<< Back to SCALA
Scala Exception Handling: Try, Catch and Throw
Handle exceptions with try, catch and throw. Use case to match exception types.Try, exceptions. A program fails. A stack trace appears. But nothing further happens in the program. Exceptions happen often in development.
With exception handling, we trap and process these exceptions. Our programs can continue as though they were never stopped. In Scala we use keywords: try, catch, throw.
An example. This program uses a try-construct. Its division expression will cause an ArithmeticException to occur. The catch block is entered.
Scala program that handles exceptions
try {
// Try to perform an impossible operation.
val mistake = 1 / 0
} catch {
// Handle exceptions.
case npe: NullPointerException =>
println("Result NPE: " + npe)
case ae: ArithmeticException =>
println("Result AE: " + ae)
}
Output
Result AE: java.lang.ArithmeticException: / by zero
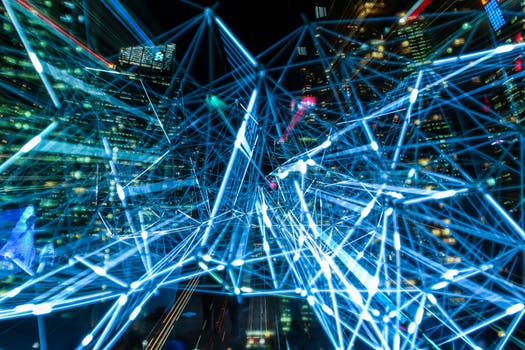
Throw, Throwable. Here we use the throw keyword to create an exception. With the case, we catch all Throwables—so all exceptions are caught here.
Tip: In Scala we must specify the Throwable type for the case to avoid a compiler warning.
Scala program that uses throw, catches Throwable
try {
// Throw an Exception.
throw new Exception("Failure")
} catch {
// Catch all Throwable exceptions.
case _: Throwable => println("An exception was thrown")
}
Output
An exception was thrown
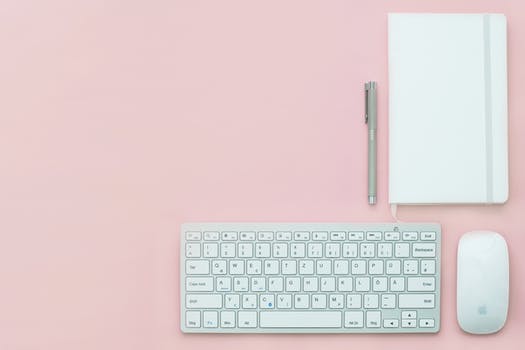
Null, NullPointerException. The NullPointerException is common. It is caused when we try to access a field or method on a null variable. Here we see a Scala stack trace.
Scala program that causes NullPointerException
// Print length of this string.
var x = "cat"
println(x.length)
// Reassign string to null.
// ... Now a NullPointerException is caused.
x = null
println(x.length)
Output
3
java.lang.NullPointerException
at Main$$anon$1.<init>(program.scala:10)
at Main$.main(program.scala:1)
at Main.main(program.scala)
at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method)
at sun.reflect.NativeMethodAccessorImpl.invoke(Unknown Source)
at sun.reflect.DelegatingMethodAccessorImpl.invoke(Unknown Source)
at java.lang.reflect.Method.invoke(Unknown Source)...
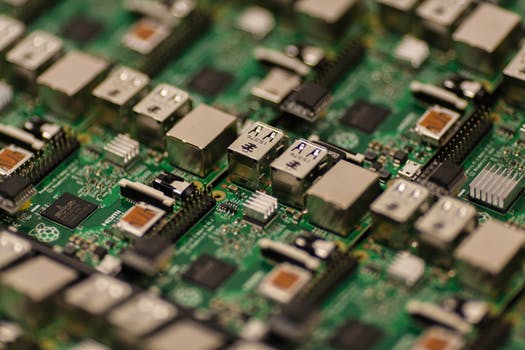
A review. With the try, catch and throw keywords, we implement basic exception handling in Scala. With cases, we match specific kinds of Throwable exceptions, like ArithmeticException.
Related Links:
- Scala Convert: String to Int, List and Array
- Scala Odd and Even Numbers
- Scala Regex, R Examples: findFirstMatchIn
- Scala Interview Questions (2021)
- Scala Class Examples: Trait Keyword
- Scala indexOf: Lists and Strings
- Scala Int Examples: Max and Min
- Scala 2D List, Array Examples: Array.ofDim
- Scala Def: return Unit and Lambdas
- Scala Initialize List: List.newBuilder, List.empty
- Scala Option: None, get and getOrElse
- Scala Exception Handling: Try, Catch and Throw
- Scala Map Examples
- Scala String Examples: StringLike
- Scala Match and Case Examples
- Scala Remove Duplicates From List: Distinct Example
- Scala If, Else Example (Match Case If)
- Scala Console: println, printf and readLine
- Scala Strip Strings: stripLineEnd and stripMargin
- Scala Vector Examples
- Scala Var and Val (Variables and Constants)
- Learn Scala Tutorial
- Scala Keywords
- Scala format and formatted Strings
- Scala List Examples
- Scala StringBuilder Examples
- Scala Set Examples: contains, intersect
- Scala Tuple Examples (Return Multiple Values)
- Scala For to, until, while Loop Examples
- Scala Slice: Substring, List Slice
- Scala Sorted List Examples: Ordering.Int
- Scala Split String Examples
- Scala Array Examples: Apply and Update
- Scala Range Examples: Start, End and Step
Related Links
Adjectives
Ado
Ai
Android
Angular
Antonyms
Apache
Articles
Asp
Autocad
Automata
Aws
Azure
Basic
Binary
Bitcoin
Blockchain
C
Cassandra
Change
Coa
Computer
Control
Cpp
Create
Creating
C-Sharp
Cyber
Daa
Data
Dbms
Deletion
Devops
Difference
Discrete
Es6
Ethical
Examples
Features
Firebase
Flutter
Fs
Git
Go
Hbase
History
Hive
Hiveql
How
Html
Idioms
Insertion
Installing
Ios
Java
Joomla
Js
Kafka
Kali
Laravel
Logical
Machine
Matlab
Matrix
Mongodb
Mysql
One
Opencv
Oracle
Ordering
Os
Pandas
Php
Pig
Pl
Postgresql
Powershell
Prepositions
Program
Python
React
Ruby
Scala
Selecting
Selenium
Sentence
Seo
Sharepoint
Software
Spellings
Spotting
Spring
Sql
Sqlite
Sqoop
Svn
Swift
Synonyms
Talend
Testng
Types
Uml
Unity
Vbnet
Verbal
Webdriver
What
Wpf