C++ Forward Iterator
- Forward Iterator is a combination of Bidirectional and Random Access iterator. Therefore, we can say that the forward iterator can be used to read and write to a container.
- Forward iterators are used to read the contents from the beginning to the end of a container.
- Forward iterator use only increments operator (++) to move through all the elements of a container. Therefore, we can say that the forward iterator can only move forward.
- A Forward iterator is a multi-pass iterator.
Operations Performed on the Forward Iterator:
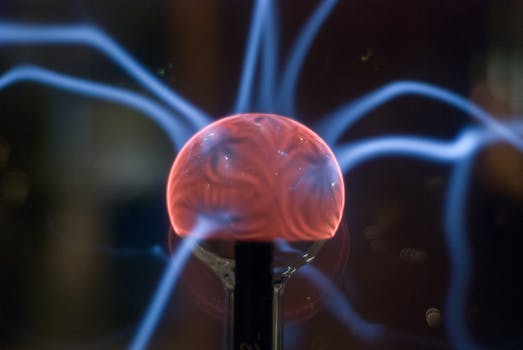
Properties |
Valid Expressions |
It is default constructible. |
A x; |
It is a copy-constructible. |
A x(y); |
It is a copy-assignable. |
y = x; |
It can be compared either by using an equality or inequality operator. |
a==b;
a!=b; |
It can be incremented. |
a++;
++a; |
It can be dereferenced as an rvalue. |
*a; |
It can also be dereferenced as an lvalue. |
*a = t; |
Where 'A' is a forward iterator type, and x and y are the objects of a forward iterator type, and t is an object pointed by the iterator type object.
Let's see a simple example:
#include <iostream>
#include <fstream>
#include <iterator>
#include <vector>
using namespace std;
template<class ForwardIterator> // function template
void display(ForwardIterator first, ForwardIterator last) // display function
{
while(first!=last)
{
cout<<*first<<" ";
first++;
}
}
int main()
{
vector<int> a; // declaration of vector.
for(int i=1;i<=10;i++)
{
a.push_back(i);
}
display(a.begin(),a.end()); // calling display() function.
return 0;
}
Output:
Features of the Forward Iterator:
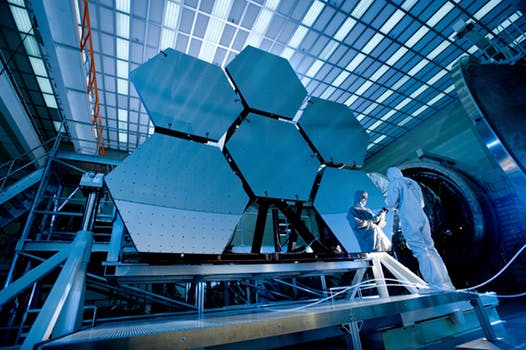
- Equality/Inequality operator: A forward iterator can be compared by using equality or an inequality operator.
Suppose 'A' and 'B' are the two iterators:
A==B; // equality operator
A!=B; // inequality operator
- Dereferencing: We can dereference the forward iterator as an rvalue as well as an lvalue. Therefore, we can access the output iterator and can also assign the value to the output iterator.
Suppose 'A' is an iterator and 't' is an integer variable:
- Incrementable: A forward iterator can be incremented but cannot be decremented.
Suppose 'A' is an iterator:
Limitations of the Forward Iterator:
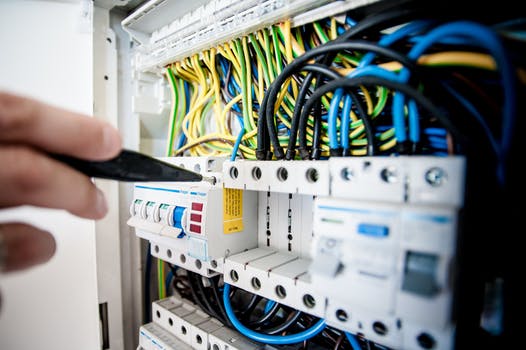
- Decrementable: A forward iterator cannot be decremented as it moves only in the forward direction.
Suppose 'A' is an iterator:
- Relational Operators: A forward iterator can be used with the equality operator, but no other relational operators can be applied on the forward iterator.
Suppose 'A' and 'B' are the two iterators:
A==B; // valid
A>=B; // invalid
- Arithmetic Operators: An arithmetic operators cannot be used with the forward iterator.
A+2; // invalid
A+3; // invalid
- Random Access: A forward iterator does not provide the random access of an element. It can only iterate through the elements of a container.
|