Example. This program causes an IndentationError (unexpected indent). In the for-loop, 2 print method calls appear. But the second print() call is indented further to the right than the first. Python raises an error on the first print() call.
Tip: To fix this program, we can change the two print() statements to be indented the same number of characters.
Python program that causes IndentationError
# An incorrectly-formatted for-loop.
for n in range(0, 1):
print(n)
print(n)
Output
print(n)
^
IndentationError: unexpected indent
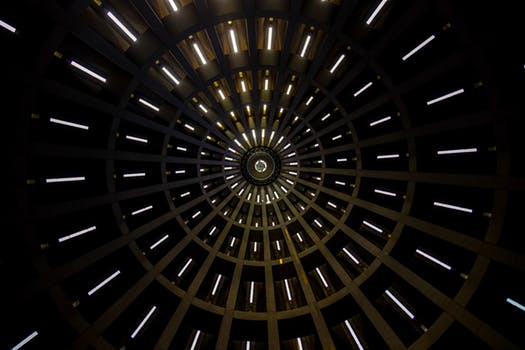
Example 2. Next, we find that indent levels have no requirement to be even throughout a program. In this example, the print-statements are unevenly-indented. But they are in separate blocks. For this reason the program executes. It raises no errors.
Python program that causes no error
# Correct.
for n in range(0, 1):
print(n)
# Correct but different.
for n in range(0, 1):
print(n)
Output
0
0
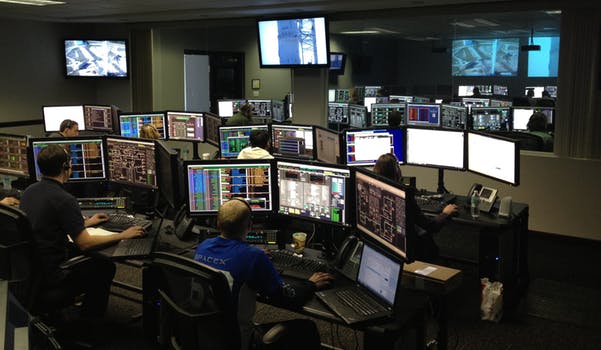
We see that the important part of indentation is consistency within blocks. It can vary between different blocks, one after another. But within the same block, indents must be correct.
Summary. Indentation is key in the Python language. It tells us how blocks are organized. Scope, a related concept, is also determined by indentation. The Python language requires strict indentation. This leads to programs more easily understood.