<< Back to JAVA
Java return Examples
Use the return keyword in methods. Return multiple values, return expressions and fix errors.Return. A stone is thrown into the air. It comes back down to the ocean and makes a splash. It returns. In Java too methods return.
In a return statement, we evaluate expressions—and as part of this evaluation, other methods may run. Types must match, or an error occurs. Code after a return may become unreachable.
Expression. We can return a single value. But often we want a more complex return statement—we use an expression after the return keyword. Here an expression that multiplies two values.
ComputeSize: This method receives two arguments, both of type int. In the return expression, the two numbers are multiplied.
And: The evaluated result is returned to the calling location. The expression itself is not returned, just its result.
Java program that returns expression
public class Program {
static int computeSize(int height, int width) {
// Return an expression based on two arguments (variables).
return height * width;
}
public static void main(String[] args) {
// Assign to the result of computeSize.
int result = computeSize(10, 3);
System.out.println(result);
}
}
Output
30
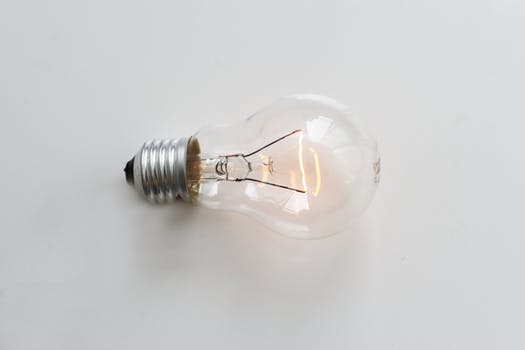
Return method result. In a return statement, we can invoke another method. In this example, we return the result of cube() when getVolume() returns.
And: The cube method itself returns the result of Math.pow, a built-in mathematics method.
Java program that calls method in return statement
public class Program {
static int cube(int value) {
// Return number to the power of 3.
return (int) Math.pow(value, 3);
}
static int getVolume(int size) {
// Return cubed number.
return cube(size);
}
public static void main(String[] args) {
// Assign to the return value of getVolume.
int volume = getVolume(2);
System.out.println(volume);
}
}
Output
8
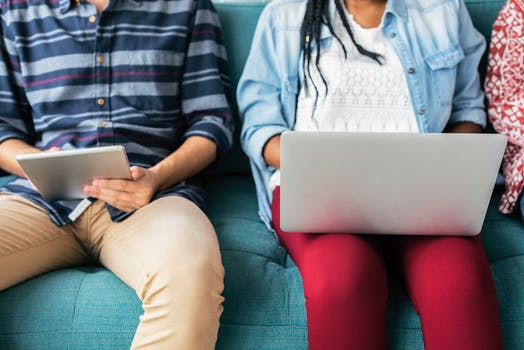
Return, void method. In a void method, an implicit (hidden) return is always at the end of the method. But we can specify a return statement (with no argument) to have an early exit.
Here: If the "password" argument has a length greater than or equal to 5, we return early. Otherwise we print a warning message.
Java program that uses return statement, void method
public class Program {
static void displayPassword(String password) {
// Write the password to the console.
System.out.println("Password: " + password);
// Return if our password is long enough.
if (password.length() >= 5) {
return;
}
System.out.println("Password too short!");
// An implicit return is here.
}
public static void main(String[] args) {
displayPassword("furball");
displayPassword("cat");
}
}
Output
Password: furball
Password: cat
Password too short!
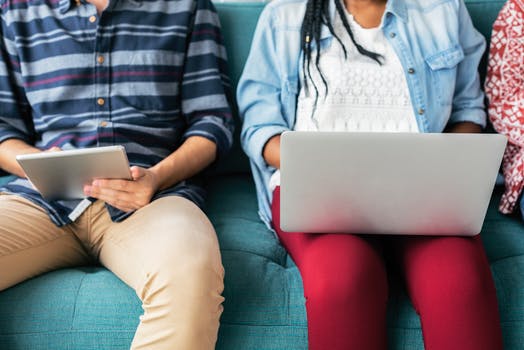
Boolean. We can use an expression to compose a boolean return value. This is a powerful technique—we combine several branches of logic into a single statement.
BooleanAnd: The result of isValid is a boolean. Both logical conditions must be satisfied for isValid to return true.
Java program that returns boolean from method
public class Program {
static boolean isValid(String name, boolean exists) {
// Return a boolean based on the two arguments.
return name.length() >= 3 && exists;
}
public static void main(String[] args) {
// Test the results of the isValid method.
System.out.println(isValid("green", true));
System.out.println(isValid("", true));
System.out.println(isValid("orchard", false));
}
}
Output
true
false
false
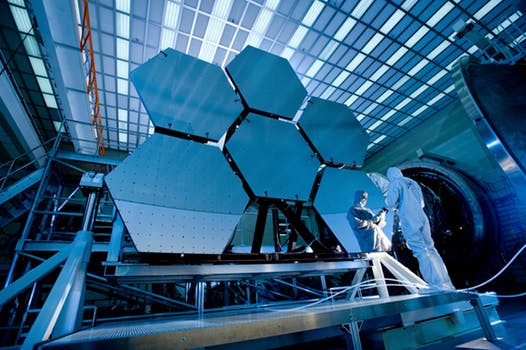
Compilation error. A method that is supposed to return a value (like an int) must return that value. Otherwise a helpful compilation error occurs.
Java program that causes compilation error
public class Program {
static int getResult(String id) {
// This method does not compile.
// ... It must return an int.
if (id.length() <= 4) {
System.out.println("Short");
}
}
public static void main(String[] args) {
int result = getResult("cat");
System.out.println(result);
}
}
Output
Exception in thread "main" java.lang.Error:
Unresolved compilation problem:
This method must return a result of type int
at Program.getResult(Program.java:3)
at Program.main(Program.java:13)
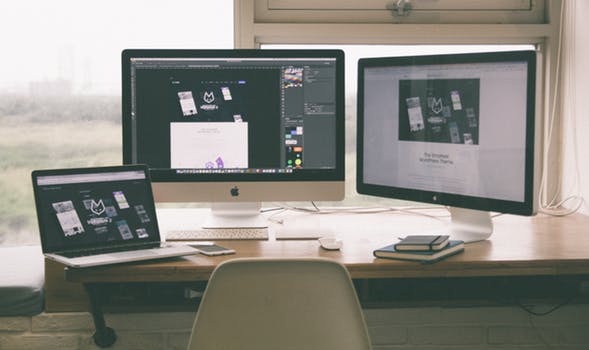
Multiple return values. This is a common programming problem. To return two values, we can use a class argument—then we set values within that class.
ClassResult: This has the same effect as returning two arguments. The syntax is more verbose. The object can be reused between method calls.
Tip: It is often a better design decision to have related methods on a class. Then those methods simply modify fields of the class.
Java program that returns multiple values
class Data {
public String name;
public int size;
}
public class Program {
static void getTwoValues(Data data) {
// This method returns two values.
// ... It sets values in a class.
data.name = "Java";
data.size = 100;
}
public static void main(String[] args) {
// Create our data object and call getTwoValues.
Data data = new Data();
getTwoValues(data);
System.out.println(data.name);
System.out.println(data.size);
}
}
Output
Java
100
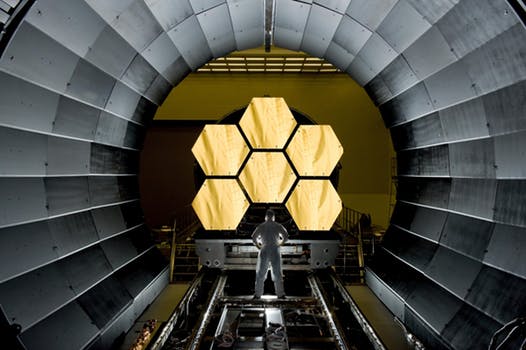
Unreachable code. This is a fatal error in Java. If code cannot be reached, we get a java.lang.Error. To fix this program, we must remove the "return" or the final, unreachable statement.
Java program that has unreachable code
public class Program {
public static void main(String[] args) {
System.out.println("Hello");
return;
System.out.println("World");
}
}
Output
Exception in thread "main" java.lang.Error:
Unresolved compilation problem:
Unreachable code
at Program.main(Program.java:9)
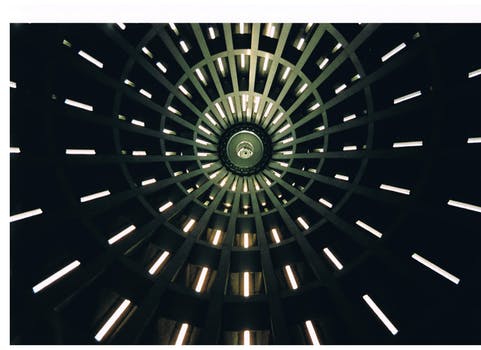
Type mismatch error. We cannot assign a variable to void method. This returns in a "type mismatch" error. Void means "no value." To fix, remove the variable assignment.
Java program that causes type mismatch error
public class Program {
static void test() {
System.out.println(123);
}
public static void main(String[] args) {
// This does not compile.
// ... We cannot assign to a void method.
int result = test();
}
}
Output
Exception in thread "main" java.lang.Error:
Unresolved compilation problem:
Type mismatch: cannot convert from void to int
at Program.main(Program.java:11)
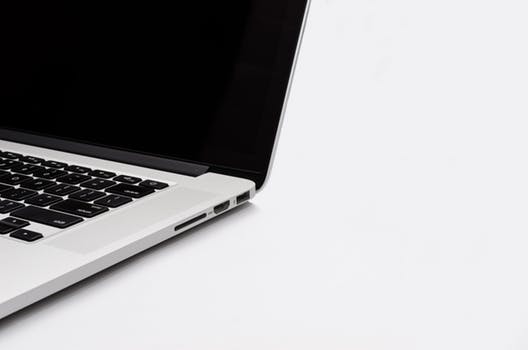
Performance. In my tests, I found that the JVM does a good job of inlining methods. So if we return a statement that evaluates into another method call, this causes no apparent slowdown.
Also: I found that expressions with temporary local variables were optimized well, to match single-expression return values.
A review. The return keyword signals an end to a method. It accepts an argument of a type that matches the method's signature. In a void method, we use a no-argument return.
To help improve quality, the Java compiler alerts us when a return renders code unreachable. It also requires that types match correctly. This helps ensure programs work as intended.
Related Links:
- Java Continue Keyword
- Java Convert Char Array to String
- Java Combine Arrays
- Java Console Examples
- Java Web Services Tutorial
- Java Odd and Even Numbers: Modulo Division
- Java IO
- Java 9 Features
- Java 8 Features
- Java String
- Java Regex | Regular Expression
- Java Filename With Date Example (Format String)
- Java Applet Tutorial
- Java Files.Copy: Copy File
- Java filter Example: findFirst, IntStream
- Java Final and final static Constants
- Java Super: Parent Class
- Java Date and Time
- Java do while loop
- Java Break
- Java Continue
- Java Comments
- Java Splitter Examples: split, splitToList
- Java Math.sqrt Method: java.lang.Math.sqrt
- Java Reflection
- Java Convert String to int
- JDBC Tutorial | What is Java Database Connectivity(JDBC)
- Java main() method
- Java HashMap Examples
- Java HashSet Examples
- Java Arrays.binarySearch
- Java Integer.bitCount and toBinaryString
- Java Overload Method Example
- Java First Words in String
- Java Convert ArrayList to String
- Java Convert boolean to int (Ternary Method)
- Java regionMatches Example and Performance
- Java ArrayDeque Examples
- Java ArrayList add and addAll (Insert Elements)
- Java ArrayList Clear
- Java ArrayList int, Integer Example
- Java ArrayList Examples
- Java Boolean Examples
- Java break Statement
- Java Newline Examples: System.lineSeparator
- Java Stream: Arrays.stream and ArrayList stream
- Java charAt Examples (String For Loop)
- Java Programs | Java Programming Examples
- Java OOPs Concepts
- Java Naming Conventions
- Java Constructor
- Java Class Example
- Java indexOf Examples
- Java Collections.addAll: Add Array to ArrayList
- Java Compound Interest
- Java Int Array
- Java Interface Examples
- Java 2D Array Examples
- Java Remove HTML Tags
- Java Stack Examples: java.util.Stack
- Java Enum Examples
- Java EnumMap Examples
- Java StackOverflowError
- Java startsWith and endsWith Methods
- Java Initialize ArrayList
- Java Object Array Examples: For, Cast and getClass
- Java Objects, Objects.requireNonNull Example
- Java Optional Examples
- Java Static Initializer
- Java static Keyword
- Java Package: Import Keyword Example
- Java Do While Loop Examples
- Java Double Numbers: Double.BYTES and Double.SIZE
- Java Truncate Number: Cast Double to Int
- Java Padding: Pad Left and Right of Strings
- Java Anagram Example: HashMap and ArrayList
- Java Math.abs: Absolute Value
- Java Extends: Class Inheritance
- Java String Class
- Java String Switch Example: Switch Versus HashMap
- Java StringBuffer: append, Performance
- Java Array Examples
- Java Remove Duplicates From ArrayList
- Java if, else if, else Statements
- Java Math.ceil Method
- Java This Keyword
- Java PriorityQueue Example (add, peek and poll)
- Java Process.start EXE: ProcessBuilder Examples
- Java Palindrome Method
- Java parseInt: Convert String to Int
- Java toCharArray: Convert String to Array
- Java Caesar Cipher
- Java Array Length: Get Size of Array
- Java String Array Examples
- Java String compareTo, compareToIgnoreCase
- Java String Concat: Append and Combine Strings
- Java Cast and Convert Types
- Java Math.floor Method, floorDiv and floorMod
- Java Math Class: java.lang.Math
- Java While Loop Examples
- Java Reverse String
- Java Download Web Pages: URL and openStream
- Java Math.pow Method
- Java Math.round Method
- Java Right String Part
- Java MongoDB Example
- Java Substring Examples, subSequence
- Java Prime Number Method
- Java Sum Methods: IntStream and reduce
- Java switch Examples
- Java Convert HashMap to ArrayList
- Java Remove Duplicate Chars
- Java Constructor: Overloaded, Default, This Constructors
- Java String isEmpty Method (Null, Empty Strings)
- Java Regex Examples (Pattern.matches)
- Java ROT13 Method
- Java Random Number Examples
- Java Recursion Example: Count Change
- Java reflect: getDeclaredMethod, invoke
- Java Count Letter Frequencies
- Java ImmutableList Examples
- Java String equals, equalsIgnoreCase and contentEquals
- Java valueOf and copyValueOf String Examples
- Java Vector Examples
- Java Word Count Methods: Split and For Loop
- Java Tutorial | Learn Java Programming
- Java toLowerCase, toUpperCase Examples
- Java Ternary Operator
- Java Tree: HashMap and Strings Example
- Java TreeMap Examples
- Java while loop
- Java Convert String to Byte Array
- Java Join Strings: String.join Method
- Java Modulo Operator Examples
- Java Integer.MAX VALUE, MIN and SIZE
- Java Lambda Expressions
- Java lastIndexOf Examples
- Java Multiple Return Values
- Java String.format Examples: Numbers and Strings
- Java Joiner Examples: join
- Java Keywords
- Java Replace Strings: replaceFirst and replaceAll
- Java return Examples
- Java Multithreading Interview Questions (2021)
- Java Collections Interview Questions (2021)
- Java Shuffle Arrays (Fisher Yates)
- Top 30 Java Design Patterns Interview Questions (2021)
- Java ListMultimap Examples
- Java String Occurrence Method: While Loop Method
- Java StringBuilder capacity
- Java Math.max and Math.min
- Java Factory Design Pattern
- Java StringBuilder Examples
- Java Mail Tutorial
- Java Swing Tutorial
- Java AWT Tutorial
- Java Fibonacci Sequence Examples
- Java StringTokenizer Example
- Java Method Examples: Instance and Static
- Java String Between, Before, After
- Java BitSet Examples
- Java System.gc, Runtime.getRuntime and freeMemory
- Java Character Examples
- Java Char Lookup Table
- Java BufferedWriter Examples: Write Strings to Text File
- Java Abstract Class
- Java Hashtable
- Java Math class with Methods
- Java Whitespace Methods
- Java Data Types
- Java Trim String Examples (Trim Start, End)
- Java Exceptions: try, catch and finally
- Java vs C#
- Java Float Numbers
- Java Truncate String
- Java Variables
- Java For Loop Examples
- Java Uppercase First Letter
- Java Inner Class
- Java Networking
- Java Keywords
- Java If else
- Java Switch
- Loops in Java | Java For Loop
- Java File: BufferedReader and FileReader
- Java Random Lowercase Letter
- Java Calendar Examples: Date and DateFormat
- Java Case Keyword
- Java Char Array
- Java ASCII Table
- Java IntStream.Range Example (Get Range of Numbers)
- Java length Example: Get Character Count
- Java Line Count for File
- Java Sort Examples: Arrays.sort, Comparable
- Java LinkedHashMap Example
- Java Split Examples
Related Links
Adjectives
Ado
Ai
Android
Angular
Antonyms
Apache
Articles
Asp
Autocad
Automata
Aws
Azure
Basic
Binary
Bitcoin
Blockchain
C
Cassandra
Change
Coa
Computer
Control
Cpp
Create
Creating
C-Sharp
Cyber
Daa
Data
Dbms
Deletion
Devops
Difference
Discrete
Es6
Ethical
Examples
Features
Firebase
Flutter
Fs
Git
Go
Hbase
History
Hive
Hiveql
How
Html
Idioms
Insertion
Installing
Ios
Java
Joomla
Js
Kafka
Kali
Laravel
Logical
Machine
Matlab
Matrix
Mongodb
Mysql
One
Opencv
Oracle
Ordering
Os
Pandas
Php
Pig
Pl
Postgresql
Powershell
Prepositions
Program
Python
React
Ruby
Scala
Selecting
Selenium
Sentence
Seo
Sharepoint
Software
Spellings
Spotting
Spring
Sql
Sqlite
Sqoop
Svn
Swift
Synonyms
Talend
Testng
Types
Uml
Unity
Vbnet
Verbal
Webdriver
What
Wpf