<< Back to SCALA
Scala Slice: Substring, List Slice
Use the slice function to create substrings and get slices of lists.Slice, substring. A slice is part of a list. It may only contain the elements at positions 4 through 8. A substring is a string slice. It might only contain some characters.
With StringOps in Scala, we apply slice to strings. We take substrings with a start index, and a last index (not a length). List uses the same syntax.
Substring example. In Scala we can call the Substring method as in Java. But a string can also be sliced with the slice function. Some implicit conversions make this possible.
First argument: The first character index where we want to start a substring. To get the first character, we use 0.
Second argument: The last index of our substring. This is not a character count or length—it should be larger than the first argument.
Scala program that uses string slice
val phrase = "a soft orange cat"
// Use slice on a string from scala.collection.immutable.StringOps.
// ... Use first index, second index.
val result = phrase.slice(2, 2 + 4)
println("[" + result + "]")
Output
[soft]
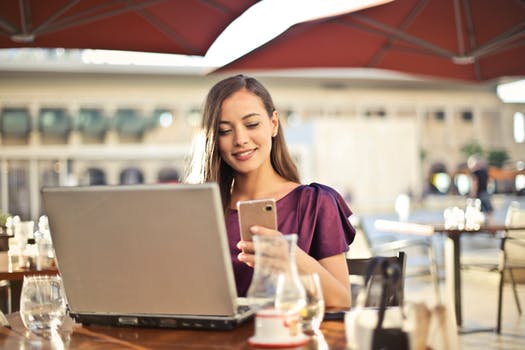
List example. A list is like a string, but has other types of elements like Ints or Doubles. We can use slice on a list. We use a first and a last index.
ListHere: We begin a slice at the second element (index 1, value 3.5) and continue until the third index.
Next: We begin at element 3 (index 2, value 10.3) and continue until the end of the list (with the list's length).
Scala program that uses list slice
val points = List(1.5, 3.5, 10.3, 11.3)
println(points)
// Get slice of list.
val slice1 = points.slice(1, 3)
println(slice1)
// Get slice until end of list.
val slice2 = points.slice(2, points.length)
println(slice2)
Output
List(1.5, 3.5, 10.3, 11.3)
List(3.5, 10.3)
List(10.3, 11.3)
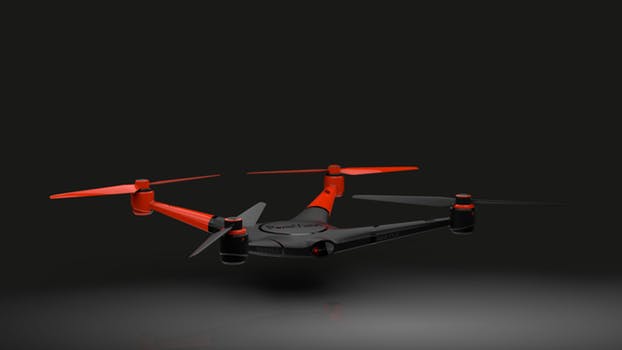
String slice, char. We can access single chars from a string with an index. But with slice, we can get one-char strings (strings of length 1). Strings are sometimes more useful than chars.
Tip: The Scala compiler helpfully warns us when we compare a string slice (a substring) to a char.
Scala program that accesses chars, slices from string
val letters = "scala"
// This is a char.
val result1 = letters(0)
// This is a string.
val result2 = letters.slice(0, 1)
println(result1)
println(result2)
// Cannot compare a char and a string.
// ... This always returns false.
println(result1 == result2)
Output
C:\programs\program.scala:13: warning:
comparing values of types Char and String using '==' will always yield false
println(result1 == result2)
^
one warning found
s
s
false
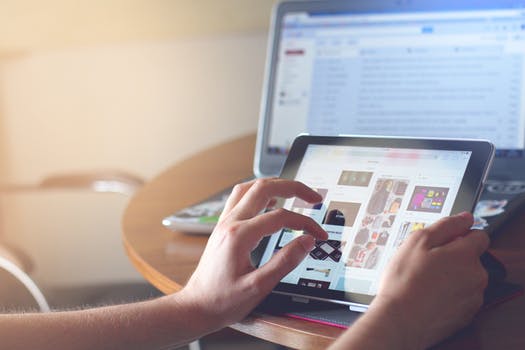
Slice versus substring. The slice() and substring methods receive the same arguments. And they return the same results. The returned string parts are equal in this program.
Scala program that uses slice, substring
val example = "California"
// Slice and substring have the same results on a string.
val firstPart = example.slice(1, 4)
val firstPart2 = example.substring(1, 4)
// Print results.
println(firstPart, firstPart2)
if (firstPart == firstPart2) {
println(true)
}
Output
(ali,ali)
true
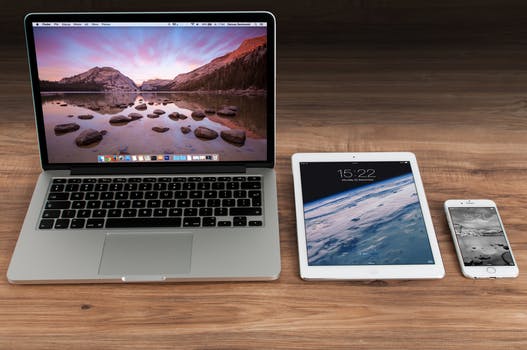
Performance. I tested the slice method and the substring method on a string. I found the performance was about the same. So we can use either one for top performance.
Version 1: This version of the code uses the slice() method on a string and tests its result.
Version 2: Here we use the substring() method on a string. This code does the same thing as version 1.
Opinion: The word "substring" makes it clearer what we are trying to do. For this reason is might be a better choice.
Scala program that benchmarks slice, substring
val data = "abcdef"
// Warm up the JIT.
val test = data.slice(3, 5)
val test2 = data.substring(3, 5)
val t1 = System.currentTimeMillis()
// Version 1: use slice.
for (i <- 0 to 100000000) {
val part = data.slice(3, 5)
if (part != "de")
println(false)
}
val t2 = System.currentTimeMillis()
// Version 2: use substring.
for (i <- 0 to 100000000) {
val part = data.substring(3, 5)
if (part != "de")
println(false)
}
val t3 = System.currentTimeMillis()
// Print times.
println(t2 - t1)
println(t3 - t2)
Output
969 ms, slice
967 ms, substring
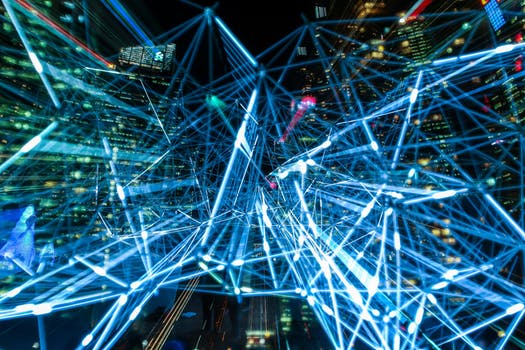
A summary. With slice we remove elements from a collection. We specify the elements we want to keep—the rest vanish. An immutable, new collection is returned.
Related Links:
- Scala Convert: String to Int, List and Array
- Scala Odd and Even Numbers
- Scala Regex, R Examples: findFirstMatchIn
- Scala Interview Questions (2021)
- Scala Class Examples: Trait Keyword
- Scala indexOf: Lists and Strings
- Scala Int Examples: Max and Min
- Scala 2D List, Array Examples: Array.ofDim
- Scala Def: return Unit and Lambdas
- Scala Initialize List: List.newBuilder, List.empty
- Scala Option: None, get and getOrElse
- Scala Exception Handling: Try, Catch and Throw
- Scala Map Examples
- Scala String Examples: StringLike
- Scala Match and Case Examples
- Scala Remove Duplicates From List: Distinct Example
- Scala If, Else Example (Match Case If)
- Scala Console: println, printf and readLine
- Scala Strip Strings: stripLineEnd and stripMargin
- Scala Vector Examples
- Scala Var and Val (Variables and Constants)
- Learn Scala Tutorial
- Scala Keywords
- Scala format and formatted Strings
- Scala List Examples
- Scala StringBuilder Examples
- Scala Set Examples: contains, intersect
- Scala Tuple Examples (Return Multiple Values)
- Scala For to, until, while Loop Examples
- Scala Slice: Substring, List Slice
- Scala Sorted List Examples: Ordering.Int
- Scala Split String Examples
- Scala Array Examples: Apply and Update
- Scala Range Examples: Start, End and Step
Related Links
Adjectives
Ado
Ai
Android
Angular
Antonyms
Apache
Articles
Asp
Autocad
Automata
Aws
Azure
Basic
Binary
Bitcoin
Blockchain
C
Cassandra
Change
Coa
Computer
Control
Cpp
Create
Creating
C-Sharp
Cyber
Daa
Data
Dbms
Deletion
Devops
Difference
Discrete
Es6
Ethical
Examples
Features
Firebase
Flutter
Fs
Git
Go
Hbase
History
Hive
Hiveql
How
Html
Idioms
Insertion
Installing
Ios
Java
Joomla
Js
Kafka
Kali
Laravel
Logical
Machine
Matlab
Matrix
Mongodb
Mysql
One
Opencv
Oracle
Ordering
Os
Pandas
Php
Pig
Pl
Postgresql
Powershell
Prepositions
Program
Python
React
Ruby
Scala
Selecting
Selenium
Sentence
Seo
Sharepoint
Software
Spellings
Spotting
Spring
Sql
Sqlite
Sqoop
Svn
Swift
Synonyms
Talend
Testng
Types
Uml
Unity
Vbnet
Verbal
Webdriver
What
Wpf