<< Back to SCALA
Scala Range Examples: Start, End and Step
Create a range with the to method. Use the by function to specify a step.Range. Numbers are in a range. We have 10, 11, 12. These start at 10, end at 12, and have a step of 1. In Scala these numbers can be specified with built-in functions.
By collecting numbers together, we can use functions (like sum) that act on a group of numbers. This is a functional programming approach. It may lead to clearer code.
A simple range. This example first creates a range from 10 to 13 inclusive with the to function. It prints the range. Then it uses some properties of the range.
IsInclusive: This returns true. An inclusive range includes the final number (the end) of the range. To() creates inclusive ranges.
IsEmpty: Returns false in this program because the range has more than zero elements. An empty range has 0 numbers.
Start, End, Step: A range's start is its first number. Its end is its last. The step is the amount each number is incremented.
IntScala program that creates range
// Create range from 10 to 13 inclusive.
val range = 10.to(13)
println(range)
// Print some properties of the range.
println("IsInclusive = " + range.isInclusive)
println("IsEmpty = " + range.isEmpty)
println("Start = " + range.start)
println("End = " + range.end)
println("Step = " + range.step)
// Sum all elements in the range.
val total = range.sum
println("Sum = " + total)
Output
Range(10, 11, 12, 13)
IsInclusive = true
IsEmpty = false
Start = 10
End = 13
Step = 1
Sum = 46
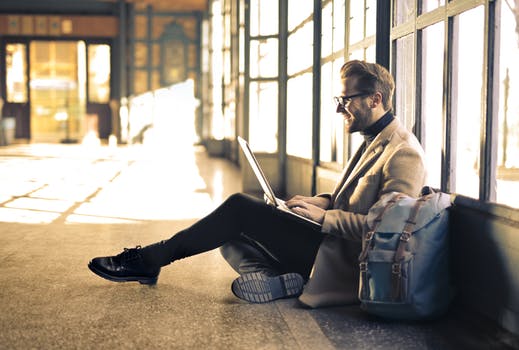
Steps. Let us continue with ranges. This program uses to() and until to build ranges. But then it adds another feature, the by() function, to specify a step on these ranges.
printlnTip: To() creates an inclusive range (the last number is included). And "until" creates an exclusive range (the last number is omitted).
Scala program that creates ranges with steps
// Create a range of even numbers.
// ... Use a step of 2 with the by function.
val evens = 10.to(20).by(2)
println(evens)
// Use until to create a range that omits the final number (exclusive end).
// ... Use by to specify a negative step.
// ... The range is descending from high to low.
val descending = 20.until(0).by(-5)
println(descending)
Output
Range(10, 12, 14, 16, 18, 20)
Range(20, 15, 10, 5)
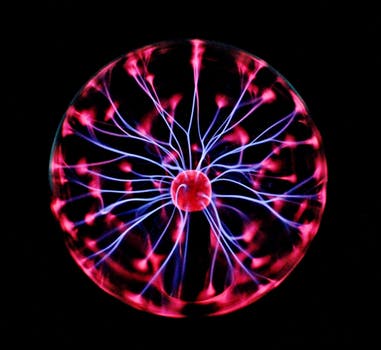
Create range. A range can be created directly with the Range type. This is sometimes a clearer way to create a range. With this syntax, the "end" is exclusive (it is not part of the range).
Tip: This syntax form has the same effect as the "until" and "by" functions on Ints.
Scala program that creates ranges
// Create a range of 10 until 13 (end is exclusive).
val range1 = Range(10, 13)
println(range1)
// Create a range of 10 until 20 with step of 3.
val range2 = Range(10, 20, 3)
println(range2)
Output
Range(10, 11, 12)
Range(10, 13, 16, 19)
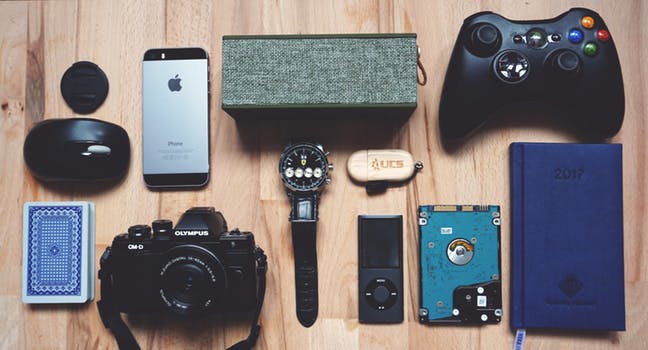
Contains. Is a value present in a range? With the contains() function, we determine if the argument exists in a range. It returns true or false (a Boolean).
Scala program that uses contains
val testRange = 10.to(12)
// This value is contained in the range.
if (testRange.contains(10)) {
println(true)
}
// This value is not present.
if (!testRange.contains(14)) {
println(false)
}
Output
true
false
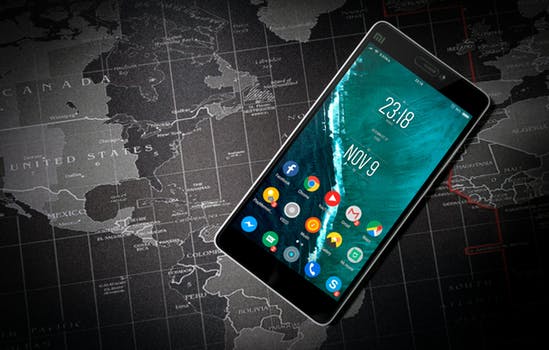
Ranges are helpful. They describe series of numbers. This makes calling functions on sequences easier and more intuitive. With methods like to, until and by we form simple ranges.
Related Links:
- Scala Convert: String to Int, List and Array
- Scala Odd and Even Numbers
- Scala Regex, R Examples: findFirstMatchIn
- Scala Interview Questions (2021)
- Scala Class Examples: Trait Keyword
- Scala indexOf: Lists and Strings
- Scala Int Examples: Max and Min
- Scala 2D List, Array Examples: Array.ofDim
- Scala Def: return Unit and Lambdas
- Scala Initialize List: List.newBuilder, List.empty
- Scala Option: None, get and getOrElse
- Scala Exception Handling: Try, Catch and Throw
- Scala Map Examples
- Scala String Examples: StringLike
- Scala Match and Case Examples
- Scala Remove Duplicates From List: Distinct Example
- Scala If, Else Example (Match Case If)
- Scala Console: println, printf and readLine
- Scala Strip Strings: stripLineEnd and stripMargin
- Scala Vector Examples
- Scala Var and Val (Variables and Constants)
- Learn Scala Tutorial
- Scala Keywords
- Scala format and formatted Strings
- Scala List Examples
- Scala StringBuilder Examples
- Scala Set Examples: contains, intersect
- Scala Tuple Examples (Return Multiple Values)
- Scala For to, until, while Loop Examples
- Scala Slice: Substring, List Slice
- Scala Sorted List Examples: Ordering.Int
- Scala Split String Examples
- Scala Array Examples: Apply and Update
- Scala Range Examples: Start, End and Step
Related Links
Adjectives
Ado
Ai
Android
Angular
Antonyms
Apache
Articles
Asp
Autocad
Automata
Aws
Azure
Basic
Binary
Bitcoin
Blockchain
C
Cassandra
Change
Coa
Computer
Control
Cpp
Create
Creating
C-Sharp
Cyber
Daa
Data
Dbms
Deletion
Devops
Difference
Discrete
Es6
Ethical
Examples
Features
Firebase
Flutter
Fs
Git
Go
Hbase
History
Hive
Hiveql
How
Html
Idioms
Insertion
Installing
Ios
Java
Joomla
Js
Kafka
Kali
Laravel
Logical
Machine
Matlab
Matrix
Mongodb
Mysql
One
Opencv
Oracle
Ordering
Os
Pandas
Php
Pig
Pl
Postgresql
Powershell
Prepositions
Program
Python
React
Ruby
Scala
Selecting
Selenium
Sentence
Seo
Sharepoint
Software
Spellings
Spotting
Spring
Sql
Sqlite
Sqoop
Svn
Swift
Synonyms
Talend
Testng
Types
Uml
Unity
Vbnet
Verbal
Webdriver
What
Wpf