<< Back to SCALA
Scala Var and Val (Variables and Constants)
Create variables and constants with var and val. Fix the reassignment to val error.Var, variables. Time changes everything. A variable at one point in time equals 10. But then it is reassigned: it now equals 20. Its value is not constant.
Val, meanwhile, is a keyword that indicates constancy. A var can be reassigned (though it does not have to be). A val can never be. A val is something you can rely on.
First example. Let us use var in a simple Scala program. We bind the variable with identifier "animal" to the string literal "cat." Then were assign it (rebind it) to the string "frog."
Tip: With this var, we can see the animal means different strings (cat and frog) at different points in time.
Scala program that uses var
// Assign variable to a string literal.
var animal = "cat"
println(animal)
// Reassign the variable.
animal = "frog"
println(animal)
Output
cat
frog
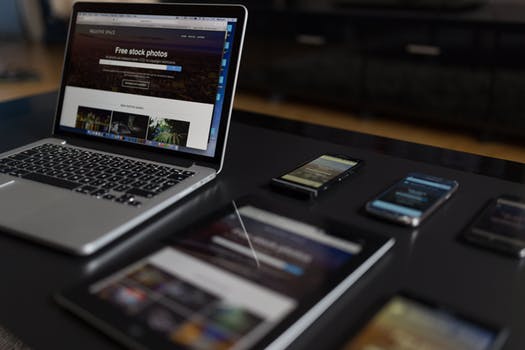
Reassignment to val. This program does not work. We introduce the val keyword, but use it incorrectly. We cannot change a val to point to a different value—it can only be set once.
Scala program that uses val, causes error
val animal = "cat"
println(animal)
// This will not compile.
animal = "frog"
println(animal)
Output
error: reassignment to val
animal = "frog"
^
one error found
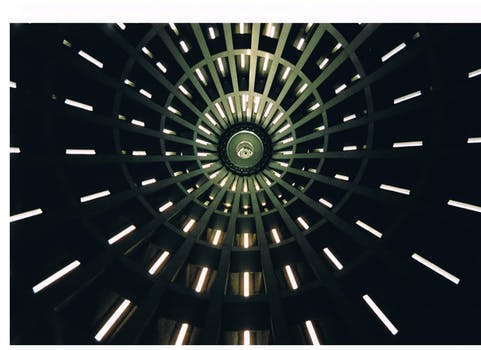
Separate val constants. This program uses val in a correct way. We rewrote our program to have two values, not a variable that is changed in the middle.
Scala program that uses val, separate values
// Use val for a value that cannot be reassigned.
val color1 = "magenta"
println(color1)
// Use a separate val.
val color2 = "aqua"
println(color2)
Output
magenta
aqua
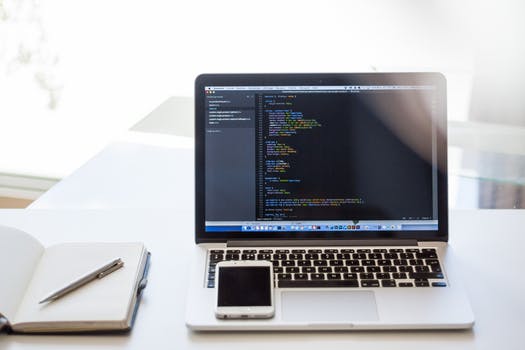
Val type. With val we can specify a type. Here we specify two numbers with initial values of 10. But we specify size2 to be a Double, not an Int. It is printed with a decimal place.
Scala program that uses val with type
// No type is specified, so Int is used.
val size1 = 10
// Specify a Double type.
val size2: Double = 10
// Print numbers.
println(size1)
println(size2)
Output
10
10.0
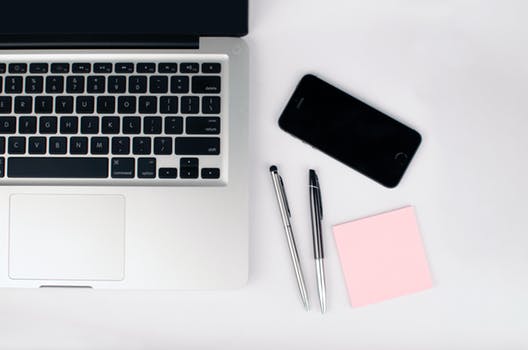
Class fields. With var and val we specify the fields of a class. In Scala these are public. Fields can be accessed outside the class body by default.
Var: We use var for width and height, as these can be mutated on Box instances. We assign them to 10 and 20.
Val: This is a constant value. It is a String. We cannot assign it, but we can access it and print its value.
StringprintlnScala program that uses class, var, val fields
class Box {
var width: Int = 0
var height: Int = 0
final val name: String = "Box"
}
// Create a new Box instance.
val box = new Box
// Assign width and height var fields.
box.width = 10
box.height = 20
// Print fields of box instance.
println(box.width)
println(box.height)
println(box.name)
Output
10
20
Box
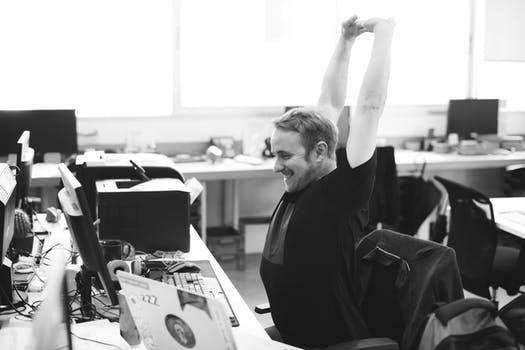
A discussion. Imagine we are writing a complex program. A variable X is needed in two places. We reassign it, but make a mistake—the second X should be separate.
And: With var, we might end up with a program that is invalid. With val, our program would not compile so we could correct it faster.
A summary. Immutable things are important in Scala. We have List, Map, Set: these are immutable data structures. With val, we have constants, immutable values—these improve program quality.
Related Links:
- Scala Convert: String to Int, List and Array
- Scala Odd and Even Numbers
- Scala Regex, R Examples: findFirstMatchIn
- Scala Interview Questions (2021)
- Scala Class Examples: Trait Keyword
- Scala indexOf: Lists and Strings
- Scala Int Examples: Max and Min
- Scala 2D List, Array Examples: Array.ofDim
- Scala Def: return Unit and Lambdas
- Scala Initialize List: List.newBuilder, List.empty
- Scala Option: None, get and getOrElse
- Scala Exception Handling: Try, Catch and Throw
- Scala Map Examples
- Scala String Examples: StringLike
- Scala Match and Case Examples
- Scala Remove Duplicates From List: Distinct Example
- Scala If, Else Example (Match Case If)
- Scala Console: println, printf and readLine
- Scala Strip Strings: stripLineEnd and stripMargin
- Scala Vector Examples
- Scala Var and Val (Variables and Constants)
- Learn Scala Tutorial
- Scala Keywords
- Scala format and formatted Strings
- Scala List Examples
- Scala StringBuilder Examples
- Scala Set Examples: contains, intersect
- Scala Tuple Examples (Return Multiple Values)
- Scala For to, until, while Loop Examples
- Scala Slice: Substring, List Slice
- Scala Sorted List Examples: Ordering.Int
- Scala Split String Examples
- Scala Array Examples: Apply and Update
- Scala Range Examples: Start, End and Step
Related Links
Adjectives
Ado
Ai
Android
Angular
Antonyms
Apache
Articles
Asp
Autocad
Automata
Aws
Azure
Basic
Binary
Bitcoin
Blockchain
C
Cassandra
Change
Coa
Computer
Control
Cpp
Create
Creating
C-Sharp
Cyber
Daa
Data
Dbms
Deletion
Devops
Difference
Discrete
Es6
Ethical
Examples
Features
Firebase
Flutter
Fs
Git
Go
Hbase
History
Hive
Hiveql
How
Html
Idioms
Insertion
Installing
Ios
Java
Joomla
Js
Kafka
Kali
Laravel
Logical
Machine
Matlab
Matrix
Mongodb
Mysql
One
Opencv
Oracle
Ordering
Os
Pandas
Php
Pig
Pl
Postgresql
Powershell
Prepositions
Program
Python
React
Ruby
Scala
Selecting
Selenium
Sentence
Seo
Sharepoint
Software
Spellings
Spotting
Spring
Sql
Sqlite
Sqoop
Svn
Swift
Synonyms
Talend
Testng
Types
Uml
Unity
Vbnet
Verbal
Webdriver
What
Wpf