<< Back to F#
F# Sort List Examples: sortBy, Lambdas
Sort a list with the List.sort function. Use sortBy with a fun to customize the sort order.Sort. A list has numbers: 10, 5, 7. They are not sorted. With a function call we can reorder them in ascending or descending order.
For more advanced sorts, we can provide a function to sortBy. This selects a key that is then sorted. Collections like arrays can be sorted with Seq.
First example. Here we introduce a string list containing the names of geometric shapes. We call List.sort with this list as the argument.
Result: The shapes are sorted in ascending order (as strings). A copy is sorted, so the original is untouched.
StringF# program that uses List.sort
let shapes = ["triangle"; "square"; "ellipse"; "rectangle"]
// Use List.sort to sort the string list.
// ... A sorted copy is returned.
let result = List.sort shapes
// Print both lists.
printfn "Unsorted: %A" shapes
printfn " Sorted: %A" result
Output
Unsorted: ["triangle"; "square"; "ellipse"; "rectangle"]
Sorted: ["ellipse"; "rectangle"; "square"; "triangle"]
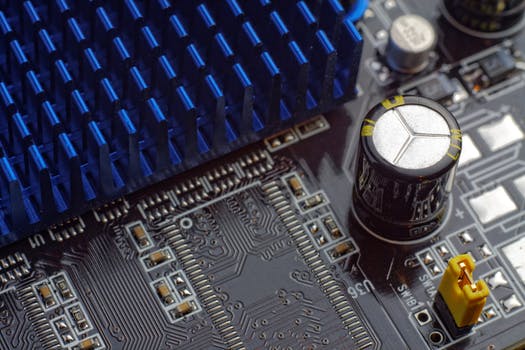
List.sortBy. This function is more advanced. It lets us select a key to sort for each element. The lambda we pass to sortBy must return a value—this is sorted.
Result: SortBy returns a copy of the List that is sorted. As with sort, the original is not changed.
F# program that uses List.sortBy, sorts strings by length
let values = ["aa"; "x"; "zzz"; "yy"; "eeee"]
// Sort the string list by length in ascending (low to high) order.
let result = List.sortBy (fun (x : string) -> x.Length) values
// Print our results.
List.iter(fun x -> printfn "%A" x) result
Output
"x"
"aa"
"yy"
"zzz"
"eeee"
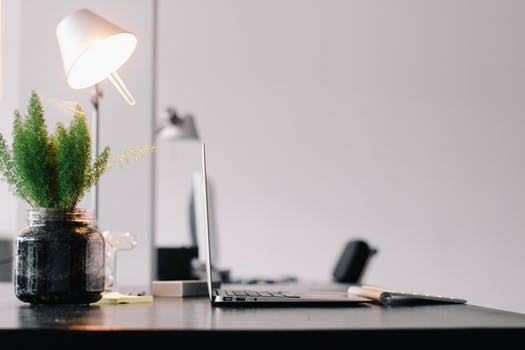
Array.sort, sortInPlace. An array in F# is not the same as a list—an array is a low-level region of memory with elements. We must use special Array functions to sort.
ArrayArray.sort: This returns a copied array that is sorted. It does not modify (mutate) the original array's data.
Array.sortInPlace: This rearranges the elements in an existing array. No new memory region is allocated.
F# program that uses Array.sort, sortInPlace
let offsets = [|10; 2; -2; 4; 40|]
let copy = Array.sort offsets
printfn "Array = %A" offsets
printfn "Copy = %A" copy
Array.sortInPlace offsets
printfn "After sortInPlace = %A" offsets
Output
Array = [|10; 2; -2; 4; 40|]
Copy = [|-2; 2; 4; 10; 40|]
After sortInPlace = [|-2; 2; 4; 10; 40|]
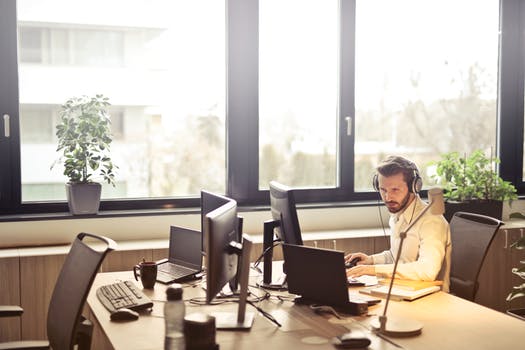
Seq.sort, pipeline. We can sort collections with Seq.sort. With Seq.ofList, we treat a list as a sequence. Then we can use functions like where and sort in a pipeline.
Here: We use "where" to only keep elements containing a lowercase "A." Then we sort them alphabetically, and convert back to a list.
F# program that uses Seq.sort
let animals = ["cat"; "bird"; "zebra"; "leopard"; "shark"]
// Act on list as a sequence.
// ... Use where to filter our list.
// Use Seq.sort to sort the sequence.
// Use Seq.toList to convert back to a list.
let sortResult =
Seq.ofList animals
|> Seq.where (fun x-> x.Contains "a")
|> Seq.sort
|> Seq.toList
// Print all our results.
List.iter (fun (x : string) -> printfn "%A" x) sortResult
Output
"cat"
"leopard"
"shark"
"zebra"
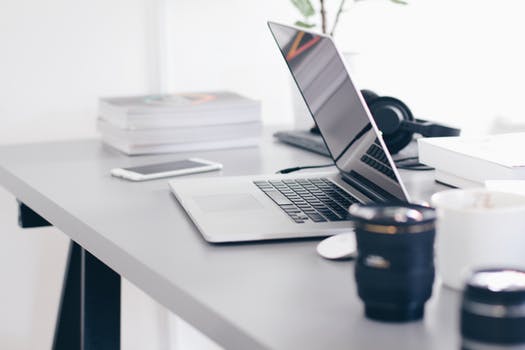
With this language, we have features that are well-suited to advanced sorting. We have lambdas and immutable collections (like lists). We invoke built-in sort methods.
Related Links:
- F# Files: open System.IO, use StreamReader
- F# Odd, Even Numbers
- F# Record Examples: Type, With Keywords
- F# Recursion Example: rec Keyword
- F# Option int Example: IsNone, IsSome and Value
- F# Array Examples
- F# dict: Dictionary Examples, Item and Keys
- F# IndexOf String Examples: LastIndexOf and Contains
- F# Replace String Examples
- F# Failwith: Exception Handling
- F# Type Example: member, get and set
- F# Match Keyword
- F# Remove Duplicates From List (Seq.distinct)
- F# If, elif and else Examples
- F# Printfn Examples: printf, Formats
- F# Math Operators: abs, floor and ceil
- F# Convert String, Int, Array and Seq
- F# Downcast and upcast Example
- F# ROT13 Cipher (String.map)
- F# String Examples: String.map
- F# Keywords
- F# Seq Examples: Seq.sum, Seq.where
- F# Measure Example: Units of Measurement
- F# Fun Keyword: Lambda Expressions, Functions
- F# Tuple Examples
- F# For and While Loop Examples: For To, For In
- F# Let Keyword: let mutable, Errors
- F# Sort List Examples: sortBy, Lambdas
- F# List Examples, String Lists
- F# Split Strings
Related Links
Adjectives
Ado
Ai
Android
Angular
Antonyms
Apache
Articles
Asp
Autocad
Automata
Aws
Azure
Basic
Binary
Bitcoin
Blockchain
C
Cassandra
Change
Coa
Computer
Control
Cpp
Create
Creating
C-Sharp
Cyber
Daa
Data
Dbms
Deletion
Devops
Difference
Discrete
Es6
Ethical
Examples
Features
Firebase
Flutter
Fs
Git
Go
Hbase
History
Hive
Hiveql
How
Html
Idioms
Insertion
Installing
Ios
Java
Joomla
Js
Kafka
Kali
Laravel
Logical
Machine
Matlab
Matrix
Mongodb
Mysql
One
Opencv
Oracle
Ordering
Os
Pandas
Php
Pig
Pl
Postgresql
Powershell
Prepositions
Program
Python
React
Ruby
Scala
Selecting
Selenium
Sentence
Seo
Sharepoint
Software
Spellings
Spotting
Spring
Sql
Sqlite
Sqoop
Svn
Swift
Synonyms
Talend
Testng
Types
Uml
Unity
Vbnet
Verbal
Webdriver
What
Wpf