<< Back to JAVA
Java Boolean Examples
Use the boolean type and the values true and false. Test them with expressions and ifs.Boolean. Day turns to night. Darkness comes over the land. Day and night are abstractions—one is true, the other false. This is a binary state.
Boolean features. With booleans, we can use the literals "true" and "false." We often use booleans inside if-statements, or while-loops.
An example. In this program we use the literal constants true and false. Often we assign a boolean to true or false as we declare it. Here we test for truth in two ifs.
True: The program first assigns the boolean of name "value" to true. The if-statement then detects "value" is true.
False: We then set the same boolean variable to false. Now the !value test evaluates to true, so the "B" is printed.
Java program that uses boolean, true, false
public class Program {
public static void main(String[] args) {
// Test true and false booleans.
boolean value = true;
if (value) {
System.out.println("A");
}
value = false;
if (!value) {
System.out.println("B");
}
}
}
Output
A
B
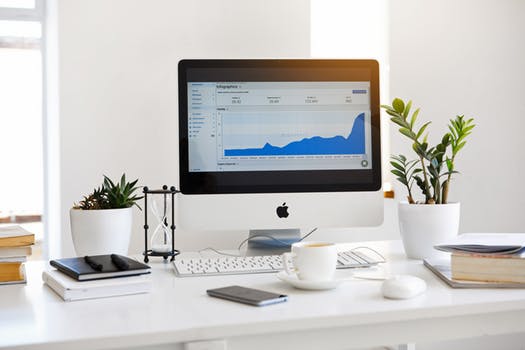
Flip. We can negate (or invert, or flip) a boolean value. This changes true to false, and false to true. We use the exclamation mark to perform this operation.
Tip: Flipping a boolean can be useful when you want "every other" iteration in a loop to perform a certain action.
Java program that flips boolean value
public class Program {
public static void main(String[] args) {
boolean value = true;
System.out.println(value);
// Flip from true to false.
value = !value;
System.out.println(value);
// Flip back to true from false.
value = !value;
System.out.println(value);
}
}
Output
true
false
true
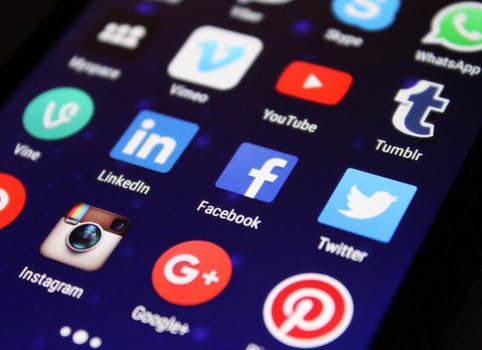
Expression variable. A boolean variable can store the result of an expression evaluation. It can then be reused, without reevaluating the expression.
Here: We see if the size and the color match the conditions for "large and blue." We can then directly test largeAndBlue.
Java program that uses expression, boolean variable
public class Program {
public static void main(String[] args) {
int size = 100;
String color = "blue";
// Use expression to compute a boolean value.
boolean largeAndBlue = size >= 50 && color == "blue";
// Test our boolean.
if (largeAndBlue) {
System.out.println("Large and blue = " + largeAndBlue);
}
}
}
Output
Large and blue = true
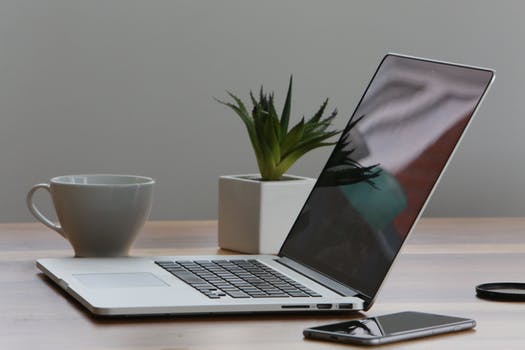
Boolean arguments. Methods often receive boolean arguments. We can pass them anything that evaluates to a boolean—this includes the constants true and false.
Java program that uses boolean arguments
public class Program {
static void display(boolean console) {
// Display a message if boolean argument is true.
if (console) {
System.out.println("The cat is cute.");
}
}
public static void main(String[] args) {
// A message is displayed.
display(true);
// These calls do not print anything to the console.
display(false);
display(false);
}
}
Output
The cat is cute.
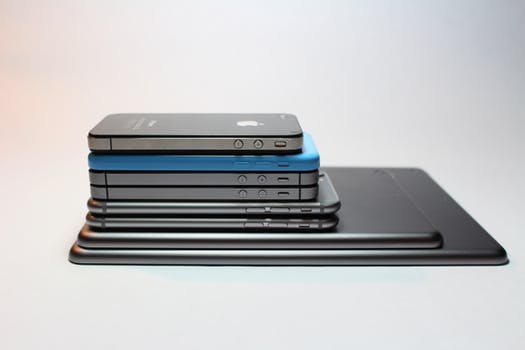
Return boolean. Here we see a method that returns a boolean based on its arguments. We prefix the method with the word "is." This is a convention.
ReturnInfo: The isValid method will return true if the three conditions it evaluates are all true. Otherwise it returns false.
Java program that uses boolean return value
public class Program {
static boolean isValid(String color, int size) {
// Return boolean based on three expressions.
return color.length() >= 1 && size >= 10 && size <= 100;
}
public static void main(String[] args) {
// This call returns true.
if (isValid("blue", 50)) {
System.out.println("A");
}
// This call is not valid because the size is too large.
if (!isValid("orange", 200)) {
System.out.println("B");
}
}
}
Output
A
B
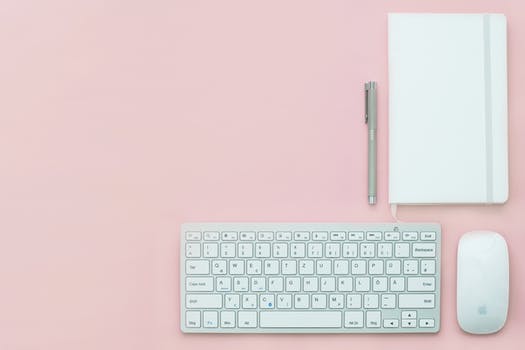
While-loop. We can use a boolean in a while-loop. Here we continue looping while the boolean is true, and we end the loop by setting the boolean to false.
WhileJava program that uses boolean in while-loop
public class Program {
public static void main(String[] args) {
boolean value = true;
// Continue looping while true.
while (value) {
System.out.println("OK");
value = false;
}
System.out.println("DONE");
}
}
Output
OK
DONE
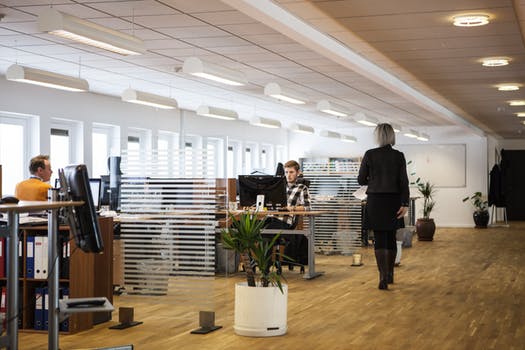
Memory usage. In this program we measure the memory usage of an array of booleans. We allocate one million boolean elements. With freeMemory(), we determine how much memory is used.
Result: The amount of free memory decreases by about 1 million bytes after the boolean array is allocated.
Thus: Each boolean element in the array requires one byte of memory. 16 bytes were used for the array itself.
Java program that measures boolean memory use
public class Program {
public static void main(String[] args) {
// Get free memory.
long memory1 = Runtime.getRuntime().freeMemory();
// Allocate and use an array of one million booleans.
boolean[] array = new boolean[1000000];
array[0] = true;
// Get free memory again.
long memory2 = Runtime.getRuntime().freeMemory();
array[1] = true;
// See how much memory was used.
System.out.println(memory1 - memory2);
}
}
Output
1000016
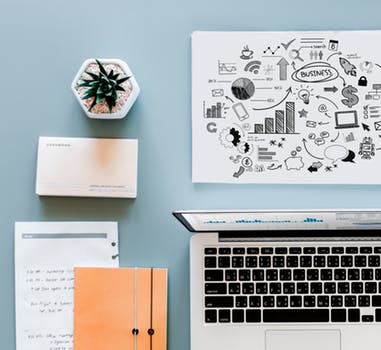
Convert to int. We cannot directly convert a boolean to an int (like 0 or 1). Often a ternary expression is helpful for this requirement.
Convert boolean to int
An optimization. Sometimes a program checks a set of conditions repeatedly in if-statements. We can place the result of this check in a boolean.
An optimization, continued. With the boolean variable, we can test without evaluating the entire expression again. We can just test that boolean value. This simplifies programs.
A summary. With booleans, we store true and false values. We store the results of complex expressions. And we can return, and receive, booleans from methods.
Related Links:
- Java Continue Keyword
- Java Convert Char Array to String
- Java Combine Arrays
- Java Console Examples
- Java Web Services Tutorial
- Java Odd and Even Numbers: Modulo Division
- Java IO
- Java 9 Features
- Java 8 Features
- Java String
- Java Regex | Regular Expression
- Java Filename With Date Example (Format String)
- Java Applet Tutorial
- Java Files.Copy: Copy File
- Java filter Example: findFirst, IntStream
- Java Final and final static Constants
- Java Super: Parent Class
- Java Date and Time
- Java do while loop
- Java Break
- Java Continue
- Java Comments
- Java Splitter Examples: split, splitToList
- Java Math.sqrt Method: java.lang.Math.sqrt
- Java Reflection
- Java Convert String to int
- JDBC Tutorial | What is Java Database Connectivity(JDBC)
- Java main() method
- Java HashMap Examples
- Java HashSet Examples
- Java Arrays.binarySearch
- Java Integer.bitCount and toBinaryString
- Java Overload Method Example
- Java First Words in String
- Java Convert ArrayList to String
- Java Convert boolean to int (Ternary Method)
- Java regionMatches Example and Performance
- Java ArrayDeque Examples
- Java ArrayList add and addAll (Insert Elements)
- Java ArrayList Clear
- Java ArrayList int, Integer Example
- Java ArrayList Examples
- Java Boolean Examples
- Java break Statement
- Java Newline Examples: System.lineSeparator
- Java Stream: Arrays.stream and ArrayList stream
- Java charAt Examples (String For Loop)
- Java Programs | Java Programming Examples
- Java OOPs Concepts
- Java Naming Conventions
- Java Constructor
- Java Class Example
- Java indexOf Examples
- Java Collections.addAll: Add Array to ArrayList
- Java Compound Interest
- Java Int Array
- Java Interface Examples
- Java 2D Array Examples
- Java Remove HTML Tags
- Java Stack Examples: java.util.Stack
- Java Enum Examples
- Java EnumMap Examples
- Java StackOverflowError
- Java startsWith and endsWith Methods
- Java Initialize ArrayList
- Java Object Array Examples: For, Cast and getClass
- Java Objects, Objects.requireNonNull Example
- Java Optional Examples
- Java Static Initializer
- Java static Keyword
- Java Package: Import Keyword Example
- Java Do While Loop Examples
- Java Double Numbers: Double.BYTES and Double.SIZE
- Java Truncate Number: Cast Double to Int
- Java Padding: Pad Left and Right of Strings
- Java Anagram Example: HashMap and ArrayList
- Java Math.abs: Absolute Value
- Java Extends: Class Inheritance
- Java String Class
- Java String Switch Example: Switch Versus HashMap
- Java StringBuffer: append, Performance
- Java Array Examples
- Java Remove Duplicates From ArrayList
- Java if, else if, else Statements
- Java Math.ceil Method
- Java This Keyword
- Java PriorityQueue Example (add, peek and poll)
- Java Process.start EXE: ProcessBuilder Examples
- Java Palindrome Method
- Java parseInt: Convert String to Int
- Java toCharArray: Convert String to Array
- Java Caesar Cipher
- Java Array Length: Get Size of Array
- Java String Array Examples
- Java String compareTo, compareToIgnoreCase
- Java String Concat: Append and Combine Strings
- Java Cast and Convert Types
- Java Math.floor Method, floorDiv and floorMod
- Java Math Class: java.lang.Math
- Java While Loop Examples
- Java Reverse String
- Java Download Web Pages: URL and openStream
- Java Math.pow Method
- Java Math.round Method
- Java Right String Part
- Java MongoDB Example
- Java Substring Examples, subSequence
- Java Prime Number Method
- Java Sum Methods: IntStream and reduce
- Java switch Examples
- Java Convert HashMap to ArrayList
- Java Remove Duplicate Chars
- Java Constructor: Overloaded, Default, This Constructors
- Java String isEmpty Method (Null, Empty Strings)
- Java Regex Examples (Pattern.matches)
- Java ROT13 Method
- Java Random Number Examples
- Java Recursion Example: Count Change
- Java reflect: getDeclaredMethod, invoke
- Java Count Letter Frequencies
- Java ImmutableList Examples
- Java String equals, equalsIgnoreCase and contentEquals
- Java valueOf and copyValueOf String Examples
- Java Vector Examples
- Java Word Count Methods: Split and For Loop
- Java Tutorial | Learn Java Programming
- Java toLowerCase, toUpperCase Examples
- Java Ternary Operator
- Java Tree: HashMap and Strings Example
- Java TreeMap Examples
- Java while loop
- Java Convert String to Byte Array
- Java Join Strings: String.join Method
- Java Modulo Operator Examples
- Java Integer.MAX VALUE, MIN and SIZE
- Java Lambda Expressions
- Java lastIndexOf Examples
- Java Multiple Return Values
- Java String.format Examples: Numbers and Strings
- Java Joiner Examples: join
- Java Keywords
- Java Replace Strings: replaceFirst and replaceAll
- Java return Examples
- Java Multithreading Interview Questions (2021)
- Java Collections Interview Questions (2021)
- Java Shuffle Arrays (Fisher Yates)
- Top 30 Java Design Patterns Interview Questions (2021)
- Java ListMultimap Examples
- Java String Occurrence Method: While Loop Method
- Java StringBuilder capacity
- Java Math.max and Math.min
- Java Factory Design Pattern
- Java StringBuilder Examples
- Java Mail Tutorial
- Java Swing Tutorial
- Java AWT Tutorial
- Java Fibonacci Sequence Examples
- Java StringTokenizer Example
- Java Method Examples: Instance and Static
- Java String Between, Before, After
- Java BitSet Examples
- Java System.gc, Runtime.getRuntime and freeMemory
- Java Character Examples
- Java Char Lookup Table
- Java BufferedWriter Examples: Write Strings to Text File
- Java Abstract Class
- Java Hashtable
- Java Math class with Methods
- Java Whitespace Methods
- Java Data Types
- Java Trim String Examples (Trim Start, End)
- Java Exceptions: try, catch and finally
- Java vs C#
- Java Float Numbers
- Java Truncate String
- Java Variables
- Java For Loop Examples
- Java Uppercase First Letter
- Java Inner Class
- Java Networking
- Java Keywords
- Java If else
- Java Switch
- Loops in Java | Java For Loop
- Java File: BufferedReader and FileReader
- Java Random Lowercase Letter
- Java Calendar Examples: Date and DateFormat
- Java Case Keyword
- Java Char Array
- Java ASCII Table
- Java IntStream.Range Example (Get Range of Numbers)
- Java length Example: Get Character Count
- Java Line Count for File
- Java Sort Examples: Arrays.sort, Comparable
- Java LinkedHashMap Example
- Java Split Examples
Related Links
Adjectives
Ado
Ai
Android
Angular
Antonyms
Apache
Articles
Asp
Autocad
Automata
Aws
Azure
Basic
Binary
Bitcoin
Blockchain
C
Cassandra
Change
Coa
Computer
Control
Cpp
Create
Creating
C-Sharp
Cyber
Daa
Data
Dbms
Deletion
Devops
Difference
Discrete
Es6
Ethical
Examples
Features
Firebase
Flutter
Fs
Git
Go
Hbase
History
Hive
Hiveql
How
Html
Idioms
Insertion
Installing
Ios
Java
Joomla
Js
Kafka
Kali
Laravel
Logical
Machine
Matlab
Matrix
Mongodb
Mysql
One
Opencv
Oracle
Ordering
Os
Pandas
Php
Pig
Pl
Postgresql
Powershell
Prepositions
Program
Python
React
Ruby
Scala
Selecting
Selenium
Sentence
Seo
Sharepoint
Software
Spellings
Spotting
Spring
Sql
Sqlite
Sqoop
Svn
Swift
Synonyms
Talend
Testng
Types
Uml
Unity
Vbnet
Verbal
Webdriver
What
Wpf