Python Tkinter Menu
The Menu widget is used to create various types of menus (top level, pull down, and pop up) in the python application.
The top-level menus are the one which is displayed just under the title bar of the parent window. We need to create a new instance of the Menu widget and add various commands to it by using the add() method.
The syntax to use the Menu widget is given below.
Syntax
A list of possible options is given below.
SN |
Option |
Description |
1 |
activebackground |
The background color of the widget when the widget is under the focus. |
2 |
activeborderwidth |
The width of the border of the widget when it is under the mouse. The default is 1 pixel. |
3 |
activeforeground |
The font color of the widget when the widget has the focus. |
4 |
bg |
The background color of the widget. |
5 |
bd |
The border width of the widget. |
6 |
cursor |
The mouse pointer is changed to the cursor type when it hovers the widget. The cursor type can be set to arrow or dot. |
7 |
disabledforeground |
The font color of the widget when it is disabled. |
8 |
font |
The font type of the text of the widget. |
9 |
fg |
The foreground color of the widget. |
10 |
postcommand |
The postcommand can be set to any of the function which is called when the mourse hovers the menu. |
11 |
relief |
The type of the border of the widget. The default type is RAISED. |
12 |
image |
It is used to display an image on the menu. |
13 |
selectcolor |
The color used to display the checkbutton or radiobutton when they are selected. |
14 |
tearoff |
By default, the choices in the menu start taking place from position 1. If we set the tearoff = 1, then it will start taking place from 0th position. |
15 |
title |
Set this option to the title of the window if you want to change the title of the window. |
Methods
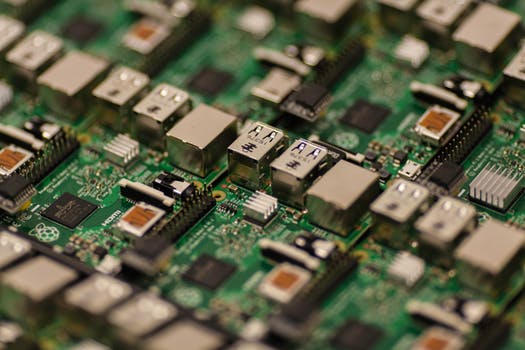
The Menu widget contains the following methods.
SN |
Option |
Description |
1 |
add_command(options) |
It is used to add the Menu items to the menu. |
2 |
add_radiobutton(options) |
This method adds the radiobutton to the menu. |
3 |
add_checkbutton(options) |
This method is used to add the checkbuttons to the menu. |
4 |
add_cascade(options) |
It is used to create a hierarchical menu to the parent menu by associating the given menu to the parent menu. |
5 |
add_seperator() |
It is used to add the seperator line to the menu. |
6 |
add(type, options) |
It is used to add the specific menu item to the menu. |
7 |
delete(startindex, endindex) |
It is used to delete the menu items exist in the specified range. |
8 |
entryconfig(index, options) |
It is used to configure a menu item identified by the given index. |
9 |
index(item) |
It is used to get the index of the specified menu item. |
10 |
insert_seperator(index) |
It is used to insert a seperator at the specified index. |
11 |
invoke(index) |
It is used to invoke the associated with the choice given at the specified index. |
12 |
type(index) |
It is used to get the type of the choice specified by the index. |
Creating a top level menu
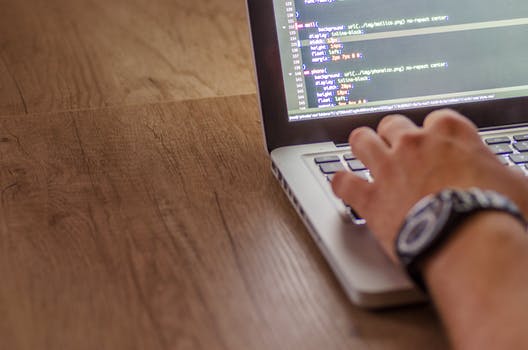
A top-level menu can be created by instantiating the Menu widget and adding the menu items to the menu.
Example 1
# !/usr/bin/python3
from tkinter import *
top = Tk()
def hello():
print("hello!")
# create a toplevel menu
menubar = Menu(root)
menubar.add_command(label="Hello!", command=hello)
menubar.add_command(label="Quit!", command=top.quit)
# display the menu
top.config(menu=menubar)
top.mainloop()
Output:
Clicking the hello Menubutton will print the hello on the console while clicking the Quit Menubutton will make an exit from the python application.
Example 2
from tkinter import Toplevel, Button, Tk, Menu
top = Tk()
menubar = Menu(top)
file = Menu(menubar, tearoff=0)
file.add_command(label="New")
file.add_command(label="Open")
file.add_command(label="Save")
file.add_command(label="Save as...")
file.add_command(label="Close")
file.add_separator()
file.add_command(label="Exit", command=top.quit)
menubar.add_cascade(label="File", menu=file)
edit = Menu(menubar, tearoff=0)
edit.add_command(label="Undo")
edit.add_separator()
edit.add_command(label="Cut")
edit.add_command(label="Copy")
edit.add_command(label="Paste")
edit.add_command(label="Delete")
edit.add_command(label="Select All")
menubar.add_cascade(label="Edit", menu=edit)
help = Menu(menubar, tearoff=0)
help.add_command(label="About")
menubar.add_cascade(label="Help", menu=help)
top.config(menu=menubar)
top.mainloop()
Output:
|