<< Back to C-SHARP
C# Dictionary Examples
Perform fast lookups with string keys. Add elements to Dictionary from System.Collections.Generic.Dictionary. The gopher digs tunnels. When hungry, it uses them to eat plants. A dictionary could map the gopher's tunnels to its food sources.
Dictionary keys. The Dictionary is a fast way to remember things. A key (like a string) maps to a value (like an int). Add() inserts keys and values.
ToDictionary
An example. Here we add 4 keys (each with an int value) to 2 separate Dictionary instances. Every Dictionary has pairs of keys and values.
String, int: Dictionary is used with different elements. We specify its key type and its value type (string, int).
Version 1: We use Add() to set 4 keys to 4 values in a Dictionary. Then we access the Count property to see how many items we added.
Version 2: This creates a Dictionary with the same internal values as the first version, but its syntax is more graceful.
C# program that uses Dictionary, Add, Initializer and Count
using System;
using System.Collections.Generic;
class Program
{
static void Main()
{
// Version 1: create a Dictionary, then add 4 pairs to it.
var dictionary = new Dictionary<string, int>();
dictionary.Add("cat", 2);
dictionary.Add("dog", 1);
dictionary.Add("llama", 0);
dictionary.Add("iguana", -1);
// The dictionary has 4 pairs.
Console.WriteLine("DICTIONARY 1: " + dictionary.Count);
// Version 2: create a Dictionary with an initializer.
var dictionary2 = new Dictionary<string, int>()
{
{"cat", 2},
{"dog", 1},
{"llama", 0},
{"iguana", -1}
};
// This dictionary has 4 pairs too.
Console.WriteLine("DICTIONARY 2: " + dictionary2.Count);
}
}
Output
DICTIONARY 1: 4
DICTIONARY 2: 4
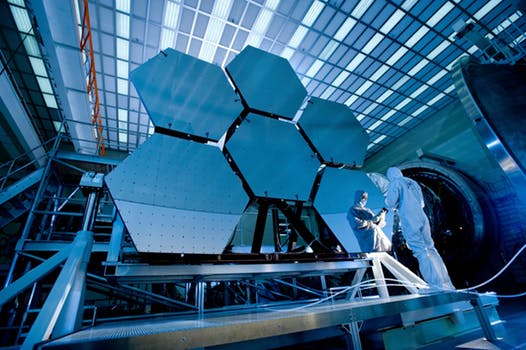
ContainsKey. This commonly-called method sees if a given string is present in a Dictionary. We use string keys here—we look at more types of Dictionaries further on.
ContainsKeyReturns: ContainsKey returns true if the key is found. Otherwise, it returns false. No exception is thrown if the key is missing.
C# program that uses ContainsKey
using System;
using System.Collections.Generic;
class Program
{
static void Main()
{
Dictionary<string, int> dictionary = new Dictionary<string, int>();
dictionary.Add("apple", 1);
dictionary.Add("windows", 5);
// See whether Dictionary contains this string.
if (dictionary.ContainsKey("apple"))
{
int value = dictionary["apple"];
Console.WriteLine(value);
}
// See whether it contains this string.
if (!dictionary.ContainsKey("acorn"))
{
Console.WriteLine(false);
}
}
}
Output
1
False
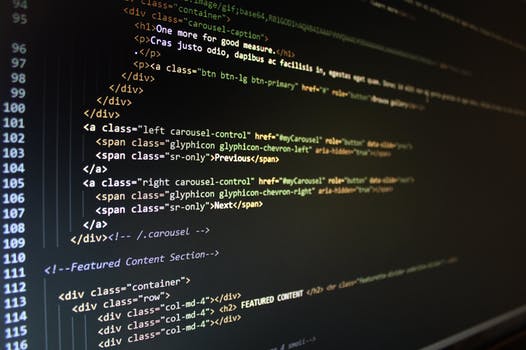
TryGetValue. This is often the most efficient lookup method. As the name TryGetValue implies, it tests for the key. It then returns the value if it finds the key.
TryGetValueImportant: Many programs can be optimized with TryGetValue. We can use the value we access without getting it a second time.
C# program that uses TryGetValue
using System;
using System.Collections.Generic;
class Program
{
static void Main()
{
Dictionary<string, string> values = new Dictionary<string, string>();
values.Add("cat", "feline");
values.Add("dog", "canine");
// Use TryGetValue.
string test;
if (values.TryGetValue("cat", out test)) // Returns true.
{
Console.WriteLine(test); // This is the value at cat.
}
if (values.TryGetValue("bird", out test)) // Returns false.
{
Console.WriteLine(false); // Not reached.
}
}
}
Output
feline
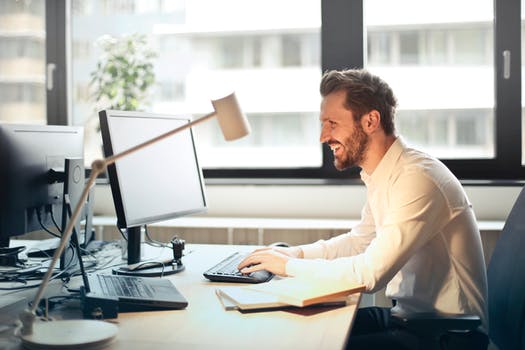
TryGetValue, syntax 2. We can declare the "out" variable directly in the method call. This can make the code easier to read—the statements are combined, but have the same effects.
Description: This variable (description) cannot be accessed elsewhere in the program other than in the if-statement.
C# program that uses inline out, TryGetValue
using System.Collections.Generic;
class Program
{
static void Main()
{
var values = new Dictionary<string, string>();
values.Add("A", "uppercase letter A");
values.Add("c", "lowercase letter C");
// Use inline "out string" with TryGetValue.
if (values.TryGetValue("c", out string description))
{
System.Console.WriteLine(description);
}
}
}
Output
lowercase letter C
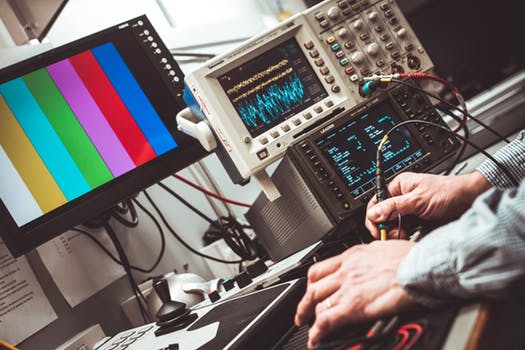
Loop. Here we loop over KeyValuePairs in a Dictionary. With collections like Dictionary, we must always know the value types. With each KeyValuePair, there is a Key member and Value member.
ForeachString, int: The code creates a Dictionary with string keys and int values. The Dictionary stores animal counts.
Tip: In the foreach-loop, each KeyValuePair struct has 2 members, a string Key and an int Value.
KeyValuePairVar: This keyword reduces the amount of typing required. And it may make code easier to read for humans (like us).
VarC# program that uses foreach on Dictionary
using System;
using System.Collections.Generic;
class Program
{
static void Main()
{
Dictionary<string, int> data = new Dictionary<string, int>()
{
{"cat", 2},
{"dog", 1},
{"llama", 0},
{"iguana", -1}
};
// Loop over pairs with foreach.
foreach (KeyValuePair<string, int> pair in data)
{
Console.WriteLine("FOREACH KEYVALUEPAIR: {0}, {1}", pair.Key, pair.Value);
}
// Use var keyword to enumerate Dictionary.
foreach (var pair in data)
{
Console.WriteLine("FOREACH VAR: {0}, {1}", pair.Key, pair.Value);
}
}
}
Output
FOREACH KEYVALUEPAIR: cat, 2
FOREACH KEYVALUEPAIR: dog, 1
FOREACH KEYVALUEPAIR: llama, 0
FOREACH KEYVALUEPAIR: iguana, -1
FOREACH VAR: cat, 2
FOREACH VAR: dog, 1
FOREACH VAR: llama, 0
FOREACH VAR: iguana, -1
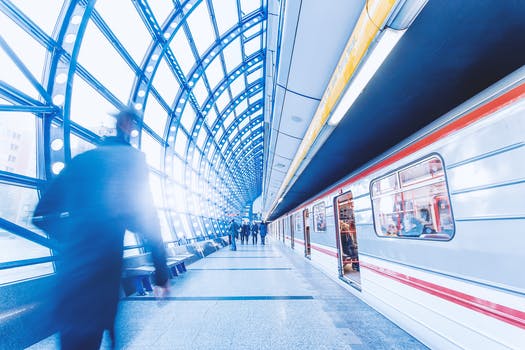
Keys. Here we use the Keys property. We then look through each key and look up the values. This method is slower but has the same results.
KeyCollection: The Keys property returns a collection of type KeyCollection, not an actual List. We can convert it into a List.
C# program that gets Keys
using System;
using System.Collections.Generic;
class Program
{
static void Main()
{
var data = new Dictionary<string, int>()
{
{"cat", 2},
{"dog", 1},
{"llama", 0},
{"iguana", -1}
};
// Store keys in a List.
var list = new List<string>(data.Keys);
// Loop through the List.
foreach (string key in list)
{
Console.WriteLine("KEY FROM LIST: " + key);
}
}
}
Output
KEY FROM LIST: cat
KEY FROM LIST: dog
KEY FROM LIST: llama
KEY FROM LIST: iguana
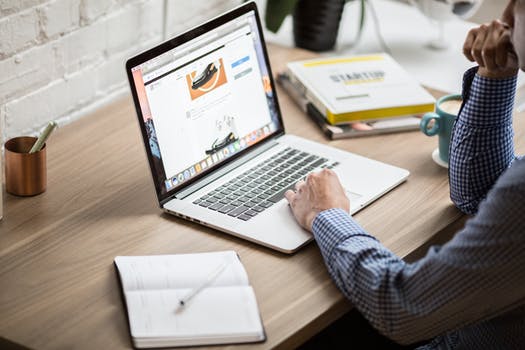
Types. Dictionary is a generic class. To use it, we must specify a type. This is a good feature. It means we can use an int key just as easily as a string key.
Int: In this program, we see an example of a Dictionary with int keys. The values can also be any type.
C# program that uses int keys
using System;
using System.Collections.Generic;
class Program
{
static void Main()
{
// Use int key type.
var data = new Dictionary<int, string>();
data.Add(100, "color");
data.Add(200, "fabric");
// Look up the int.
if (data.ContainsKey(200))
{
Console.WriteLine("CONTAINS KEY 200");
}
}
}
Output
CONTAINS KEY 200
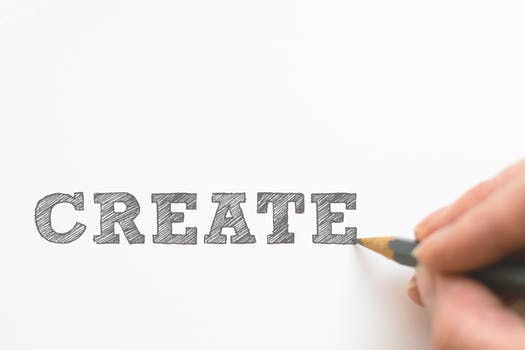
LINQ. Extension methods can be used with Dictionary. We use the ToDictionary method. This is an extension method on IEnumerable. It places keys and values into a new Dictionary.
Lambda: The program uses lambda expressions. With these small functions, we specify a method directly as an argument.
LambdaHere: The example uses ToDictionary, from System.Linq, on a string array. It creates a lookup table for the strings.
C# program that uses System.Linq, ToDictionary
using System;
using System.Linq;
class Program
{
static void Main()
{
string[] values = new string[]
{
"One",
"Two"
};
// Create Dictionary with ToDictionary.
// ... Specify a key creation method, and a value creation method.
var result = values.ToDictionary(item => item, item => true);
foreach (var pair in result)
{
Console.WriteLine("RESULT PAIR: {0}, {1}", pair.Key, pair.Value);
}
}
}
Output
RESULT PAIR: One, True
RESULT PAIR: Two, True
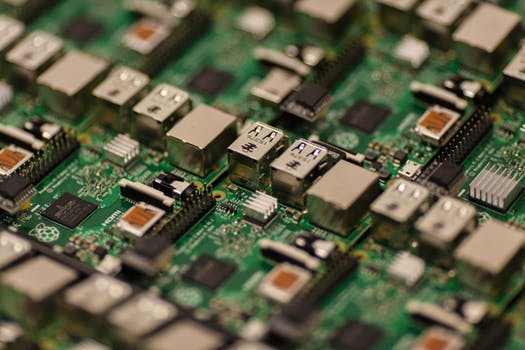
ContainsValue. This method lacks the constant-time look up speed of ContainsKey. It instead searches the entire collection. It is linear in complexity.
ContainsValueNote: This example will loop through all elements in the Dictionary until it finds a match, or there are no more elements to check.
Speed: Microsoft states that "this method is an O(N) operation, where N is Count." It does not run in constant time.
C# program that uses ContainsValue
using System;
using System.Collections.Generic;
class Program
{
static void Main()
{
var data = new Dictionary<string, int>();
data.Add("cat", 1);
data.Add("dog", 2);
// Use ContainsValue to see if the value is present with any key.
if (data.ContainsValue(1))
{
Console.WriteLine("VALUE 1 IS PRESENT");
}
}
}
Output
VALUE 1 IS PRESENT
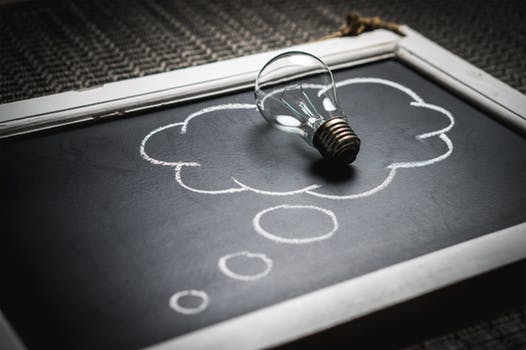
Indexer. We do not need to use Add to insert into a Dictionary. We can instead use the indexer, with the "[" and "]" brackets. This syntax also gets the value at the key.
Caution: If we try to get a value at a key that doesn't exist, an exception is thrown.
Note: With the indexer, an exception is not thrown when we assign to a key that already has a value. But with Add, an exception is thrown.
C# program that uses Dictionary indexer
using System;
using System.Collections.Generic;
class Program
{
static void Main()
{
Dictionary<int, int> dictionary = new Dictionary<int, int>();
// We can assign with the indexer.
dictionary[1] = 2;
dictionary[2] = 1;
dictionary[1] = 3; // Reassign.
// Read with the indexer.
// ... An exception occurs if no element exists.
Console.WriteLine(dictionary[1]);
Console.WriteLine(dictionary[2]);
}
}
Output
3
1
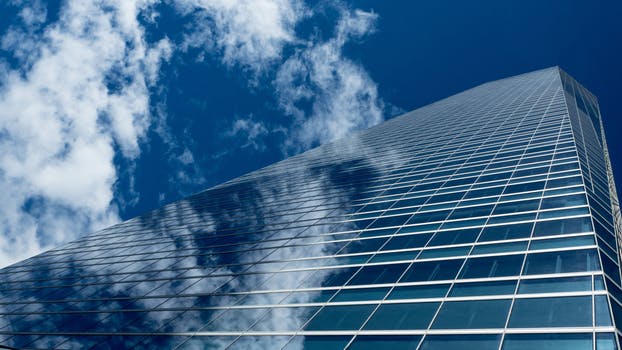
Remove, Count. With Remove, no remnants of the key-value pair are kept. Sometimes using null as a key's value is a better option—but this may add complexity to a program.
Note: Running the code in Visual Studio, no exceptions are thrown. When we remove a key that doesn't exist, nothing happens.
However: Remove() throws System.ArgumentNullException with a null parameter. We cannot remove null.
Count: This computes the total number of keys. Count is the simplest way to count all pairs in a Dictionary.
CountC# program that uses Remove, Count
using System;
using System.Collections.Generic;
class Program
{
static void Main()
{
var data = new Dictionary<string, int>();
data.Add("cat", 1);
data.Add("dog", 2);
Console.WriteLine("COUNT: " + data.Count);
// Remove cat.
data.Remove("cat");
Console.WriteLine("COUNT: " + data.Count);
// This does not remove anything.
data.Remove("nothing");
Console.WriteLine("COUNT: " + data.Count);
}
}
Output
COUNT: 2
COUNT: 1
COUNT: 1
Copy. Dictionary provides a constructor that copies all values and keys into a new Dictionary instance. This constructor improves code reuse. It makes copying simpler.
Copy DictionaryTest: The "test" Dictionary in this program is created, and has just 1 key and value pair in it.
Copy: We create a copy by passing the "test" Dictionary to its constructor. We add a key. The copy has 2 keys—but "test" still has 1.
C# program that copies Dictionary
using System;
using System.Collections.Generic;
class Program
{
static void Main()
{
var test = new Dictionary<int, int>();
test[20] = 30;
// Copy the dictionary, and add another key, so we now have 2 keys.
var copy = new Dictionary<int, int>(test);
copy[30] = 40;
Console.WriteLine("TEST COUNT: {0}", test.Count);
Console.WriteLine("COPY COUNT: {0}", copy.Count);
}
}
Output
TEST COUNT: 1
COPY COUNT: 2
Composite keys. We can sometimes use multiple variables in a key. We can create a special function that transforms those variables into a string, serializing them.
SetComposite: This method receives 2 ints—the parts of the key. It creates a string with a comma, and assigns into the Dictionary.
GetComposite: This looks up a value in the Dictionary with 2 int arguments—the same string key is generated on each call.
Result: We use the string "1,2" to mean the ints 1 and 2. A string is created each time. We have 2 keys per value.
C# program that uses composite keys
using System;
using System.Collections.Generic;
class Program
{
static Dictionary<string, string> _composite = new Dictionary<string, string>();
static void SetComposite(int part1, int part2, string value)
{
// Use 2 ints for the string key.
_composite[part1.ToString() + "," + part2.ToString()] = value;
}
static string GetComposite(int part1, int part2)
{
// Create composite string to look up result.
_composite.TryGetValue(part1.ToString() + "," + part2.ToString(),
out string result);
return result;
}
static void Main()
{
// Set values in Dictionary with composite keys.
SetComposite(1, 2, "hello");
SetComposite(300, 400, "aloha");
// Get values in Dictionary with composite keys.
Console.WriteLine("GETCOMPOSITE: {0}", GetComposite(1, 2));
Console.WriteLine("GETCOMPOSITE: {0}", GetComposite(300, 400));
}
}
Output
GETCOMPOSITE: hello
GETCOMPOSITE: aloha
Dictionary field. Sometimes it is useful to have a Dictionary at the class level, as a field. And if we have a static class, we should initialize the Dictionary at the class level.
Note: Avoid the static constructor—static constructors often carry performance penalties.
C# program that uses Dictionary field
using System;
using System.Collections.Generic;
class Example
{
Dictionary<int, int> _lookup = new Dictionary<int, int>()
{
{1, 1},
{2, 3},
{3, 5},
{6, 10}
};
public int GetValue()
{
return _lookup[2]; // Example only.
}
}
class Program
{
static void Main()
{
// Create Example object instance.
var example = new Example();
// Look up a value from the Dictionary field.
Console.WriteLine("RESULT: " + example.GetValue());
}
}
Output
RESULT: 3
KeyNotFoundException. This error happens when we access a nonexistent key. With Dictionary we should test keys for existence first, with ContainsKey or TryGetValue.
KeyNotFoundExceptionC# program that shows KeyNotFoundException
using System.Collections.Generic;
class Program
{
static void Main()
{
var colors = new Dictionary<string, int>() { { "blue", 10 } };
// Use ContainsKey or TryGetValue instead.
int result = colors["fuschia"];
}
}
Output
Unhandled Exception: System.Collections.Generic.KeyNotFoundException:
The given key was not present in the dictionary.
at System.Collections.Generic.Dictionary`2.get_Item(TKey key)
at Program.Main() in ...
Benchmark, StringComparer. When you do a lookup on a Dictionary of string keys, the string must be hashed. If we pass StringComparer.Ordinal to our Dictionary, we have a faster algorithm.
Version 1: We look up the key "Las Vegas" in a Dictionary with no comparer specified in its constructor.
Version 2: In this version we look up the string "Las Vegas" in a Dictionary with StringComparer.Ordinal.
Result: When we consider a string as "ordinal," we lose some string features, but gain performance on comparison and hashing.
C# program that times StringComparer.Ordinal
using System;
using System.Collections.Generic;
using System.Diagnostics;
class Program
{
const int _max = 10000000;
static void Main()
{
var data1 = new Dictionary<string, bool>();
data1["Las Vegas"] = true;
var data2 = new Dictionary<string, bool>(StringComparer.Ordinal);
data2["Las Vegas"] = true;
// Version 1: do lookups on Dictionary with default string comparisons.
Stopwatch s1 = Stopwatch.StartNew();
for (int i = 0; i < _max; i++)
{
if (!data1.ContainsKey("Las Vegas"))
{
return;
}
}
s1.Stop();
// Version 2: do lookups in Dictionary that has StringComparer.Ordinal.
Stopwatch s2 = Stopwatch.StartNew();
for (int i = 0; i < _max; i++)
{
if (!data2.ContainsKey("Las Vegas"))
{
return;
}
}
s2.Stop();
Console.WriteLine(((double)(s1.Elapsed.TotalMilliseconds * 1000000) /
_max).ToString("0.00 ns"));
Console.WriteLine(((double)(s2.Elapsed.TotalMilliseconds * 1000000) /
_max).ToString("0.00 ns"));
}
}
Output
19.65 ns ContainsKey, default comparer
18.99 ns ContainsKey, StringComparer.Ordinal
Benchmark, Keys. Here we compare loops. A foreach-loop on KeyValuePairs is faster than the looping over Keys and accessing values in the loop body.
Version 1: This code loops over the dictionary in a foreach-loop directly, without accessing the Keys property.
Version 2: This version of the code accesses the Keys property, and then looks up each value in a foreach-loop.
Result: When possible, loop over the pairs in a Dictionary directly. Eliminating lookups will improve performance.
C# program that benchmarks foreach on Dictionary
using System;
using System.Collections.Generic;
using System.Diagnostics;
class Program
{
static void Main()
{
var test = new Dictionary<string, int>();
test["bird"] = 10;
test["frog"] = 20;
test["cat"] = 60;
int sum = 0;
const int _max = 1000000;
// Version 1: use foreach loop directly on Dictionary.
var s1 = Stopwatch.StartNew();
for (int i = 0; i < _max; i++)
{
foreach (var pair in test)
{
sum += pair.Value;
}
}
s1.Stop();
// Version 2: use foreach loop on Keys, then access values.
var s2 = Stopwatch.StartNew();
for (int i = 0; i < _max; i++)
{
foreach (var key in test.Keys)
{
sum += test[key];
}
}
s2.Stop();
Console.WriteLine(s1.Elapsed.TotalMilliseconds);
Console.WriteLine(s2.Elapsed.TotalMilliseconds);
}
}
Output
28.117 ms Dictionary foreach
83.3468 ms Keys foreach
Benchmark, GetEnumerator. With the GetEnumerator method we can loop over a Dictionary. We call GetEnumerator() and then use a while-loop on the MoveNext method.
Version 1: This code uses the foreach loop to sum the values in a Dictionary collection.
Version 2: This method uses the GetEnumerator method, the MoveNext method, and the Current property to sum the values.
GetEnumeratorResult: By using GetEnumerator, our loop is faster. The IL generated is a bit different (no try-finally block is generated).
C# program that times GetEnumerator, MoveNext
using System;
using System.Collections.Generic;
using System.Diagnostics;
class Program
{
static int Version1(Dictionary<string, int> values)
{
int sum = 0;
foreach (var pair in values)
{
sum += pair.Value;
}
return sum;
}
static int Version2(Dictionary<string, int> values)
{
int sum = 0;
var enumerator = values.GetEnumerator();
while (enumerator.MoveNext())
{
var pair = enumerator.Current;
sum += pair.Value;
}
return sum;
}
const int _max = 1000000;
static void Main()
{
var data = new Dictionary<string, int>();
data["bird"] = 1;
data["cat"] = 2;
data["feather"] = -1;
data["fur"] = -2;
// Version 1: use foreach to loop over a Dictionary.
var s1 = Stopwatch.StartNew();
for (int i = 0; i < _max; i++)
{
Version1(data);
}
s1.Stop();
// Version 2: use GetEnumerator to loop over a Dictionary.
var s2 = Stopwatch.StartNew();
for (int i = 0; i < _max; i++)
{
Version2(data);
}
s2.Stop();
Console.WriteLine(((double)(s1.Elapsed.TotalMilliseconds * 1000000) /
_max).ToString("0.00 ns"));
Console.WriteLine(((double)(s2.Elapsed.TotalMilliseconds * 1000000) /
_max).ToString("0.00 ns"));
}
}
Output
41.07 ns foreach
39.51 ns GetEnumerator, MoveNext
Benchmark, key length. When a string key is looked up in a Dictionary, it must be hashed and compared. Logically a shorter string key should be faster, as less data is being tested.
Version 1: This code looks up a short string key in the Dictionary—each lookup is just 4 chars.
Version 2: Here we look up a string key that is longer. Each lookup succeeds (in the same way as version 1).
Result: The version that looks up the key "Alps" is faster than the version that looks up the string key "Appalachian."
C# program that times string key length
using System;
using System.Collections.Generic;
using System.Diagnostics;
class Program
{
const int _max = 10000000;
static void Main()
{
var places = new Dictionary<string, int>()
{
{ "Alps", 1 },
{ "Appalachian", 2 }
};
// Version 1: look up a short string key.
var s1 = Stopwatch.StartNew();
for (int i = 0; i < _max; i++)
{
if (!places.ContainsKey("Alps"))
{
return;
}
}
s1.Stop();
// Version 2: look up a long string key.
var s2 = Stopwatch.StartNew();
for (int i = 0; i < _max; i++)
{
if (!places.ContainsKey("Appalachian"))
{
return;
}
}
s2.Stop();
Console.WriteLine(((double)(s1.Elapsed.TotalMilliseconds * 1000000) /
_max).ToString("0.00 ns"));
Console.WriteLine(((double)(s2.Elapsed.TotalMilliseconds * 1000000) /
_max).ToString("0.00 ns"));
}
}
Output
18.32 ns 4-char string key
21.00 ns 12-char string key
Clear. We can erase all pairs with the Clear method. Or we can assign the Dictionary variable to null. This causes little difference in memory usage—the entries are garbage-collected.
Dictionary Clear
Return. A Dictionary can be returned, or passed as an argument. The Dictionary type is defined as a class. It is always passed as a reference type.
And: This means only 32-64 bits will be copied on the method invocation. The same principle applies when returning values.
Return
IEqualityComparer. Dictionary uses an IEqualityComparer to compute the hash code for its keys. We can implement this interface with a class. This can improve performance.
IEqualityComparerMap. The Dictionary is a map. A real map helps us find our destination, but a digital map directs us from a key to a value. In languages we often find a map type.
MapCase-insensitive keys. With a special StringComparer we can have case-insensitive key lookups on a dictionary. This reduces the need to call ToLower. It reduces string creation.
Case, DictionaryResearch. A Dictionary is powerful, but it is not magic. It just uses a good algorithm. This requires a hash code. We take keys and use arithmetic to transform them into numbers.
Locations: We then store them in locations based on those numbers in the Dictionary. We select a "bucket" based on the hash.
Finally: When we go to look up a key, we compute a new hash code and look for it in the Dictionary. Less searching is needed.
Quote: We try to reference items in a table directly by doing arithmetic operations to transform keys into table addresses (Algorithms in C++ Third Edition).
A summary. It is good that fast lookups are important. We just spent lots of time researching them. Dictionary, like all hash tables, is fascinating. It is fast—and useful in many places.
Related Links:
- C# Array Examples, String Arrays
- C# ArrayList Examples
- C# ArraySegment: Get Array Range, Offset and Count
- C# break Statement
- C# Buffer BlockCopy Example
- C# BufferedStream: Optimize Read and Write
- 404 Not Found
- C# 24 Hour Time Formats
- C# 2D Array Examples
- 7 Zip Command Line Examples
- C# 7 Zip Executable (Process.Start)
- C# All Method: All Elements Match a Condition
- C# Alphabetize String
- C# Alphanumeric Sorting
- C# Arithmetic Expression Optimization
- C# Array.AsReadOnly Method (ObjectModel)
- C# Array.BinarySearch Method
- C# Array.Clear Examples
- C# Array.IndexOf, LastIndexOf: Search Arrays
- C# async, await Examples
- C# Attribute Examples
- C# Average Method
- C# BackgroundWorker
- C# base Keyword
- C# String Between, Before, After
- C# Binary Representation int (Convert, toBase 2)
- C# BinarySearch List
- C# bool Array (Memory Usage, One Byte Per Element)
- C# bool.Parse, TryParse: Convert String to Bool
- C# bool Type
- C# Array Length Property, Get Size of Array
- C# Button Example
- C# Byte Array: Memory Usage, Read All Bytes
- C# Byte and sbyte Types
- C# Capacity for List, Dictionary
- C# Case Insensitive Dictionary
- C# case Example (Switch Case)
- C# Char Array
- C# Checked and Unchecked Keywords
- C# CheckedListBox: Windows Forms
- C# Color Table
- C# Color Examples: FromKnownColor, FromName
- C# ColorDialog Example
- C# Comment: Single Line and Multiline
- C# Concat Extension: Combine Lists and Arrays
- C# Conditional Attribute
- C# Console Color, Text and BackgroundColor
- C# String Clone() method
- C# Constructor Examples
- C# Contains Extension Method
- C# String GetTypeCode() method
- C# String ToLowerInvariant() method
- C# Customized Dialog Box
- C# DataColumn Example: Columns.Add
- C# DataGridView Add Rows
- DataGridView Columns, Edit Columns Dialog
- C# DataGridView Row Colors (Alternating)
- C# DataGridView Tutorial
- C# DataGridView
- C# DataRow Examples
- C# DataSet Examples
- C# DataSource Example
- C# DataTable Compare Rows
- C# DataTable foreach Loop
- C# DataTable RowChanged Example: AcceptChanges
- C# DataTable Select Example
- C# DataTable Examples
- C# DataView Examples
- C# String ToString() method
- C# String ToUpper() method
- C# Digit Separator
- C# DateTime.MinValue (Null DateTime)
- C# DateTime.Month Property
- C# DateTime.Parse: Convert String to DateTime
- C# DateTime Subtract Method
- C# Decompress GZIP
- C# Remove Duplicates From List
- C# dynamic Keyword
- C# ElementAt, ElementAtOrDefault Use
- C# Encapsulate Field
- C# Enum Array Example, Use Enum as Array Index
- C# enum Flags Attribute Examples
- C# Enum ToString: Convert Enum to String
- C# enum Examples
- C# Enumerable.Range, Repeat and Empty
- C# Environment Type
- C# EventLog Example
- C# Exception Handling
- C# explicit and implicit Keywords
- C# Factory Design Pattern
- C# File.Copy Examples
- C# typeof and nameof Operators
- C# String TrimEnd() method
- C# var Examples
- C# virtual Keyword
- C# void Method, Return No Value
- C# volatile Example
- C# WebBrowser Control (Navigate Method)
- C# WebClient: DownloadData, Headers
- C# Where Method and Keyword
- C# String TrimStart() method
- C# delegate Keyword
- C# descending, ascending Keywords
- C# while Loop Examples
- C# Whitespace Methods: Convert UNIX, Windows Newlines
- C# XmlReader, Parse XML File
- C# XmlTextReader
- C# XmlTextWriter
- C# XmlWriter, Create XML File
- C# XOR Operator (Bitwise)
- C# yield Example
- C# float Numbers
- FlowLayoutPanel Control
- C# Focused Property
- C# FolderBrowserDialog Control
- C# Font Type: FontFamily and FontStyle
- C# FontDialog Example
- C# for Loop Examples
- C# foreach Loop Examples
- ForeColor, BackColor: Windows Forms
- C# Form: Event Handlers
- C# Contains String Method
- C# ContainsValue Method (Value Exists in Dictionary)
- C# ContextMenuStrip Example
- C# continue Keyword
- C# Control: Windows Forms
- C# Windows Forms Controls
- C# Convert Char Array to String
- C# Convert Char to String
- C# Convert Days to Months
- C# Convert String to Byte Array
- C# String Format
- C# Func Object (Lambda That Returns a Value)
- C# GC.Collect Examples: CollectionCount, GetTotalMemory
- C# Path.GetDirectoryName (Remove File From Path)
- C# goto Examples
- C# HttpClient Example: System.Net.Http
- ASP.NET HttpContext Request Property
- IL Disassembler Tutorial
- C# Intermediate Language (IL)
- C# IndexOf Examples
- C# IndexOfAny Examples
- C# Initialize Array
- C# Initialize List
- C# InitializeComponent Method: Windows Forms
- C# Inline Optimization
- C# Dictionary Equals: If Contents Are the Same
- C# Dictionary Versus List Loop
- C# Dictionary Order, Use Keys Added Last
- C# Dictionary Size and Performance
- C# Dictionary Versus List Lookup Time
- C# Dictionary Examples
- C# Get Directory Size (Total Bytes in All Files)
- C# Directory Type
- C# Distinct Method, Get Unique Elements Only
- C# Divide by Powers of Two (Bitwise Shift)
- C# Divide Numbers (Cast Ints)
- C# DomainUpDown Control Example
- C# Double Type: double.MaxValue, double.Parse
- C# Remove Duplicate Chars
- C# IEqualityComparer
- C# If Preprocessing Directive: Elif and Endif
- C# If Versus Switch Performance
- C# if Statement
- C# int.MaxValue, MinValue (Get Lowest Number)
- C# Program to reverse number
- C# Int and uint Types
- C# Integer Append Optimization
- C# Keywords
- C# Label Example: Windows Forms
- C# Lambda Expressions
- C# LastIndexOf Examples
- C# Last, LastOrDefault Methods
- C# Mutex Example (OpenExisting)
- C# Named Parameters
- C# Let Keyword (Use Variable in Query Expression)
- C# Levenshtein Distance
- C# LinkLabel Example: Windows Forms
- C# LINQ
- C# List Add Method, Append Element to List
- C# List AddRange, InsertRange (Append Array to List)
- C# List Clear Example
- C# List Contains Method
- C# List Remove Examples
- C# List Examples
- C# ListBox Tutorial (DataSource, SelectedIndex)
- C# ListView Tutorial: Windows Forms
- C# Maze Pathfinding Algorithm
- C# Memoization
- C# Memory Usage for Arrays of Objects
- C# MessageBox.Show Examples
- C# Method Call Depth Performance
- C# Method Parameter Performance
- C# Method Size Optimization
- C# Multidimensional Array
- C# MultiMap Class (Dictionary of Lists)
- C# Optimization
- C# new Keyword
- C# NotifyIcon: Windows Forms
- C# NotImplementedException
- C# Null Array
- C# String GetType() method
- C# Null Coalescing and Null Conditional Operators
- C# Null List (NullReferenceException)
- C# Numeric Casts
- C# NumericUpDown Control: Windows Forms
- C# object.ReferenceEquals Method
- C# Object Examples
- C# Optional Parameters
- C# Prime Number
- C# OrderBy, OrderByDescending Examples
- C# Process Examples (Process.Start)
- Panel, Windows Forms (Create Group of Controls)
- C# Path Examples
- C# Get Percentage From Number With Format String
- ASP.NET PhysicalApplicationPath
- C# PictureBox: Windows Forms
- C# PNG Optimization
- C# Position Windows: Use WorkingArea and Location
- Visual Studio Post Build, Pre Build Macros
- C# ProfileOptimization
- C# ProgressBar Example
- C# Property Examples
- C# PropertyGrid: Windows Forms
- C# Protected and internal Keywords
- C# Public and private Methods
- C# Remove Punctuation From String
- C# Query Windows Forms (Controls.OfType)
- C# Queryable: IQueryable Interface and AsQueryable
- ASP.NET QueryString Examples
- C# Queue Collection: Enqueue
- C# RadioButton Use: Windows Forms
- C# ReadOnlyCollection Use (ObjectModel)
- C# Recursion Optimization
- C# Recursive File List: GetFiles With AllDirectories
- C# ref Keyword
- C# Reflection Examples
- C# Regex.Escape and Unescape Methods
- C# StringBuilder Examples
- C# StringComparison and StringComparer
- C# StringReader Class (Get Parts of Strings)
- C# String GetEnumerator() method
- C# String GetHashCode() method
- C# Regex Versus Loop: Use For Loop Instead of Regex
- C# Regex.Match Examples: Regular Expressions
- C# RemoveAll: Use Lambda to Delete From List
- C# Replace String Examples
- ASP.NET Response.BinaryWrite
- ASP.NET Response.Write
- C# Return Optimization: out Performance
- C# SaveFileDialog: Use ShowDialog and FileName
- C# Scraping HTML Links
- C# sealed Keyword
- C# Seek File Examples: ReadBytes
- C# select new Example: LINQ
- C# Select Method (Use Lambda to Modify Elements)
- C# Serialize List (Write to File With BinaryFormatter)
- C# Settings.settings in Visual Studio
- C# Shuffle Array: KeyValuePair and List
- C# Single and Double Types
- C# Single Instance Windows Form
- C# Snippet Examples
- C# Sort DateTime List
- C# Sort List With Lambda, Comparison Method
- C# Sort Number Strings
- C# Sort Examples: Arrays and Lists
- C# SortedDictionary
- C# SortedList
- C# SortedSet Examples
- C# Split String Examples
- C# String Copy() method
- C# SplitContainer: Windows Forms
- C# SqlClient Tutorial: SqlConnection, SqlCommand
- C# SqlCommand Example: SELECT TOP, ORDER BY
- C# SqlCommandBuilder Example: GetInsertCommand
- C# SqlConnection Example: Using, SqlCommand
- C# SqlDataAdapter Example
- C# SqlDataReader: GetInt32, GetString
- C# SqlParameter Example: Constructor, Add
- C# Stack Collection: Push, Pop
- C# Static List: Global List Variable
- C# Static Regex
- C# Static String
- C# static Keyword
- C# StatusStrip Example: Windows Forms
- C# String Chars (Get Char at Index)
- C# string.Concat Examples
- C# String Interpolation Examples
- C# string.Join Examples
- C# String Performance, Memory Usage Info
- C# String Property
- C# String Slice, Get Substring Between Indexes
- C# String Switch Examples
- C# String
- C# StringBuilder Append Performance
- C# StringBuilder Cache
- C# ToBase64String (Data URI Image)
- C# Struct Versus Class
- C# struct Examples
- C# Substring Examples
- C# Numeric Suffix Examples
- C# switch Examples
- C# String IsNormalized() method
- C# TabControl: Windows Forms
- TableLayoutPanel: Windows Forms
- C# Take and TakeWhile Examples
- C# Task Examples (Task.Run, ContinueWith and Wait)
- C# Ternary Operator
- C# Text Property: Windows Forms
- C# TextBox.AppendText Method
- C# TextBox Example
- C# TextChanged Event
- C# TextFieldParser Examples: Read CSV
- C# ThreadStart and ParameterizedThreadStart
- C# throw Keyword Examples
- C# Timer Examples
- C# TimeSpan Examples
- C# TrimEnd and TrimStart
- C# True and False
- C# Truncate String
- C# String ToLower() method
- C# String ToCharArray() method
- C# String ToUpperInvariant() method
- C# String Trim() method
- C# Assign Variables Optimization
- C# Array.Resize Examples
- C# Array.Sort: Keys, Values and Ranges
- C# Array.Reverse Example
- C# Array Slice, Get Elements Between Indexes
- C# Array.TrueForAll: Use Lambda to Test All Elements
- C# ArrayTypeMismatchException
- C# as: Cast Examples
- C# ASCII Table
- C# ASCII Transformation, Convert Char to Index
- C# AsEnumerable Method
- C# AsParallel Example
- ASP.NET AspLiteral
- C# BaseStream Property
- C# Console.Beep Example
- C# Benchmark
- C# BinaryReader Example (Use ReadInt32)
- C# BinaryWriter Type
- C# BitArray Examples
- C# BitConverter Examples
- C# Bitcount Examples
- C# Bool Methods, Return True and False
- C# bool Sort Examples (True to False)
- C# Caesar Cipher
- C# Cast Extension: System.Linq
- C# Cast to Int (Convert Double to Int)
- C# Cast Examples
- C# catch Examples
- C# Change Characters in String (ToCharArray, For Loop)
- C# Char Combine: Get String From Chars
- C# char.IsDigit (If Char Is Between 0 and 9)
- C# char.IsLower and IsUpper
- C# Character Literal (const char)
- C# Char Lowercase Optimization
- C# Char Test (If Char in String Equals a Value)
- C# char.ToLower and ToUpper
- C# char Examples
- C# abstract Keyword
- C# Action Object (Lambda That Returns Void)
- C# Aggregate: Use Lambda to Accumulate Value
- C# AggressiveInlining: MethodImpl Attribute
- C# Anagram Method
- C# And Bitwise Operator
- C# Anonymous Function (Delegate With No Name)
- C# Any Method, Returns True If Match Exists
- C# StringBuilder Append and AppendLine
- C# StringBuilder AppendFormat
- ASP.NET appSettings Example
- C# ArgumentException: Invalid Arguments
- C# Array.ConvertAll, Change Type of Elements
- C# Array.Copy Examples
- C# Array.CreateInstance Method
- C# Array and Dictionary Test, Integer Lookups
- C# Array.Exists Method, Search Arrays
- C# Array.Find Examples, Search Array With Lambda
- C# Array.ForEach: Use Lambda on Every Element
- C# Array Versus List Memory Usage
- C# Array Property, Return Empty Array
- C# CharEnumerator
- C# Chart, Windows Forms (Series and Points)
- C# CheckBox: Windows Forms
- C# class Examples
- C# Clear Dictionary: Remove All Keys
- C# Clone Examples: ICloneable Interface
- C# Closest Date (Find Dates Nearest in Time)
- C# Combine Arrays: List, Array.Copy and Buffer.BlockCopy
- C# Combine Dictionary Keys
- C# ComboBox: Windows Forms
- C# CompareTo Int Method
- C# Comparison Object, Used With Array.Sort
- C# Compress Data: GZIP
- C# Console.Read Method
- C# Console.ReadKey Example
- C# Console.ReadLine Example (While Loop)
- C# Console.SetOut and Console.SetIn
- C# Console.WindowHeight
- C# Console.Write, Append With No Newline
- C# Console.WriteLine (Print)
- C# const Example
- C# Constraint Puzzle Solver
- C# Count Characters in String
- C# Count, Dictionary (Get Number of Keys)
- C# Count Letter Frequencies
- C# Count Extension Method: Use Lambda to Count
- C# CSV Methods (Parse and Segment)
- C# DataRow Field Method: Cast DataTable Cell
- C# Get Day Count Elapsed From DateTime
- C# DateTime Format
- C# DateTime.Now (Current Time)
- C# DateTime.Today (Current Day With Zero Time)
- C# DateTime.TryParse and TryParseExact
- C# DateTime Examples
- C# DateTimePicker Example
- C# Debug.Write Examples
- C# Visual Studio Debugging Tutorial
- C# decimal Examples
- C# DayOfWeek
- C# Enum.Format Method (typeof Enum)
- C# Enum.GetName, GetNames: Get String Array From Enum
- C# Enum.Parse, TryParse: Convert String to Enum
- C# Error and Warning Directives
- C# ErrorProvider Control: Windows Forms
- C# event Examples
- C# Get Every Nth Element From List (Modulo)
- C# Excel Interop Example
- C# Except (Remove Elements From Collection)
- C# Extension Method
- C# extern alias Example
- C# Convert Feet, Inches
- C# File.Delete
- C# File Equals: Compare Files
- C# File.Exists Method
- C# try Keyword
- C# TryGetValue (Get Value From Dictionary)
- C# Tuple Examples
- C# Type Class: Returned by typeof, GetType
- C# TypeInitializationException
- C# Union: Combine and Remove Duplicate Elements
- C# Unreachable Code Detected
- C# Unsafe Keyword: Fixed, Pointers
- C# Uppercase First Letter
- C# Uri and UriBuilder Classes
- C# Using Alias Example
- C# using Statement: Dispose and IDisposable
- C# value Keyword
- C# ValueTuple Examples (System.ValueTuple, ToTuple)
- C# ValueType Examples
- C# Variable Initializer for Class Field
- C# Word Count
- C# Word Interop: Microsoft.Office.Interop.Word
- C# XElement Example (XElement.Load, XName)
- C# Zip Method (Use Lambda on Two Collections)
- C# File.Move Method, Rename File
- C# File.Open Examples
- C# File.ReadAllBytes, Get Byte Array From File
- C# File.ReadAllLines, Get String Array From File
- C# File.ReadAllText, Get String From File
- C# File.ReadLines, Use foreach Over Strings
- C# File.Replace Method
- C# FileInfo Length, Get File Size
- C# FileInfo Examples
- C# File Handling
- C# Filename With Date Example (DateTime.Now)
- C# FileNotFoundException (catch Example)
- C# FileStream Length, Get Byte Count From File
- C# FileStream Example, File.Create
- C# FileSystemWatcher Tutorial (Changed, e.Name)
- C# finally Keyword
- C# First Sentence
- C# FirstOrDefault (Get First Element If It Exists)
- C# Fisher Yates Shuffle: Generic Method
- C# fixed Keyword (unsafe)
- C# Flatten Array (Convert 2D to 1D)
- C# First Words in String
- C# First (Get Matching Element With Lambda)
- C# ContainsKey Method (Key Exists in Dictionary)
- C# Convert ArrayList to Array (Use ToArray)
- C# Convert ArrayList to List
- C# Convert Bool to Int
- C# Convert Bytes to Megabytes
- C# Convert Degrees Celsius to Fahrenheit
- C# Convert Dictionary to List
- C# Convert Dictionary to String (Write to File)
- C# Convert List to Array
- C# Convert List to DataTable (DataGridView)
- C# Convert List to String
- C# Convert Miles to Kilometers
- C# Convert Milliseconds, Seconds, Minutes
- C# Convert Nanoseconds, Microseconds, Milliseconds
- C# Convert String Array to String
- C# Convert TimeSpan to Long
- C# Convert Types
- C# Copy Dictionary
- C# Count Elements in Array and List
- C# FromOADate and Excel Dates
- C# Generic Class, Generic Method Examples
- C# GetEnumerator: While MoveNext, Get Current
- C# GetHashCode (String Method)
- C# Thumbnail Image With GetThumbnailImage
- C# GetType Method
- C# Global Variable Examples (Public Static Property)
- ASP.NET Global Variables Example
- C# Group By Operator: LINQ
- GroupBox: Windows Forms
- C# GroupBy Method: LINQ
- C# GroupJoin Method
- C# GZipStream Example (DeflateStream)
- C# HashSet Examples
- C# Hashtable Examples
- HelpProvider Control Use
- C# HTML and XML Handling
- C# HtmlEncode and HtmlDecode
- C# HtmlTextWriter Example
- C# HttpUtility.HtmlEncode Methods
- C# HybridDictionary
- C# default Operator
- C# DefaultIfEmpty Method
- C# Define and Undef Directives
- C# Destructor
- C# DialogResult: Windows Forms
- C# Dictionary, Read and Write Binary File
- C# Dictionary Memory
- C# Dictionary Optimization, Increase Capacity
- C# Dictionary Optimization, Test With ContainsKey
- C# DictionaryEntry Example (Hashtable)
- C# Directives
- C# Directory.CreateDirectory, Create New Folder
- C# Directory.GetFiles Example (Get List of Files)
- C# DivideByZeroException
- C# DllImport Attribute
- C# Do While Loop Example
- C# DriveInfo Examples
- C# DropDownItems Control
- C# IComparable Example, CompareTo: Sort Objects
- C# IDictionary Generic Interface
- C# IEnumerable Examples
- C# IList Generic Interface: List and Array
- C# Image Type
- C# ImageList Use: Windows Forms
- C# Increment String That Contains a Number
- C# Increment, Preincrement and Decrement Ints
- Dot Net Perls
- C# Indexer Examples (This Keyword, get and set)
- C# IndexOutOfRangeException
- C# Inheritance
- C# Insert String Examples
- C# int Array
- C# Interface Examples
- C# Interlocked Examples: Add, CompareExchange
- C# Intersect: Get Common Elements
- C# InvalidCastException
- C# InvalidOperationException: Collection Was Modified
- C# IOException Type: File, Directory Not Found
- C# IOrderedEnumerable (Query Expression With orderby)
- C# is: Cast Examples
- C# IsFixedSize, IsReadOnly and IsSynchronized Arrays
- C# string.IsNullOrEmpty, IsNullOrWhiteSpace
- C# IsSorted Method: If Array Is Already Sorted
- C# Jagged Array Examples
- C# join Examples (LINQ)
- C# KeyCode Property and KeyDown
- C# KeyNotFoundException: Key Not Present in Dictionary
- C# KeyValuePair Examples
- C# Line Count for File
- C# Line Directive
- C# LinkedList
- C# List CopyTo (Copy List Elements to Array)
- C# List Equals (If Elements Are the Same)
- C# List Find and Exists Examples
- C# List Insert Performance
- ASP.NET LiteralControl Example
- C# Locality Optimizations (Memory Hierarchy)
- C# lock Keyword
- C# Long and ulong Types
- C# Loop Over String Chars: Foreach, For
- C# Loop Over String Array
- C# Main args Examples
- C# Map Example
- ASP.NET MapPath: Virtual and Physical Paths
- C# Mask Optimization
- C# MaskedTextBox Example
- C# Math.Abs: Absolute Value
- C# Math.Ceiling Usage
- C# Math.Floor Method
- C# Math.Max and Math.Min Examples
- C# Math.Pow Method, Exponents
- C# Math.Round Examples: MidpointRounding
- C# Math Type
- C# Max and Min: Get Highest or Lowest Element
- C# MemoryFailPoint and InsufficientMemoryException
- C# MemoryStream: Use Stream on Byte Array
- C# MenuStrip: Windows Forms
- C# Modulo Operator: Get Remainder From Division
- C# MonthCalendar Control: Windows Forms
- C# Multiple Return Values
- C# Multiply Numbers
- C# namespace Keyword
- C# NameValueCollection Usage
- C# Nested Lists: Create 2D List or Jagged List
- C# Nested Switch Statement
- C# Environment.NewLine
- C# Normalize, IsNormalized Methods
- C# Null String Example
- C# null Keyword
- C# Nullable Examples
- C# NullReferenceException and Null Parameter
- C# Object Array
- C# Obsolete Attribute
- C# OfType Examples
- C# OpenFileDialog Example
- C# operator Keyword
- C# Odd and Even Numbers
- C# Bitwise Or
- C# orderby Query Keyword
- C# out Parameter
- C# OutOfMemoryException
- C# OverflowException
- C# Overload Method
- C# Override Method
- C# PadRight and PadLeft: String Columns
- C# Get Paragraph From HTML With Regex
- C# Parallel.For Example (Benchmark)
- C# Parallel.Invoke: Run Methods on Separate Threads
- C# Parameter Optimization
- C# Parameter Passing, ref and out
- C# params Keyword
- C# int.Parse: Convert Strings to Integers
- C# partial Keyword
- C# Path.ChangeExtension
- C# Path Exists Example
- C# Path.GetExtension: File Extension
- C# Path.GetRandomFileName Method
- C# Pragma Directive
- C# Predicate (Lambda That Returns True or False)
- C# Pretty Date Format (Hours or Minutes Ago)
- C# PreviewKeyDown Event
- C# Random Lowercase Letter
- C# Random Paragraphs and Sentences
- C# Random String
- C# Random Number Examples
- C# StreamReader ReadLine, ReadLineAsync Examples
- C# readonly Keyword
- C# Recursion Example
- C# Regex, Read and Match File Lines
- C# Regex Groups, Named Group Example
- C# Regex.Matches Quote Example
- C# Regex.Matches Method: foreach Match, Capture
- C# Regex.Replace, Matching End of String
- C# Regex.Replace, Remove Numbers From String
- C# Regex.Replace, Merge Multiple Spaces
- C# Regex.Replace Examples: MatchEvaluator
- C# Regex.Split, Get Numbers From String
- C# Regex.Split Examples
- C# Regex Trim, Remove Start and End Spaces
- C# RegexOptions.Compiled
- C# RegexOptions.IgnoreCase Example
- C# RegexOptions.Multiline
- C# Region and endregion
- C# Remove Char From String at Index
- C# Remove Element
- C# Remove HTML Tags
- C# Remove String
- C# Reserved Filenames
- C# return Keyword
- C# Reverse String
- C# Reverse Words
- C# Reverse Extension Method
- C# RichTextBox Example
- C# Right String Part
- C# RNGCryptoServiceProvider Example
- C# ROT13 Method, Char Lookup Table
- C# SelectMany Example: LINQ
- C# Sentinel Optimization
- C# SequenceEqual Method (If Two Arrays Are Equal)
- C# Shift Operators (Bitwise)
- C# Short and ushort Types
- C# Single Method: Get Element If Only One Matches
- C# SingleOrDefault
- C# Singleton Pattern Versus Static Class
- C# Singleton Class
- C# sizeof Keyword
- C# Skip and SkipWhile Examples
- C# Sleep Method (Pause)
- C# Sort Dictionary: Keys and Values
- C# Sort by File Size
- C# Sort, Ignore Leading Chars
- C# Sort KeyValuePair List: CompareTo
- C# Sort Strings by Length
- C# Thread.SpinWait Example
- C# Math.Sqrt Method
- C# stackalloc Operator
- C# StackOverflowException
- C# StartsWith and EndsWith String Methods
- C# Static Array
- C# Static Dictionary
- C# Stopwatch Examples
- C# Stream
- C# StreamReader ReadToEnd Example (Read Entire File)
- C# StreamReader ReadToEndAsync Example (Performance)
- C# StreamReader Examples
- C# StreamWriter Examples
- C# String Append (Add Strings)
- C# String Compare and CompareTo Methods
- C# String Constructor (new string)
- C# string.Copy Method
- C# CopyTo String Method: Put Chars in Array
- C# Empty String Examples
- C# String Equals Examples
- C# String For Loop, Count Spaces in String
- C# string.Intern and IsInterned
- C# String IsUpper, IsLower
- C# String Length Property: Get Character Count
- C# String Literal: Newline and Quote Examples
- C# StringBuilder Capacity
- C# StringBuilder Clear (Set Length to Zero)
- C# StringBuilder Data Types
- C# StringBuilder Performance
- C# StringBuilder Equals (If Chars Are Equal)
- C# StringBuilder Memory
- C# StringBuilder ToString: Get String From StringBuilder
- C# StringWriter Class
- C# Sum Method: Add up All Numbers
- C# Switch Char, Test Chars With Cases
- C# Switch Enum
- C# System (using System namespace)
- C# Tag Property: Windows Forms
- C# TextInfo Examples
- C# TextReader, Returned by File.OpenText
- C# TextWriter, Returned by File.CreateText
- C# this Keyword
- C# ThreadPool
- C# Thread Join Method (Join Array of Threads)
- C# ThreadPool.SetMinThreads Method
- C# TimeZone Examples
- C# Get Title From HTML With Regex
- C# ToArray Extension Method
- C# ToCharArray: Convert String to Array
- C# ToDictionary Method
- C# Token
- C# ToList Extension Method
- C# ToLookup Method (Get ILookup)
- C# ToLower and ToUpper: Uppercase and Lowercase Strings
- ToolStripContainer Control: Dock, Properties
- C# ToolTip: Windows Forms
- C# ToString Integer Optimization
- C# ToString: Get String From Object
- C# ToTitleCase Method
- C# TrackBar: Windows Forms
- C# Tree and Nodes Example: Directed Acyclic Word Graph
- C# TreeView Tutorial
- C# Trim Strings
- C# Thread Methods
- C# History
- C# Features
- C# Variables
- C# Data Types
- C# Operators
- C# Keywords
- C# New Features | C# Version Features
- C# Programs
- C# Program to swap numbers without third variable
- C# Program to convert Decimal to Binary
- C# Program to Convert Number in Characters
- C# Program to Print Alphabet Triangle
- C# Program to print Number Triangle
- C# Program to generate Fibonacci Triangle
- C# String Compare() method
- C# String CompareOrdinal() method
- C# String CompareTo() method
- C# String Concat() method
- C# String Contains() method
- C# String CopyTo() method
- C# String EndsWith() method
- C# String Equals() method
- C# String Format() method
- C# String IndexOf() method
- C# String Insert() method
- C# String Intern(String str) method
- C# String IsInterned() method
- C# String Normalize() method
- C# String IsNullOrEmpty() method
- C# String IsNullOrWhiteSpace() method
- C# String Join() method
- C# String LastIndexOf() method
- C# String LastIndexOfAny() method
- C# String PadLeft() method
- C# String PadRight() method
- C# Nullable
- C# String Remove() method
- C# String Replace() method
- C# String Split() method
- C# String StartsWith() method
- C# String SubString() method
- C# Partial Types
- C# Iterators
- C# Delegate Covariance
- C# Delegate Inference
- C# Anonymous Types
- C# Extension Methods
- C# Query Expression
- C# Partial Method
- C# Implicitly Typed Local Variable
- C# Object and Collection Initializer
- C# Auto Implemented Properties
- C# Dynamic Binding
- C# Named and Optional Arguments
- C# Asynchronous Methods
- C# Caller Info Attributes
- C# Using Static Directive
- C# Exception Filters
- C# Await in Catch Finally Blocks
- C# Default Values for Getter Only Properties
- C# Expression Bodied Members
- C# Null Propagator
- C# String Interpolation
- C# nameof operator
- C# Dictionary Initializer
- C# Pattern Matching
- C# Tuples
- C# Deconstruction
- C# Local Functions
- C# Binary Literals
- C# Ref Returns and Locals
- C# Expression Bodied Constructors and Finalizers
- C# Expression Bodied Getters and Setters
- C# Async Main
- C# Default Expression
Related Links
Adjectives
Ado
Ai
Android
Angular
Antonyms
Apache
Articles
Asp
Autocad
Automata
Aws
Azure
Basic
Binary
Bitcoin
Blockchain
C
Cassandra
Change
Coa
Computer
Control
Cpp
Create
Creating
C-Sharp
Cyber
Daa
Data
Dbms
Deletion
Devops
Difference
Discrete
Es6
Ethical
Examples
Features
Firebase
Flutter
Fs
Git
Go
Hbase
History
Hive
Hiveql
How
Html
Idioms
Insertion
Installing
Ios
Java
Joomla
Js
Kafka
Kali
Laravel
Logical
Machine
Matlab
Matrix
Mongodb
Mysql
One
Opencv
Oracle
Ordering
Os
Pandas
Php
Pig
Pl
Postgresql
Powershell
Prepositions
Program
Python
React
Ruby
Scala
Selecting
Selenium
Sentence
Seo
Sharepoint
Software
Spellings
Spotting
Spring
Sql
Sqlite
Sqoop
Svn
Swift
Synonyms
Talend
Testng
Types
Uml
Unity
Vbnet
Verbal
Webdriver
What
Wpf