Python Lambda Functions
Python Lambda function is known as the anonymous function that is defined without a name. Python allows us to not declare the function in the standard manner, i.e., by using the def keyword. Rather, the anonymous functions are declared by using the lambda keyword. However, Lambda functions can accept any number of arguments, but they can return only one value in the form of expression.
The anonymous function contains a small piece of code. It simulates inline functions of C and C++, but it is not exactly an inline function.
The syntax to define an anonymous function is given below.
Syntax
lambda arguments: expression
It can accept any number of arguments and has only one expression. It is useful when the function objects are required.
Consider the following example of the lambda function.
Example 1
# a is an argument and a+10 is an expression which got evaluated and returned.
x = lambda a:a+10
# Here we are printing the function object
print(x)
print("sum = ",x(20))
Output:
<function <lambda> at 0x0000019E285D16A8>
sum = 30
In the above example, we have defined the lambda a: a+10 anonymous function where a is an argument and a+10 is an expression. The given expression gets evaluated and returned the result. The above lambda function is same as the normal function.
def x(a):
return a+10
print(sum = x(10))
Example 2
Multiple arguments to Lambda function
# a and b are the arguments and a*b is the expression which gets evaluated and returned.
x = lambda a,b: a*b
print("mul = ", x(20,10))
Output:
Why use lambda function?
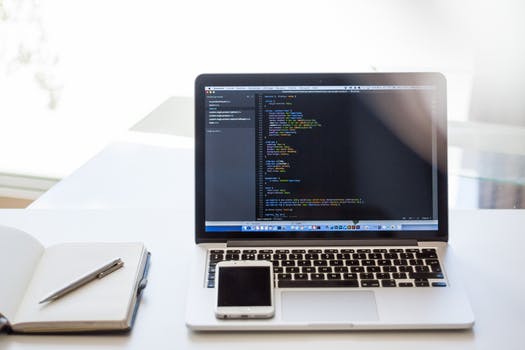
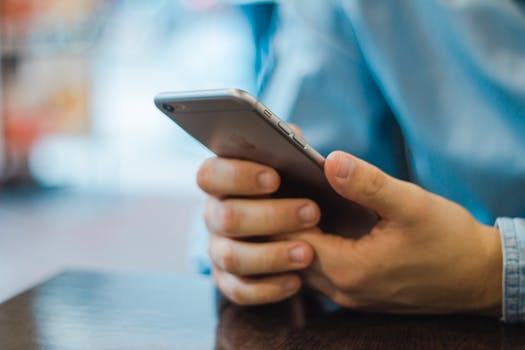
The main role of the lambda function is better described in the scenarios when we use them anonymously inside another function. In Python, the lambda function can be used as an argument to the higher-order functions which accepts other functions as arguments.
Consider the following example:
Example 1:
#the function table(n) prints the table of n
def table(n):
return lambda a:a*n # a will contain the iteration variable i and a multiple of n is returned at each function call
n = int(input("Enter the number:"))
b = table(n) #the entered number is passed into the function table. b will contain a lambda function which is called again and again with the iteration variable i
for i in range(1,11):
print(n,"X",i,"=",b(i)) #the lambda function b is called with the iteration variable i
Output:
Enter the number:10
10 X 1 = 10
10 X 2 = 20
10 X 3 = 30
10 X 4 = 40
10 X 5 = 50
10 X 6 = 60
10 X 7 = 70
10 X 8 = 80
10 X 9 = 90
10 X 10 = 100
The lambda function is commonly used with Python built-in functions filter() function and map() function.
Use lambda function with filter()
The Python built-in filter() function accepts a function and a list as an argument. It provides an effective way to filter out all elements of the sequence. It returns the new sequence in which the function evaluates to True.
Consider the following example where we filter out the only odd number from the given list.
#program to filter out the tuple which contains odd numbers
lst = (10,22,37,41,100,123,29)
oddlist = tuple(filter(lambda x:(x%3 == 0),lst)) # the tuple contains all the items of the tuple for which the lambda function evaluates to true
print(oddlist)
Output:
Using lambda function with map()
The map() function in Python accepts a function and a list. It gives a new list which contains all modified items returned by the function for each item.
Consider the following example of map() function.
#program to filter out the list which contains odd numbers
lst = (10,20,30,40,50,60)
square_list = list(map(lambda x:x**2,lst)) # the tuple contains all the items of the list for which the lambda function evaluates to true
print(square_tuple)
Output:
(100, 400, 900, 1600, 2500, 3600)
|