<< Back to SCALA
Scala Tuple Examples (Return Multiple Values)
Use tuples to store multiple variables together. Return a tuple from a function.Tuple. In a tuple we can combine 2 values in a single unit. We combine "cat" and 10 (its weight) with some simple syntax. The tuple is a single variable.
With tuples, we build abstractions over related values. This helps simplify many parts of our Scala programs. A function can return more than 1 value in a tuple.
First example. Here we create 2 tuples, each with 2 items. The tuples have a String and Int. We can access the first and second items in them.
Underscore: We can access the first and second items from a tuple with the "_1" and "_2" syntax.
Note: The first item in a tuple is at index 1, not index 0, and we must use "_1" to get it.
Scala program that uses tuples
// Create and print two tuples.
val identity1 = ("ABC", 10)
val identity2 = ("DEF", 20)
println(identity1)
println(identity2)
// Get first and second items from a tuple.
val first = identity1._1
val second = identity1._2
println(first)
println(second)
Output
(ABC,10)
(DEF,20)
ABC
10
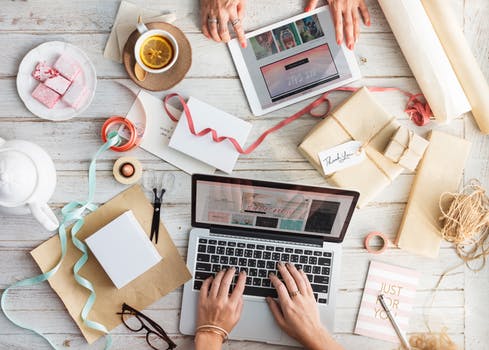
Return multiple values. Often in programs we want to return multiple values from a function. Parameters, classes, or tuples may be used. Here we return a tuple from function.
Note: The upperName def receives a name String and returns a two-item tuple containing the uppercase name and the original name.
DefScala program that returns tuple from function
// This def returns a two-item tuple.
// ... It uppercases the argument String.
def upperName(name: String): (String, String) = (name.toUpperCase(), name)
// Call upperName function with String.
val name = "scala"
val result = upperName(name)
println(result)
Output
(SCALA,scala)
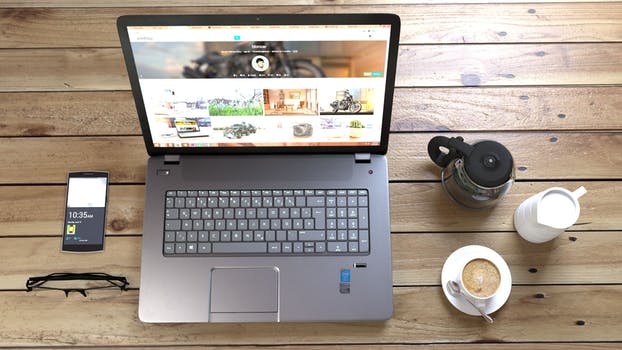
Unpack. We can unpack a tuple in an assignment statement. Here the function returns a tuple with two items in it. We assign the values number1 and number2 to those items.
Tip: This syntax immediately unpacks a tuple. This can make a program simpler to read.
Scala program that unpacks tuple
// Return a tuple.
def twoNumbers(x: Int): (Int, Int) = (x * 2, x * 4)
// Unpack the tuple returned by the function.
val (number1, number2) = twoNumbers(3)
println(number1)
println(number2)
Output
6
12
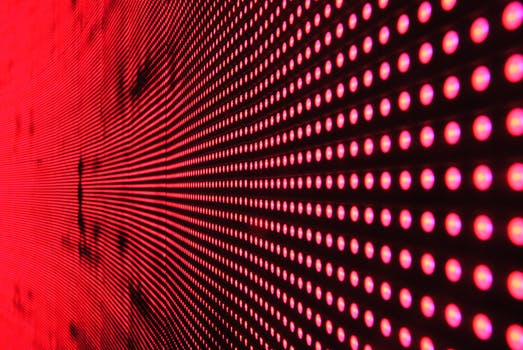
Match. We can use a tuple as the argument of a match construct. We specify values within match cases to require specific values in a tuple.
MatchHere: The test() method receives a String, Int tuple. If the first item is "bird" the first case is matched.
Scala program that uses tuple with match
def test(animal: (String, Int)) = {
animal match {
case ("bird", size) =>
println("Bird weighs", size)
case (animal, size) =>
println("Other animal", animal, size)
}
}
// Call test method with tuple argument.
val animal = ("bird", 20)
test(animal)
val animal2 = ("cat", 300)
test(animal2)
Output
(Bird weighs,20)
(Other animal,cat,300)
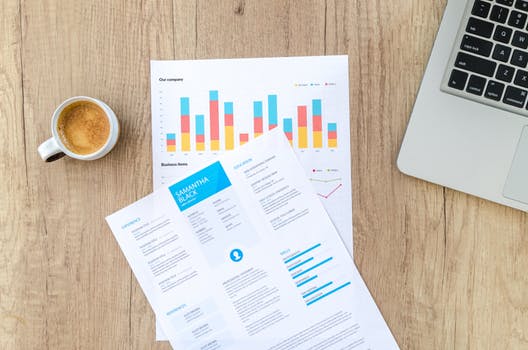
Tuple list. With a list of tuples we can make a 2D array or a collection of complex elements. Here we use 3 items in each tuple and access them in a for-loop.
ListScala program that uses list of tuples
// Create list of tuples.
val animals = List(("cat", 10, "yellow"), ("bird", 1, "blue"))
// Loop over tuples.
for (value <- animals) {
println(value._1 + " is " + value._3)
println("... weighs " + value._2)
}
Output
cat is yellow
... weighs 10
bird is blue
... weighs 1
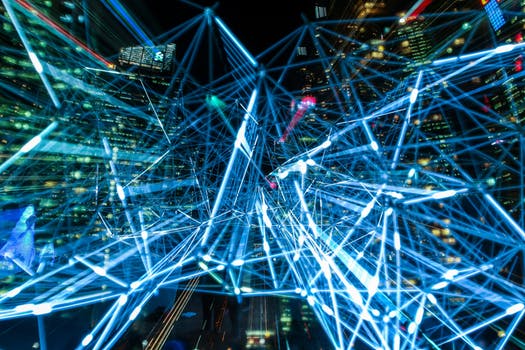
Tuples are common in Scala. They have a simple syntax form, so we can use them with minimal effort. They combine data together, enabling manipulation of data units.
Related Links:
- Scala Convert: String to Int, List and Array
- Scala Odd and Even Numbers
- Scala Regex, R Examples: findFirstMatchIn
- Scala Interview Questions (2021)
- Scala Class Examples: Trait Keyword
- Scala indexOf: Lists and Strings
- Scala Int Examples: Max and Min
- Scala 2D List, Array Examples: Array.ofDim
- Scala Def: return Unit and Lambdas
- Scala Initialize List: List.newBuilder, List.empty
- Scala Option: None, get and getOrElse
- Scala Exception Handling: Try, Catch and Throw
- Scala Map Examples
- Scala String Examples: StringLike
- Scala Match and Case Examples
- Scala Remove Duplicates From List: Distinct Example
- Scala If, Else Example (Match Case If)
- Scala Console: println, printf and readLine
- Scala Strip Strings: stripLineEnd and stripMargin
- Scala Vector Examples
- Scala Var and Val (Variables and Constants)
- Learn Scala Tutorial
- Scala Keywords
- Scala format and formatted Strings
- Scala List Examples
- Scala StringBuilder Examples
- Scala Set Examples: contains, intersect
- Scala Tuple Examples (Return Multiple Values)
- Scala For to, until, while Loop Examples
- Scala Slice: Substring, List Slice
- Scala Sorted List Examples: Ordering.Int
- Scala Split String Examples
- Scala Array Examples: Apply and Update
- Scala Range Examples: Start, End and Step
Related Links
Adjectives
Ado
Ai
Android
Angular
Antonyms
Apache
Articles
Asp
Autocad
Automata
Aws
Azure
Basic
Binary
Bitcoin
Blockchain
C
Cassandra
Change
Coa
Computer
Control
Cpp
Create
Creating
C-Sharp
Cyber
Daa
Data
Dbms
Deletion
Devops
Difference
Discrete
Es6
Ethical
Examples
Features
Firebase
Flutter
Fs
Git
Go
Hbase
History
Hive
Hiveql
How
Html
Idioms
Insertion
Installing
Ios
Java
Joomla
Js
Kafka
Kali
Laravel
Logical
Machine
Matlab
Matrix
Mongodb
Mysql
One
Opencv
Oracle
Ordering
Os
Pandas
Php
Pig
Pl
Postgresql
Powershell
Prepositions
Program
Python
React
Ruby
Scala
Selecting
Selenium
Sentence
Seo
Sharepoint
Software
Spellings
Spotting
Spring
Sql
Sqlite
Sqoop
Svn
Swift
Synonyms
Talend
Testng
Types
Uml
Unity
Vbnet
Verbal
Webdriver
What
Wpf