<< Back to JAVA
Java Split Examples
Separate strings on a delimiter with the split method. Split lines of a file.Split. Often strings are read in from lines of a file. And these lines have many parts, separated by delimiters. With use split() to break them apart.
Regex. Split in Java uses a Regex. A single character (like a comma) can be split upon. Or a more complex pattern (with character codes) can be used.
A simple example. Let's begin with this example. We introduce a string that has 2 commas in it, separating 3 strings (cat, dog, bird). We split on a comma.
For: Split returns a String array. We then loop over that array's elements with a for-each loop. We display them.
Java program that uses split
public class Program {
public static void main(String[] args) {
// This string has three words separated by commas.
String value = "cat,dog,bird";
// Split on a comma.
String parts[] = value.split(",");
// Display result parts.
for (String part : parts) {
System.out.println(part);
}
}
}
Output
cat
dog
rat
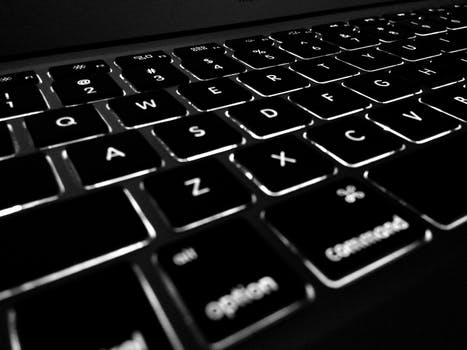
Split lines in file. Here we use BufferedReader and FileReader to read in a text file. Then, while looping over it, we split each line. In this way we parse a CSV file with split.
FilePrintln: Finally we use the System.out.println method to display each part from each line to the screen.
ConsoleContents: file.txt
carrot,squash,turnip
potato,spinach,kale
Java program that reads file, splits lines
import java.io.BufferedReader;
import java.io.FileReader;
import java.io.IOException;
public class Program {
public static void main(String[] args) throws IOException {
// Open this file.
BufferedReader reader = new BufferedReader(new FileReader(
"C:\\programs\\file.txt"));
// Read lines from file.
while (true) {
String line = reader.readLine();
if (line == null) {
break;
}
// Split line on comma.
String[] parts = line.split(",");
for (String part : parts) {
System.out.println(part);
}
System.out.println();
}
reader.close();
}
}
Output
carrot
squash
turnip
potato
spinach
kale
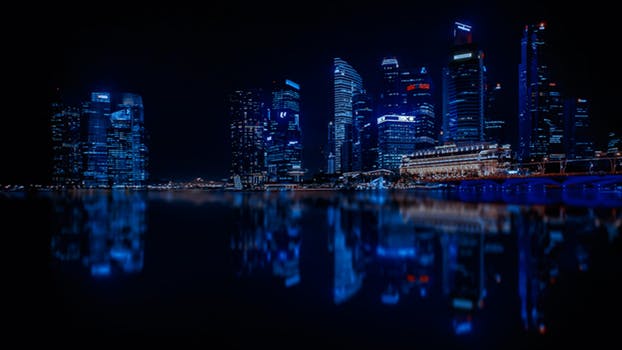
Either character. Often data is inconsistent. Sometimes we need to split on a range or set of characters. With split, this is possible. Here we split on a comma and a colon.
Tip: With square brackets, we specify the possible characters to split upon. So we split on all colons and commas, with one call.
Java program that splits on either character
public class Program {
public static void main(String[] args) {
String line = "carrot:orange,apple:red";
// Split on comma or colon.
String[] parts = line.split("[,:]");
for (String part : parts) {
System.out.println(part);
}
}
}
Output
carrot
orange
apple
red
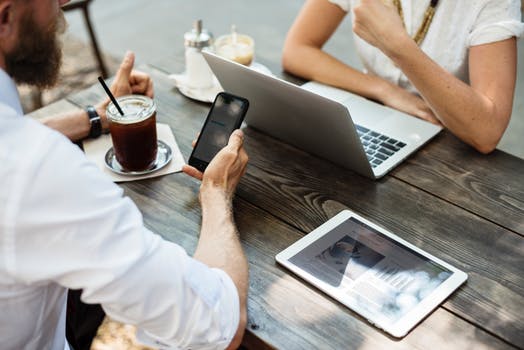
Count, separate words. We can use more advanced character patterns in split. Here we separate a String based on non-word characters. We use "\W+" to mean this.
Pattern: The pattern means "one or more non-word characters." A plus means "one or more" and a W means non-word.
Note: The comma and its following space are treated as a single delimiter. So two characters are matched as one delimiter.
Java program that counts, splits words
public class Program {
public static void main(String[] args) {
String line = "hello, how are you?";
// Split on 1+ non-word characters.
String[] words = line.split("\\W+");
// Count words.
System.out.println(words.length);
// Display words.
for (String word : words) {
System.out.println(word);
}
}
}
Output
4
hello
how
are
you
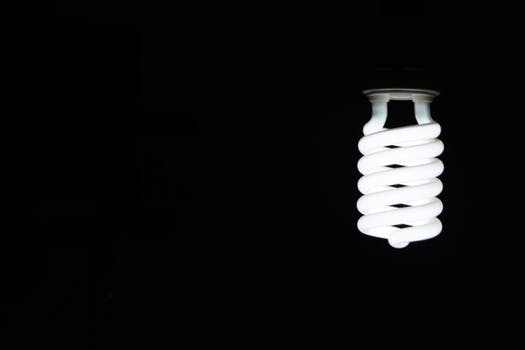
Numbers. This example splits a string apart and then uses parseInt to convert those parts into ints. It splits on a two-char sequence. Then in a loop, it calls parseInt on each String.
ParseIntJava program that uses split, parseInt
public class Program {
public static void main(String[] args) {
String line = "1, 2, 3";
// Split on two-char sequence.
String[] numbers = line.split(", ");
// Display numbers.
for (String number : numbers) {
int value = Integer.parseInt(number);
System.out.println(value + " * 20 = " + value * 20);
}
}
}
Output
1 * 20 = 20
2 * 20 = 40
3 * 20 = 60
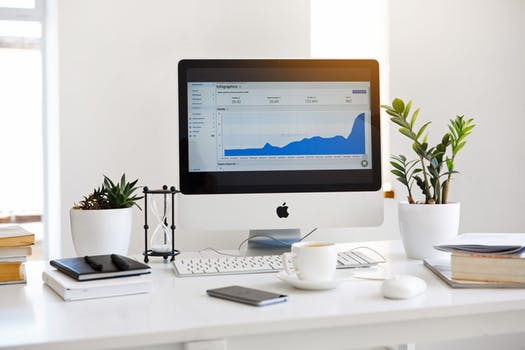
Limit. Split accepts an optional second parameter, a limit Integer. If we provide this, the result array has (at most) that many elements. Any extra parts remain part of the last element.
Info: To have a limit argument, we must use a Regex. Here we escape the vertical bar so it is treated like a normal char.
Here: We get the first 2 parts split apart correctly, and the third part has all the remaining (unsplit) parts.
Java program that uses split with limit
public class Program {
public static void main(String[] args) {
String value = "a|b|c|d|e";
// Use limit of just 3 parts.
// ... Escape the bar for a Regex.
String parts[] = value.split("\\|", 3);
// Only 3 elements are in the result array.
for (String part : parts) {
System.out.println(part);
}
}
}
Output
a
b
c|d|e
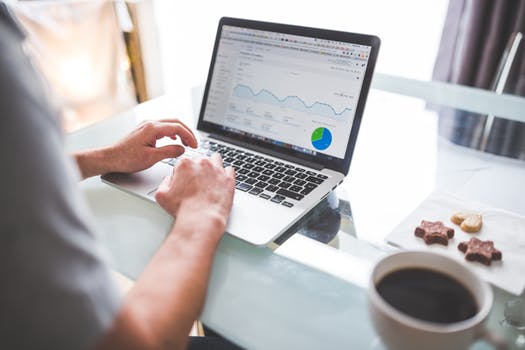
Pattern.compile, split. A split method is available on the Pattern class, found in java.util.regex. We can compile a Pattern and reuse it many times. This can enhance performance.
Note: A call to Pattern.compile optimizes all split() calls afterwards. But this only helps if many splits are done.
PatternJava program that uses Pattern.compile, split
import java.util.regex.Pattern;
public class Program {
public static void main(String[] args) {
// Separate based on number delimiters.
Pattern p = Pattern.compile("\\d+");
String value = "abc100defgh9ij";
String[] elements = p.split(value);
// Display our results.
for (String element : elements) {
System.out.println(element);
}
}
}
Output
abc
defgh
ij
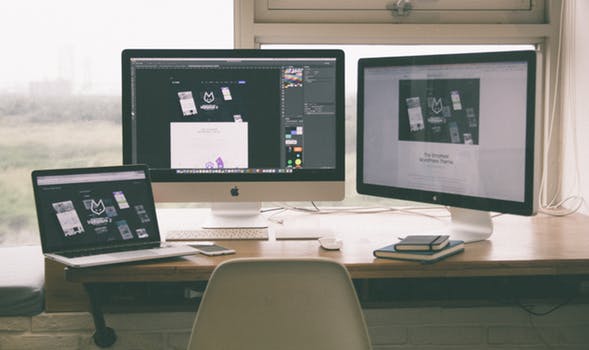
Benchmark, pattern split. We can improve the speed of splitting strings based on regular expressions by using Pattern.compile. We create a delimiter pattern. Then we call split() with it.
Version 1: This version of the code uses Pattern split(): it reuses the same Pattern instance many times.
Version 2: This code uses split() with a Regex argument, so it does not reuse the same Regex.
Result: When many Strings are split, a call Pattern.compile before using its Split method optimizes performance.
Java program that times Pattern split
import java.util.regex.Pattern;
public class Program {
public static void main(String[] args) {
// ... Create a delimiter pattern.
Pattern pattern = Pattern.compile("\\W+");
String line = "cat; dog--ABC";
long t1 = System.currentTimeMillis();
// Version 1: use split method on Pattern.
for (int i = 0; i < 1000000; i++) {
String[] values = pattern.split(line);
if (values.length != 3) {
System.out.println(false);
}
}
long t2 = System.currentTimeMillis();
// Version 2: use String split method.
for (int i = 0; i < 1000000; i++) {
String[] values = line.split("\\W+");
if (values.length != 3) {
System.out.println(false);
}
}
long t3 = System.currentTimeMillis();
// ... Benchmark results.
System.out.println(t2 - t1);
System.out.println(t3 - t2);
}
}
Output
471 ms, Pattern split
549 ms, String split
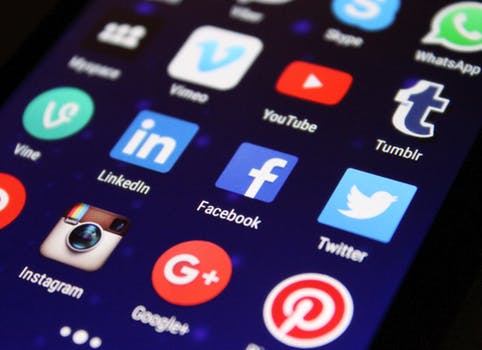
Join. This method combines Strings together—we specify our desired delimiter String. Join is sophisticated. It can handle a String array or individual Strings.
Join
Word count. We can count the words in a string by splitting the string on non-word (or space) characters. This is not the fastest method, but it tends to be a fairly accurate one.
Word Count
With split, we use a regular expression-based pattern. But for simple cases, we provide the delimiter itself as the pattern. This too works. Split is elegant and powerful.
Related Links:
- Java Continue Keyword
- Java Convert Char Array to String
- Java Combine Arrays
- Java Console Examples
- Java Web Services Tutorial
- Java Odd and Even Numbers: Modulo Division
- Java IO
- Java 9 Features
- Java 8 Features
- Java String
- Java Regex | Regular Expression
- Java Filename With Date Example (Format String)
- Java Applet Tutorial
- Java Files.Copy: Copy File
- Java filter Example: findFirst, IntStream
- Java Final and final static Constants
- Java Super: Parent Class
- Java Date and Time
- Java do while loop
- Java Break
- Java Continue
- Java Comments
- Java Splitter Examples: split, splitToList
- Java Math.sqrt Method: java.lang.Math.sqrt
- Java Reflection
- Java Convert String to int
- JDBC Tutorial | What is Java Database Connectivity(JDBC)
- Java main() method
- Java HashMap Examples
- Java HashSet Examples
- Java Arrays.binarySearch
- Java Integer.bitCount and toBinaryString
- Java Overload Method Example
- Java First Words in String
- Java Convert ArrayList to String
- Java Convert boolean to int (Ternary Method)
- Java regionMatches Example and Performance
- Java ArrayDeque Examples
- Java ArrayList add and addAll (Insert Elements)
- Java ArrayList Clear
- Java ArrayList int, Integer Example
- Java ArrayList Examples
- Java Boolean Examples
- Java break Statement
- Java Newline Examples: System.lineSeparator
- Java Stream: Arrays.stream and ArrayList stream
- Java charAt Examples (String For Loop)
- Java Programs | Java Programming Examples
- Java OOPs Concepts
- Java Naming Conventions
- Java Constructor
- Java Class Example
- Java indexOf Examples
- Java Collections.addAll: Add Array to ArrayList
- Java Compound Interest
- Java Int Array
- Java Interface Examples
- Java 2D Array Examples
- Java Remove HTML Tags
- Java Stack Examples: java.util.Stack
- Java Enum Examples
- Java EnumMap Examples
- Java StackOverflowError
- Java startsWith and endsWith Methods
- Java Initialize ArrayList
- Java Object Array Examples: For, Cast and getClass
- Java Objects, Objects.requireNonNull Example
- Java Optional Examples
- Java Static Initializer
- Java static Keyword
- Java Package: Import Keyword Example
- Java Do While Loop Examples
- Java Double Numbers: Double.BYTES and Double.SIZE
- Java Truncate Number: Cast Double to Int
- Java Padding: Pad Left and Right of Strings
- Java Anagram Example: HashMap and ArrayList
- Java Math.abs: Absolute Value
- Java Extends: Class Inheritance
- Java String Class
- Java String Switch Example: Switch Versus HashMap
- Java StringBuffer: append, Performance
- Java Array Examples
- Java Remove Duplicates From ArrayList
- Java if, else if, else Statements
- Java Math.ceil Method
- Java This Keyword
- Java PriorityQueue Example (add, peek and poll)
- Java Process.start EXE: ProcessBuilder Examples
- Java Palindrome Method
- Java parseInt: Convert String to Int
- Java toCharArray: Convert String to Array
- Java Caesar Cipher
- Java Array Length: Get Size of Array
- Java String Array Examples
- Java String compareTo, compareToIgnoreCase
- Java String Concat: Append and Combine Strings
- Java Cast and Convert Types
- Java Math.floor Method, floorDiv and floorMod
- Java Math Class: java.lang.Math
- Java While Loop Examples
- Java Reverse String
- Java Download Web Pages: URL and openStream
- Java Math.pow Method
- Java Math.round Method
- Java Right String Part
- Java MongoDB Example
- Java Substring Examples, subSequence
- Java Prime Number Method
- Java Sum Methods: IntStream and reduce
- Java switch Examples
- Java Convert HashMap to ArrayList
- Java Remove Duplicate Chars
- Java Constructor: Overloaded, Default, This Constructors
- Java String isEmpty Method (Null, Empty Strings)
- Java Regex Examples (Pattern.matches)
- Java ROT13 Method
- Java Random Number Examples
- Java Recursion Example: Count Change
- Java reflect: getDeclaredMethod, invoke
- Java Count Letter Frequencies
- Java ImmutableList Examples
- Java String equals, equalsIgnoreCase and contentEquals
- Java valueOf and copyValueOf String Examples
- Java Vector Examples
- Java Word Count Methods: Split and For Loop
- Java Tutorial | Learn Java Programming
- Java toLowerCase, toUpperCase Examples
- Java Ternary Operator
- Java Tree: HashMap and Strings Example
- Java TreeMap Examples
- Java while loop
- Java Convert String to Byte Array
- Java Join Strings: String.join Method
- Java Modulo Operator Examples
- Java Integer.MAX VALUE, MIN and SIZE
- Java Lambda Expressions
- Java lastIndexOf Examples
- Java Multiple Return Values
- Java String.format Examples: Numbers and Strings
- Java Joiner Examples: join
- Java Keywords
- Java Replace Strings: replaceFirst and replaceAll
- Java return Examples
- Java Multithreading Interview Questions (2021)
- Java Collections Interview Questions (2021)
- Java Shuffle Arrays (Fisher Yates)
- Top 30 Java Design Patterns Interview Questions (2021)
- Java ListMultimap Examples
- Java String Occurrence Method: While Loop Method
- Java StringBuilder capacity
- Java Math.max and Math.min
- Java Factory Design Pattern
- Java StringBuilder Examples
- Java Mail Tutorial
- Java Swing Tutorial
- Java AWT Tutorial
- Java Fibonacci Sequence Examples
- Java StringTokenizer Example
- Java Method Examples: Instance and Static
- Java String Between, Before, After
- Java BitSet Examples
- Java System.gc, Runtime.getRuntime and freeMemory
- Java Character Examples
- Java Char Lookup Table
- Java BufferedWriter Examples: Write Strings to Text File
- Java Abstract Class
- Java Hashtable
- Java Math class with Methods
- Java Whitespace Methods
- Java Data Types
- Java Trim String Examples (Trim Start, End)
- Java Exceptions: try, catch and finally
- Java vs C#
- Java Float Numbers
- Java Truncate String
- Java Variables
- Java For Loop Examples
- Java Uppercase First Letter
- Java Inner Class
- Java Networking
- Java Keywords
- Java If else
- Java Switch
- Loops in Java | Java For Loop
- Java File: BufferedReader and FileReader
- Java Random Lowercase Letter
- Java Calendar Examples: Date and DateFormat
- Java Case Keyword
- Java Char Array
- Java ASCII Table
- Java IntStream.Range Example (Get Range of Numbers)
- Java length Example: Get Character Count
- Java Line Count for File
- Java Sort Examples: Arrays.sort, Comparable
- Java LinkedHashMap Example
- Java Split Examples
Related Links
Adjectives
Ado
Ai
Android
Angular
Antonyms
Apache
Articles
Asp
Autocad
Automata
Aws
Azure
Basic
Binary
Bitcoin
Blockchain
C
Cassandra
Change
Coa
Computer
Control
Cpp
Create
Creating
C-Sharp
Cyber
Daa
Data
Dbms
Deletion
Devops
Difference
Discrete
Es6
Ethical
Examples
Features
Firebase
Flutter
Fs
Git
Go
Hbase
History
Hive
Hiveql
How
Html
Idioms
Insertion
Installing
Ios
Java
Joomla
Js
Kafka
Kali
Laravel
Logical
Machine
Matlab
Matrix
Mongodb
Mysql
One
Opencv
Oracle
Ordering
Os
Pandas
Php
Pig
Pl
Postgresql
Powershell
Prepositions
Program
Python
React
Ruby
Scala
Selecting
Selenium
Sentence
Seo
Sharepoint
Software
Spellings
Spotting
Spring
Sql
Sqlite
Sqoop
Svn
Swift
Synonyms
Talend
Testng
Types
Uml
Unity
Vbnet
Verbal
Webdriver
What
Wpf