C++ Tutorial
C++ tutorial provides basic and advanced concepts of C++. Our C++ tutorial is designed for beginners and professionals.
C++ is an object-oriented programming language. It is an extension to C programming.
Our C++ tutorial includes all topics of C++ such as first example, control statements, objects and classes, inheritance, constructor, destructor, this, static, polymorphism, abstraction, abstract class, interface, namespace, encapsulation, arrays, strings, exception handling, File IO, etc.
What is C++
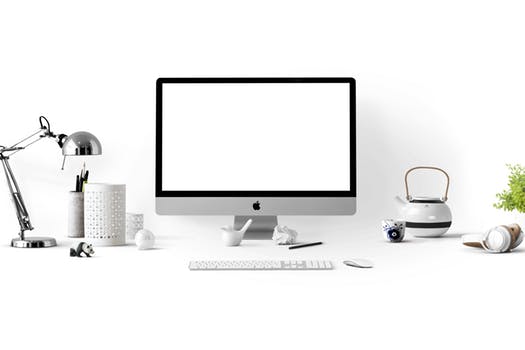
C++ is a general purpose, case-sensitive, free-form programming language that supports object-oriented, procedural and generic programming.
C++ is a middle-level language, as it encapsulates both high and low level language features.
Object-Oriented Programming (OOPs)
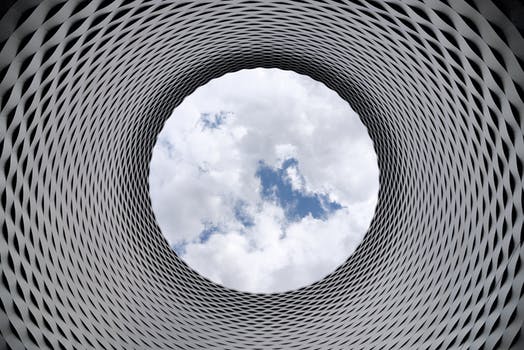
C++ supports the object-oriented programming, the four major pillar of object-oriented programming (OOPs) used in C++ are:
- Inheritance
- Polymorphism
- Encapsulation
- Abstraction
C++ Standard Libraries
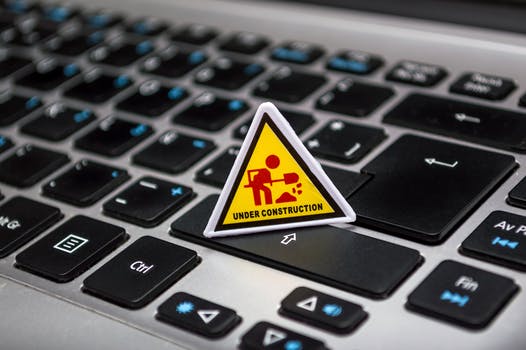
Standard C++ programming is divided into three important parts:
- The core library includes the data types, variables and literals, etc.
- The standard library includes the set of functions manipulating strings, files, etc.
- The Standard Template Library (STL) includes the set of methods manipulating a data structure.
Usage of C++
By the help of C++ programming language, we can develop different types of secured and robust applications:
- Window application
- Client-Server application
- Device drivers
- Embedded firmware etc
C++ Program
In this tutorial, all C++ programs are given with C++ compiler so that you can easily change the C++ program code.
File: main.cpp
#include
using namespace std;
int main() {
cout << "Hello C++ Programming";
return 0;
}
A detailed explanation of first C++ program is given in next chapters.
C++ Index
C++ Tutorial
C++ Control Statement
C++ Functions
C++ Arrays
C++ Pointers
C++ Object Class
C++ Inheritance
C++ Polymorphism
C++ Abstraction
C++ Namespaces
C++ Templates
C++ Strings
C++ Exceptions
C++ File & Stream
C++ Programs
C++ STL Tutorial
C++ STL Bitset
C++ STL Deque
C++ STL List
C++ STL Map
C++ STL Math
C++ STL priority_queue
C++ STL Stack
C++ STL Queue
C++ STL Multiset
C++ STL Multimap
C++ STL Set
C++ STD Strings
C++ STL Vector
Interview Questions
Prerequisite
Before learning C++, you must have the basic knowledge of C.
Audience
Our C++ tutorial is designed to help beginners and professionals.
Problem
We assure that you will not find any problem in this C++ tutorial. But if there is any mistake, please post the problem in contact form.
|