C++ STL Set
Introduction to set
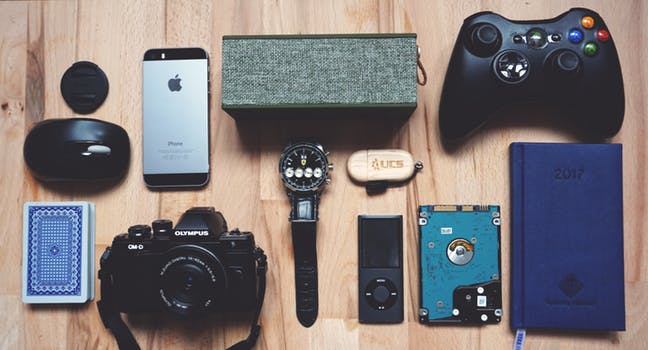
Sets are part of the C++ STL (Standard Template Library). Sets are the associative containers that stores sorted key, in which each key is unique and it can be inserted or deleted but cannot be altered.
Syntax
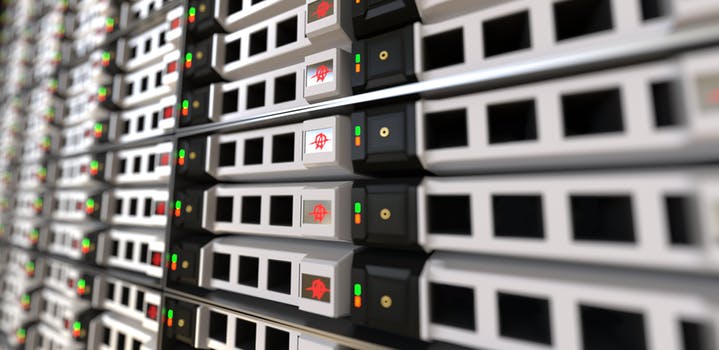
template < class T, // set::key_type/value_type
class Compare = less<T>, // set::key_compare/value_compare
class Alloc = allocator<T> // set::allocator_type
> class set;
Parameter
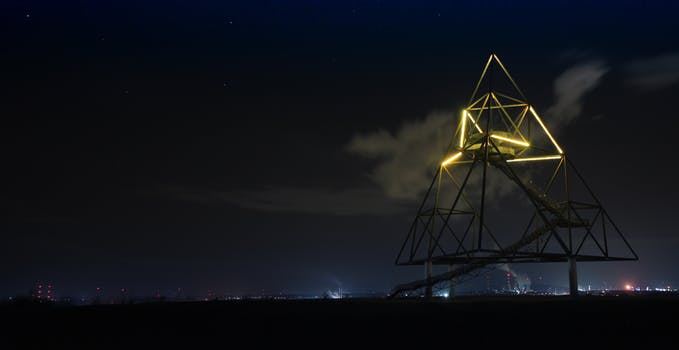
T: Type of element stored in the container set.
Compare: A comparison class that takes two arguments of the same type bool and returns a value. This argument is optional and the binary predicate less<T>, is the default value.
Alloc: Type of the allocator object which is used to define the storage allocation model.
Member Functions
Below is the list of all member functions of set:
Constructor/Destructor
Iterators
Functions |
Description |
Begin |
Returns an iterator pointing to the first element in the set. |
cbegin |
Returns a const iterator pointing to the first element in the set. |
End |
Returns an iterator pointing to the past-end. |
Cend |
Returns a constant iterator pointing to the past-end. |
rbegin |
Returns a reverse iterator pointing to the end. |
Rend |
Returns a reverse iterator pointing to the beginning. |
crbegin |
Returns a constant reverse iterator pointing to the end. |
Crend |
Returns a constant reverse iterator pointing to the beginning. |
Capacity
Functions |
Description |
empty |
Returns true if set is empty. |
Size |
Returns the number of elements in the set. |
max_size |
Returns the maximum size of the set. |
Modifiers
Functions |
Description |
insert |
Insert element in the set. |
Erase |
Erase elements from the set. |
Swap |
Exchange the content of the set. |
Clear |
Delete all the elements of the set. |
emplace |
Construct and insert the new elements into the set. |
emplace_hint |
Construct and insert new elements into the set by hint. |
Observers
Functions |
Description |
key_comp |
Return a copy of key comparison object. |
value_comp |
Return a copy of value comparison object. |
Operations
Functions |
Description |
Find |
Search for an element with given key. |
count |
Gets the number of elements matching with given key. |
lower_bound |
Returns an iterator to lower bound. |
upper_bound |
Returns an iterator to upper bound. |
equal_range |
Returns the range of elements matches with given key. |
Allocator
Functions |
Description |
get_allocator |
Returns an allocator object that is used to construct the set. |
Non-Member Overloaded Functions
Functions |
Description |
operator== |
Checks whether the two sets are equal or not. |
operator!= |
Checks whether the two sets are equal or not. |
operator< |
Checks whether the first set is less than other or not. |
operator<= |
Checks whether the first set is less than or equal to other or not. |
operator> |
Checks whether the first set is greater than other or not. |
operator>= |
Checks whether the first set is greater than equal to other or not. |
swap() |
Exchanges the element of two sets. |
|