<< Back to F#
F# Math Operators: abs, floor and ceil
Perform computations on numbers with math operators. Call pown, abs, floor and ceil.Math. N is -10. Now it is 10. An absolute value is always positive. We can compute this with some logic. But abs is built-in—it is easier to call.
With special operators, we call built-in mathematical methods. For example, with "pown" we compute an exponent of an integer. This makes programs clearer to read.
Exponents. Let us begin with some exponentiation. Please note that we can call methods from System.Math also, but F# provides some shortcuts.
Pown: We first use pown to take the power of a number (an int). We square the value 4 to get 16.
Exponents: We use two starts to apply a power to a double. We must use 2.0 instead of 2 to square here.
F# program that uses pown, exponents
// Use pown to implement integer exponents.
let number = 4
let squared = pown number 2
printfn "%A" squared
// Use two stars to implement double-type exponents.
let numberDouble = 4.0
let squaredDouble = numberDouble ** 2.0
printfn "%A" squaredDouble
Output
16
16.0
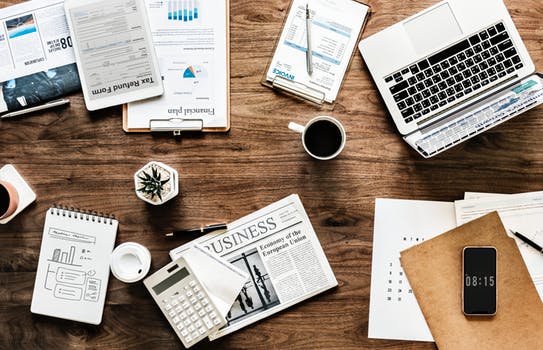
Abs. The absolute value of a number is positive. Numbers that are already positive are not changed. Abs is useful when computing indexes (like with hash codes).
F# program that uses abs
// The absolute value of -10 is 10.
let result1 = abs -10
printfn "%A" result1
// For positive numbers, nothing is changed.
let result2 = abs 1
printfn "%A" result2
Output
10
1
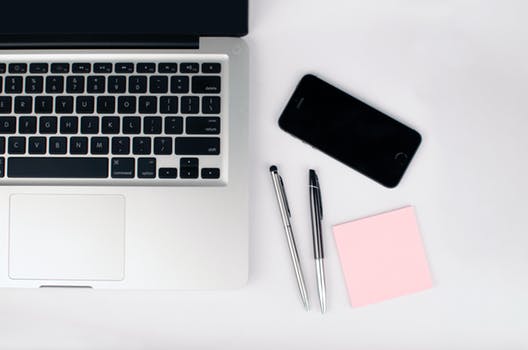
Floor, ceil. We have operators that access many common math methods. For example floor and ceil access the Math.Floor and Math.Ceiling methods.
F# program that uses floor and ceil
// Take the floor of a number with the operator.
let result1 = floor 2.9
printfn "%A" result1
// Take ceiling of a number.
let result2 = ceil 1.1
printfn "%A" result2
Output
2.0
2.0
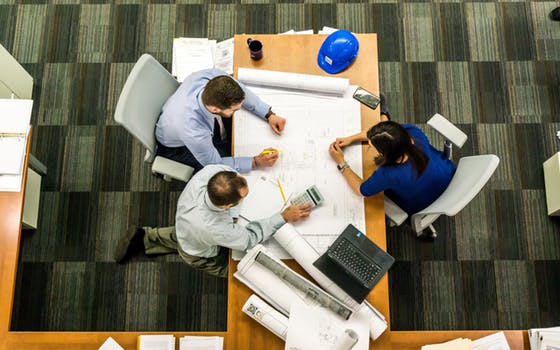
Max, min. We pass two Int arguments to these functions. They return the bigger (for max) or the smaller number (for min). These are built into the F# language.
F# program that uses max, min
let numberSmaller = 10
let numberBigger = 20
// Get the max of the two numbers.
let bigger = max numberSmaller numberBigger
printfn "%A" bigger
// Get the min.
let smaller = min numberSmaller numberBigger
printfn "%A" smaller
Output
20
10
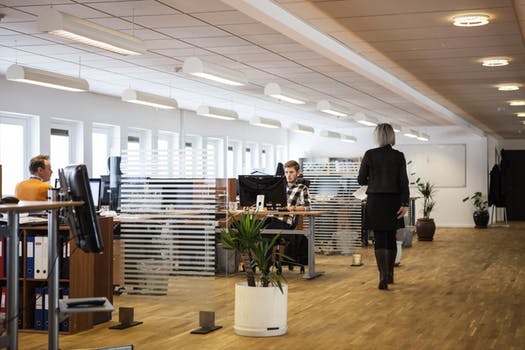
Odd, even. A number can be odd or even—this is the parity of the number. In F# we use Seq.where to filter based on the parity of numbers. We even generate odd and even sequences.
Odd, Even
A summary. F# programs often extensively use numbers. We find these mathematical operators make programs easier to understand to write. When compatible, they should be preferred.
Related Links:
- F# Files: open System.IO, use StreamReader
- F# Odd, Even Numbers
- F# Record Examples: Type, With Keywords
- F# Recursion Example: rec Keyword
- F# Option int Example: IsNone, IsSome and Value
- F# Array Examples
- F# dict: Dictionary Examples, Item and Keys
- F# IndexOf String Examples: LastIndexOf and Contains
- F# Replace String Examples
- F# Failwith: Exception Handling
- F# Type Example: member, get and set
- F# Match Keyword
- F# Remove Duplicates From List (Seq.distinct)
- F# If, elif and else Examples
- F# Printfn Examples: printf, Formats
- F# Math Operators: abs, floor and ceil
- F# Convert String, Int, Array and Seq
- F# Downcast and upcast Example
- F# ROT13 Cipher (String.map)
- F# String Examples: String.map
- F# Keywords
- F# Seq Examples: Seq.sum, Seq.where
- F# Measure Example: Units of Measurement
- F# Fun Keyword: Lambda Expressions, Functions
- F# Tuple Examples
- F# For and While Loop Examples: For To, For In
- F# Let Keyword: let mutable, Errors
- F# Sort List Examples: sortBy, Lambdas
- F# List Examples, String Lists
- F# Split Strings
Related Links
Adjectives
Ado
Ai
Android
Angular
Antonyms
Apache
Articles
Asp
Autocad
Automata
Aws
Azure
Basic
Binary
Bitcoin
Blockchain
C
Cassandra
Change
Coa
Computer
Control
Cpp
Create
Creating
C-Sharp
Cyber
Daa
Data
Dbms
Deletion
Devops
Difference
Discrete
Es6
Ethical
Examples
Features
Firebase
Flutter
Fs
Git
Go
Hbase
History
Hive
Hiveql
How
Html
Idioms
Insertion
Installing
Ios
Java
Joomla
Js
Kafka
Kali
Laravel
Logical
Machine
Matlab
Matrix
Mongodb
Mysql
One
Opencv
Oracle
Ordering
Os
Pandas
Php
Pig
Pl
Postgresql
Powershell
Prepositions
Program
Python
React
Ruby
Scala
Selecting
Selenium
Sentence
Seo
Sharepoint
Software
Spellings
Spotting
Spring
Sql
Sqlite
Sqoop
Svn
Swift
Synonyms
Talend
Testng
Types
Uml
Unity
Vbnet
Verbal
Webdriver
What
Wpf