1 if count is equal to or greater than 200. It also has two other conditions.
F# program that uses if inside let statement
let count = 50
// Use an if, elif, else construct within a variable assignment.
let result =
if count >= 200 then 1
elif count <= 100 then 2
else 3
// Write results.
printfn "%A" count
printfn "%A" result
Output
50
2
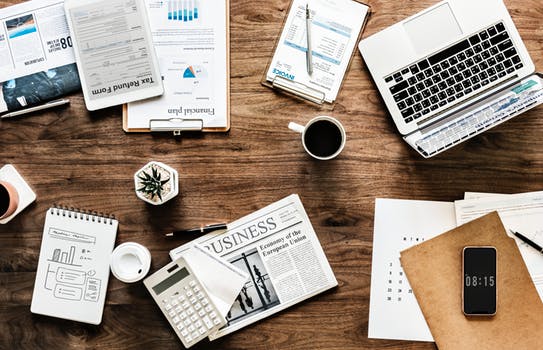
Not. There is no "!=" operator for ints in F#. To see if an int does not equal a value, we use the equals operator and then surround that expression with the "not" operator.
F# program that uses if-not
let code = 10
// Use an if-not statement to test a variable.
if not (code = 5) then
printfn "Not five!"
Output
Not five!
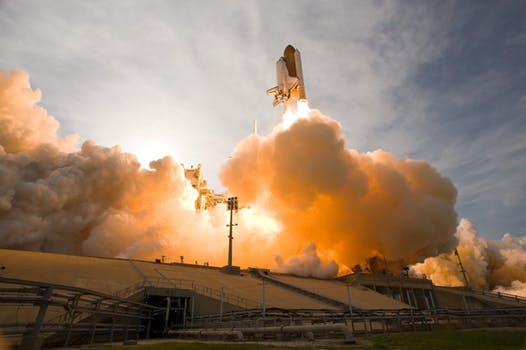
Match versus if. We can write a logical test with a match or an if-expression. The syntax for match is closer to a "switch" in C-like languages. A match may be easier to use an expression.
TestPrint: This statement creates a function that tests its argument "v" and prints a statement based on its value. It uses "match."
TestPrintIf: This does the same thing but uses an if-statement. You can see this version looks more like C# or C code.
F# program that uses match, if expressions
// We can use a match to handle the argument.
let testPrint v =
match v with
| 0 | 1 | 2 -> printfn "[MATCH] branch A: %A" v
| _ -> printfn "[MATCH] branch B: %A" v
// We can use an if-else to handle the argument.
let testPrintIf v =
if v = 0 || v = 1 || v = 2 then
printfn "[IF] branch A: %A" v
else
printfn "[IF] branch B: %A" v
// Test functions.
testPrint 0
testPrint 9
testPrintIf 0
testPrintIf 9
Output
[MATCH] branch A: 0
[MATCH] branch B: 9
[IF] branch A: 0
[IF] branch B: 9
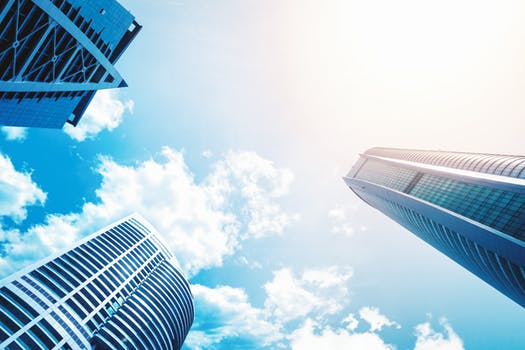
Convert bool to int. F# offers no C-like ternary operator. Instead, we use inline if-expressions. With an if-expression we can convert a bool to an int.
Convert: bool to int
A review. An if-block operates in F# much like in other languages. But it has a special feature. It can evaluate an if as an expression, which can return values or be used in assignments.
Related Links:
- F# Files: open System.IO, use StreamReader
- F# Odd, Even Numbers
- F# Record Examples: Type, With Keywords
- F# Recursion Example: rec Keyword
- F# Option int Example: IsNone, IsSome and Value
- F# Array Examples
- F# dict: Dictionary Examples, Item and Keys
- F# IndexOf String Examples: LastIndexOf and Contains
- F# Replace String Examples
- F# Failwith: Exception Handling
- F# Type Example: member, get and set
- F# Match Keyword
- F# Remove Duplicates From List (Seq.distinct)
- F# If, elif and else Examples
- F# Printfn Examples: printf, Formats
- F# Math Operators: abs, floor and ceil
- F# Convert String, Int, Array and Seq
- F# Downcast and upcast Example
- F# ROT13 Cipher (String.map)
- F# String Examples: String.map
- F# Keywords
- F# Seq Examples: Seq.sum, Seq.where
- F# Measure Example: Units of Measurement
- F# Fun Keyword: Lambda Expressions, Functions
- F# Tuple Examples
- F# For and While Loop Examples: For To, For In
- F# Let Keyword: let mutable, Errors
- F# Sort List Examples: sortBy, Lambdas
- F# List Examples, String Lists
- F# Split Strings
Related Links
Adjectives
Ado
Ai
Android
Angular
Antonyms
Apache
Articles
Asp
Autocad
Automata
Aws
Azure
Basic
Binary
Bitcoin
Blockchain
C
Cassandra
Change
Coa
Computer
Control
Cpp
Create
Creating
C-Sharp
Cyber
Daa
Data
Dbms
Deletion
Devops
Difference
Discrete
Es6
Ethical
Examples
Features
Firebase
Flutter
Fs
Git
Go
Hbase
History
Hive
Hiveql
How
Html
Idioms
Insertion
Installing
Ios
Java
Joomla
Js
Kafka
Kali
Laravel
Logical
Machine
Matlab
Matrix
Mongodb
Mysql
One
Opencv
Oracle
Ordering
Os
Pandas
Php
Pig
Pl
Postgresql
Powershell
Prepositions
Program
Python
React
Ruby
Scala
Selecting
Selenium
Sentence
Seo
Sharepoint
Software
Spellings
Spotting
Spring
Sql
Sqlite
Sqoop
Svn
Swift
Synonyms
Talend
Testng
Types
Uml
Unity
Vbnet
Verbal
Webdriver
What
Wpf