<< Back to F#
Split. A string contains many values. It has names of animals separated by commas. We must split this string. Splitting is not exciting. But it is useful.
With Split, a method on the String type in the .NET Framework, we receive a String array. And with the helpful Seq.toList we can convert his into an F# list for further use.
Simple example. We introduce a string with three fields separated by commas. We call the Split method with a single comma char—this is expanded to a char array.
Array: We receive a string array from Split containing 3 elements. We use a for-in loop to display them to the screen.
ArrayPrintfnF# program that uses Split
let animals = "bear,frog,eagle"
// Split on the comma.
// ... This is creating a single-element character array for us.
let result = animals.Split ','
// Loop over and display strings.
for value in result do
printfn "%A" value
Output
"bear"
"frog"
"eagle"
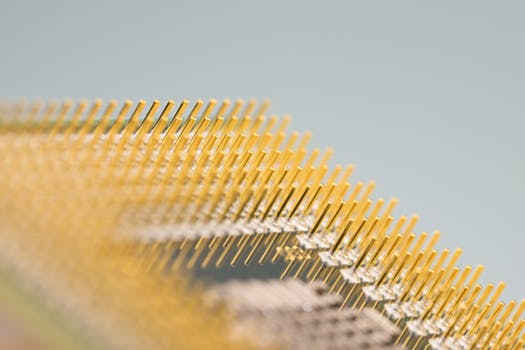
Seq.toList. This example adds some complexity. We introduce a splitLine function. This receives a string and calls Split on it. Then it returns the result of Seq.toList.
SeqSo: When we invoke splitLine on a string, we can access the data in an F# list.
ListF# program that uses split, Seq.toList
// Create a splitLine function.
// ... It uses Seq.toList on the result of line.Split.
// So we can access a list.
let splitLine = (fun (line : string) -> Seq.toList (line.Split ','))
// Split this line into a list.
let plants = "turnip,carrot,lettuce"
let result = splitLine plants
// Display our results in a for-loop.
for value in result do
printfn "%A" value
Output
"turnip"
"carrot"
"lettuce"
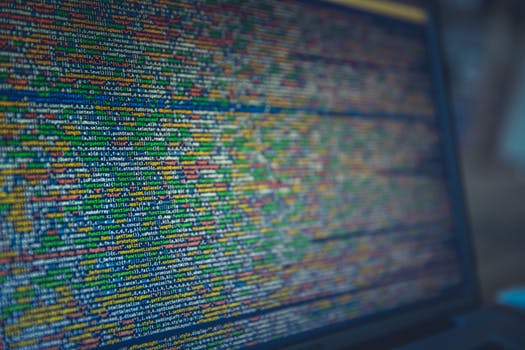
String separator. With Split() we can separate a string based on a substring delimiter. We create a string array containing all delimiters and pass it to Split.
RemoveEmptyEntries: This is part of the System namespace. With it, empty array elements are removed before Split returns.
Result: The items string has an empty string between two delimiters. But this is not part of the result.
F# program that uses Split, string separator, RemoveEmptyEntries
open System
let items = "keyboard; mouse; ; monitor"
// Call split with a string array as the first argument.
// ... This splits on a two-char sequence.
// Also remove empty array elements.
let result = items.Split([|"; "|], StringSplitOptions.RemoveEmptyEntries)
// Print all elements in the array with Seq.iter.
Seq.iter (fun x -> printfn "%A" x) result
Output
"keyboard"
"mouse"
"monitor"
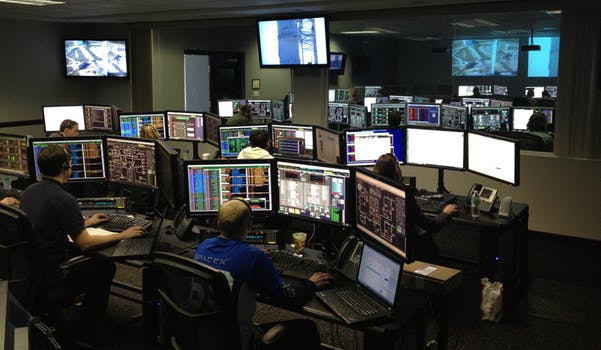
Multiple delimiters. Here we Split on two different character delimiters. We provide a char array to Split() as the first argument. We use a for-loop to print results.
F# program that uses Split, for-loop
let clothes = "pants.shoes:socks"
// Split on two different characters.
let result = clothes.Split([|'.'; ':'|])
// Print results with for-loop.
for r in result do
printfn "%s" r
Output
pants
shoes
socks
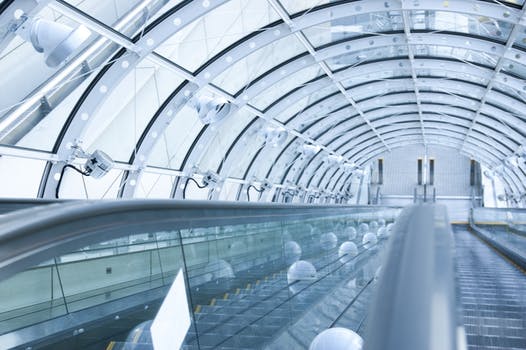
To summarize: F# adds no special splitting methods. But it allows easy access to the powerful .NET Framework string split method. With some conversion, we can split into a list.
Related Links:
- F# Files: open System.IO, use StreamReader
- F# Odd, Even Numbers
- F# Record Examples: Type, With Keywords
- F# Recursion Example: rec Keyword
- F# Option int Example: IsNone, IsSome and Value
- F# Array Examples
- F# dict: Dictionary Examples, Item and Keys
- F# IndexOf String Examples: LastIndexOf and Contains
- F# Replace String Examples
- F# Failwith: Exception Handling
- F# Type Example: member, get and set
- F# Match Keyword
- F# Remove Duplicates From List (Seq.distinct)
- F# If, elif and else Examples
- F# Printfn Examples: printf, Formats
- F# Math Operators: abs, floor and ceil
- F# Convert String, Int, Array and Seq
- F# Downcast and upcast Example
- F# ROT13 Cipher (String.map)
- F# String Examples: String.map
- F# Keywords
- F# Seq Examples: Seq.sum, Seq.where
- F# Measure Example: Units of Measurement
- F# Fun Keyword: Lambda Expressions, Functions
- F# Tuple Examples
- F# For and While Loop Examples: For To, For In
- F# Let Keyword: let mutable, Errors
- F# Sort List Examples: sortBy, Lambdas
- F# List Examples, String Lists
- F# Split Strings
Related Links
Adjectives
Ado
Ai
Android
Angular
Antonyms
Apache
Articles
Asp
Autocad
Automata
Aws
Azure
Basic
Binary
Bitcoin
Blockchain
C
Cassandra
Change
Coa
Computer
Control
Cpp
Create
Creating
C-Sharp
Cyber
Daa
Data
Dbms
Deletion
Devops
Difference
Discrete
Es6
Ethical
Examples
Features
Firebase
Flutter
Fs
Git
Go
Hbase
History
Hive
Hiveql
How
Html
Idioms
Insertion
Installing
Ios
Java
Joomla
Js
Kafka
Kali
Laravel
Logical
Machine
Matlab
Matrix
Mongodb
Mysql
One
Opencv
Oracle
Ordering
Os
Pandas
Php
Pig
Pl
Postgresql
Powershell
Prepositions
Program
Python
React
Ruby
Scala
Selecting
Selenium
Sentence
Seo
Sharepoint
Software
Spellings
Spotting
Spring
Sql
Sqlite
Sqoop
Svn
Swift
Synonyms
Talend
Testng
Types
Uml
Unity
Vbnet
Verbal
Webdriver
What
Wpf