<< Back to F#
F# Fun Keyword: Lambda Expressions, Functions
Describe higher-order procedures and the fun keyword. Specify lambda expressions.Fun, lambdas. A function is an object. In F# we use the fun-keyword to create lambda expressions. This is powerful. We can pass these functions as arguments.
With map and filter, methods on Lists, we can apply transformations with functions. We can pipeline these calls for simpler syntax. A pipeline operator is available.
First example. Here we specify a lambda expression with "fun" as the argument to List.map. The lambda begins with the fun-keyword.
Fun: On the left side of the lambda, after "fun" we have the argument to the lambda. And the right side is the expression that is returned.
Here: We use List.map to multiply each element in the list by 2. We print the before and after lists.
F# program that uses fun, List.map
let values = [10; 20; 30; 40; 50]
// Use List.map with a lambda expression.
// ... The lambda doubles each element.
let result = List.map (fun x -> x * 2) values
// Print our before and after lists.
printfn "%A" values
printfn "%A" result
Output
[10; 20; 30; 40; 50]
[20; 40; 60; 80; 100]
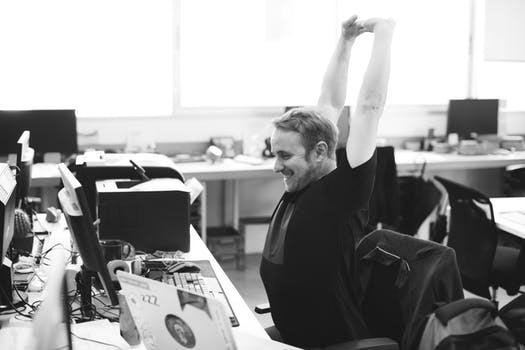
Pipeline. Often we chain multiple method calls together. With the pipeline operator, we can do this without complex nesting of method calls.
Instead: We call one method after another, using the return value from the first as the argument to the second.
Here: We use List.filter to eliminate values less than 3. Then we multiply all remaining values by 2.
F# program that uses pipeline operator
let values = [1; 2; 3; 4; 5]
// Use filter to get only elements greater than or equal to 3.
// ... Use map to double their values.
// Use pipeline operator.
let result =
values
|> List.filter (fun v -> v >= 3)
|> List.map (fun x -> x * 2)
// Print lists.
printfn "%A" values
printfn "%A" result
Output
[1; 2; 3; 4; 5]
[6; 8; 10]
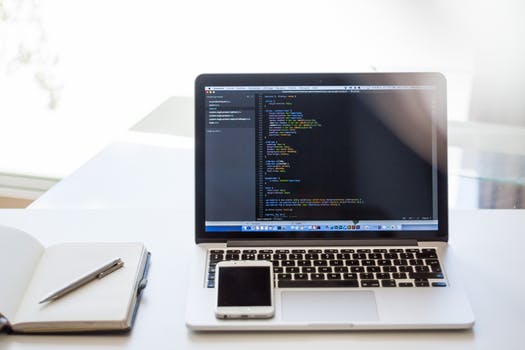
Function composition. With these operators we call two functions, one before the other. A right-pointing arrow calls the functions from left to right. Left arrows go from right to left.
Here: We have two functions, one that divides by 3 and one that adds 1. These are called in different orders (with different arguments).
So: Order matters. Unlike in a pipeline, the same argument is passed to both functions.
F# program that uses function composition
// Some functions.
let divideNumber x = x / 3
let addOneToNumber n = n + 1
// Use function composition, left to right.
// ... This divides then adds (so equals 4).
let composition1 = divideNumber >> addOneToNumber
let result1 = composition1 9
printfn "%A" result1
// Use function composition, right to left.
// ... This adds then divides (so equals 4).
let composition2 = divideNumber << addOneToNumber
let result2 = composition2 11
printfn "%A" result2
Output
4
4
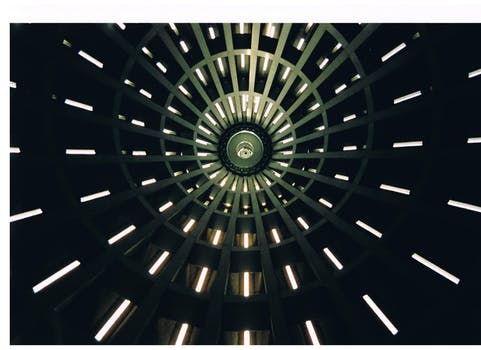
Expression type error. The F# compiler issues a warning when an expression has a return value that is discarded. We use the keyword ignore to specify this is expected.
F# program that causes warning, ignore
let multiplyNumbers left right = left * right
// This causes a warning.
multiplyNumbers 5 10
Output
warning FS0020:
This expression should have type 'unit', but has type 'int'.
Use 'ignore' to discard the result of the expression,
or 'let' to bind the result to a name.
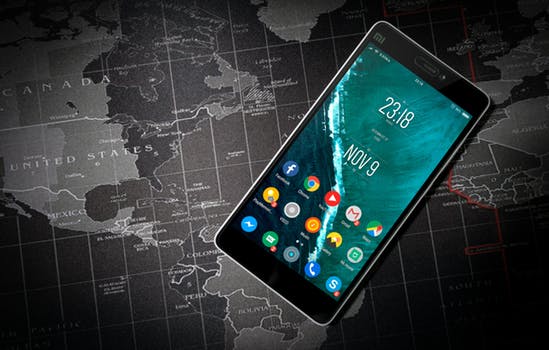
Ignore keyword. Here we use "ignore" to specify that we want the result of an expression to be discarded. The F# compiler issues no warning on this program. And the function has no effect.
F# program that uses ignore
// This function computes a value.
let multiplyNumbers left right = left * right
// Ignore the result of multiplyNumbers.
ignore (multiplyNumbers 5 10)
// Program is done.
printfn "Done"
Output
Done
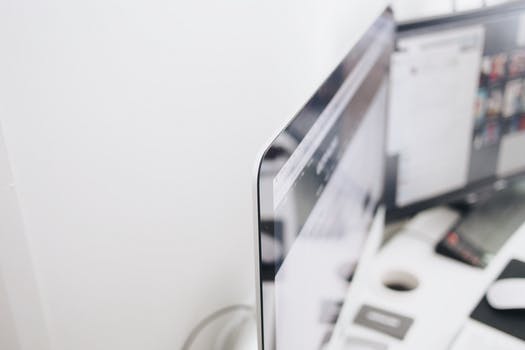
Pattern-matching function. In F# we use the "function" keyword to create a pattern-matching function. No "match" keyword is needed—we directly match arguments.
Pattern-matching Function
Some notes. At first, writing higher-order procedures and lambdas is more complex. But it has a benefit. With code written with pipelines and lambdas, fewer small errors may occur.
And: The goals of the code are expressed closer to their logic. The implementation is emphasized less.
A summary. Higher-order procedures are a key part to F# programming. These lead to clearer, less bug-prone code. We describe logic at a higher level.
Related Links:
- F# Files: open System.IO, use StreamReader
- F# Odd, Even Numbers
- F# Record Examples: Type, With Keywords
- F# Recursion Example: rec Keyword
- F# Option int Example: IsNone, IsSome and Value
- F# Array Examples
- F# dict: Dictionary Examples, Item and Keys
- F# IndexOf String Examples: LastIndexOf and Contains
- F# Replace String Examples
- F# Failwith: Exception Handling
- F# Type Example: member, get and set
- F# Match Keyword
- F# Remove Duplicates From List (Seq.distinct)
- F# If, elif and else Examples
- F# Printfn Examples: printf, Formats
- F# Math Operators: abs, floor and ceil
- F# Convert String, Int, Array and Seq
- F# Downcast and upcast Example
- F# ROT13 Cipher (String.map)
- F# String Examples: String.map
- F# Keywords
- F# Seq Examples: Seq.sum, Seq.where
- F# Measure Example: Units of Measurement
- F# Fun Keyword: Lambda Expressions, Functions
- F# Tuple Examples
- F# For and While Loop Examples: For To, For In
- F# Let Keyword: let mutable, Errors
- F# Sort List Examples: sortBy, Lambdas
- F# List Examples, String Lists
- F# Split Strings
Related Links
Adjectives
Ado
Ai
Android
Angular
Antonyms
Apache
Articles
Asp
Autocad
Automata
Aws
Azure
Basic
Binary
Bitcoin
Blockchain
C
Cassandra
Change
Coa
Computer
Control
Cpp
Create
Creating
C-Sharp
Cyber
Daa
Data
Dbms
Deletion
Devops
Difference
Discrete
Es6
Ethical
Examples
Features
Firebase
Flutter
Fs
Git
Go
Hbase
History
Hive
Hiveql
How
Html
Idioms
Insertion
Installing
Ios
Java
Joomla
Js
Kafka
Kali
Laravel
Logical
Machine
Matlab
Matrix
Mongodb
Mysql
One
Opencv
Oracle
Ordering
Os
Pandas
Php
Pig
Pl
Postgresql
Powershell
Prepositions
Program
Python
React
Ruby
Scala
Selecting
Selenium
Sentence
Seo
Sharepoint
Software
Spellings
Spotting
Spring
Sql
Sqlite
Sqoop
Svn
Swift
Synonyms
Talend
Testng
Types
Uml
Unity
Vbnet
Verbal
Webdriver
What
Wpf