<< Back to JAVA
Java Lambda Expressions
Invoke lambda expressions, creating instances of Functions, Suppliers and Consumers.Lambdas. We use this syntax are used to create function objects. We can specify methods inside other methods—and even pass methods as arguments to other methods.
A lambda has a shape, one determined by its parameters and return values (if any) and their types. Classes like Function, Supplier, Consumer, accept lambdas with specific shapes.
Example expression. This program creates a Function object from a lambda expression. The lambda expression accepts one argument, an Integer, and returns another Integer.
Left side: On the left of a lambda expression, we have the parameters. Two or more parameters can be surrounded by "(" and ")" chars.
Right side: This is the return expression—it is evaluated using the parameters. It is executed and, when required, returned.
Apply: In this program, we call apply() on the Function object. This executes and returns the expression—10 is changed to 20.
Java program that uses lambda expression
import java.util.function.*;
public class Program {
public static void main(String[] args) {
// Create a Function from a lambda expression.
// ... It returns the argument multiplied by two.
Function<Integer, Integer> func = x -> x * 2;
// Apply the function to an argument of 10.
int result = func.apply(10);
System.out.println(result);
}
}
Output
20
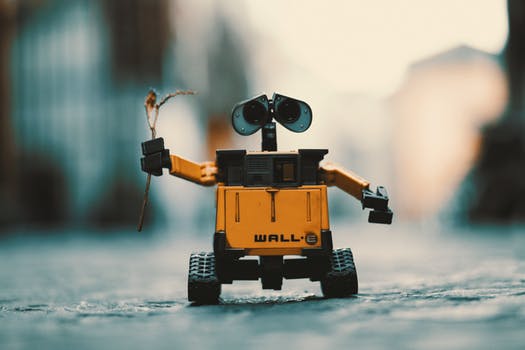
Supplier, lambda arguments. A Supplier object receives no arguments. We use an empty argument list to specify a lambda expression with no arguments.
Tip: A Supplier provides values. We call get() on it to retrieve its value—it may return different values when called more than once.
Java program that uses Supplier, lambdas
import java.util.function.*;
public class Program {
static void display(Supplier<Integer> arg) {
System.out.println(arg.get());
}
public static void main(String[] args) {
// Pass lambdas to the display method.
// ... These conform to the Supplier class.
// ... Each returns an Integer.
display(() -> 10);
display(() -> 100);
display(() -> (int) (Math.random() * 100));
}
}
Output
10
100
21
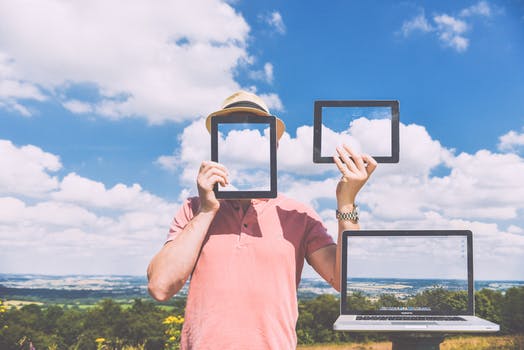
Predicate Lambda, ArrayList. The term predicate is used in computer science to mean a boolean-returning method. A Predicate object receives one value and returns true or false.
RemoveIf: This method on ArrayList receives a Predicate. Here, we remove all elements starting with the letter "c."
ArrayListJava program that uses removeIf, Predicate lambda
import java.util.ArrayList;
public class Program {
public static void main(String[] args) {
// Create ArrayList and add four String elements.
ArrayList<String> list = new ArrayList<>();
list.add("cat");
list.add("dog");
list.add("cheetah");
list.add("deer");
// Remove elements that start with c.
list.removeIf(element -> element.startsWith("c"));
System.out.println(list.toString());
}
}
Output
[dog, deer]
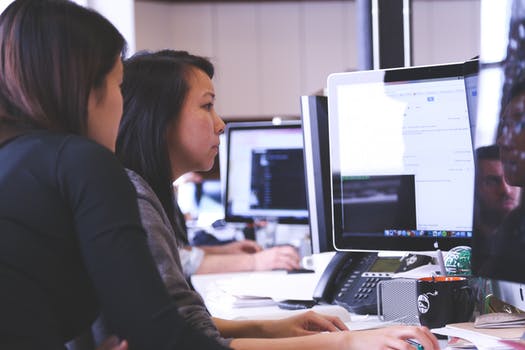
Consumer. Opposite a Supplier, a Consumer acts upon a value but returns nothing. It means a void method. We can use a consumer to call println or other void methods.
Also: A Consumer can be used to mutate data, as in an array, ArrayList or even just a class field.
Java program that uses Consumer
import java.util.function.*;
public class Program {
static void display(int value) {
switch (value) {
case 1:
System.out.println("There is 1 value");
return;
default:
System.out.println("There are " + Integer.toString(value)
+ " values");
return;
}
}
public static void main(String[] args) {
// This consumer calls a void method with the value.
Consumer<Integer> consumer = x -> display(x - 1);
// Use the consumer with three numbers.
consumer.accept(1);
consumer.accept(2);
consumer.accept(3);
}
}
Output
There are 0 values
There is 1 value
There are 2 values
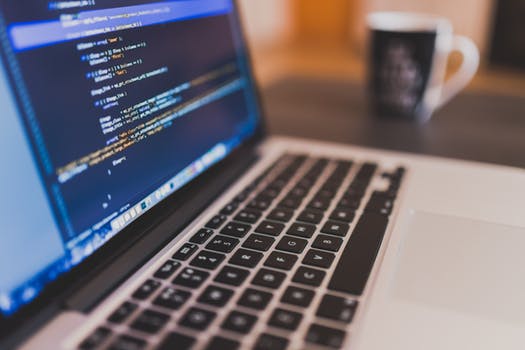
UnaryOperator. This functional object receives a value of a certain type (like Integer) and returns a same-typed value. So it operates on, and returns, a value.
Java program that uses UnaryOperator
import java.util.function.*;
public class Program {
public static void main(String[] args) {
// This returns one value of the same type as its one parameter.
// ... It means the same as the Function below.
UnaryOperator<Integer> operator = v -> v * 100;
// This is a generalized form of UnaryOperator.
Function<Integer, Integer> function = v -> v * 100;
System.out.println(operator.apply(5));
System.out.println(function.apply(6));
}
}
Output
500
600
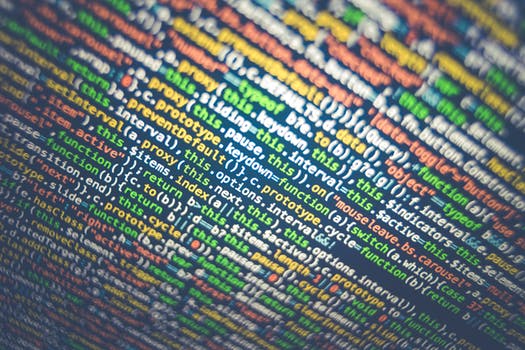
UnaryOperator, ArrayList. This example uses a lambda expression as a UnaryOperator argument to the ArrayList's replaceAll method. It adds ten to all elements.
ArrayList add, insertNote: The forEach method on ArrayList does not change element values. ReplaceAll allows this action.
Java program that uses replaceAll, UnaryOperator
import java.util.ArrayList;
public class Program {
public static void main(String[] args) {
// Add ten to each element in the ArrayList.
ArrayList<Integer> list = new ArrayList<>();
list.add(5);
list.add(6);
list.add(7);
list.replaceAll(element -> element + 10);
// ... Display the results.
System.out.println(list);
}
}
Output
[15, 16, 17]
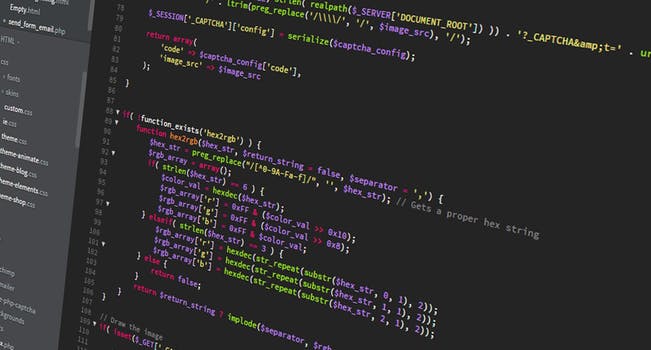
BiConsumer, HashMap. A BiConsumer is a functional object that receives two parameters. Here we use a BiConsumer in the forEach method on HashMap.
HashMapNote: The forEach lambda here, which is a valid BiConsumer, prints out all keys, values, and the keys' lengths.
Java program that uses BiConsumer, HashMap forEach
import java.util.HashMap;
public class Program {
public static void main(String[] args) {
HashMap<String, String> hash = new HashMap<>();
hash.put("cat", "orange");
hash.put("dog", "black");
hash.put("snake", "green");
// Use lambda expression that matches BiConsumer to display HashMap.
hash.forEach((string1, string2) -> System.out.println(string1 + "..."
+ string2 + ", " + string1.length()));
}
}
Output
cat...orange, 3
snake...green, 5
dog...black, 3
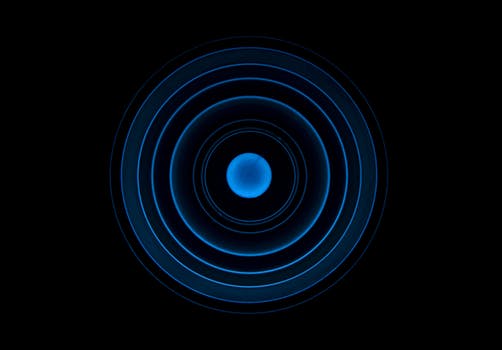
Identifiers. These do not matter in a lambda expression. The identifiers do not impact external parts of the program, but can be accessed on both sides of the lambda.
Note: As with variables, there is no reason to name the lambda expression variable a specific thing. Here we use the word "carrot."
Java program that uses unusual lambda identifier
import java.util.function.Consumer;
public class Program {
public static void main(String[] args) {
// The identifier in the lambda expression can be anything.
Consumer<Integer> consumer = carrot -> System.out.println(carrot);
consumer.accept(1989);
}
}
Output
1989
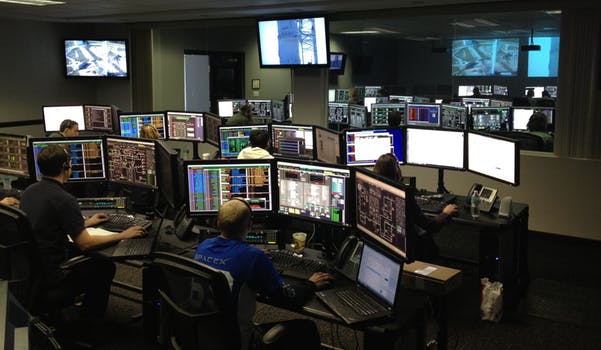
Benchmark, apply. Here we benchmark a Function object, which we invoke with apply(), against a static method. Both code blocks do the same thing.
Version 1: This version of the code uses a lambda expression and calls the apply() method to execute it many times.
Version 2: Here we invoke a method directly, using traditional Java syntax. This version is much faster.
Result: If a method can be called with no loss of code clarity, this may result in better performance over a lambda or functional object.
MethodsJava program that times Function apply, method call
import java.util.function.*;
public class Program {
static int method(int element) {
return element + 1;
}
public static void main(String[] args) {
Function<Integer, Integer> function = element -> element + 1;
long t1 = System.currentTimeMillis();
// Version 1: apply a function specified as a lambda expression.
for (int i = 0; i < 10000000; i++) {
int result = function.apply(i);
if (result == -1) {
System.out.println(false);
}
}
long t2 = System.currentTimeMillis();
// Version 2: call a static method.
for (int i = 0; i < 10000000; i++) {
int result = method(i);
if (result == -1) {
System.out.println(false);
}
}
long t3 = System.currentTimeMillis();
// ... Benchmark results.
System.out.println(t2 - t1);
System.out.println(t3 - t2);
}
}
Output
93 ms, Function apply()
6 ms, method call
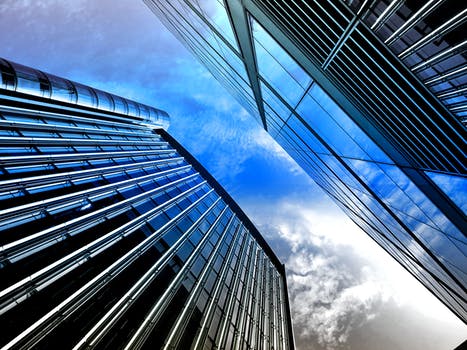
Filter. This method works on streams like IntStream. It returns a modified stream. And we can use methods like findFirst to access elements from filtered streams.
Filter
Sum. With a lambda expression, IntStream and the reduce() method we can sum an array. This approach has better parallel potential. But it is slow in simple cases.
Sum
Lambda calculus was introduced in 1936. In this form of mathematics, a function is an object. In a higher-order function, we pass a function object as an argument to a function.
Quote: Lambda calculus is a conceptually simple universal model of computation.
Lambda calculus: WikipediaA review. At first, functional object names in Java are confusing. What is a Supplier? What is a Consumer? What is supplying what, and who is consuming?
With practice, and some effort, the functional object system in this language is powerful and expressive. It is beautiful. It condenses syntax for complex logical forms.
Related Links:
- Java Continue Keyword
- Java Convert Char Array to String
- Java Combine Arrays
- Java Console Examples
- Java Web Services Tutorial
- Java Odd and Even Numbers: Modulo Division
- Java IO
- Java 9 Features
- Java 8 Features
- Java String
- Java Regex | Regular Expression
- Java Filename With Date Example (Format String)
- Java Applet Tutorial
- Java Files.Copy: Copy File
- Java filter Example: findFirst, IntStream
- Java Final and final static Constants
- Java Super: Parent Class
- Java Date and Time
- Java do while loop
- Java Break
- Java Continue
- Java Comments
- Java Splitter Examples: split, splitToList
- Java Math.sqrt Method: java.lang.Math.sqrt
- Java Reflection
- Java Convert String to int
- JDBC Tutorial | What is Java Database Connectivity(JDBC)
- Java main() method
- Java HashMap Examples
- Java HashSet Examples
- Java Arrays.binarySearch
- Java Integer.bitCount and toBinaryString
- Java Overload Method Example
- Java First Words in String
- Java Convert ArrayList to String
- Java Convert boolean to int (Ternary Method)
- Java regionMatches Example and Performance
- Java ArrayDeque Examples
- Java ArrayList add and addAll (Insert Elements)
- Java ArrayList Clear
- Java ArrayList int, Integer Example
- Java ArrayList Examples
- Java Boolean Examples
- Java break Statement
- Java Newline Examples: System.lineSeparator
- Java Stream: Arrays.stream and ArrayList stream
- Java charAt Examples (String For Loop)
- Java Programs | Java Programming Examples
- Java OOPs Concepts
- Java Naming Conventions
- Java Constructor
- Java Class Example
- Java indexOf Examples
- Java Collections.addAll: Add Array to ArrayList
- Java Compound Interest
- Java Int Array
- Java Interface Examples
- Java 2D Array Examples
- Java Remove HTML Tags
- Java Stack Examples: java.util.Stack
- Java Enum Examples
- Java EnumMap Examples
- Java StackOverflowError
- Java startsWith and endsWith Methods
- Java Initialize ArrayList
- Java Object Array Examples: For, Cast and getClass
- Java Objects, Objects.requireNonNull Example
- Java Optional Examples
- Java Static Initializer
- Java static Keyword
- Java Package: Import Keyword Example
- Java Do While Loop Examples
- Java Double Numbers: Double.BYTES and Double.SIZE
- Java Truncate Number: Cast Double to Int
- Java Padding: Pad Left and Right of Strings
- Java Anagram Example: HashMap and ArrayList
- Java Math.abs: Absolute Value
- Java Extends: Class Inheritance
- Java String Class
- Java String Switch Example: Switch Versus HashMap
- Java StringBuffer: append, Performance
- Java Array Examples
- Java Remove Duplicates From ArrayList
- Java if, else if, else Statements
- Java Math.ceil Method
- Java This Keyword
- Java PriorityQueue Example (add, peek and poll)
- Java Process.start EXE: ProcessBuilder Examples
- Java Palindrome Method
- Java parseInt: Convert String to Int
- Java toCharArray: Convert String to Array
- Java Caesar Cipher
- Java Array Length: Get Size of Array
- Java String Array Examples
- Java String compareTo, compareToIgnoreCase
- Java String Concat: Append and Combine Strings
- Java Cast and Convert Types
- Java Math.floor Method, floorDiv and floorMod
- Java Math Class: java.lang.Math
- Java While Loop Examples
- Java Reverse String
- Java Download Web Pages: URL and openStream
- Java Math.pow Method
- Java Math.round Method
- Java Right String Part
- Java MongoDB Example
- Java Substring Examples, subSequence
- Java Prime Number Method
- Java Sum Methods: IntStream and reduce
- Java switch Examples
- Java Convert HashMap to ArrayList
- Java Remove Duplicate Chars
- Java Constructor: Overloaded, Default, This Constructors
- Java String isEmpty Method (Null, Empty Strings)
- Java Regex Examples (Pattern.matches)
- Java ROT13 Method
- Java Random Number Examples
- Java Recursion Example: Count Change
- Java reflect: getDeclaredMethod, invoke
- Java Count Letter Frequencies
- Java ImmutableList Examples
- Java String equals, equalsIgnoreCase and contentEquals
- Java valueOf and copyValueOf String Examples
- Java Vector Examples
- Java Word Count Methods: Split and For Loop
- Java Tutorial | Learn Java Programming
- Java toLowerCase, toUpperCase Examples
- Java Ternary Operator
- Java Tree: HashMap and Strings Example
- Java TreeMap Examples
- Java while loop
- Java Convert String to Byte Array
- Java Join Strings: String.join Method
- Java Modulo Operator Examples
- Java Integer.MAX VALUE, MIN and SIZE
- Java Lambda Expressions
- Java lastIndexOf Examples
- Java Multiple Return Values
- Java String.format Examples: Numbers and Strings
- Java Joiner Examples: join
- Java Keywords
- Java Replace Strings: replaceFirst and replaceAll
- Java return Examples
- Java Multithreading Interview Questions (2021)
- Java Collections Interview Questions (2021)
- Java Shuffle Arrays (Fisher Yates)
- Top 30 Java Design Patterns Interview Questions (2021)
- Java ListMultimap Examples
- Java String Occurrence Method: While Loop Method
- Java StringBuilder capacity
- Java Math.max and Math.min
- Java Factory Design Pattern
- Java StringBuilder Examples
- Java Mail Tutorial
- Java Swing Tutorial
- Java AWT Tutorial
- Java Fibonacci Sequence Examples
- Java StringTokenizer Example
- Java Method Examples: Instance and Static
- Java String Between, Before, After
- Java BitSet Examples
- Java System.gc, Runtime.getRuntime and freeMemory
- Java Character Examples
- Java Char Lookup Table
- Java BufferedWriter Examples: Write Strings to Text File
- Java Abstract Class
- Java Hashtable
- Java Math class with Methods
- Java Whitespace Methods
- Java Data Types
- Java Trim String Examples (Trim Start, End)
- Java Exceptions: try, catch and finally
- Java vs C#
- Java Float Numbers
- Java Truncate String
- Java Variables
- Java For Loop Examples
- Java Uppercase First Letter
- Java Inner Class
- Java Networking
- Java Keywords
- Java If else
- Java Switch
- Loops in Java | Java For Loop
- Java File: BufferedReader and FileReader
- Java Random Lowercase Letter
- Java Calendar Examples: Date and DateFormat
- Java Case Keyword
- Java Char Array
- Java ASCII Table
- Java IntStream.Range Example (Get Range of Numbers)
- Java length Example: Get Character Count
- Java Line Count for File
- Java Sort Examples: Arrays.sort, Comparable
- Java LinkedHashMap Example
- Java Split Examples
Related Links
Adjectives
Ado
Ai
Android
Angular
Antonyms
Apache
Articles
Asp
Autocad
Automata
Aws
Azure
Basic
Binary
Bitcoin
Blockchain
C
Cassandra
Change
Coa
Computer
Control
Cpp
Create
Creating
C-Sharp
Cyber
Daa
Data
Dbms
Deletion
Devops
Difference
Discrete
Es6
Ethical
Examples
Features
Firebase
Flutter
Fs
Git
Go
Hbase
History
Hive
Hiveql
How
Html
Idioms
Insertion
Installing
Ios
Java
Joomla
Js
Kafka
Kali
Laravel
Logical
Machine
Matlab
Matrix
Mongodb
Mysql
One
Opencv
Oracle
Ordering
Os
Pandas
Php
Pig
Pl
Postgresql
Powershell
Prepositions
Program
Python
React
Ruby
Scala
Selecting
Selenium
Sentence
Seo
Sharepoint
Software
Spellings
Spotting
Spring
Sql
Sqlite
Sqoop
Svn
Swift
Synonyms
Talend
Testng
Types
Uml
Unity
Vbnet
Verbal
Webdriver
What
Wpf