<< Back to PYTHON
Python If Examples: Elif, Else
Use the if, elif and else-statements. Write expressions and nested ifs.If, else. The bee moves through the warm air. A tree's leaves shift in the wind. The bee makes many decisions. It judges each one to optimize its outcome.
With an if-statement, we test a condition. Like the bee we make a decision. With keywords (and, not, or) we modify the expressions within an if.
An example. An if-statement can be followed by an elif-statement and an else-statement. The if can stand alone—we do not need to have any other parts following it.
If: This is followed by a colon character. Its expression is evaluated to true or false.
Elif: This is the same as if, but it must come after if and before else. It can come at the end.
Else: It must come at the end. It has no expression to evaluate. It is executed when if and elif evaluate to false.
Tip: Blocks of code within if, elif and else scopes must have an additional level of indentation. This program uses 4 spaces.
Python program that uses if-statements
def test(n):
if n == 0:
print("None")
elif n < 0:
print("Low")
else:
# Handle values greater than zero.
if n > 100:
print("Out of range")
else:
print("High")
test(-1)
test(0)
test(1)
test(1000)
Output
Low
None
High
Out of range
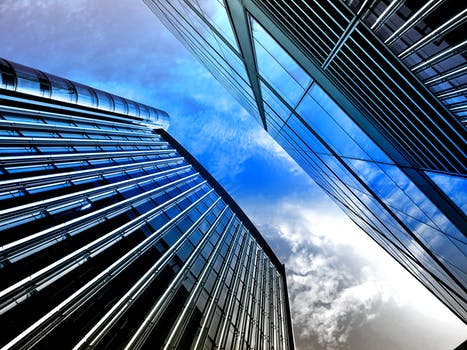
Same line. An if-statement can be on the same line as its body. This only works if there is one only statement in the body. A syntax error occurs otherwise.
Often: Indenting the statements, as in this example, is visually helpful. This improves readability.
Tip: You can mix same-line statements with multiple-line blocks in the same if-else chain. The goal is easy-to-read code.
Python program that uses same-line if
# A variable.
a = 1
# Test variable.
if a == 1: print("1")
elif a == 2: print("2")
Output
1
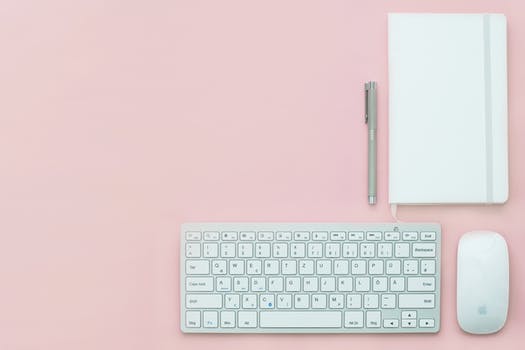
In keyword. Collections such as dictionaries can be used with the if-statement. With a dictionary, the in keyword is used in an if-statement. We test the collection for a key's existence.
InPython program that uses if with dictionary
birds = {"parakeet": 1, "parrot": 2}
if "parrot" in birds:
print("Found")
Output
Found
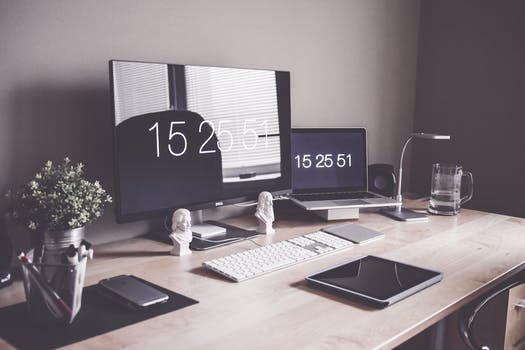
And, or. The && and || operators are not available in Python. We use instead the "and" and "or" keywords. This syntax is easier to read. We can use parentheses to form expressions.
Note: These if-statements have expressions that use "and" or "or." They all evaluate to true, and the inner print statements are reached.
Tip: Expressions can be even more complex. And you can call methods and use them instead of variables.
Tip 2: If-statements can also be nested. And sometimes it is possible to change complex expressions into a simpler if-else chain.
Python program that uses logical operators
a = 1
b = 2
if a == 1 and b == 2:
print(True)
if a == 0 or b == 2:
print(True)
if not (a == 1 and b == 3):
print(True)
if a != 0 and b != 3:
print(True)
Output
True
True
True
True
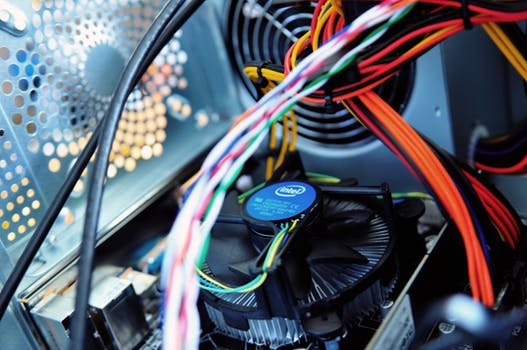
Not keyword. This is used in ifs. It causes false expressions to evaluate to true ones. It comes before some expressions, but for "in" expressions, we use the "not in" construct.
NotCaution: For the first example of "not" here, I recommend instead using the != operator. This style is more familiar to many programmers.
Python program that uses not
number = 1
# See if number is not equal to 2.
if not number == 2:
print("True")
birds = {"cormorant" : 1, "cardinal" : 2}
# See if string not in collection.
if "automobile" not in birds:
print("Not found")
Output
True
Not found
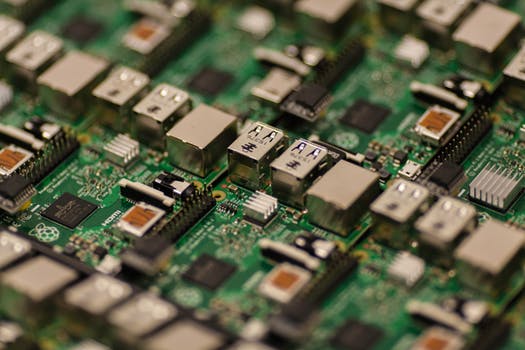
Store expressions. If-statements can become complex. Often repetition is at fault. The same variables, in the same expressions, are tested many times.
Result: We can store the result of an expression in a variable. Then, we test the variable.
Tip: This reduces repetition. It may also improve processing speed: fewer comparisons are done.
Here: We store the result of an expression in the "all" variable. We then test it twice.
Python program that stores expression result, variable
a = 1
b = 2
c = 3
# Store result in expression.
all = a == 1 and b == 2 and c == 3
# Test variable.
if all:
print(1)
# Use it again.
if all:
print(2)
Output
1
2
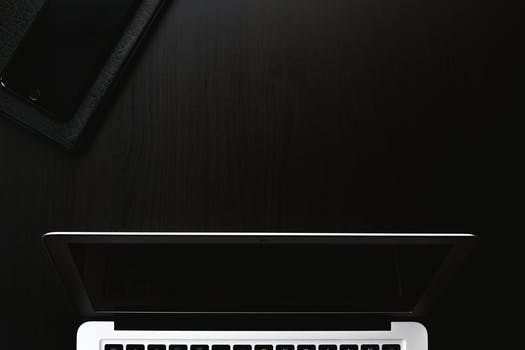
Tuple. The if-statement is helpful with a tuple. In this example, we use a tuple directly inside the if-statement. The method test() prints "Match" if its argument is 0, 2 or 4.
TuplePython program that uses tuple, if-statement
def test(x):
# Test x with tuple syntax.
if x in (0, 2, 4):
print("Match")
else:
print("Nope")
test(0)
test(1)
test(2)
Output
Match x = 0
Nope x = 1
Match x = 2
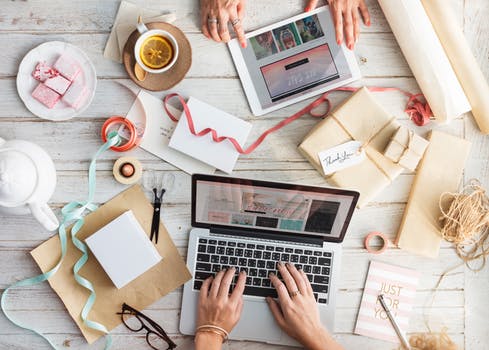
Truth. Let us consider the truth of things. Python considers some values True, in an if-statement, and others false. The "None" value is False, and a class instance is True.
Negative 1: This evaluates to True. Nonzero numbers, like -1 and 1 are considered True.
Zero: This is False. Zero is the only False integer—all others, negative and positive, are considered True.
None: This is also False. Many methods return None when they find no answer. We can directly test these in an if.
Class: A Class instance is considered True. So if we test a new class instance, we will enter the if-block.
Empty list: The [] characters denote an empty list. Python evaluates zero-element collections to False.
Python program that tests values for true or false
# An empty class for testing.
class Box:
pass
# Some values to test.
values = [-1, 0, 1, None, Box(), []]
# See if the values are treated as "true" or "false".
for value in values:
if value: print("True ", end="")
else: print("False", end="")
# Display value.
print("... ", value)
Output
True ... -1
False... 0
True ... 1
False... None
True ... <__main__.Box object at 0x01984f50>
False... []
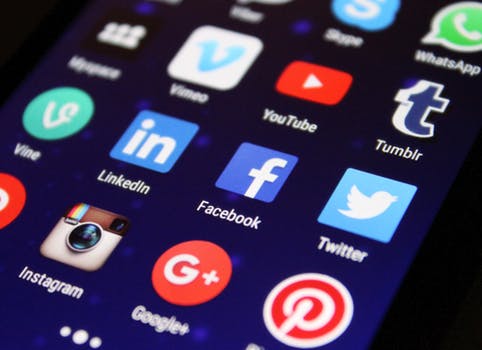
True, False. Programs can always access the True and False constants. We can use True and False in ifs to make our logic clearer. This code makes explicit what value we are comparing.
Python program that uses True, False
value1 = 10
value2 = 10
# See if these two values are equal.
equal = value1 == value2
# Test True and False constants.
if equal == True: print(1)
if equal != False: print(2)
Output
1
2
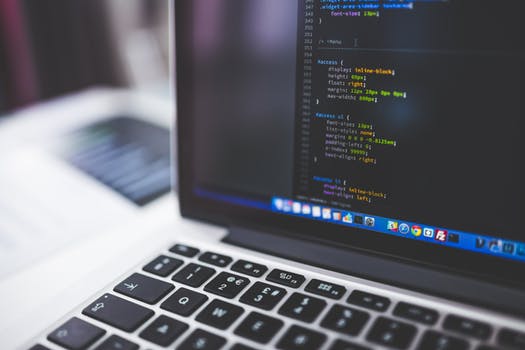
Method. Often we test variables directly in if-statements. But we can test the result of methods. This can simplify complex logic. Here, we invoke result() in if-statements.
Caution: For predicate methods, where a truth value is returned, side effects (like a field assignment) can lead to confusing code.
Python program that uses method in if
def result(a):
if a == 10:
return True
# Use method in an if-statement condition.
if result(10):
print("A")
if not result(5):
print("B")
Output
A
B
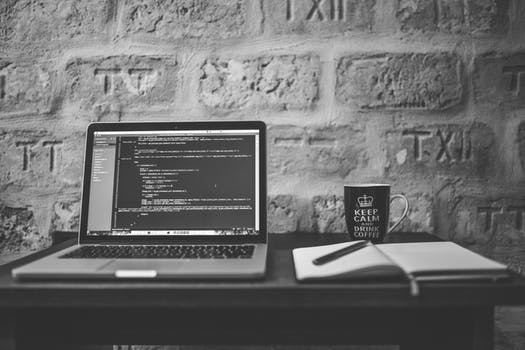
Is-keyword. This tests identity. Every object (like integers, classes) has an id. When the ids of two objects are equal, their identities too are equal. And the is-test will return True.
Quote: The operators "is" and "is not" test for object identity: "x is y" is true if and only if "x" and "y" are the same object (Python help).
Python program that uses is
class Bird:
pass
# Create two separate objects.
owl = Bird()
parrot = Bird()
# The objects are not identical.
if owl is not parrot:
print(False, id(owl), id(parrot))
# These objects are identical.
copy = owl
if copy is owl:
print(True, id(copy), id(owl))
Output
False 4353337736 4353337792
True 4353337736 4353337736
SyntaxError. When comparing two values in an if-statement, two equals signs are required. Python reports a compile-time error when only one is found. One equals sign means an assignment.
Note: The programs with this problem can never be executed. This is helpful: it prevents invalid programs from causing a headache.
Python program that causes SyntaxError
value = 100
# This should have two equals signs!
if value = 1000:
print(True)
Output
File "C:\programs\file.py", line 6
if value = 1000:
^
SyntaxError: invalid syntax
Reorder. The and-operator short-circuits. This means when a sub-expression evaluates to false, no further ones are evaluated. We can exploit this principle to improve speed.
Here: A list contains four sub-lists. In the value pairs, the first element differs most: there are three unique first numbers.
Value 2: This is always 1 in the pairs. It is least specific when checking the pairs.
So: To find pairs with both values set to 1, we should first check the most specific value—the first one.
Python program that reorders if-checks
values = [[1, 1], [2, 1], [1, 1], [3, 1]]
for pair in values:
# We test the first part of each list first.
# ... It is most specific.
# ... This reduces total checks.
if pair[0] == 1 and pair[1] == 1:
print(True)
Output
True
True
Two comparisons. We can use two greater-than or less-than operators in a single expression. So we can see if a number is between 6 and 4.
Also: The "greater than or equal to" operator (and less than or equal to) are available here.
Python program that uses two comparisons
value = 5
# Use two comparison operators to test for a range of values.
if 6 > value > 4:
print("Value is between 6 and 4")
if 4 < value < 10:
print("Value is between 4 and 10")
Output
Value is between 6 and 4
Value is between 4 and 10
A benchmark. If-statements can be written in many ways. A tuple can be used inside of an if-statement condition to replace (and optimize) a group of if-else branches.
Version 1: We test an if that checks a variable against a tuple of 3 values. Only one if-statement is needed.
Version 2: We then time an if-elif construct that does the same thing. Multiple lines of Python code are required here.
Result: The syntax that uses (1, 2, 3) was faster than the form that uses if and two elifs. The tuple-style syntax is efficient.
Python program that benchmarks if, tuple
import time
print(time.time())
# Version 1: use tuple.
v = 0
i = 0
x = 2
while i < 10000000:
if x in (1, 2, 3):
v += 1
i += 1
print(time.time())
# Version 2: use if-elif.
v = 0
i = 0
while i < 10000000:
if x == 1:
v += 1
elif x == 2:
v += 1
elif x == 3:
v += 1
i += 1
print(time.time())
Output
1361579543.768
1361579547.067 Tuple syntax = 3.23 s
1361579550.634 If-elif = 3.57 s
Control flow is a key concept in programs. Constructs such as loops and the if-statement manipulate control flow inside methods. And methods themselves influence flow outside this scope.
A summary. These control flow statements are used in nearly every program. The else-statement is used with loop constructs. If a loop never iterates once, the else is reached.
Related Links:
- Python global and nonlocal
- Python not: If Not True
- Python Convert Decimal Binary Octal and Hexadecimal
- Python Tkinter Scale
- Python Tkinter Scrollbar
- Python Tkinter Text
- Python History
- Python Number: random, float and divmod
- Python Tkinter Toplevel
- Python Tkinter Spinbox
- Python Tkinter PanedWindow
- Python Tkinter LabelFrame
- Python Tkinter MessageBox
- Python Website Blocker
- Python Console Programs: Input and Print
- Python Display Calendar
- Python Check Number Odd or Even
- Python readline Example: Read Next Line
- Python Anagram Find Method
- Python Any: Any Versus All, List Performance
- Python Filename With Date Example (date.today)
- Python Find String: index and count
- Python filter (Lambda Removes From List or Range)
- Python ASCII Value of Character
- Python Sum Example
- Python make simple Calculator
- Python Add Two Matrices
- Python Multiply Two Matrices
- Python SyntaxError (invalid syntax)
- Python Transpose Matrix
- Python Remove Punctuation from String
- Python Dictionary items() method with Examples
- Python Dictionary keys() method with Examples
- Python Textwrap Wrap Example
- Python Dictionary popitem() method with Examples
- Python Dictionary pop() method with Examples
- Python HTML: HTMLParser, Read Markup
- Python Tkinter Tutorial
- Python Array Examples
- Python ord, chr Built Ins
- Python Dictionary setdefault() method with Examples
- Python Dictionary update() method with Examples
- Python Dictionary values() method with Examples
- Python complex() function with Examples
- Python delattr() function with Examples
- Python dir() function with Examples
- Python divmod() function with Examples
- Python Loops
- Python for loop
- Python while loop
- Python enumerate() function with Examples
- Python break
- Python continue
- Python dict() function with Examples
- Python pass
- Python Strings
- Python Lists
- Python Tuples
- Python Sets
- Python Built-in Functions
- Python filter() function with Examples
- Python dict Keyword (Copy Dictionary)
- Python Dictionary Order Benchmark
- Python Dictionary String Key Performance
- Python 2D Array: Create 2D Array of Integers
- Python Divmod Examples, Modulo Operator
- bin() in Python | Python bin() Function with Examples
- Python Oops Concept
- Python Object Classes
- Python Constructors
- Python hash() function with Examples
- Python Pandas | Python Pandas Tutorial
- Python Class Examples: Init and Self
- Python help() function with Examples
- Python IndentationError (unexpected indent)
- Python Index and Count (Search List)
- Python min() function with Examples
- Python classmethod and staticmethod Use
- Python set() function with Examples
- Python hex() function with Examples
- Python id() function with Examples
- Python sorted() function with Examples
- Python next() function with Examples
- Python Compound Interest
- Python List insert() method with Examples
- Python Datetime Methods: Date, Timedelta
- Python setattr() function with Examples
- Python 2D List Examples
- Python Pandas Data operations
- Python Def Methods and Arguments (callable)
- Python slice() function with Examples
- Python Remove HTML Tags
- Python input() function with Examples
- Python enumerate (For Index, Element)
- Python Display the multiplication Table
- Python int() function with Examples
- Python Error: Try, Except and Raise
- Python isinstance() function with Examples
- Python oct() function with Examples
- Python startswith, endswith Examples
- Python List append() method with Examples
- Python NumPy Examples (array, random, arange)
- Python Replace Example
- Python List clear() method with Examples
- Python List copy() method with Examples
- Python Lower Dictionary: String Performance
- Python Lower and Upper: Capitalize String
- Python Dictionary Examples
- Python map Examples
- Python Len (String Length)
- Python Padding Examples: ljust, rjust
- Python Type: setattr and getattr Examples
- Python String List Examples
- Python String
- Python Remove Duplicates From List
- Python If Examples: Elif, Else
- Python Programs | Python Programming Examples
- Python List count() method with Examples
- Python List extend() method with Examples
- Python List index() method with Examples
- Python List pop() method with Examples
- Python Palindrome Method: Detect Words, Sentences
- Python Path: os.path Examples
- Python List remove() method with Examples
- Python List reverse() method with Examples
- Top 50+ Python Interview Questions (2021)
- Python List sort() method with Examples
- Python sort word in Alphabetic Order
- abs() in Python | Python abs() Function with Examples
- Python String | encode() method with Examples
- all() in Python | Python all() Function with Examples
- any() in Python | Python any() Function with Examples
- Python Built In Functions
- ascii() in Python | Python ascii() Function with Examples
- Python bytes, bytearray Examples (memoryview)
- bool() in Python | Python bool() Function with Examples
- bytearray() in Python | Python bytearray() Function with Examples
- Python Caesar Cipher
- bytes() in Python | Python bytes() Function with Examples
- Python Sum of Natural Numbers
- callable() in Python | Python callable() Function with Examples
- Python Set add() method with Examples
- Python Set discard() method with Examples
- Python Set pop() method with Examples
- Python math.floor, import math Examples
- Python Return Keyword (Return Multiple Values)
- Python while Loop Examples
- Python Math Examples
- Python Reverse String
- Python max, min Examples
- Python pass Statement
- Python Set remove() method with Examples
- Python Dictionary
- Python Functions
- Python String | capitalize() method with Examples
- Python String | casefold() method with Examples
- Python re.sub, subn Methods
- Python subprocess Examples: subprocess.run
- Python Tkinter Checkbutton
- Python Tkinter Entry
- Python String | center() method with Examples
- Python Substring Examples
- Python pow Example, Power Operator
- Python Lambda
- Python Files I/O
- Python Modules
- Python String | count() method with Examples
- Python String | endswith() method with Examples
- Python String | expandtabs() method with Examples
- Python Prime Number Method
- Python String | find() method with Examples
- Python String | format() method with Examples
- Python String | index() method with Examples
- Python String | isalnum() method with Examples
- Python String | isalpha() method with Examples
- Python String | isdecimal() method with Examples
- Python Pandas Sorting
- Python String | isdigit() method with Examples
- Python Convert Types
- Python String | isidentifier() method with Examples
- Python Pandas Add column to DataFrame columns
- Python String | islower() method with Examples
- Python Pandas Reading Files
- Python Right String Part
- Python IOError Fix, os.path.exists
- Python Punctuation and Whitespace (string.punctuation)
- Python isalnum: String Is Alphanumeric
- Python Pandas Series
- Python Pandas DataFrame
- Python Recursion Example
- Python ROT13 Method
- Python StringIO Examples and Benchmark
- Python Import Syntax Examples: Modules, NameError
- Python in Keyword
- Python iter Example: next
- Python Round Up and Down (Math Round)
- Python List Comprehension
- Python Collection Module
- Python Math Module
- Python OS Module
- Python Random Module
- Python Statistics Module
- Python String Equals: casefold
- Python Sys Module
- Top 10 Python IDEs | Python IDEs
- Python Arrays
- Python Magic Method
- Python Stack and Queue
- Python MySQL Environment Setup
- Python MySQL Database Connection
- Python MySQL Creating New Database
- Python MySQL Creating Tables
- Python Word Count Method (re.findall)
- Python String Literal: F, R Strings
- Python MySQL Update Operation
- Python MySQL Join Operation
- Python Armstrong Number
- Learn Python Tutorial
- Python Factorial Number using Recursion
- Python Features
- Python Comments
- Python if else
- Python Translate and Maketrans Examples
- Python Website Blocker | Building Python Script
- Python Itertools Module: Cycle and Repeat
- Python Operators
- Python Int Example
- Python join Example: Combine Strings From List
- Python Read CSV File
- Python Write CSV File
- Python Read Excel File
- Python Write Excel File
- Python json: Import JSON, load and dumps
- Python Lambda Expressions
- Python Print the Fibonacci sequence
- Python format Example (Format Literal)
- Python Namedtuple Example
- Python SciPy Tutorial
- Python Applications
- Python KeyError Fix: Use Dictionary get
- Python Resize List: Slice and Append
- Python String | translate() method with Examples
- Python Copy List (Slice Entire List)
- Python None: TypeError, NoneType Has No Length
- Python MySQL Performing Transactions
- Python String | isnumeric() method with Examples
- Python MongoDB Example
- Python String | isprintable() method with Examples
- Python Tkinter Canvas
- Python String | isspace() method with Examples
- Python Tkinter Frame
- Python Tkinter Label
- Python Tkinter Listbox
- Python String | istitle() method with Examples
- Python Website Blocker | Script Deployment on Linux
- Python Website Blocker | Script Deployment on Windows
- Python String | isupper() method with Examples
- Python String split() method with Examples
- Python Slice Examples: Start, Stop and Step
- Python String | join() method with Examples
- Python String | ljust() method with Examples
- Python Sort by File Size
- Python Arithmetic Operations
- Python String | lower() method with Examples
- Python Exception Handling | Python try except
- Python Date
- Python Regex | Regular Expression
- Python Sending Email using SMTP
- Python Command Line Arguments
- Python List Comprehension Examples
- Python Assert Keyword
- Python Set Examples
- Python Fibonacci Sequence
- Python Maze Pathfinding Example
- Python Memoize: Dictionary, functools.lru_cache
- Python Timeit, Repeat Examples
- Python Strip Examples
- Python asyncio Example: yield from asyncio.sleep
- Python String Between, Before and After Methods
- Python bool Use (Returns True or False)
- Python Counter Example
- Python frozenset: Immutable Sets
- Python Generator Examples: Yield, Expressions
- Python CSV: csv.reader and Sniffer
- Python globals, locals, vars and dir
- Python abs: Absolute Value
- Python gzip: Compression Examples
- Python Function Display Calendar
- Python Display Fibonacci Sequence Recursion
- Python String | lstrip() method with Examples
- Python del Operator (Remove at Index or Key)
- Python String | partition() method with Examples
- Python String | replace() method with Examples
- Python Zip Examples: Zip Objects
- Python String | rfind() method with Examples
- Python String | rindex() method with Examples
- Python String rjust() method with Examples
- Python String rpartition() method with Examples
- Python String rsplit() method with Examples
- Python Area Of Triangle
- Python Quadratic Equation
- Python swap two Variables
- Python Generate Random Number
- Python Convert Kilometers to Miles
- Python Convert Celsius to Fahrenheit
- Python Check Number Positive Negative or Zero
- Python Check Leap Year
- Python Check Prime Number
- Top 40 Python Pandas Interview Questions (2021)
- Python Check Armstrong Number
- Python SQLite Example
- Python Tkinter Button
- Python Find LCM
- Python Find HCF
- Python Tuple Examples
- Python String | rstrip() method with Examples
- Python String splitlines() method with Examples
- Python String | startswith() method with Examples
- Python String | swapcase() method with Examples
- Python Truncate String
- Python String | upper() method with Examples
- Python for: Loop Over String Characters
- Python String | zfill() method with Examples
- Python Sort Examples: Sorted List, Dictionary
- Python XML: Expat, StartElementHandler
- Python Urllib Usage: Urlopen, UrlParse
- Python File Handling (with open, write)
- Python Example
- Python variables
- Python Random Numbers: randint, random.choice
- Python assert, O Option
- Python Data Types
- Python keywords
- Python literals
- Python MySQL Insert Operation
- Python MySQL Read Operation
- Python ascii Example
- Python ASCII Table Generator: chr
- Python Range: For Loop, Create List From Range
- Python re.match Performance
- Python re.match, search Examples
- Python Tkinter Menubutton
- Python Tkinter Menu
- Python Tkinter Message
- Python Tkinter Radiobutton
- Python List Examples
- Python Split String Examples
Related Links
Adjectives
Ado
Ai
Android
Angular
Antonyms
Apache
Articles
Asp
Autocad
Automata
Aws
Azure
Basic
Binary
Bitcoin
Blockchain
C
Cassandra
Change
Coa
Computer
Control
Cpp
Create
Creating
C-Sharp
Cyber
Daa
Data
Dbms
Deletion
Devops
Difference
Discrete
Es6
Ethical
Examples
Features
Firebase
Flutter
Fs
Git
Go
Hbase
History
Hive
Hiveql
How
Html
Idioms
Insertion
Installing
Ios
Java
Joomla
Js
Kafka
Kali
Laravel
Logical
Machine
Matlab
Matrix
Mongodb
Mysql
One
Opencv
Oracle
Ordering
Os
Pandas
Php
Pig
Pl
Postgresql
Powershell
Prepositions
Program
Python
React
Ruby
Scala
Selecting
Selenium
Sentence
Seo
Sharepoint
Software
Spellings
Spotting
Spring
Sql
Sqlite
Sqoop
Svn
Swift
Synonyms
Talend
Testng
Types
Uml
Unity
Vbnet
Verbal
Webdriver
What
Wpf