<< Back to SCALA
Scala For to, until, while Loop Examples
Use the for-loop on a List. Loop over a range of values with the for-to syntax.For loops. A loop always looks to the future. It proceeds in order, 1 then 2 and 3. With functions we can apply logic to ranges all at once. But "for" is often simpler.
With for, we can enumerate a collection like a list. We can use the "to" method to advance through a range of numbers. We must choose the best loop.
For example. Let us begin with a for-loop that enumerates a list. We create a list with 3 strings in it. Then we use the for-each loop on the list.
Tip: The syntax for a for-each loop in Scala is similar to that for Java. The language handles most of the iteration for us.
Scala program that uses for on list
// A list with 3 elements.
val languages = List("Java", "Scala", "F#")
// Use a for-each loop over all the elements in the list.
// ... Print the strings.
for (language <- languages)
println(language)
Output
Java
Scala
F#
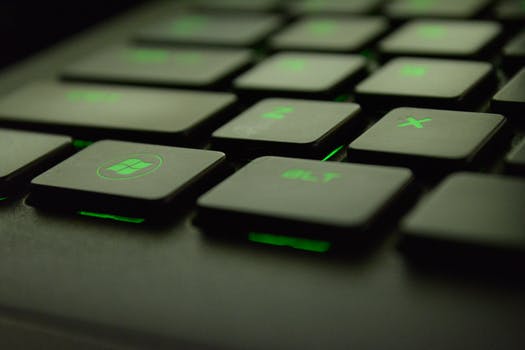
For to. Here we introduce a for-to loop. We use the to-keyword to specify to what number the loop advances. Once an iteration is past 5, the loop here terminates.
Scala program that uses for-to loop
// Use a for-to loop to iterate over a range of numbers.
// ... Start at 0 and continue until 5.
for (i <- 0 to 5)
println(i)
Output
0
1
2
3
4
5
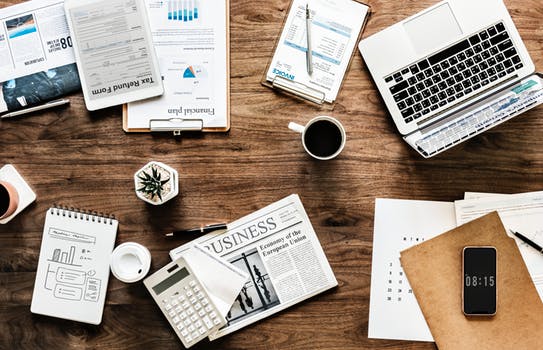
For until. With a for-loop we can also use the "until" keyword. To and until are methods that return an enumeration of numbers. Until() stops one number before the final number.
Scala program that uses for-until
// With until, the final number is not included.
// ... It is an exclusive bound.
for (i <- 100 until 105) {
println(i)
}
Output
100
101
102
103
104
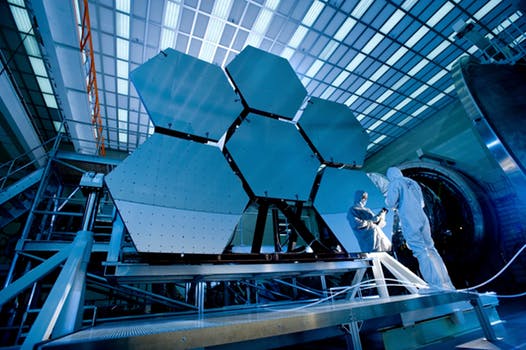
For to, until syntax. Sometimes a program is clearer to read (or understand) with more exact syntax. Scala's syntax is flexible. We can omit, or add, parentheses in for-to and until loops.
Scala program that shows for-to, until syntax
// Use parentheses around with to method.
for (i <- 0.to(3)) {
println("for to", i)
}
// Use parentheses with until.
for (i <- 0.until(3)) {
println("for until", i)
}
Output
(for to,0)
(for to,1)
(for to,2)
(for to,3)
(for until,0)
(for until,1)
(for until,2)
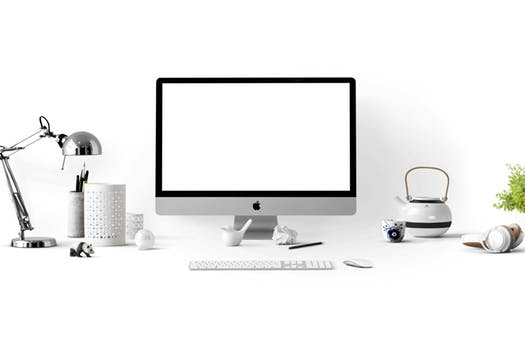
Ranges. We can directly loop over the numbers in a Range. The first argument to Range is the start, the second is the exclusive end. The third, optional argument is the step.
RangeTip: The to() and until() methods return ranges. The for-loop can also loop over a stored local range val.
Scala program that uses for-range
// Loop over the numbers in a range.
for (i <- Range(5, 8)) {
println("range1", i)
}
// Loop with a step of 2.
for (i <- Range(0, 5, 2)) {
println("range2", i)
}
// A decrement for-loop.
// ... Step is negative 1.
for (i <- Range(10, 5, -1)) {
println("range3", i)
}
Output
(range1,5)
(range1,6)
(range1,7)
(range2,0)
(range2,2)
(range2,4)
(range3,10)
(range3,9)
(range3,8)
(range3,7)
(range3,6)
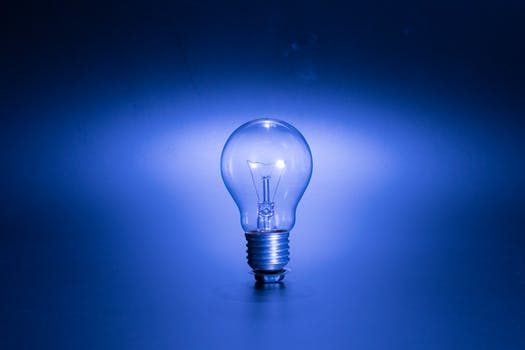
While. This loop continues until the condition is not true. Here we begin with a var assigned to 3. This is the iteration variable, and it cannot be constant.
VarThen: We loop while "i" is greater than or equal to zero. We decrement its value at the end of each iteration.
Scala program that uses while, decrement
// Begin at 3.
// ... Use var to have a mutable variable.
var i = 3
// Continue looping while "i" is greater than or equal to zero.
while (i >= 0) {
println(i)
// Decrement.
i -= 1
}
Output
3
2
1
0
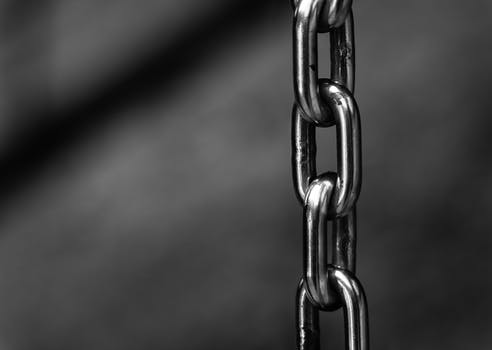
Do-while. This loop is similar to a while-loop, but the first iteration is entered without checking a condition. Instead the condition is checked after each iteration.
Scala program that uses do-while
var x = 0
// Begin a do-while loop.
// ... Continue while x is less than 3.
do {
println(x)
x += 1
} while (x < 3)
Output
0
1
2
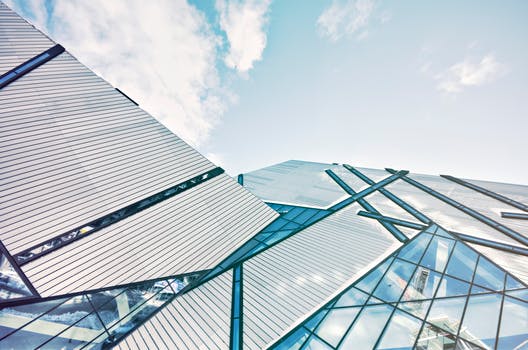
While true. Sometimes we want a loop to repeat indefinitely. With a while-true loop this is possible. This program continues looping until a random number less than or equal to 0.1 appears.
Random: JavaScala program that uses while-true loop
def test(): Unit = {
// Print random numbers in a loop.
while (true) {
val rand = Math.random()
// Return if random number is too small.
if (rand <= 0.1)
return
println(rand)
}
}
// Call test method.
test()
Output
0.5623560178530611
0.8956585369821868
0.4589158906009855
0.25810376023585613
0.6010527197089275
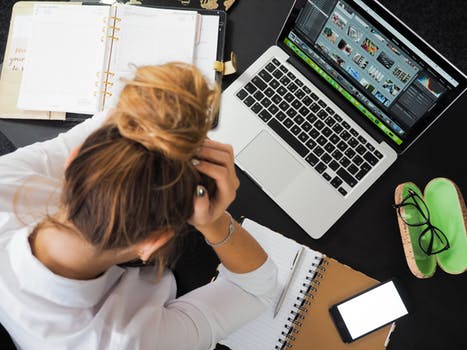
A summary. Loops are not the most glamorous programming construct. But they are effective. We have many for-loops available in the Scala language.
Related Links:
- Scala Convert: String to Int, List and Array
- Scala Odd and Even Numbers
- Scala Regex, R Examples: findFirstMatchIn
- Scala Interview Questions (2021)
- Scala Class Examples: Trait Keyword
- Scala indexOf: Lists and Strings
- Scala Int Examples: Max and Min
- Scala 2D List, Array Examples: Array.ofDim
- Scala Def: return Unit and Lambdas
- Scala Initialize List: List.newBuilder, List.empty
- Scala Option: None, get and getOrElse
- Scala Exception Handling: Try, Catch and Throw
- Scala Map Examples
- Scala String Examples: StringLike
- Scala Match and Case Examples
- Scala Remove Duplicates From List: Distinct Example
- Scala If, Else Example (Match Case If)
- Scala Console: println, printf and readLine
- Scala Strip Strings: stripLineEnd and stripMargin
- Scala Vector Examples
- Scala Var and Val (Variables and Constants)
- Learn Scala Tutorial
- Scala Keywords
- Scala format and formatted Strings
- Scala List Examples
- Scala StringBuilder Examples
- Scala Set Examples: contains, intersect
- Scala Tuple Examples (Return Multiple Values)
- Scala For to, until, while Loop Examples
- Scala Slice: Substring, List Slice
- Scala Sorted List Examples: Ordering.Int
- Scala Split String Examples
- Scala Array Examples: Apply and Update
- Scala Range Examples: Start, End and Step
Related Links
Adjectives
Ado
Ai
Android
Angular
Antonyms
Apache
Articles
Asp
Autocad
Automata
Aws
Azure
Basic
Binary
Bitcoin
Blockchain
C
Cassandra
Change
Coa
Computer
Control
Cpp
Create
Creating
C-Sharp
Cyber
Daa
Data
Dbms
Deletion
Devops
Difference
Discrete
Es6
Ethical
Examples
Features
Firebase
Flutter
Fs
Git
Go
Hbase
History
Hive
Hiveql
How
Html
Idioms
Insertion
Installing
Ios
Java
Joomla
Js
Kafka
Kali
Laravel
Logical
Machine
Matlab
Matrix
Mongodb
Mysql
One
Opencv
Oracle
Ordering
Os
Pandas
Php
Pig
Pl
Postgresql
Powershell
Prepositions
Program
Python
React
Ruby
Scala
Selecting
Selenium
Sentence
Seo
Sharepoint
Software
Spellings
Spotting
Spring
Sql
Sqlite
Sqoop
Svn
Swift
Synonyms
Talend
Testng
Types
Uml
Unity
Vbnet
Verbal
Webdriver
What
Wpf