<< Back to SCALA
Scala Convert: String to Int, List and Array
Convert string values to Ints. Change Lists and Maps to strings.Convert. A String contains a number like 20. But these are characters. To get an Int we must call a parsing method. We must convert the string.
Conversion and parsing. Scala provides many built-in methods to convert Strings, Ints, Lists and Arrays. We use these for the clearest, smallest code.
ToString. Here we convert an Int to a string. And then we convert that string into an Int again. We use the toString def (part of scala.Any) and the toInt def (part of StringLike).
Scala program that uses toString, toInt
val number = 123
// Convert Int to String.
val result = number.toString()
if (result == "123")
println(result)
// Convert String back to Int.
val original = result.toInt
if (original == 123)
println(original)
Output
123
123
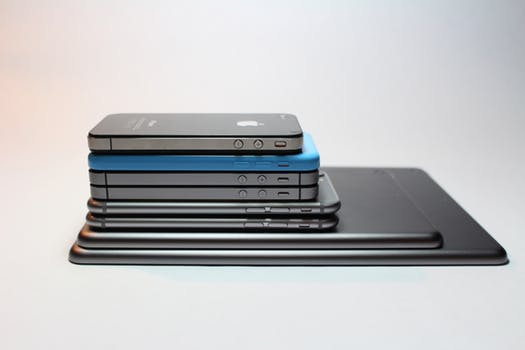
ToArray. In Scala we usually use Lists to store and manipulate data. But Arrays are also available—these are somewhat harder to work with.
Here: We use toArray to convert the List to an Array. We print the values of the Array with a foreach call.
Scala program that converts List to array
val colors = List("blue", "yellow", "red")
// Convert list to array.
val result = colors.toArray
// Print list.
println(colors)
// Print length and elements of the array.
println(result.length)
result.foreach(println(_))
Output
List(blue, yellow, red)
3
blue
yellow
red
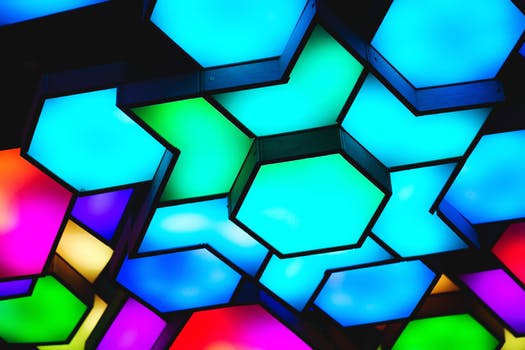
List to string, StringBuilder. With addString (a method on List) we can add all list elements to a StringBuilder. The result by default has no separators.
StringBuilderHowever: We can specify a separator string as the second argument to addString. This is like a join method on list.
Scala program that converts list to string
val animals = List("cat", "bird", "fish")
// Create a StringBuilder to store all list element values.
val builder1 = StringBuilder.newBuilder
// Call addString to add all strings with no separator.
animals.addString(builder1)
val result1 = builder1.toString()
println(result1)
// Use a separator with addString.
// ... Converts a list to a string.
val builder2 = StringBuilder.newBuilder
animals.addString(builder2, "; ")
val result2 = builder2.toString()
println(result2)
Output
catbirdfish
cat; bird; fish
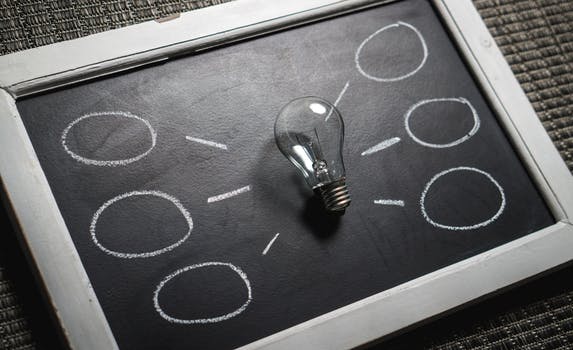
Range, list. In Scala we find a toList function that can convert many iterable collections into a list. This is part of scala.collection.TraversableOnce.
Here: We convert a range (specified with the to and by methods) into a list, with the toList function.
RangeScala program that converts range to list
// Create a range from 10 to 20.
// ... Step is 3 after each element.
val range = 10.to(20).by(3)
println(range)
// Use toList to convert a range to a list.
val list = range.toList
println(list)
Output
Range(10, 13, 16, 19)
List(10, 13, 16, 19)
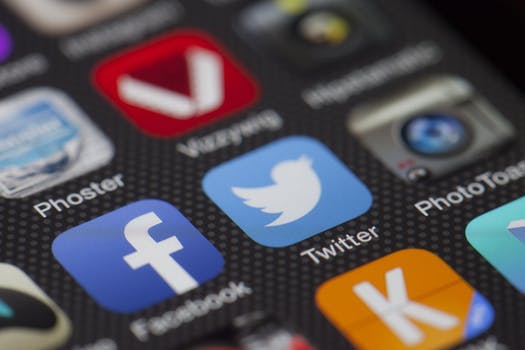
Vector, List. The Vector has different performance characteristics than a List: it can be added to and updated faster. We sometimes have a Vector, but need a List.
ListVectorTip: With toList we can convert a Vector to a List. Here we have a Vector with 2 elements, and get a List with those same 2 elements.
Note: Excess conversions of the Vector and List should be avoided. It is possible to directly use the Vector for storing elements.
Scala program that converts Vector to List
// An empty vector.
val vector = scala.collection.immutable.Vector.empty
println(vector)
// Add 2 new values at the end.
val vector2 = vector :+ 1 :+ 5
println(vector2)
// Convert Vector to a List.
val result = vector2.toList
println(result)
Output
Vector()
Vector(1, 5)
List(1, 5)
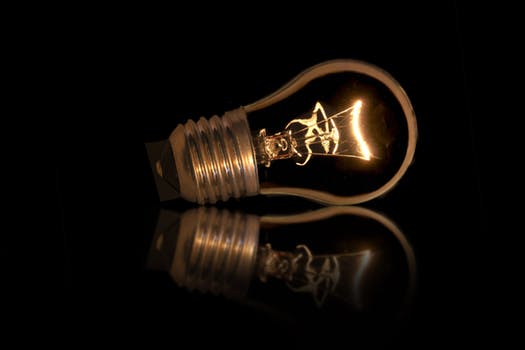
StringLike. In Scala we find the StringLike type. This helps us convert and modify strings. As in Java, converting strings makes copies of existing strings.
String: StringLike
Some notes. Usually it is best to use the data type that is closest to the meaning of a value. So a string that means 100 is less effective than an Int of 100.
Related Links:
- Scala Convert: String to Int, List and Array
- Scala Odd and Even Numbers
- Scala Regex, R Examples: findFirstMatchIn
- Scala Interview Questions (2021)
- Scala Class Examples: Trait Keyword
- Scala indexOf: Lists and Strings
- Scala Int Examples: Max and Min
- Scala 2D List, Array Examples: Array.ofDim
- Scala Def: return Unit and Lambdas
- Scala Initialize List: List.newBuilder, List.empty
- Scala Option: None, get and getOrElse
- Scala Exception Handling: Try, Catch and Throw
- Scala Map Examples
- Scala String Examples: StringLike
- Scala Match and Case Examples
- Scala Remove Duplicates From List: Distinct Example
- Scala If, Else Example (Match Case If)
- Scala Console: println, printf and readLine
- Scala Strip Strings: stripLineEnd and stripMargin
- Scala Vector Examples
- Scala Var and Val (Variables and Constants)
- Learn Scala Tutorial
- Scala Keywords
- Scala format and formatted Strings
- Scala List Examples
- Scala StringBuilder Examples
- Scala Set Examples: contains, intersect
- Scala Tuple Examples (Return Multiple Values)
- Scala For to, until, while Loop Examples
- Scala Slice: Substring, List Slice
- Scala Sorted List Examples: Ordering.Int
- Scala Split String Examples
- Scala Array Examples: Apply and Update
- Scala Range Examples: Start, End and Step
Related Links
Adjectives
Ado
Ai
Android
Angular
Antonyms
Apache
Articles
Asp
Autocad
Automata
Aws
Azure
Basic
Binary
Bitcoin
Blockchain
C
Cassandra
Change
Coa
Computer
Control
Cpp
Create
Creating
C-Sharp
Cyber
Daa
Data
Dbms
Deletion
Devops
Difference
Discrete
Es6
Ethical
Examples
Features
Firebase
Flutter
Fs
Git
Go
Hbase
History
Hive
Hiveql
How
Html
Idioms
Insertion
Installing
Ios
Java
Joomla
Js
Kafka
Kali
Laravel
Logical
Machine
Matlab
Matrix
Mongodb
Mysql
One
Opencv
Oracle
Ordering
Os
Pandas
Php
Pig
Pl
Postgresql
Powershell
Prepositions
Program
Python
React
Ruby
Scala
Selecting
Selenium
Sentence
Seo
Sharepoint
Software
Spellings
Spotting
Spring
Sql
Sqlite
Sqoop
Svn
Swift
Synonyms
Talend
Testng
Types
Uml
Unity
Vbnet
Verbal
Webdriver
What
Wpf