<< Back to SCALA
Scala If, Else Example (Match Case If)
Use an if, else if and else construct to test values. See the match case if syntax.If, else. A number is 10. It is not 20. But when we run our program we do not know this. We must use an if, else-if statement to test its value.
An expression. In Scala an if-else construct returns a value—the result of the expression. After the condition we specify the return value. No return statement is needed.
First example. Let us begin. First we assign the var number to the value 10. We then use an if, else-if statement. We use println in the blocks.
Result: The program prints "Not 20 or 30" because the number is 10. The if and else-if conditions do not evaluate to true.
Scala program that uses if, else-if, else statements
var number = 10
// Test number if an if, else-if, else construct.
if (number == 20)
println(20)
else if (number == 30)
println(30)
else
println("Not 20 or 30")
Output
Not 20 or 30
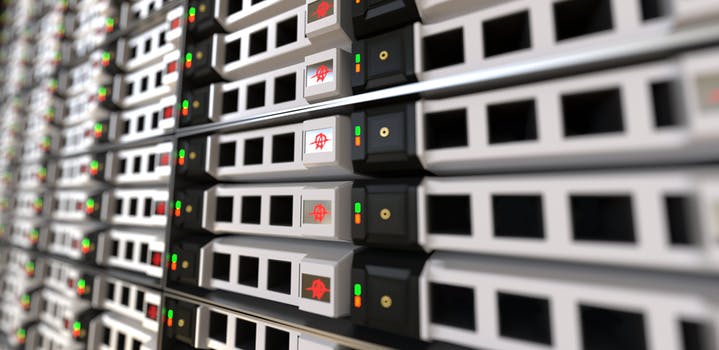
Expression result. An if-statement in Scala returns a value. So we can assign a value to an if-else expression. This returns the values specified after the conditions.
Here: The size is set to 20 if the animal is "bird." Otherwise size is set to 10.
Scala program that uses expression result
var animal = "bird"
// Use an if-else expression.
// ... This returns 20 or 10 based on the animal.
val size = if (animal == "bird") 20
else 10
// Print result of if-else.
println(size)
Output
20
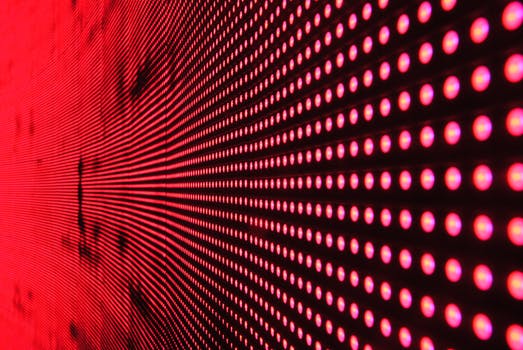
If versus match. Here we convert an if-else expression into a match expression. The if-else uses fewer characters and lines. But the match may be clearer and easier to add more cases to.
Scala program that tests if, match
val x = 100
// Use if-statement to set value of y1 based on x.
val y1 = if (x == 100) 20
else 30
// Use match to set value of y2 based on x.
// ... Equivalent to above if-statement.
val y2 = x match {
case 100 => 20
case _ => 30
}
// Print results.
println(y1)
println(y2)
Output
20
20
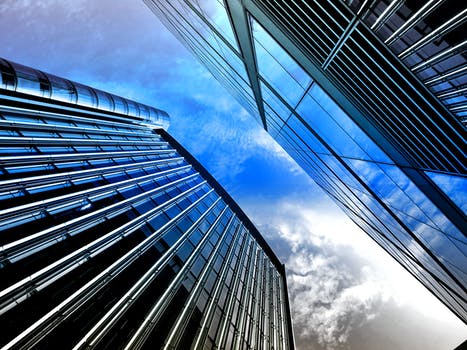
If inside match. We can use guarded cases inside a match construct. These use an if-expression as part of the case. We capture a variable ("c") to be used later in the case.
Tip: With guarded cases, we can use more complex expressions to test inside a match. We combine if-statements with pattern matching.
Scala program that uses if inside case
// Print a message based on Int argument.
// ... Use if-statement inside cases to determine message.
def printMessage(code: Int) = code match {
case c if c > 0 => println("Important, code is " + c)
case c if c <= 0 => println("Not important, code is " + c)
}
// Call def.
printMessage(1)
printMessage(-50)
Output
Important, code is 1
Not important, code is -50
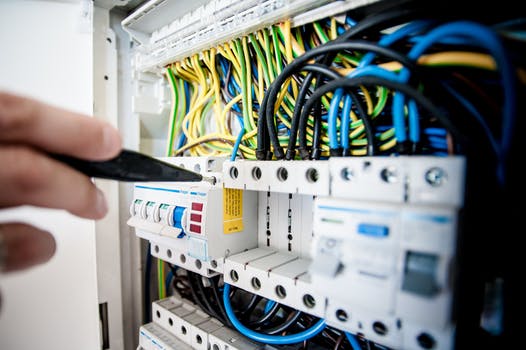
Match, continued. An alternative to the if-construct is match. This may be clearer in some cases. We think of match more like a traditional switch in C-like languages.
Match
A summary. In Scala we use if-else statements in an expression context. This makes an if-construct similar to a function. It has logic and returns values.
Related Links:
- Scala Convert: String to Int, List and Array
- Scala Odd and Even Numbers
- Scala Regex, R Examples: findFirstMatchIn
- Scala Interview Questions (2021)
- Scala Class Examples: Trait Keyword
- Scala indexOf: Lists and Strings
- Scala Int Examples: Max and Min
- Scala 2D List, Array Examples: Array.ofDim
- Scala Def: return Unit and Lambdas
- Scala Initialize List: List.newBuilder, List.empty
- Scala Option: None, get and getOrElse
- Scala Exception Handling: Try, Catch and Throw
- Scala Map Examples
- Scala String Examples: StringLike
- Scala Match and Case Examples
- Scala Remove Duplicates From List: Distinct Example
- Scala If, Else Example (Match Case If)
- Scala Console: println, printf and readLine
- Scala Strip Strings: stripLineEnd and stripMargin
- Scala Vector Examples
- Scala Var and Val (Variables and Constants)
- Learn Scala Tutorial
- Scala Keywords
- Scala format and formatted Strings
- Scala List Examples
- Scala StringBuilder Examples
- Scala Set Examples: contains, intersect
- Scala Tuple Examples (Return Multiple Values)
- Scala For to, until, while Loop Examples
- Scala Slice: Substring, List Slice
- Scala Sorted List Examples: Ordering.Int
- Scala Split String Examples
- Scala Array Examples: Apply and Update
- Scala Range Examples: Start, End and Step
Related Links
Adjectives
Ado
Ai
Android
Angular
Antonyms
Apache
Articles
Asp
Autocad
Automata
Aws
Azure
Basic
Binary
Bitcoin
Blockchain
C
Cassandra
Change
Coa
Computer
Control
Cpp
Create
Creating
C-Sharp
Cyber
Daa
Data
Dbms
Deletion
Devops
Difference
Discrete
Es6
Ethical
Examples
Features
Firebase
Flutter
Fs
Git
Go
Hbase
History
Hive
Hiveql
How
Html
Idioms
Insertion
Installing
Ios
Java
Joomla
Js
Kafka
Kali
Laravel
Logical
Machine
Matlab
Matrix
Mongodb
Mysql
One
Opencv
Oracle
Ordering
Os
Pandas
Php
Pig
Pl
Postgresql
Powershell
Prepositions
Program
Python
React
Ruby
Scala
Selecting
Selenium
Sentence
Seo
Sharepoint
Software
Spellings
Spotting
Spring
Sql
Sqlite
Sqoop
Svn
Swift
Synonyms
Talend
Testng
Types
Uml
Unity
Vbnet
Verbal
Webdriver
What
Wpf