Python Tkinter Entry
The Entry widget is used to provde the single line text-box to the user to accept a value from the user. We can use the Entry widget to accept the text strings from the user. It can only be used for one line of text from the user. For multiple lines of text, we must use the text widget.
The syntax to use the Entry widget is given below.
Syntax
w = Entry (parent, options)
A list of possible options is given below.
SN |
Option |
Description |
1 |
bg |
The background color of the widget. |
2 |
bd |
The border width of the widget in pixels. |
3 |
cursor |
The mouse pointer will be changed to the cursor type set to the arrow, dot, etc. |
4 |
exportselection |
The text written inside the entry box will be automatically copied to the clipboard by default. We can set the exportselection to 0 to not copy this. |
5 |
fg |
It represents the color of the text. |
6 |
font |
It represents the font type of the text. |
7 |
highlightbackground |
It represents the color to display in the traversal highlight region when the widget does not have the input focus. |
8 |
highlightcolor |
It represents the color to use for the traversal highlight rectangle that is drawn around the widget when it has the input focus. |
9 |
highlightthickness |
It represents a non-negative value indicating the width of the highlight rectangle to draw around the outside of the widget when it has the input focus. |
10 |
insertbackground |
It represents the color to use as background in the area covered by the insertion cursor. This color will normally override either the normal background for the widget. |
11 |
insertborderwidth |
It represents a non-negative value indicating the width of the 3-D border to draw around the insertion cursor. The value may have any of the forms acceptable to Tk_GetPixels. |
12 |
insertofftime |
It represents a non-negative integer value indicating the number of milliseconds the insertion cursor should remain "off" in each blink cycle. If this option is zero, then the cursor doesn't blink: it is on all the time. |
13 |
insertontime |
Specifies a non-negative integer value indicating the number of milliseconds the insertion cursor should remain "on" in each blink cycle. |
14 |
insertwidth |
It represents the value indicating the total width of the insertion cursor. The value may have any of the forms acceptable to Tk_GetPixels. |
15 |
justify |
It specifies how the text is organized if the text contains multiple lines. |
16 |
relief |
It specifies the type of the border. Its default value is FLAT. |
17 |
selectbackground |
The background color of the selected text. |
18 |
selectborderwidth |
The width of the border to display around the selected task. |
19 |
selectforeground |
The font color of the selected task. |
20 |
show |
It is used to show the entry text of some other type instead of the string. For example, the password is typed using stars (*). |
21 |
textvariable |
It is set to the instance of the StringVar to retrieve the text from the entry. |
22 |
width |
The width of the displayed text or image. |
23 |
xscrollcommand |
The entry widget can be linked to the horizontal scrollbar if we want the user to enter more text then the actual width of the widget. |
Example
# !/usr/bin/python3
from tkinter import *
top = Tk()
top.geometry("400x250")
name = Label(top, text = "Name").place(x = 30,y = 50)
email = Label(top, text = "Email").place(x = 30, y = 90)
password = Label(top, text = "Password").place(x = 30, y = 130)
sbmitbtn = Button(top, text = "Submit",activebackground = "pink", activeforeground = "blue").place(x = 30, y = 170)
e1 = Entry(top).place(x = 80, y = 50)
e2 = Entry(top).place(x = 80, y = 90)
e3 = Entry(top).place(x = 95, y = 130)
top.mainloop()
Output:
Entry widget methods
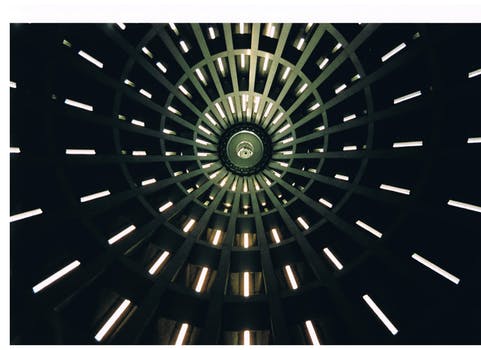
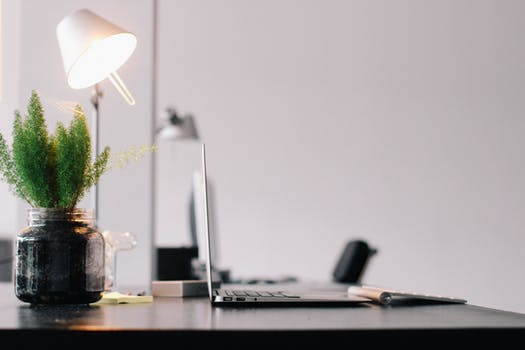
Python provides various methods to configure the data written inside the widget. There are the following methods provided by the Entry widget.
SN |
Method |
Description |
1 |
delete(first, last = none) |
It is used to delete the specified characters inside the widget. |
2 |
get() |
It is used to get the text written inside the widget. |
3 |
icursor(index) |
It is used to change the insertion cursor position. We can specify the index of the character before which, the cursor to be placed. |
4 |
index(index) |
It is used to place the cursor to the left of the character written at the specified index. |
5 |
insert(index,s) |
It is used to insert the specified string before the character placed at the specified index. |
6 |
select_adjust(index) |
It includes the selection of the character present at the specified index. |
7 |
select_clear() |
It clears the selection if some selection has been done. |
8 |
select_form(index) |
It sets the anchor index position to the character specified by the index. |
9 |
select_present() |
It returns true if some text in the Entry is selected otherwise returns false. |
10 |
select_range(start,end) |
It selects the characters to exist between the specified range. |
11 |
select_to(index) |
It selects all the characters from the beginning to the specified index. |
12 |
xview(index) |
It is used to link the entry widget to a horizontal scrollbar. |
13 |
xview_scroll(number,what) |
It is used to make the entry scrollable horizontally. |
Example: A simple calculator
import tkinter as tk
from functools import partial
def call_result(label_result, n1, n2):
num1 = (n1.get())
num2 = (n2.get())
result = int(num1)+int(num2)
label_result.config(text="Result = %d" % result)
return
root = tk.Tk()
root.geometry('400x200+100+200')
root.title('Calculator')
number1 = tk.StringVar()
number2 = tk.StringVar()
labelNum1 = tk.Label(root, text="A").grid(row=1, column=0)
labelNum2 = tk.Label(root, text="B").grid(row=2, column=0)
labelResult = tk.Label(root)
labelResult.grid(row=7, column=2)
entryNum1 = tk.Entry(root, textvariable=number1).grid(row=1, column=2)
entryNum2 = tk.Entry(root, textvariable=number2).grid(row=2, column=2)
call_result = partial(call_result, labelResult, number1, number2)
buttonCal = tk.Button(root, text="Calculate", command=call_result).grid(row=3, column=0)
root.mainloop()
Output:
|