<< Back to C-SHARP
C# List Examples
Create a new List and add elements to it. Loop over its elements with for and foreach.List. As millions of years pass, layers of rock are added to the ground. The fossil record is a list. In a program, we could use a List collection to represent this.
List details. The List is initialized with the new keyword. When we call Add, the List adjusts its size as needed. It is used in nearly all larger C# programs.
Initialize List
First example. Here we create 2 separate lists of ints. We add 4 prime numbers to each List. The values are stored in the order added—2, 3, 5 and then 7.
Version 1: We call Add() 4 times with the number as the argument. The end count of the list is 4.
AddVersion 2: This code adds all 4 numbers in a single expression—it is easier to read, and the code generated is the same.
Note: The angle brackets are part of the declaration type. They are not conditional (less or more than) operators.
C# program that uses List, Add, initializer
using System;
using System.Collections.Generic;
class Program
{
static void Main()
{
// Version 1: create a List of ints.
// ... Add 4 ints to it.
var numbers = new List<int>();
numbers.Add(2);
numbers.Add(3);
numbers.Add(5);
numbers.Add(7);
Console.WriteLine("LIST 1: " + numbers.Count);
// Version 2: create a List with an initializer.
var numbers2 = new List<int>() { 2, 3, 5, 7 };
Console.WriteLine("LIST 2: " + numbers2.Count);
}
}
Output
LIST 1: 4
LIST 2: 4
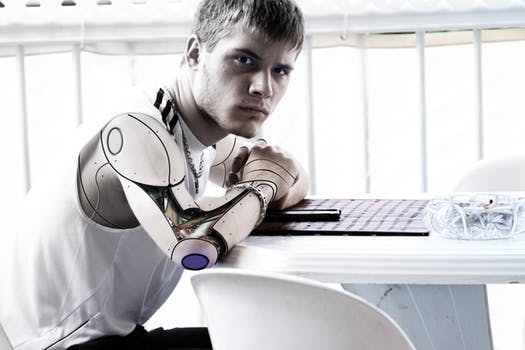
Foreach-loop. This is the clearest loop when no index is needed. It automatically sets each element to a name we specify—here we use the identifier "prime."
Part A: We use the "foreach" keyword and declare a variable that is assigned to each element as we pass over it.
Part B: In the foreach-loop, we access the "prime" variable, which is the current element. We print each int to the screen.
C# program that uses foreach, List
using System.Collections.Generic;
class Program
{
static void Main()
{
List<int> list = new List<int>() { 2, 3, 7 };
// Part A: loop through List with foreach.
foreach (int prime in list)
{
// Part B: access each element with name.
System.Console.WriteLine("PRIME ELEMENT: {0}", prime);
}
}
}
Output
PRIME ELEMENT: 2
PRIME ELEMENT: 3
PRIME ELEMENT: 7
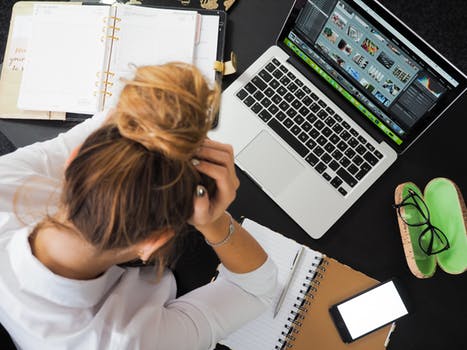
For-loop. Sometimes we want to access indexes of a List as we loop over its elements. With the index, we can test other collections, or perform validations. A for-loop is ideal here.
Part A: Here we use a for-loop. We begin at zero. We end at Count: the Count property returns the number of elements in the List.
Part B: We print each element index with Console.WriteLine. We use a string interpolation expression to format the string.
C# program that uses for, List
using System;
using System.Collections.Generic;
class Program
{
static void Main()
{
List<int> list = new List<int>(new int[]{ 2, 3, 7 });
// Part A: loop with for and access count.
for (int i = 0; i < list.Count; i++)
{
// Part B: access element with index.
Console.WriteLine($"{i} = {list[i]}");
}
}
}
Output
0 = 2
1 = 3
2 = 7
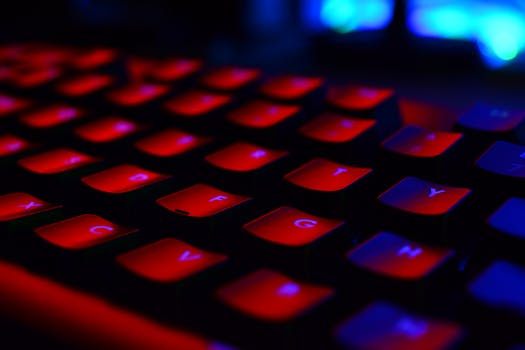
For-loop, reverse. Much like an array, we can access the elements in a List in any way we like. We can loop over the List elements in reverse with a for-loop.
Start: For a reverse loop, we must access the last element first, so we get the Count and subtract one from it. This is the last index.
C# program that uses for reverse, List
using System;
using System.Collections.Generic;
class Program
{
static void Main()
{
var votes = new List<bool> { false, false, true };
// Loop through votes in reverse order.
for (int i = votes.Count - 1; i >= 0; i--)
{
Console.WriteLine("DECREMENT LIST LOOP: {0}", votes[i]);
}
}
}
Output
DECREMENT LIST LOOP: True
DECREMENT LIST LOOP: False
DECREMENT LIST LOOP: False
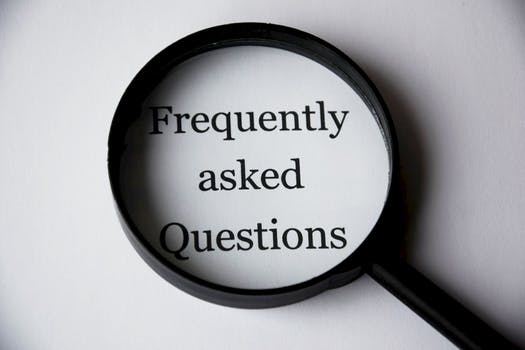
AddRange, InsertRange. For adding many elements at once, we use the InsertRange and AddRange methods. InsertRange can insert at an index, while AddRange adds at the end.
AddRange, InsertRangeArgument 1: The first argument to InsertRange here is the position (index) where we want to insert new elements in the List.
Argument 2: The second argument to InsertRange is an IEnumerable—here, a string array. These strings are inserted at the index specified.
Result: We create a List of 2 strings, then add 2 strings from an array to index 1. The result List has 4 strings.
C# program that uses AddRange
using System;
using System.Collections.Generic;
class Program
{
static void Main()
{
// Create a list of 2 strings.
var animals = new List<string>() { "bird", "dog" };
// Insert strings from an array in position 1.
animals.InsertRange(1, new string[] { "frog", "snake" });
foreach (string value in animals)
{
Console.WriteLine("RESULT: " + value);
}
}
}
Output
RESULT: bird
RESULT: frog
RESULT: snake
RESULT: dog
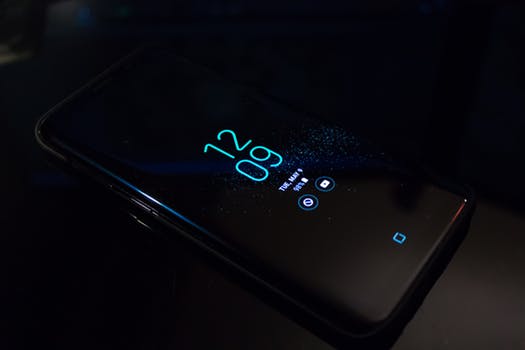
Count, clear. To get the number of elements, access the Count property. This is fast—just avoid the Count extension method. Count, on the List type, is equal to Length on arrays.
Clear: Here we use the Clear method, along with the Count property, to erase all the elements in a List.
ClearInfo: Before Clear is called, this List has 3 elements. After Clear is called, it has 0 elements.
Null: We can assign the List to null instead of calling Clear, with similar performance.
C# program that counts List
using System;
using System.Collections.Generic;
class Program
{
static void Main()
{
List<bool> list = new List<bool>();
list.Add(true);
list.Add(false);
list.Add(true);
Console.WriteLine(list.Count); // 3
list.Clear();
Console.WriteLine(list.Count); // 0
}
}
Output
3
0
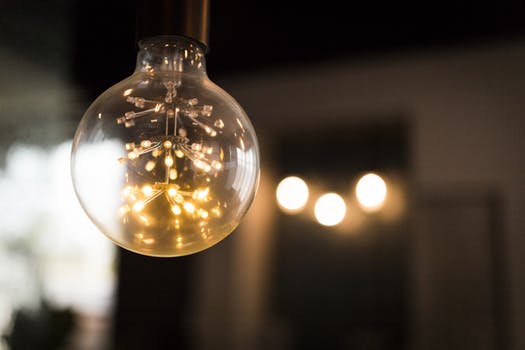
Copy array. Here we create a List with elements from an array. We use the List constructor and pass it the array. List receives this parameter and fills its values from it.
Caution: The array element type must match the List element type or compilation will fail.
C# program that copies array to List
using System;
using System.Collections.Generic;
class Program
{
static void Main()
{
// Create new array with 3 elements.
int[] array = new int[] { 2, 3, 5 };
// Copy the array to a List.
List<int> copied = new List<int>(array);
// Print size of List.
Console.WriteLine("COPIED COUNT: {0}", copied.Count);
}
}
Output
COPIED COUNT: 3
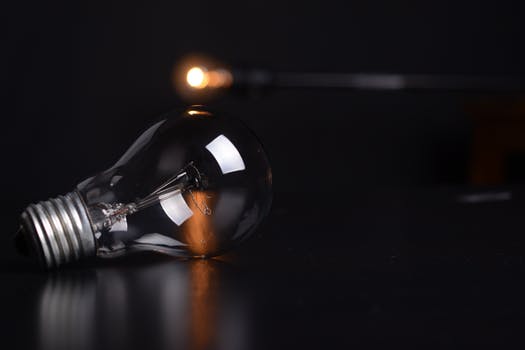
List parameter. Lists do not exist just to be allocated and looped over. We want to test and examine elements to solve real-world problems in our programs.
Main: Here we pass a List to ContainsValue300. The List can be passed as an argument—only the reference, not all elements, are copied.
ContainsValue300: This method uses a foreach-loop, which tests to see if 300 is in a list of numbers.
IfResult: The values List contains the value 300, so our custom method returns the boolean true.
C# program that uses foreach
using System;
using System.Collections.Generic;
class Program
{
static void Main()
{
var values = new List<int>() { 200, 300, 500 };
// Pass list the method.
if (ContainsValue300(values))
{
Console.WriteLine("RETURNED TRUE");
}
}
static bool ContainsValue300(List<int> list)
{
foreach (int number in list)
{
// See if the element in the list equals 300.
if (number == 300)
{
return true;
}
}
// No return was reached, so nothing matched.
return false;
}
}
Output
RETURNED TRUE
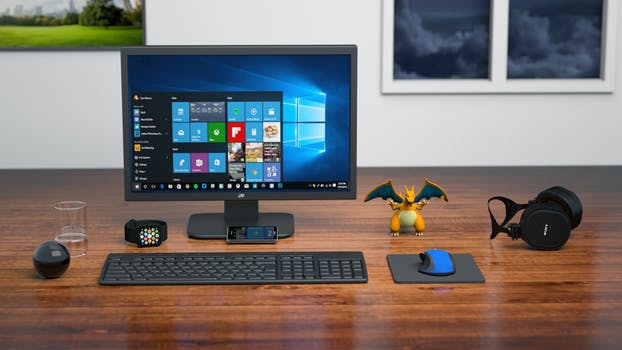
IndexOf. This determines the element index of a certain value in the List collection. It searches for the first position (from the start) of the value.
Note: IndexOf has two overloads. It works in the same way as string's IndexOf. It searches by value and returns the location.
C# program that uses IndexOf
using System;
using System.Collections.Generic;
class Program
{
static void Main()
{
List<int> primes = new List<int>(new int[] { 19, 23, 29 });
int index = primes.IndexOf(23); // Exists.
Console.WriteLine(index);
index = primes.IndexOf(10); // Does not exist.
Console.WriteLine(index);
}
}
Output
1
-1
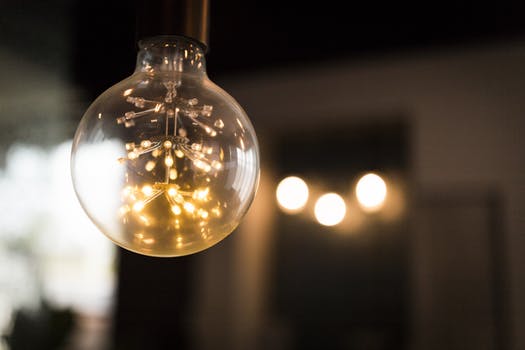
ForEach. This is a method. Sometimes we may not want to write a traditional foreach-loop. Here ForEach is useful. It accepts an Action.
Example: We have a 2-element string List. Then we call ForEach with a lambda that writes each element "a" to the console.
C# program that uses ForEach on List
using System;
using System.Collections.Generic;
class Program
{
static void Main()
{
var animals = new List<string>() { "bird", "dog" };
// Use ForEach with a lambda action.
// ... Write each string to the console.
animals.ForEach(a => Console.WriteLine("ANIMAL: " + a));
}
}
Output
ANIMAL: bird
ANIMAL: dog
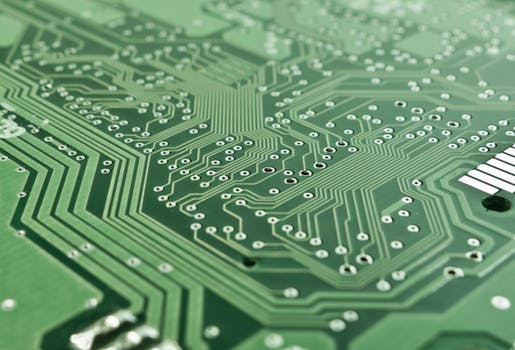
TrueForAll. This method accepts a Predicate. If the Predicate returns true for each element in the List, TrueForAll() will also return true.
And: TrueForAll() checks the entire list—unless an element doesn't match (it has an early exit condition).
C# program that uses TrueForAll on List
using System;
using System.Collections.Generic;
class Program
{
static void Main()
{
var numbers = new List<int> { 10, 11, 12 };
// Call TrueForAll to ensure a condition is true.
if (numbers.TrueForAll(element => element < 20))
{
Console.WriteLine("All elements less than 20");
}
}
}
Output
All elements less than 20
Join string list. Next we use string.Join on a List of strings. This is helpful when we need to turn several strings into one comma-delimited string.
ToArray: It requires the ToArray instance method on List. This ToArray is not an extension method.
Tip: The biggest advantage of Join here is that no trailing comma is present on the resulting string.
C# program that joins List
using System;
using System.Collections.Generic;
class Program
{
static void Main()
{
// List of cities we need to join.
List<string> cities = new List<string>();
cities.Add("New York");
cities.Add("Mumbai");
cities.Add("Berlin");
cities.Add("Istanbul");
// Join strings into one CSV line.
string line = string.Join(",", cities.ToArray());
Console.WriteLine(line);
}
}
Output
New York,Mumbai,Berlin,Istanbul
Keys in Dictionary. We use the List constructor to get a List of keys from a Dictionary. This is a simple way to iterate over Dictionary keys (or store them elsewhere).
Keys: The Keys property returns an enumerable collection of keys. But a List of these elements is more usable.
C# program that converts Keys
using System;
using System.Collections.Generic;
class Program
{
static void Main()
{
// Populate example Dictionary.
var dict = new Dictionary<int, bool>();
dict.Add(3, true);
dict.Add(5, false);
// Get a List of all the Keys.
List<int> keys = new List<int>(dict.Keys);
foreach (int key in keys)
{
Console.WriteLine(key);
}
}
}
Output
3, 5
Insert. This is a useful but slow method. The string here is inserted at index 1. This makes it the second element. If you Insert often, consider Queue and LinkedList.
InsertAlso: A Queue may allow simpler usage of the collection in our code. This may be easier to understand.
QueueC# program that inserts into List
using System;
using System.Collections.Generic;
class Program
{
static void Main()
{
List<string> dogs = new List<string>(); // Example list.
dogs.Add("spaniel"); // Contains: spaniel.
dogs.Add("beagle"); // Contains: spaniel, beagle.
dogs.Insert(1, "dalmatian"); // Spaniel, dalmatian, beagle.
foreach (string dog in dogs) // Display for verification.
{
Console.WriteLine(dog);
}
}
}
Output
spaniel
dalmatian
beagle
Remove. With this method we eliminate the first matching element in the List. If we pass the value 20 to Remove, the first element in the list with value 20 is removed.
Info: We can call Remove with an argument that does not occur in the list. This will not cause an exception.
List RemoveRemoveAll: We can remove more than 1 element at once with the RemoveAll method.
RemoveAllC# program that uses Remove
using System;
using System.Collections.Generic;
class Program
{
static void Main()
{
var numbers = new List<int>() { 10, 20, 30 };
// Remove this element by value.
numbers.Remove(20);
foreach (int number in numbers)
{
Console.WriteLine("NOT REMOVED: {0}", number);
}
// This has no effect.
numbers.Remove(2000);
}
}
Output
NOT REMOVED: 10
NOT REMOVED: 30
Reverse. With this method no sorting occurs—the original order is intact but inverted. The strings contained in the List are left unchanged.
Array.ReverseInternally: This method invokes the Array.Reverse method. Many list methods are implemented with Array methods.
C# program that uses Reverse
using System;
using System.Collections.Generic;
class Program
{
static void Main()
{
List<string> list = new List<string>();
list.Add("anchovy");
list.Add("barracuda");
list.Add("bass");
list.Add("viperfish");
// Reverse List in-place, no new variables required.
list.Reverse();
foreach (string value in list)
{
Console.WriteLine(value);
}
}
}
Output
viperfish
bass
barracuda
anchovy
GetRange. This returns a range of elements in a List. This is similar to the Take and Skip methods from LINQ. It has different syntax. The result List can be used like any other List.
LINQC# program that gets ranges from List
using System;
using System.Collections.Generic;
class Program
{
static void Main()
{
List<string> rivers = new List<string>(new string[]
{
"nile",
"amazon", // River 2.
"yangtze", // River 3.
"mississippi",
"yellow"
});
// Get rivers 2 through 3.
List<string> range = rivers.GetRange(1, 2);
foreach (string river in range)
{
Console.WriteLine(river);
}
}
}
Output
amazon
yangtze
SequenceEqual. This is a method from System.Linq. It tells us whether 2 collections (IEnumerables) have the same exact elements. The number of elements and order must be the same.
Tip: We can use SequenceEqual on Lists, arrays, or other collections. It is not optimally fast, but it can reduce code size.
SequenceEqualC# program that uses SequenceEqual
using System;
using System.Collections.Generic;
using System.Linq;
class Program
{
static void Main()
{
var numbers = new List<int> { 10, 20, 30 };
var numbers2 = new List<int> { 10, 20, 30 };
// See if the two lists are equal.
if (numbers.SequenceEqual(numbers2))
{
Console.WriteLine("LISTS ARE EQUAL");
}
}
}
Output
LISTS ARE EQUAL
Var keyword. This shortens lines of code, which sometimes improves readability. Var has no effect on performance, only readability for programmers.
VarC# program that uses var with List
using System.Collections.Generic;
class Program
{
static void Main()
{
var list1 = new List<int>(); // Var keyword used.
List<int> list2 = new List<int>(); // This is equivalent.
}
}
Benchmark, create List. Suppose we want to create a List of 3 elements with an initializer. If we can use an array instead, the program will be faster.
Version 1: We create a string array of 3 elements with an array initializer. We test its length.
Initialize ArrayVersion 2: This code creates a List of strings with an initializer. The List is an additional object—performance is affected.
Result: It is faster to allocate an array of strings. For some programs, this could help improve performance.
C# program that tests List creation time
using System;
using System.Collections.Generic;
using System.Diagnostics;
class Program
{
const int _max = 1000000;
static void Main()
{
// Version 1: create string array with 3 elements in it.
var s1 = Stopwatch.StartNew();
for (int i = 0; i < _max; i++)
{
var items = new string[] { "bird", "frog", "fish" };
if (items.Length == 0)
{
return;
}
}
s1.Stop();
// Version 2: create string list with 3 elements in it.
var s2 = Stopwatch.StartNew();
for (int i = 0; i < _max; i++)
{
var items = new List<string>() { "bird", "frog", "fish" };
if (items.Count == 0)
{
return;
}
}
s2.Stop();
Console.WriteLine(((double)(s1.Elapsed.TotalMilliseconds * 1000000) /
_max).ToString("0.00 ns"));
Console.WriteLine(((double)(s2.Elapsed.TotalMilliseconds * 1000000) /
_max).ToString("0.00 ns"));
}
}
Output
14.03 ns Create string array
40.79 ns Create string List
Contains, Find and Exist. These methods all provide searching. They vary in arguments accepted. With Predicates, we influence what elements match.
List ContainsList Find, Exists
Capacity. We can use the Capacity property on List, or pass an integer to the constructor (which sets an initial capacity) to improve allocation performance.
CapacityNote: Setting a capacity sometimes improves performance by nearly two times for adding elements.
However: Adding elements, and resizing List, is not usually a performance bottleneck in programs that access data.
Sort. This orders the elements in the List. For strings it orders alphabetically. For integers (or other numbers) it orders from lowest to highest.
SortNote: Sort acts upon elements depending on type. It is possible to provide a custom method.
TrimExcess. This method's usage is limited. It reduces the memory used by lists with large capacities. And as Microsoft states, TrimExcess often does nothing.
Note: It is unclear how TrimExcess feels about its status. I wouldn't want to upset its feelings.
Quote: The TrimExcess method does nothing if the list is at more than 90 percent of capacity.
List.TrimExcess: Microsoft DocsBinarySearch. This implements (fittingly) the binary search algorithm. Binary search uses guesses to find the correct element faster than linear searching.
BinarySearchConversion. We can convert a List to an array of the same type using the instance method ToArray. There are examples of these conversions.
List to ArrayCopyToList to string: Some string methods are used with lists. We use Concat and Join. Sometimes StringBuilder is also useful.
Convert List, StringConcatJoin: string.JoinDataTable: Sometimes it is better to convert a List to a DataTable. For a List of string arrays, this will work better.
Convert List, DataTableEquality. Sometimes we need to test two Lists for equality, even when their elements are unordered. We can sort and then compare, or use a custom List equality method.
List Element EqualityStructs. When using List, we can improve performance and reduce memory usage with structs instead of classes. A List of structs is allocated in contiguous memory, unlike a List of classes.
However: Using structs will actually decrease performance when they are used as parameters in methods such as those on the List type.
StructsGetEnumerator. Programs are built upon many abstractions. With List, even loops can be abstracted (into an Enumerator). We use the same methods to loop over a List or an array.
GetEnumeratorCombine lists. With Concat, a method from the System.Linq namespace, we can add one list to another. Only a single method call is required.
Concat, ListRemove duplicates. With Distinct() we can remove duplicates from a List. Other algorithms, that use Dictionary, can be used to scan for and erase duplicates.
Dedupe ListSerialize list. A List can be read from, and written to, a file. This is list serialization. The "Serializable" attribute is useful here.
Serialize ListNotes, List. This generic (like all others) is created with a type parameter. List is powerful: it provides flexible allocation and growth. Lists can be nested, static, or assigned to null.
Nested ListNull ListStatic ListA summary. List's syntax is at first confusing. But we become used to it. In most programs lacking strict memory or performance constraints, List is ideal.
Related Links:
- C# Array Examples, String Arrays
- C# ArrayList Examples
- C# ArraySegment: Get Array Range, Offset and Count
- C# break Statement
- C# Buffer BlockCopy Example
- C# BufferedStream: Optimize Read and Write
- 404 Not Found
- C# 24 Hour Time Formats
- C# 2D Array Examples
- 7 Zip Command Line Examples
- C# 7 Zip Executable (Process.Start)
- C# All Method: All Elements Match a Condition
- C# Alphabetize String
- C# Alphanumeric Sorting
- C# Arithmetic Expression Optimization
- C# Array.AsReadOnly Method (ObjectModel)
- C# Array.BinarySearch Method
- C# Array.Clear Examples
- C# Array.IndexOf, LastIndexOf: Search Arrays
- C# async, await Examples
- C# Attribute Examples
- C# Average Method
- C# BackgroundWorker
- C# base Keyword
- C# String Between, Before, After
- C# Binary Representation int (Convert, toBase 2)
- C# BinarySearch List
- C# bool Array (Memory Usage, One Byte Per Element)
- C# bool.Parse, TryParse: Convert String to Bool
- C# bool Type
- C# Array Length Property, Get Size of Array
- C# Button Example
- C# Byte Array: Memory Usage, Read All Bytes
- C# Byte and sbyte Types
- C# Capacity for List, Dictionary
- C# Case Insensitive Dictionary
- C# case Example (Switch Case)
- C# Char Array
- C# Checked and Unchecked Keywords
- C# CheckedListBox: Windows Forms
- C# Color Table
- C# Color Examples: FromKnownColor, FromName
- C# ColorDialog Example
- C# Comment: Single Line and Multiline
- C# Concat Extension: Combine Lists and Arrays
- C# Conditional Attribute
- C# Console Color, Text and BackgroundColor
- C# String Clone() method
- C# Constructor Examples
- C# Contains Extension Method
- C# String GetTypeCode() method
- C# String ToLowerInvariant() method
- C# Customized Dialog Box
- C# DataColumn Example: Columns.Add
- C# DataGridView Add Rows
- DataGridView Columns, Edit Columns Dialog
- C# DataGridView Row Colors (Alternating)
- C# DataGridView Tutorial
- C# DataGridView
- C# DataRow Examples
- C# DataSet Examples
- C# DataSource Example
- C# DataTable Compare Rows
- C# DataTable foreach Loop
- C# DataTable RowChanged Example: AcceptChanges
- C# DataTable Select Example
- C# DataTable Examples
- C# DataView Examples
- C# String ToString() method
- C# String ToUpper() method
- C# Digit Separator
- C# DateTime.MinValue (Null DateTime)
- C# DateTime.Month Property
- C# DateTime.Parse: Convert String to DateTime
- C# DateTime Subtract Method
- C# Decompress GZIP
- C# Remove Duplicates From List
- C# dynamic Keyword
- C# ElementAt, ElementAtOrDefault Use
- C# Encapsulate Field
- C# Enum Array Example, Use Enum as Array Index
- C# enum Flags Attribute Examples
- C# Enum ToString: Convert Enum to String
- C# enum Examples
- C# Enumerable.Range, Repeat and Empty
- C# Environment Type
- C# EventLog Example
- C# Exception Handling
- C# explicit and implicit Keywords
- C# Factory Design Pattern
- C# File.Copy Examples
- C# typeof and nameof Operators
- C# String TrimEnd() method
- C# var Examples
- C# virtual Keyword
- C# void Method, Return No Value
- C# volatile Example
- C# WebBrowser Control (Navigate Method)
- C# WebClient: DownloadData, Headers
- C# Where Method and Keyword
- C# String TrimStart() method
- C# delegate Keyword
- C# descending, ascending Keywords
- C# while Loop Examples
- C# Whitespace Methods: Convert UNIX, Windows Newlines
- C# XmlReader, Parse XML File
- C# XmlTextReader
- C# XmlTextWriter
- C# XmlWriter, Create XML File
- C# XOR Operator (Bitwise)
- C# yield Example
- C# float Numbers
- FlowLayoutPanel Control
- C# Focused Property
- C# FolderBrowserDialog Control
- C# Font Type: FontFamily and FontStyle
- C# FontDialog Example
- C# for Loop Examples
- C# foreach Loop Examples
- ForeColor, BackColor: Windows Forms
- C# Form: Event Handlers
- C# Contains String Method
- C# ContainsValue Method (Value Exists in Dictionary)
- C# ContextMenuStrip Example
- C# continue Keyword
- C# Control: Windows Forms
- C# Windows Forms Controls
- C# Convert Char Array to String
- C# Convert Char to String
- C# Convert Days to Months
- C# Convert String to Byte Array
- C# String Format
- C# Func Object (Lambda That Returns a Value)
- C# GC.Collect Examples: CollectionCount, GetTotalMemory
- C# Path.GetDirectoryName (Remove File From Path)
- C# goto Examples
- C# HttpClient Example: System.Net.Http
- ASP.NET HttpContext Request Property
- IL Disassembler Tutorial
- C# Intermediate Language (IL)
- C# IndexOf Examples
- C# IndexOfAny Examples
- C# Initialize Array
- C# Initialize List
- C# InitializeComponent Method: Windows Forms
- C# Inline Optimization
- C# Dictionary Equals: If Contents Are the Same
- C# Dictionary Versus List Loop
- C# Dictionary Order, Use Keys Added Last
- C# Dictionary Size and Performance
- C# Dictionary Versus List Lookup Time
- C# Dictionary Examples
- C# Get Directory Size (Total Bytes in All Files)
- C# Directory Type
- C# Distinct Method, Get Unique Elements Only
- C# Divide by Powers of Two (Bitwise Shift)
- C# Divide Numbers (Cast Ints)
- C# DomainUpDown Control Example
- C# Double Type: double.MaxValue, double.Parse
- C# Remove Duplicate Chars
- C# IEqualityComparer
- C# If Preprocessing Directive: Elif and Endif
- C# If Versus Switch Performance
- C# if Statement
- C# int.MaxValue, MinValue (Get Lowest Number)
- C# Program to reverse number
- C# Int and uint Types
- C# Integer Append Optimization
- C# Keywords
- C# Label Example: Windows Forms
- C# Lambda Expressions
- C# LastIndexOf Examples
- C# Last, LastOrDefault Methods
- C# Mutex Example (OpenExisting)
- C# Named Parameters
- C# Let Keyword (Use Variable in Query Expression)
- C# Levenshtein Distance
- C# LinkLabel Example: Windows Forms
- C# LINQ
- C# List Add Method, Append Element to List
- C# List AddRange, InsertRange (Append Array to List)
- C# List Clear Example
- C# List Contains Method
- C# List Remove Examples
- C# List Examples
- C# ListBox Tutorial (DataSource, SelectedIndex)
- C# ListView Tutorial: Windows Forms
- C# Maze Pathfinding Algorithm
- C# Memoization
- C# Memory Usage for Arrays of Objects
- C# MessageBox.Show Examples
- C# Method Call Depth Performance
- C# Method Parameter Performance
- C# Method Size Optimization
- C# Multidimensional Array
- C# MultiMap Class (Dictionary of Lists)
- C# Optimization
- C# new Keyword
- C# NotifyIcon: Windows Forms
- C# NotImplementedException
- C# Null Array
- C# String GetType() method
- C# Null Coalescing and Null Conditional Operators
- C# Null List (NullReferenceException)
- C# Numeric Casts
- C# NumericUpDown Control: Windows Forms
- C# object.ReferenceEquals Method
- C# Object Examples
- C# Optional Parameters
- C# Prime Number
- C# OrderBy, OrderByDescending Examples
- C# Process Examples (Process.Start)
- Panel, Windows Forms (Create Group of Controls)
- C# Path Examples
- C# Get Percentage From Number With Format String
- ASP.NET PhysicalApplicationPath
- C# PictureBox: Windows Forms
- C# PNG Optimization
- C# Position Windows: Use WorkingArea and Location
- Visual Studio Post Build, Pre Build Macros
- C# ProfileOptimization
- C# ProgressBar Example
- C# Property Examples
- C# PropertyGrid: Windows Forms
- C# Protected and internal Keywords
- C# Public and private Methods
- C# Remove Punctuation From String
- C# Query Windows Forms (Controls.OfType)
- C# Queryable: IQueryable Interface and AsQueryable
- ASP.NET QueryString Examples
- C# Queue Collection: Enqueue
- C# RadioButton Use: Windows Forms
- C# ReadOnlyCollection Use (ObjectModel)
- C# Recursion Optimization
- C# Recursive File List: GetFiles With AllDirectories
- C# ref Keyword
- C# Reflection Examples
- C# Regex.Escape and Unescape Methods
- C# StringBuilder Examples
- C# StringComparison and StringComparer
- C# StringReader Class (Get Parts of Strings)
- C# String GetEnumerator() method
- C# String GetHashCode() method
- C# Regex Versus Loop: Use For Loop Instead of Regex
- C# Regex.Match Examples: Regular Expressions
- C# RemoveAll: Use Lambda to Delete From List
- C# Replace String Examples
- ASP.NET Response.BinaryWrite
- ASP.NET Response.Write
- C# Return Optimization: out Performance
- C# SaveFileDialog: Use ShowDialog and FileName
- C# Scraping HTML Links
- C# sealed Keyword
- C# Seek File Examples: ReadBytes
- C# select new Example: LINQ
- C# Select Method (Use Lambda to Modify Elements)
- C# Serialize List (Write to File With BinaryFormatter)
- C# Settings.settings in Visual Studio
- C# Shuffle Array: KeyValuePair and List
- C# Single and Double Types
- C# Single Instance Windows Form
- C# Snippet Examples
- C# Sort DateTime List
- C# Sort List With Lambda, Comparison Method
- C# Sort Number Strings
- C# Sort Examples: Arrays and Lists
- C# SortedDictionary
- C# SortedList
- C# SortedSet Examples
- C# Split String Examples
- C# String Copy() method
- C# SplitContainer: Windows Forms
- C# SqlClient Tutorial: SqlConnection, SqlCommand
- C# SqlCommand Example: SELECT TOP, ORDER BY
- C# SqlCommandBuilder Example: GetInsertCommand
- C# SqlConnection Example: Using, SqlCommand
- C# SqlDataAdapter Example
- C# SqlDataReader: GetInt32, GetString
- C# SqlParameter Example: Constructor, Add
- C# Stack Collection: Push, Pop
- C# Static List: Global List Variable
- C# Static Regex
- C# Static String
- C# static Keyword
- C# StatusStrip Example: Windows Forms
- C# String Chars (Get Char at Index)
- C# string.Concat Examples
- C# String Interpolation Examples
- C# string.Join Examples
- C# String Performance, Memory Usage Info
- C# String Property
- C# String Slice, Get Substring Between Indexes
- C# String Switch Examples
- C# String
- C# StringBuilder Append Performance
- C# StringBuilder Cache
- C# ToBase64String (Data URI Image)
- C# Struct Versus Class
- C# struct Examples
- C# Substring Examples
- C# Numeric Suffix Examples
- C# switch Examples
- C# String IsNormalized() method
- C# TabControl: Windows Forms
- TableLayoutPanel: Windows Forms
- C# Take and TakeWhile Examples
- C# Task Examples (Task.Run, ContinueWith and Wait)
- C# Ternary Operator
- C# Text Property: Windows Forms
- C# TextBox.AppendText Method
- C# TextBox Example
- C# TextChanged Event
- C# TextFieldParser Examples: Read CSV
- C# ThreadStart and ParameterizedThreadStart
- C# throw Keyword Examples
- C# Timer Examples
- C# TimeSpan Examples
- C# TrimEnd and TrimStart
- C# True and False
- C# Truncate String
- C# String ToLower() method
- C# String ToCharArray() method
- C# String ToUpperInvariant() method
- C# String Trim() method
- C# Assign Variables Optimization
- C# Array.Resize Examples
- C# Array.Sort: Keys, Values and Ranges
- C# Array.Reverse Example
- C# Array Slice, Get Elements Between Indexes
- C# Array.TrueForAll: Use Lambda to Test All Elements
- C# ArrayTypeMismatchException
- C# as: Cast Examples
- C# ASCII Table
- C# ASCII Transformation, Convert Char to Index
- C# AsEnumerable Method
- C# AsParallel Example
- ASP.NET AspLiteral
- C# BaseStream Property
- C# Console.Beep Example
- C# Benchmark
- C# BinaryReader Example (Use ReadInt32)
- C# BinaryWriter Type
- C# BitArray Examples
- C# BitConverter Examples
- C# Bitcount Examples
- C# Bool Methods, Return True and False
- C# bool Sort Examples (True to False)
- C# Caesar Cipher
- C# Cast Extension: System.Linq
- C# Cast to Int (Convert Double to Int)
- C# Cast Examples
- C# catch Examples
- C# Change Characters in String (ToCharArray, For Loop)
- C# Char Combine: Get String From Chars
- C# char.IsDigit (If Char Is Between 0 and 9)
- C# char.IsLower and IsUpper
- C# Character Literal (const char)
- C# Char Lowercase Optimization
- C# Char Test (If Char in String Equals a Value)
- C# char.ToLower and ToUpper
- C# char Examples
- C# abstract Keyword
- C# Action Object (Lambda That Returns Void)
- C# Aggregate: Use Lambda to Accumulate Value
- C# AggressiveInlining: MethodImpl Attribute
- C# Anagram Method
- C# And Bitwise Operator
- C# Anonymous Function (Delegate With No Name)
- C# Any Method, Returns True If Match Exists
- C# StringBuilder Append and AppendLine
- C# StringBuilder AppendFormat
- ASP.NET appSettings Example
- C# ArgumentException: Invalid Arguments
- C# Array.ConvertAll, Change Type of Elements
- C# Array.Copy Examples
- C# Array.CreateInstance Method
- C# Array and Dictionary Test, Integer Lookups
- C# Array.Exists Method, Search Arrays
- C# Array.Find Examples, Search Array With Lambda
- C# Array.ForEach: Use Lambda on Every Element
- C# Array Versus List Memory Usage
- C# Array Property, Return Empty Array
- C# CharEnumerator
- C# Chart, Windows Forms (Series and Points)
- C# CheckBox: Windows Forms
- C# class Examples
- C# Clear Dictionary: Remove All Keys
- C# Clone Examples: ICloneable Interface
- C# Closest Date (Find Dates Nearest in Time)
- C# Combine Arrays: List, Array.Copy and Buffer.BlockCopy
- C# Combine Dictionary Keys
- C# ComboBox: Windows Forms
- C# CompareTo Int Method
- C# Comparison Object, Used With Array.Sort
- C# Compress Data: GZIP
- C# Console.Read Method
- C# Console.ReadKey Example
- C# Console.ReadLine Example (While Loop)
- C# Console.SetOut and Console.SetIn
- C# Console.WindowHeight
- C# Console.Write, Append With No Newline
- C# Console.WriteLine (Print)
- C# const Example
- C# Constraint Puzzle Solver
- C# Count Characters in String
- C# Count, Dictionary (Get Number of Keys)
- C# Count Letter Frequencies
- C# Count Extension Method: Use Lambda to Count
- C# CSV Methods (Parse and Segment)
- C# DataRow Field Method: Cast DataTable Cell
- C# Get Day Count Elapsed From DateTime
- C# DateTime Format
- C# DateTime.Now (Current Time)
- C# DateTime.Today (Current Day With Zero Time)
- C# DateTime.TryParse and TryParseExact
- C# DateTime Examples
- C# DateTimePicker Example
- C# Debug.Write Examples
- C# Visual Studio Debugging Tutorial
- C# decimal Examples
- C# DayOfWeek
- C# Enum.Format Method (typeof Enum)
- C# Enum.GetName, GetNames: Get String Array From Enum
- C# Enum.Parse, TryParse: Convert String to Enum
- C# Error and Warning Directives
- C# ErrorProvider Control: Windows Forms
- C# event Examples
- C# Get Every Nth Element From List (Modulo)
- C# Excel Interop Example
- C# Except (Remove Elements From Collection)
- C# Extension Method
- C# extern alias Example
- C# Convert Feet, Inches
- C# File.Delete
- C# File Equals: Compare Files
- C# File.Exists Method
- C# try Keyword
- C# TryGetValue (Get Value From Dictionary)
- C# Tuple Examples
- C# Type Class: Returned by typeof, GetType
- C# TypeInitializationException
- C# Union: Combine and Remove Duplicate Elements
- C# Unreachable Code Detected
- C# Unsafe Keyword: Fixed, Pointers
- C# Uppercase First Letter
- C# Uri and UriBuilder Classes
- C# Using Alias Example
- C# using Statement: Dispose and IDisposable
- C# value Keyword
- C# ValueTuple Examples (System.ValueTuple, ToTuple)
- C# ValueType Examples
- C# Variable Initializer for Class Field
- C# Word Count
- C# Word Interop: Microsoft.Office.Interop.Word
- C# XElement Example (XElement.Load, XName)
- C# Zip Method (Use Lambda on Two Collections)
- C# File.Move Method, Rename File
- C# File.Open Examples
- C# File.ReadAllBytes, Get Byte Array From File
- C# File.ReadAllLines, Get String Array From File
- C# File.ReadAllText, Get String From File
- C# File.ReadLines, Use foreach Over Strings
- C# File.Replace Method
- C# FileInfo Length, Get File Size
- C# FileInfo Examples
- C# File Handling
- C# Filename With Date Example (DateTime.Now)
- C# FileNotFoundException (catch Example)
- C# FileStream Length, Get Byte Count From File
- C# FileStream Example, File.Create
- C# FileSystemWatcher Tutorial (Changed, e.Name)
- C# finally Keyword
- C# First Sentence
- C# FirstOrDefault (Get First Element If It Exists)
- C# Fisher Yates Shuffle: Generic Method
- C# fixed Keyword (unsafe)
- C# Flatten Array (Convert 2D to 1D)
- C# First Words in String
- C# First (Get Matching Element With Lambda)
- C# ContainsKey Method (Key Exists in Dictionary)
- C# Convert ArrayList to Array (Use ToArray)
- C# Convert ArrayList to List
- C# Convert Bool to Int
- C# Convert Bytes to Megabytes
- C# Convert Degrees Celsius to Fahrenheit
- C# Convert Dictionary to List
- C# Convert Dictionary to String (Write to File)
- C# Convert List to Array
- C# Convert List to DataTable (DataGridView)
- C# Convert List to String
- C# Convert Miles to Kilometers
- C# Convert Milliseconds, Seconds, Minutes
- C# Convert Nanoseconds, Microseconds, Milliseconds
- C# Convert String Array to String
- C# Convert TimeSpan to Long
- C# Convert Types
- C# Copy Dictionary
- C# Count Elements in Array and List
- C# FromOADate and Excel Dates
- C# Generic Class, Generic Method Examples
- C# GetEnumerator: While MoveNext, Get Current
- C# GetHashCode (String Method)
- C# Thumbnail Image With GetThumbnailImage
- C# GetType Method
- C# Global Variable Examples (Public Static Property)
- ASP.NET Global Variables Example
- C# Group By Operator: LINQ
- GroupBox: Windows Forms
- C# GroupBy Method: LINQ
- C# GroupJoin Method
- C# GZipStream Example (DeflateStream)
- C# HashSet Examples
- C# Hashtable Examples
- HelpProvider Control Use
- C# HTML and XML Handling
- C# HtmlEncode and HtmlDecode
- C# HtmlTextWriter Example
- C# HttpUtility.HtmlEncode Methods
- C# HybridDictionary
- C# default Operator
- C# DefaultIfEmpty Method
- C# Define and Undef Directives
- C# Destructor
- C# DialogResult: Windows Forms
- C# Dictionary, Read and Write Binary File
- C# Dictionary Memory
- C# Dictionary Optimization, Increase Capacity
- C# Dictionary Optimization, Test With ContainsKey
- C# DictionaryEntry Example (Hashtable)
- C# Directives
- C# Directory.CreateDirectory, Create New Folder
- C# Directory.GetFiles Example (Get List of Files)
- C# DivideByZeroException
- C# DllImport Attribute
- C# Do While Loop Example
- C# DriveInfo Examples
- C# DropDownItems Control
- C# IComparable Example, CompareTo: Sort Objects
- C# IDictionary Generic Interface
- C# IEnumerable Examples
- C# IList Generic Interface: List and Array
- C# Image Type
- C# ImageList Use: Windows Forms
- C# Increment String That Contains a Number
- C# Increment, Preincrement and Decrement Ints
- Dot Net Perls
- C# Indexer Examples (This Keyword, get and set)
- C# IndexOutOfRangeException
- C# Inheritance
- C# Insert String Examples
- C# int Array
- C# Interface Examples
- C# Interlocked Examples: Add, CompareExchange
- C# Intersect: Get Common Elements
- C# InvalidCastException
- C# InvalidOperationException: Collection Was Modified
- C# IOException Type: File, Directory Not Found
- C# IOrderedEnumerable (Query Expression With orderby)
- C# is: Cast Examples
- C# IsFixedSize, IsReadOnly and IsSynchronized Arrays
- C# string.IsNullOrEmpty, IsNullOrWhiteSpace
- C# IsSorted Method: If Array Is Already Sorted
- C# Jagged Array Examples
- C# join Examples (LINQ)
- C# KeyCode Property and KeyDown
- C# KeyNotFoundException: Key Not Present in Dictionary
- C# KeyValuePair Examples
- C# Line Count for File
- C# Line Directive
- C# LinkedList
- C# List CopyTo (Copy List Elements to Array)
- C# List Equals (If Elements Are the Same)
- C# List Find and Exists Examples
- C# List Insert Performance
- ASP.NET LiteralControl Example
- C# Locality Optimizations (Memory Hierarchy)
- C# lock Keyword
- C# Long and ulong Types
- C# Loop Over String Chars: Foreach, For
- C# Loop Over String Array
- C# Main args Examples
- C# Map Example
- ASP.NET MapPath: Virtual and Physical Paths
- C# Mask Optimization
- C# MaskedTextBox Example
- C# Math.Abs: Absolute Value
- C# Math.Ceiling Usage
- C# Math.Floor Method
- C# Math.Max and Math.Min Examples
- C# Math.Pow Method, Exponents
- C# Math.Round Examples: MidpointRounding
- C# Math Type
- C# Max and Min: Get Highest or Lowest Element
- C# MemoryFailPoint and InsufficientMemoryException
- C# MemoryStream: Use Stream on Byte Array
- C# MenuStrip: Windows Forms
- C# Modulo Operator: Get Remainder From Division
- C# MonthCalendar Control: Windows Forms
- C# Multiple Return Values
- C# Multiply Numbers
- C# namespace Keyword
- C# NameValueCollection Usage
- C# Nested Lists: Create 2D List or Jagged List
- C# Nested Switch Statement
- C# Environment.NewLine
- C# Normalize, IsNormalized Methods
- C# Null String Example
- C# null Keyword
- C# Nullable Examples
- C# NullReferenceException and Null Parameter
- C# Object Array
- C# Obsolete Attribute
- C# OfType Examples
- C# OpenFileDialog Example
- C# operator Keyword
- C# Odd and Even Numbers
- C# Bitwise Or
- C# orderby Query Keyword
- C# out Parameter
- C# OutOfMemoryException
- C# OverflowException
- C# Overload Method
- C# Override Method
- C# PadRight and PadLeft: String Columns
- C# Get Paragraph From HTML With Regex
- C# Parallel.For Example (Benchmark)
- C# Parallel.Invoke: Run Methods on Separate Threads
- C# Parameter Optimization
- C# Parameter Passing, ref and out
- C# params Keyword
- C# int.Parse: Convert Strings to Integers
- C# partial Keyword
- C# Path.ChangeExtension
- C# Path Exists Example
- C# Path.GetExtension: File Extension
- C# Path.GetRandomFileName Method
- C# Pragma Directive
- C# Predicate (Lambda That Returns True or False)
- C# Pretty Date Format (Hours or Minutes Ago)
- C# PreviewKeyDown Event
- C# Random Lowercase Letter
- C# Random Paragraphs and Sentences
- C# Random String
- C# Random Number Examples
- C# StreamReader ReadLine, ReadLineAsync Examples
- C# readonly Keyword
- C# Recursion Example
- C# Regex, Read and Match File Lines
- C# Regex Groups, Named Group Example
- C# Regex.Matches Quote Example
- C# Regex.Matches Method: foreach Match, Capture
- C# Regex.Replace, Matching End of String
- C# Regex.Replace, Remove Numbers From String
- C# Regex.Replace, Merge Multiple Spaces
- C# Regex.Replace Examples: MatchEvaluator
- C# Regex.Split, Get Numbers From String
- C# Regex.Split Examples
- C# Regex Trim, Remove Start and End Spaces
- C# RegexOptions.Compiled
- C# RegexOptions.IgnoreCase Example
- C# RegexOptions.Multiline
- C# Region and endregion
- C# Remove Char From String at Index
- C# Remove Element
- C# Remove HTML Tags
- C# Remove String
- C# Reserved Filenames
- C# return Keyword
- C# Reverse String
- C# Reverse Words
- C# Reverse Extension Method
- C# RichTextBox Example
- C# Right String Part
- C# RNGCryptoServiceProvider Example
- C# ROT13 Method, Char Lookup Table
- C# SelectMany Example: LINQ
- C# Sentinel Optimization
- C# SequenceEqual Method (If Two Arrays Are Equal)
- C# Shift Operators (Bitwise)
- C# Short and ushort Types
- C# Single Method: Get Element If Only One Matches
- C# SingleOrDefault
- C# Singleton Pattern Versus Static Class
- C# Singleton Class
- C# sizeof Keyword
- C# Skip and SkipWhile Examples
- C# Sleep Method (Pause)
- C# Sort Dictionary: Keys and Values
- C# Sort by File Size
- C# Sort, Ignore Leading Chars
- C# Sort KeyValuePair List: CompareTo
- C# Sort Strings by Length
- C# Thread.SpinWait Example
- C# Math.Sqrt Method
- C# stackalloc Operator
- C# StackOverflowException
- C# StartsWith and EndsWith String Methods
- C# Static Array
- C# Static Dictionary
- C# Stopwatch Examples
- C# Stream
- C# StreamReader ReadToEnd Example (Read Entire File)
- C# StreamReader ReadToEndAsync Example (Performance)
- C# StreamReader Examples
- C# StreamWriter Examples
- C# String Append (Add Strings)
- C# String Compare and CompareTo Methods
- C# String Constructor (new string)
- C# string.Copy Method
- C# CopyTo String Method: Put Chars in Array
- C# Empty String Examples
- C# String Equals Examples
- C# String For Loop, Count Spaces in String
- C# string.Intern and IsInterned
- C# String IsUpper, IsLower
- C# String Length Property: Get Character Count
- C# String Literal: Newline and Quote Examples
- C# StringBuilder Capacity
- C# StringBuilder Clear (Set Length to Zero)
- C# StringBuilder Data Types
- C# StringBuilder Performance
- C# StringBuilder Equals (If Chars Are Equal)
- C# StringBuilder Memory
- C# StringBuilder ToString: Get String From StringBuilder
- C# StringWriter Class
- C# Sum Method: Add up All Numbers
- C# Switch Char, Test Chars With Cases
- C# Switch Enum
- C# System (using System namespace)
- C# Tag Property: Windows Forms
- C# TextInfo Examples
- C# TextReader, Returned by File.OpenText
- C# TextWriter, Returned by File.CreateText
- C# this Keyword
- C# ThreadPool
- C# Thread Join Method (Join Array of Threads)
- C# ThreadPool.SetMinThreads Method
- C# TimeZone Examples
- C# Get Title From HTML With Regex
- C# ToArray Extension Method
- C# ToCharArray: Convert String to Array
- C# ToDictionary Method
- C# Token
- C# ToList Extension Method
- C# ToLookup Method (Get ILookup)
- C# ToLower and ToUpper: Uppercase and Lowercase Strings
- ToolStripContainer Control: Dock, Properties
- C# ToolTip: Windows Forms
- C# ToString Integer Optimization
- C# ToString: Get String From Object
- C# ToTitleCase Method
- C# TrackBar: Windows Forms
- C# Tree and Nodes Example: Directed Acyclic Word Graph
- C# TreeView Tutorial
- C# Trim Strings
- C# Thread Methods
- C# History
- C# Features
- C# Variables
- C# Data Types
- C# Operators
- C# Keywords
- C# New Features | C# Version Features
- C# Programs
- C# Program to swap numbers without third variable
- C# Program to convert Decimal to Binary
- C# Program to Convert Number in Characters
- C# Program to Print Alphabet Triangle
- C# Program to print Number Triangle
- C# Program to generate Fibonacci Triangle
- C# String Compare() method
- C# String CompareOrdinal() method
- C# String CompareTo() method
- C# String Concat() method
- C# String Contains() method
- C# String CopyTo() method
- C# String EndsWith() method
- C# String Equals() method
- C# String Format() method
- C# String IndexOf() method
- C# String Insert() method
- C# String Intern(String str) method
- C# String IsInterned() method
- C# String Normalize() method
- C# String IsNullOrEmpty() method
- C# String IsNullOrWhiteSpace() method
- C# String Join() method
- C# String LastIndexOf() method
- C# String LastIndexOfAny() method
- C# String PadLeft() method
- C# String PadRight() method
- C# Nullable
- C# String Remove() method
- C# String Replace() method
- C# String Split() method
- C# String StartsWith() method
- C# String SubString() method
- C# Partial Types
- C# Iterators
- C# Delegate Covariance
- C# Delegate Inference
- C# Anonymous Types
- C# Extension Methods
- C# Query Expression
- C# Partial Method
- C# Implicitly Typed Local Variable
- C# Object and Collection Initializer
- C# Auto Implemented Properties
- C# Dynamic Binding
- C# Named and Optional Arguments
- C# Asynchronous Methods
- C# Caller Info Attributes
- C# Using Static Directive
- C# Exception Filters
- C# Await in Catch Finally Blocks
- C# Default Values for Getter Only Properties
- C# Expression Bodied Members
- C# Null Propagator
- C# String Interpolation
- C# nameof operator
- C# Dictionary Initializer
- C# Pattern Matching
- C# Tuples
- C# Deconstruction
- C# Local Functions
- C# Binary Literals
- C# Ref Returns and Locals
- C# Expression Bodied Constructors and Finalizers
- C# Expression Bodied Getters and Setters
- C# Async Main
- C# Default Expression
Related Links
Adjectives
Ado
Ai
Android
Angular
Antonyms
Apache
Articles
Asp
Autocad
Automata
Aws
Azure
Basic
Binary
Bitcoin
Blockchain
C
Cassandra
Change
Coa
Computer
Control
Cpp
Create
Creating
C-Sharp
Cyber
Daa
Data
Dbms
Deletion
Devops
Difference
Discrete
Es6
Ethical
Examples
Features
Firebase
Flutter
Fs
Git
Go
Hbase
History
Hive
Hiveql
How
Html
Idioms
Insertion
Installing
Ios
Java
Joomla
Js
Kafka
Kali
Laravel
Logical
Machine
Matlab
Matrix
Mongodb
Mysql
One
Opencv
Oracle
Ordering
Os
Pandas
Php
Pig
Pl
Postgresql
Powershell
Prepositions
Program
Python
React
Ruby
Scala
Selecting
Selenium
Sentence
Seo
Sharepoint
Software
Spellings
Spotting
Spring
Sql
Sqlite
Sqoop
Svn
Swift
Synonyms
Talend
Testng
Types
Uml
Unity
Vbnet
Verbal
Webdriver
What
Wpf