<< Back to C-SHARP
C# StringBuilder Examples
Improve string append performance with StringBuilder. Save allocations when building strings.StringBuilder. Like the pyramids, a string can be built one piece at a time. For strings, each append causes a string copy. With StringBuilder we eliminate this copy.
Unlike a string, a StringBuilder can be changed. With it, an algorithm that modifies characters in a loop runs fast. Many string copies are avoided.
An intro. This program uses StringBuilder to build up a buffer of characters. We call Append() to add more data to our StringBuilder.
Append: This method can be called directly on its own result, in the same statement.
Because: Append(), and other methods like AppendFormat, return the same StringBuilder.
C# program that uses StringBuilder
using System;
using System.Text;
class Program
{
static void Main()
{
StringBuilder builder = new StringBuilder();
// Append to StringBuilder.
for (int i = 0; i < 10; i++)
{
builder.Append(i).Append(" ");
}
Console.WriteLine(builder);
}
}
Output
0 1 2 3 4 5 6 7 8 9
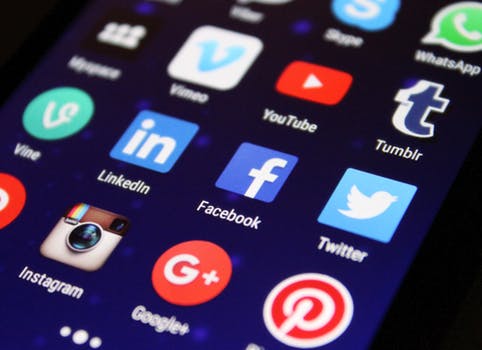
Methods. Next, we look at other essential methods on StringBuilder. The methods shown here allow us to use it effectively in many programs. We append strings and lines.
Note: This example shows no loop, and is not ideal as a program. It is for demonstration purposes.
Append: Adds the contents of its argument to the buffer in the StringBuilder. Arguments are converted to strings with ToString.
AppendAppendLine: This does the same thing as Append, except with a newline on the end. We use a terse syntax form.
ToString: This returns the buffer. We will almost always want ToString—it will return the contents as a string.
C# program that uses StringBuilder methods
using System;
using System.Text;
using System.Diagnostics;
class Program
{
static void Main()
{
// Declare a new StringBuilder.
StringBuilder builder = new StringBuilder();
builder.Append("The list starts here:");
builder.AppendLine();
builder.Append("1 cat").AppendLine();
// Get a reference to the StringBuilder's buffer content.
string innerString = builder.ToString();
// Display with Debug.
Debug.WriteLine(innerString);
}
}
Output
The list starts here:
1 cat
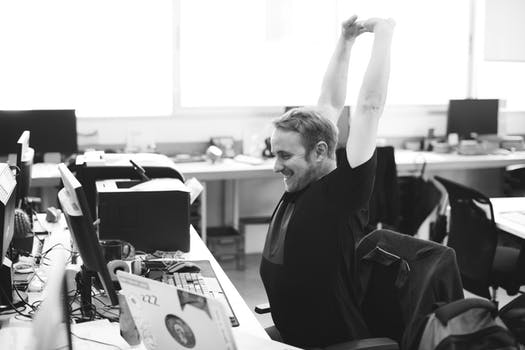
Replace. This method replaces all instances of one string with another. A string is required, but we do not need to use a string literal. The example exchanges "an" with "the."
Caution: The Replace method will replace all instances of the specified value. To replace one instance, we will need a custom method.
C# program that uses Replace
using System;
using System.Text;
class Program
{
static void Main()
{
StringBuilder builder = new StringBuilder(
"This is an example string that is an example.");
builder.Replace("an", "the"); // Replaces 'an' with 'the'.
Console.WriteLine(builder.ToString());
Console.ReadLine();
}
}
Output
This is the example string that is the example.
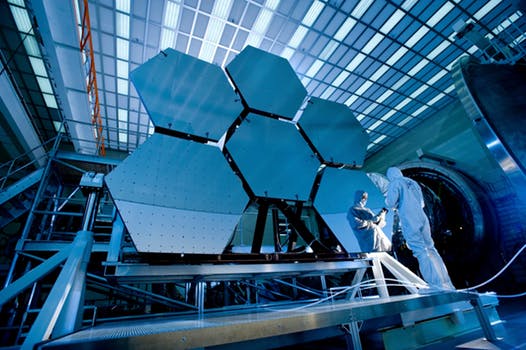
AppendFormat. With this method, we add text to a StringBuilder based on a pattern. We can use substitution markers to fill fields in this pattern.
AppendFormatTip: Many versions of AppendFormat in the .NET Framework (such as Console.WriteLine) are implemented with StringBuilder.
ConsoleHowever: It is usually faster to call Append repeatedly with all the required parts. But the syntax of AppendFormat may be clearer.
string.FormatC# program that uses AppendFormat
using System;
using System.Text;
class Program
{
static void Main()
{
var builder = new StringBuilder();
// Append a format string directly.
builder.AppendFormat("Hello {0}. It is {1}.",
"Ankit",
"Thursday");
Console.WriteLine(builder);
}
}
Output
Hello Ankit. It is Thursday.
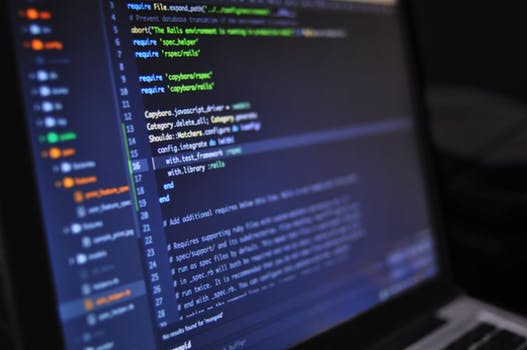
Loops. Often we use StringBuilders in loops. If many appends are needed, sometimes StringBuilder is helpful in other contexts. Here is an example of StringBuilder in a foreach-loop.
ForeachNote: Many looping constructs, including for, while and foreach, are effective when used with StringBuilder.
C# program that uses foreach
using System;
using System.Text;
class Program
{
static void Main()
{
string[] items = { "Cat", "Dog", "Celebrity" };
StringBuilder builder2 = new StringBuilder(
"These items are required:").AppendLine();
foreach (string item in items)
{
builder2.Append(item).AppendLine();
}
Console.WriteLine(builder2.ToString());
Console.ReadLine();
}
}
Output
These items are required:
Cat
Dog
Celebrity
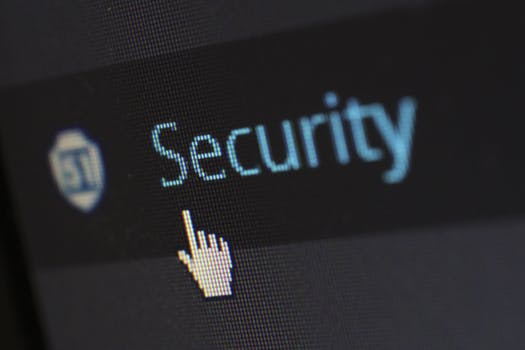
Argument. StringBuilder can be passed as an argument. This is a nice optimization. It avoids converting back and forth to strings.
Tip: Eliminating allocations of strings (and StringBuilders) is an effective way to improve program performance.
Caution: Usually it is best to use descriptive names, not "A1" or "b." Not all examples can be perfect.
C# program that uses StringBuilder argument
using System;
using System.Text;
class Program
{
static string[] _items = new string[]
{
"cat",
"dog",
"giraffe"
};
/// <summary>
/// Append to the StringBuilder param, void method.
/// </summary>
static void A2(StringBuilder b)
{
foreach (string item in _items)
{
b.AppendLine(item);
}
}
static void Main()
{
StringBuilder b = new StringBuilder();
A2(b);
}
}
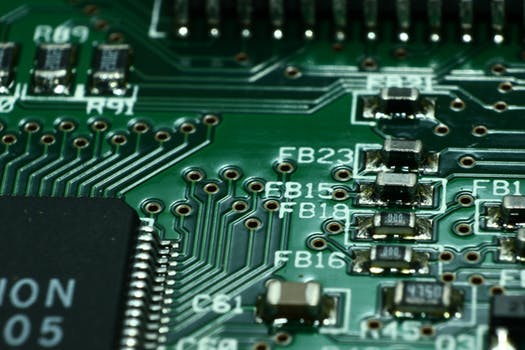
Indexer. It is possible to use the indexer to access or change certain characters. This syntax is the same as the syntax for accessing characters in a string instance.
Next: The example tests and changes characters in a StringBuilder. It uses the indexer.
IndexerC# program that uses indexer
using System;
using System.Text;
class Program
{
static void Main()
{
StringBuilder builder = new StringBuilder();
builder.Append("cat");
// Write second letter.
Console.WriteLine(builder[1]);
// Change first letter.
builder[0] = 'r';
Console.WriteLine(builder.ToString());
}
}
Output
a
rat
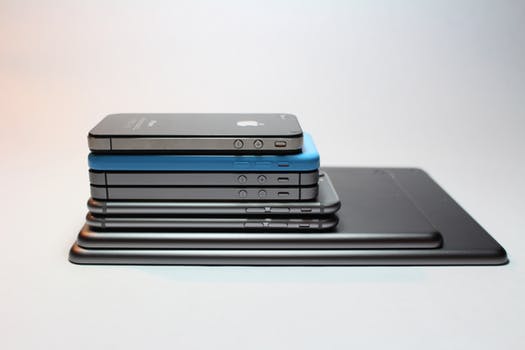
Remove. This method removes a range of characters by index from the internal buffer. As with other StringBuilder methods, this just rearranges the internal buffer.
Here: We remove characters starting at index 4. We remove three characters past that index.
C# program that uses Remove
using System;
using System.Text;
class Program
{
static void Main()
{
StringBuilder builder = new StringBuilder("The Dev Codes");
builder.Remove(4, 3);
Console.WriteLine(builder);
}
}
Output
Dot Perls
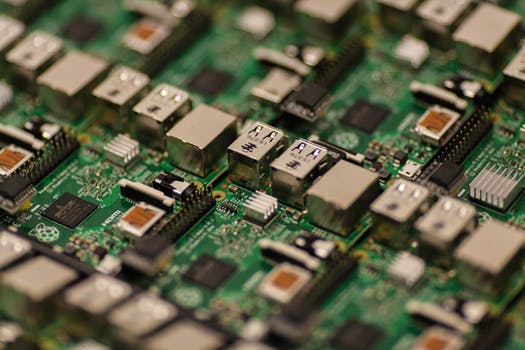
Append substring. We can append a substring directly from another string. No Substring call is needed. We use the Append method to do this.
IndexOf: We try to find the index of the space in the string. The char after the space is the start of the substring we want.
IndexOfAppend: We pass the string, the start index, and then the computed length (which continues until the end of the string).
Tip: Appending a substring directly is faster than calling Substring() to create an intermediate string first.
C# program that append Substring directly
using System;
using System.Text;
class Program
{
static void Main()
{
var builder = new StringBuilder();
string value = "bird frog";
// Get the index of the char after the space.
int afterSpace = value.IndexOf(' ') + 1;
// Append a substring, computing the length of the target range.
builder.Append(value, afterSpace, value.Length - afterSpace);
Console.WriteLine("APPEND SUBSTRING: {0}", builder);
}
}
Output
APPEND SUBSTRING: frog
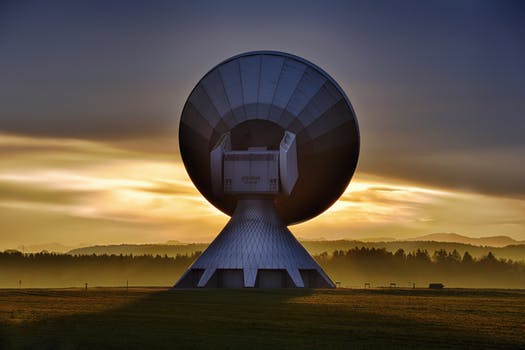
ToString. This method is optimized. It will not copy data in certain situations. These optimizations are hard to duplicate in custom code.
No arguments: We can call ToString with no arguments. This converts the entire StringBuilder into a string.
Range: We can pass a start index, and a length, to the ToString method. The second argument is the count of chars, not the end index.
Info: The ToString method has some advanced optimizations to reduce copying. It should be used when a string is required.
ToStringC# program that uses ToString
using System;
using System.Text;
class Program
{
static void Main()
{
var builder = new StringBuilder("abcdef");
// Use ToString with no arguments.
string result = builder.ToString();
Console.WriteLine("TOSTRING: {0}", result);
// Use a start and length.
string resultRange = builder.ToString(3, 3);
Console.WriteLine("TOSTRING RANGE: {0}", resultRange);
}
}
Output
TOSTRING: abcdef
TOSTRING RANGE: def
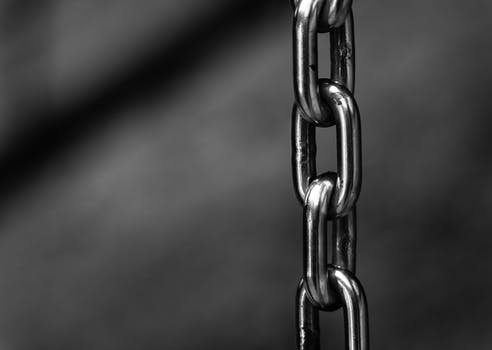
Trim. StringBuilder has no Trim, TrimStart or TrimEnd methods. But we can implement similar methods. Here we add a TrimEnd method that removes a final character.
TrimEnd: This custom method tests the last character of a StringBuilder for a matching char. It then reduces Length by 1 to erase it.
Caution: There are issues here. Only one char will be removed—we could use a while-loop to remove multiple matching chars.
C# program that implements Trim on StringBuilder
using System;
using System.Text;
class Program
{
static void TrimEnd(StringBuilder builder, char letter)
{
// ... If last char matches argument, reduce length by 1.
if (builder[builder.Length - 1] == letter)
{
builder.Length -= 1;
}
}
static void Main()
{
StringBuilder builder = new StringBuilder();
builder.Append("This has an end period.");
Console.WriteLine(builder);
TrimEnd(builder, '.');
Console.WriteLine(builder);
}
}
Output
This has an end period.
This has an end period
Benchmark, constructor. Often we need to append a string to a StringBuilder right after allocating it. When we specify that string in the constructor, performance improves.
Tip: This optimization can be used with a capacity. Specify the initial string value along with the capacity.
Version 1: This code creates a StringBuilder with capacity of 10 chars, and then appends the string "Cat" to it.
Version 2: This code creates a 10-char StringBuilder with the value "Cat" in the constructor.
Result: It is faster to initialize a StringBuilder with a string if we need to append something immediately to the StringBuilder.
C# program that benchmarks StringBuilder constructor
using System;
using System.Diagnostics;
using System.Text;
class Program
{
const int _max = 1000000;
static void Main()
{
// Version 1: create StringBuilder then Append a string.
var s1 = Stopwatch.StartNew();
for (int i = 0; i < _max; i++)
{
var builder = new StringBuilder(10);
builder.Append("Cat");
if (builder.Length == 0)
{
return;
}
}
s1.Stop();
// Version 2: create a StringBuilder with a string argument.
var s2 = Stopwatch.StartNew();
for (int i = 0; i < _max; i++)
{
var builder = new StringBuilder("Cat", 10);
if (builder.Length == 0)
{
return;
}
}
s2.Stop();
Console.WriteLine(((double)(s1.Elapsed.TotalMilliseconds * 1000000) /
_max).ToString("0.00 ns"));
Console.WriteLine(((double)(s2.Elapsed.TotalMilliseconds * 1000000) /
_max).ToString("0.00 ns"));
}
}
Output
23.73 ns new StringBuilder, Append
19.42 ns new StringBuilder(string)
Benchmark, string concat. Sometimes we make a StringBuilder mistake that reduces speed. We use the plus operator on strings within a StringBuilder. This is bad.
Version 1: This concats 2 strings and then passes that string to the StringBuilder Append() method.
Version 2: This version appends each string individually, avoiding a temporary string creation.
Result: It is much faster to call Append() individually and never to create any temporary strings with the plus operator.
C# program that improves StringBuilder performance
using System;
using System.Diagnostics;
using System.Text;
class Program
{
const int _max = 1000000;
static void Main()
{
var builder1 = new StringBuilder();
var builder2 = new StringBuilder();
var tempString = 100.ToString();
// Version 1: concat strings then Append.
var s1 = Stopwatch.StartNew();
for (int i = 0; i < _max; i++)
{
builder1.Append(tempString + ",");
}
s1.Stop();
// Version 2: append individual strings.
var s2 = Stopwatch.StartNew();
for (int i = 0; i < _max; i++)
{
builder2.Append(tempString).Append(",");
}
s2.Stop();
Console.WriteLine(((double)(s1.Elapsed.TotalMilliseconds * 1000000) /
_max).ToString("0.00 ns"));
Console.WriteLine(((double)(s2.Elapsed.TotalMilliseconds * 1000000) /
_max).ToString("0.00 ns"));
}
}
Output
38.92 ns Concat strings, then Append
15.42 ns Append twice
Benchmark, append substring. With the appropriate StringBuilder Append overload, we append a part of another string. This eliminates extra string copies.
Version 1: Here we take a substring of the input string and then append that to the StringBuilder.
Version 2: We call Append with 3 arguments. This is equivalent to the Substring call but much faster.
Result: The StringBuilder Append version that avoids a separate Substring call is faster.
SubstringOverloadC# program that uses StringBuilder overload
using System;
using System.Diagnostics;
using System.Text;
class Program
{
static void Method1(string input, StringBuilder buffer)
{
buffer.Clear();
string temp = input.Substring(3, 2);
buffer.Append(temp);
}
static void Method2(string input, StringBuilder buffer)
{
buffer.Clear();
buffer.Append(input, 3, 2);
}
static void Main()
{
const int m = 100000000;
var builder = new StringBuilder();
var s1 = Stopwatch.StartNew();
// Version 1: take Substring.
for (int i = 0; i < m; i++)
{
Method1("Codex", builder);
}
s1.Stop();
var s2 = Stopwatch.StartNew();
// Version 2: append range with Append.
for (int i = 0; i < m; i++)
{
Method2("Codex", builder);
}
s2.Stop();
Console.WriteLine(((double)(s1.Elapsed.TotalMilliseconds * 1000000) /
m).ToString("0.00 ns"));
Console.WriteLine(((double)(s2.Elapsed.TotalMilliseconds * 1000000) /
m).ToString("0.00 ns"));
Console.Read();
}
}
Output
33.47 ns Append string
25.14 ns Append range of string
Equals. This method compares the contents of 2 StringBuilders. It avoids lots of error-prone code that might otherwise be needed. It returns true or false.
EqualsCaution: The Equals method will return false if the capacities of the objects are different, even if their data is identical.
Clear. To clear a StringBuilder, it is sometimes best to allocate a new one. Other times, we can assign the Length property to zero or use the Clear method.
Clear
Exceptions. We get an ArgumentOutOfRangeException if we put too much data in a StringBuilder. The maximum number of characters is equal to Int32.MaxValue.
ArgumentExceptionNote: The Int32.MaxValue constant is equal to 2,147,483,647. This is the max length of a StringBuilder.
int.MaxValueImmutable. This term indicates that an object's data is not changeable. To see an example of an immutable object, try to assign to a character in a string. This causes a compile-time error.
And: This happens because the string type does not define a set accessor. It cannot be modified once changed.
Arrays: Character arrays can be changed. Internally StringBuilder uses mutable char arrays for its buffer.
Memory. In garbage collection, there is memory pressure. As more temporary objects are created, GC runs more often. StringBuilder creates fewer temporary objects than string appends.
StringBuilder MemoryPerformance, char arrays. To use char arrays, code must be more precise. We must know a maximum or absolute size of the output string. This can enhance performance.
Char ArrayA review. StringBuilder is mainly a performance optimization. Here we test the performance and memory usage of it. In reviewing, we learn when it is superior to strings.
StringBuilder: optimizationSome comments. These secrets are widely known. But this makes them no less effective. StringBuilder can improve the performance of a program—even if we misuse it.
Related Links:
- C# Array Examples, String Arrays
- C# ArrayList Examples
- C# ArraySegment: Get Array Range, Offset and Count
- C# break Statement
- C# Buffer BlockCopy Example
- C# BufferedStream: Optimize Read and Write
- 404 Not Found
- C# 24 Hour Time Formats
- C# 2D Array Examples
- 7 Zip Command Line Examples
- C# 7 Zip Executable (Process.Start)
- C# All Method: All Elements Match a Condition
- C# Alphabetize String
- C# Alphanumeric Sorting
- C# Arithmetic Expression Optimization
- C# Array.AsReadOnly Method (ObjectModel)
- C# Array.BinarySearch Method
- C# Array.Clear Examples
- C# Array.IndexOf, LastIndexOf: Search Arrays
- C# async, await Examples
- C# Attribute Examples
- C# Average Method
- C# BackgroundWorker
- C# base Keyword
- C# String Between, Before, After
- C# Binary Representation int (Convert, toBase 2)
- C# BinarySearch List
- C# bool Array (Memory Usage, One Byte Per Element)
- C# bool.Parse, TryParse: Convert String to Bool
- C# bool Type
- C# Array Length Property, Get Size of Array
- C# Button Example
- C# Byte Array: Memory Usage, Read All Bytes
- C# Byte and sbyte Types
- C# Capacity for List, Dictionary
- C# Case Insensitive Dictionary
- C# case Example (Switch Case)
- C# Char Array
- C# Checked and Unchecked Keywords
- C# CheckedListBox: Windows Forms
- C# Color Table
- C# Color Examples: FromKnownColor, FromName
- C# ColorDialog Example
- C# Comment: Single Line and Multiline
- C# Concat Extension: Combine Lists and Arrays
- C# Conditional Attribute
- C# Console Color, Text and BackgroundColor
- C# String Clone() method
- C# Constructor Examples
- C# Contains Extension Method
- C# String GetTypeCode() method
- C# String ToLowerInvariant() method
- C# Customized Dialog Box
- C# DataColumn Example: Columns.Add
- C# DataGridView Add Rows
- DataGridView Columns, Edit Columns Dialog
- C# DataGridView Row Colors (Alternating)
- C# DataGridView Tutorial
- C# DataGridView
- C# DataRow Examples
- C# DataSet Examples
- C# DataSource Example
- C# DataTable Compare Rows
- C# DataTable foreach Loop
- C# DataTable RowChanged Example: AcceptChanges
- C# DataTable Select Example
- C# DataTable Examples
- C# DataView Examples
- C# String ToString() method
- C# String ToUpper() method
- C# Digit Separator
- C# DateTime.MinValue (Null DateTime)
- C# DateTime.Month Property
- C# DateTime.Parse: Convert String to DateTime
- C# DateTime Subtract Method
- C# Decompress GZIP
- C# Remove Duplicates From List
- C# dynamic Keyword
- C# ElementAt, ElementAtOrDefault Use
- C# Encapsulate Field
- C# Enum Array Example, Use Enum as Array Index
- C# enum Flags Attribute Examples
- C# Enum ToString: Convert Enum to String
- C# enum Examples
- C# Enumerable.Range, Repeat and Empty
- C# Environment Type
- C# EventLog Example
- C# Exception Handling
- C# explicit and implicit Keywords
- C# Factory Design Pattern
- C# File.Copy Examples
- C# typeof and nameof Operators
- C# String TrimEnd() method
- C# var Examples
- C# virtual Keyword
- C# void Method, Return No Value
- C# volatile Example
- C# WebBrowser Control (Navigate Method)
- C# WebClient: DownloadData, Headers
- C# Where Method and Keyword
- C# String TrimStart() method
- C# delegate Keyword
- C# descending, ascending Keywords
- C# while Loop Examples
- C# Whitespace Methods: Convert UNIX, Windows Newlines
- C# XmlReader, Parse XML File
- C# XmlTextReader
- C# XmlTextWriter
- C# XmlWriter, Create XML File
- C# XOR Operator (Bitwise)
- C# yield Example
- C# float Numbers
- FlowLayoutPanel Control
- C# Focused Property
- C# FolderBrowserDialog Control
- C# Font Type: FontFamily and FontStyle
- C# FontDialog Example
- C# for Loop Examples
- C# foreach Loop Examples
- ForeColor, BackColor: Windows Forms
- C# Form: Event Handlers
- C# Contains String Method
- C# ContainsValue Method (Value Exists in Dictionary)
- C# ContextMenuStrip Example
- C# continue Keyword
- C# Control: Windows Forms
- C# Windows Forms Controls
- C# Convert Char Array to String
- C# Convert Char to String
- C# Convert Days to Months
- C# Convert String to Byte Array
- C# String Format
- C# Func Object (Lambda That Returns a Value)
- C# GC.Collect Examples: CollectionCount, GetTotalMemory
- C# Path.GetDirectoryName (Remove File From Path)
- C# goto Examples
- C# HttpClient Example: System.Net.Http
- ASP.NET HttpContext Request Property
- IL Disassembler Tutorial
- C# Intermediate Language (IL)
- C# IndexOf Examples
- C# IndexOfAny Examples
- C# Initialize Array
- C# Initialize List
- C# InitializeComponent Method: Windows Forms
- C# Inline Optimization
- C# Dictionary Equals: If Contents Are the Same
- C# Dictionary Versus List Loop
- C# Dictionary Order, Use Keys Added Last
- C# Dictionary Size and Performance
- C# Dictionary Versus List Lookup Time
- C# Dictionary Examples
- C# Get Directory Size (Total Bytes in All Files)
- C# Directory Type
- C# Distinct Method, Get Unique Elements Only
- C# Divide by Powers of Two (Bitwise Shift)
- C# Divide Numbers (Cast Ints)
- C# DomainUpDown Control Example
- C# Double Type: double.MaxValue, double.Parse
- C# Remove Duplicate Chars
- C# IEqualityComparer
- C# If Preprocessing Directive: Elif and Endif
- C# If Versus Switch Performance
- C# if Statement
- C# int.MaxValue, MinValue (Get Lowest Number)
- C# Program to reverse number
- C# Int and uint Types
- C# Integer Append Optimization
- C# Keywords
- C# Label Example: Windows Forms
- C# Lambda Expressions
- C# LastIndexOf Examples
- C# Last, LastOrDefault Methods
- C# Mutex Example (OpenExisting)
- C# Named Parameters
- C# Let Keyword (Use Variable in Query Expression)
- C# Levenshtein Distance
- C# LinkLabel Example: Windows Forms
- C# LINQ
- C# List Add Method, Append Element to List
- C# List AddRange, InsertRange (Append Array to List)
- C# List Clear Example
- C# List Contains Method
- C# List Remove Examples
- C# List Examples
- C# ListBox Tutorial (DataSource, SelectedIndex)
- C# ListView Tutorial: Windows Forms
- C# Maze Pathfinding Algorithm
- C# Memoization
- C# Memory Usage for Arrays of Objects
- C# MessageBox.Show Examples
- C# Method Call Depth Performance
- C# Method Parameter Performance
- C# Method Size Optimization
- C# Multidimensional Array
- C# MultiMap Class (Dictionary of Lists)
- C# Optimization
- C# new Keyword
- C# NotifyIcon: Windows Forms
- C# NotImplementedException
- C# Null Array
- C# String GetType() method
- C# Null Coalescing and Null Conditional Operators
- C# Null List (NullReferenceException)
- C# Numeric Casts
- C# NumericUpDown Control: Windows Forms
- C# object.ReferenceEquals Method
- C# Object Examples
- C# Optional Parameters
- C# Prime Number
- C# OrderBy, OrderByDescending Examples
- C# Process Examples (Process.Start)
- Panel, Windows Forms (Create Group of Controls)
- C# Path Examples
- C# Get Percentage From Number With Format String
- ASP.NET PhysicalApplicationPath
- C# PictureBox: Windows Forms
- C# PNG Optimization
- C# Position Windows: Use WorkingArea and Location
- Visual Studio Post Build, Pre Build Macros
- C# ProfileOptimization
- C# ProgressBar Example
- C# Property Examples
- C# PropertyGrid: Windows Forms
- C# Protected and internal Keywords
- C# Public and private Methods
- C# Remove Punctuation From String
- C# Query Windows Forms (Controls.OfType)
- C# Queryable: IQueryable Interface and AsQueryable
- ASP.NET QueryString Examples
- C# Queue Collection: Enqueue
- C# RadioButton Use: Windows Forms
- C# ReadOnlyCollection Use (ObjectModel)
- C# Recursion Optimization
- C# Recursive File List: GetFiles With AllDirectories
- C# ref Keyword
- C# Reflection Examples
- C# Regex.Escape and Unescape Methods
- C# StringBuilder Examples
- C# StringComparison and StringComparer
- C# StringReader Class (Get Parts of Strings)
- C# String GetEnumerator() method
- C# String GetHashCode() method
- C# Regex Versus Loop: Use For Loop Instead of Regex
- C# Regex.Match Examples: Regular Expressions
- C# RemoveAll: Use Lambda to Delete From List
- C# Replace String Examples
- ASP.NET Response.BinaryWrite
- ASP.NET Response.Write
- C# Return Optimization: out Performance
- C# SaveFileDialog: Use ShowDialog and FileName
- C# Scraping HTML Links
- C# sealed Keyword
- C# Seek File Examples: ReadBytes
- C# select new Example: LINQ
- C# Select Method (Use Lambda to Modify Elements)
- C# Serialize List (Write to File With BinaryFormatter)
- C# Settings.settings in Visual Studio
- C# Shuffle Array: KeyValuePair and List
- C# Single and Double Types
- C# Single Instance Windows Form
- C# Snippet Examples
- C# Sort DateTime List
- C# Sort List With Lambda, Comparison Method
- C# Sort Number Strings
- C# Sort Examples: Arrays and Lists
- C# SortedDictionary
- C# SortedList
- C# SortedSet Examples
- C# Split String Examples
- C# String Copy() method
- C# SplitContainer: Windows Forms
- C# SqlClient Tutorial: SqlConnection, SqlCommand
- C# SqlCommand Example: SELECT TOP, ORDER BY
- C# SqlCommandBuilder Example: GetInsertCommand
- C# SqlConnection Example: Using, SqlCommand
- C# SqlDataAdapter Example
- C# SqlDataReader: GetInt32, GetString
- C# SqlParameter Example: Constructor, Add
- C# Stack Collection: Push, Pop
- C# Static List: Global List Variable
- C# Static Regex
- C# Static String
- C# static Keyword
- C# StatusStrip Example: Windows Forms
- C# String Chars (Get Char at Index)
- C# string.Concat Examples
- C# String Interpolation Examples
- C# string.Join Examples
- C# String Performance, Memory Usage Info
- C# String Property
- C# String Slice, Get Substring Between Indexes
- C# String Switch Examples
- C# String
- C# StringBuilder Append Performance
- C# StringBuilder Cache
- C# ToBase64String (Data URI Image)
- C# Struct Versus Class
- C# struct Examples
- C# Substring Examples
- C# Numeric Suffix Examples
- C# switch Examples
- C# String IsNormalized() method
- C# TabControl: Windows Forms
- TableLayoutPanel: Windows Forms
- C# Take and TakeWhile Examples
- C# Task Examples (Task.Run, ContinueWith and Wait)
- C# Ternary Operator
- C# Text Property: Windows Forms
- C# TextBox.AppendText Method
- C# TextBox Example
- C# TextChanged Event
- C# TextFieldParser Examples: Read CSV
- C# ThreadStart and ParameterizedThreadStart
- C# throw Keyword Examples
- C# Timer Examples
- C# TimeSpan Examples
- C# TrimEnd and TrimStart
- C# True and False
- C# Truncate String
- C# String ToLower() method
- C# String ToCharArray() method
- C# String ToUpperInvariant() method
- C# String Trim() method
- C# Assign Variables Optimization
- C# Array.Resize Examples
- C# Array.Sort: Keys, Values and Ranges
- C# Array.Reverse Example
- C# Array Slice, Get Elements Between Indexes
- C# Array.TrueForAll: Use Lambda to Test All Elements
- C# ArrayTypeMismatchException
- C# as: Cast Examples
- C# ASCII Table
- C# ASCII Transformation, Convert Char to Index
- C# AsEnumerable Method
- C# AsParallel Example
- ASP.NET AspLiteral
- C# BaseStream Property
- C# Console.Beep Example
- C# Benchmark
- C# BinaryReader Example (Use ReadInt32)
- C# BinaryWriter Type
- C# BitArray Examples
- C# BitConverter Examples
- C# Bitcount Examples
- C# Bool Methods, Return True and False
- C# bool Sort Examples (True to False)
- C# Caesar Cipher
- C# Cast Extension: System.Linq
- C# Cast to Int (Convert Double to Int)
- C# Cast Examples
- C# catch Examples
- C# Change Characters in String (ToCharArray, For Loop)
- C# Char Combine: Get String From Chars
- C# char.IsDigit (If Char Is Between 0 and 9)
- C# char.IsLower and IsUpper
- C# Character Literal (const char)
- C# Char Lowercase Optimization
- C# Char Test (If Char in String Equals a Value)
- C# char.ToLower and ToUpper
- C# char Examples
- C# abstract Keyword
- C# Action Object (Lambda That Returns Void)
- C# Aggregate: Use Lambda to Accumulate Value
- C# AggressiveInlining: MethodImpl Attribute
- C# Anagram Method
- C# And Bitwise Operator
- C# Anonymous Function (Delegate With No Name)
- C# Any Method, Returns True If Match Exists
- C# StringBuilder Append and AppendLine
- C# StringBuilder AppendFormat
- ASP.NET appSettings Example
- C# ArgumentException: Invalid Arguments
- C# Array.ConvertAll, Change Type of Elements
- C# Array.Copy Examples
- C# Array.CreateInstance Method
- C# Array and Dictionary Test, Integer Lookups
- C# Array.Exists Method, Search Arrays
- C# Array.Find Examples, Search Array With Lambda
- C# Array.ForEach: Use Lambda on Every Element
- C# Array Versus List Memory Usage
- C# Array Property, Return Empty Array
- C# CharEnumerator
- C# Chart, Windows Forms (Series and Points)
- C# CheckBox: Windows Forms
- C# class Examples
- C# Clear Dictionary: Remove All Keys
- C# Clone Examples: ICloneable Interface
- C# Closest Date (Find Dates Nearest in Time)
- C# Combine Arrays: List, Array.Copy and Buffer.BlockCopy
- C# Combine Dictionary Keys
- C# ComboBox: Windows Forms
- C# CompareTo Int Method
- C# Comparison Object, Used With Array.Sort
- C# Compress Data: GZIP
- C# Console.Read Method
- C# Console.ReadKey Example
- C# Console.ReadLine Example (While Loop)
- C# Console.SetOut and Console.SetIn
- C# Console.WindowHeight
- C# Console.Write, Append With No Newline
- C# Console.WriteLine (Print)
- C# const Example
- C# Constraint Puzzle Solver
- C# Count Characters in String
- C# Count, Dictionary (Get Number of Keys)
- C# Count Letter Frequencies
- C# Count Extension Method: Use Lambda to Count
- C# CSV Methods (Parse and Segment)
- C# DataRow Field Method: Cast DataTable Cell
- C# Get Day Count Elapsed From DateTime
- C# DateTime Format
- C# DateTime.Now (Current Time)
- C# DateTime.Today (Current Day With Zero Time)
- C# DateTime.TryParse and TryParseExact
- C# DateTime Examples
- C# DateTimePicker Example
- C# Debug.Write Examples
- C# Visual Studio Debugging Tutorial
- C# decimal Examples
- C# DayOfWeek
- C# Enum.Format Method (typeof Enum)
- C# Enum.GetName, GetNames: Get String Array From Enum
- C# Enum.Parse, TryParse: Convert String to Enum
- C# Error and Warning Directives
- C# ErrorProvider Control: Windows Forms
- C# event Examples
- C# Get Every Nth Element From List (Modulo)
- C# Excel Interop Example
- C# Except (Remove Elements From Collection)
- C# Extension Method
- C# extern alias Example
- C# Convert Feet, Inches
- C# File.Delete
- C# File Equals: Compare Files
- C# File.Exists Method
- C# try Keyword
- C# TryGetValue (Get Value From Dictionary)
- C# Tuple Examples
- C# Type Class: Returned by typeof, GetType
- C# TypeInitializationException
- C# Union: Combine and Remove Duplicate Elements
- C# Unreachable Code Detected
- C# Unsafe Keyword: Fixed, Pointers
- C# Uppercase First Letter
- C# Uri and UriBuilder Classes
- C# Using Alias Example
- C# using Statement: Dispose and IDisposable
- C# value Keyword
- C# ValueTuple Examples (System.ValueTuple, ToTuple)
- C# ValueType Examples
- C# Variable Initializer for Class Field
- C# Word Count
- C# Word Interop: Microsoft.Office.Interop.Word
- C# XElement Example (XElement.Load, XName)
- C# Zip Method (Use Lambda on Two Collections)
- C# File.Move Method, Rename File
- C# File.Open Examples
- C# File.ReadAllBytes, Get Byte Array From File
- C# File.ReadAllLines, Get String Array From File
- C# File.ReadAllText, Get String From File
- C# File.ReadLines, Use foreach Over Strings
- C# File.Replace Method
- C# FileInfo Length, Get File Size
- C# FileInfo Examples
- C# File Handling
- C# Filename With Date Example (DateTime.Now)
- C# FileNotFoundException (catch Example)
- C# FileStream Length, Get Byte Count From File
- C# FileStream Example, File.Create
- C# FileSystemWatcher Tutorial (Changed, e.Name)
- C# finally Keyword
- C# First Sentence
- C# FirstOrDefault (Get First Element If It Exists)
- C# Fisher Yates Shuffle: Generic Method
- C# fixed Keyword (unsafe)
- C# Flatten Array (Convert 2D to 1D)
- C# First Words in String
- C# First (Get Matching Element With Lambda)
- C# ContainsKey Method (Key Exists in Dictionary)
- C# Convert ArrayList to Array (Use ToArray)
- C# Convert ArrayList to List
- C# Convert Bool to Int
- C# Convert Bytes to Megabytes
- C# Convert Degrees Celsius to Fahrenheit
- C# Convert Dictionary to List
- C# Convert Dictionary to String (Write to File)
- C# Convert List to Array
- C# Convert List to DataTable (DataGridView)
- C# Convert List to String
- C# Convert Miles to Kilometers
- C# Convert Milliseconds, Seconds, Minutes
- C# Convert Nanoseconds, Microseconds, Milliseconds
- C# Convert String Array to String
- C# Convert TimeSpan to Long
- C# Convert Types
- C# Copy Dictionary
- C# Count Elements in Array and List
- C# FromOADate and Excel Dates
- C# Generic Class, Generic Method Examples
- C# GetEnumerator: While MoveNext, Get Current
- C# GetHashCode (String Method)
- C# Thumbnail Image With GetThumbnailImage
- C# GetType Method
- C# Global Variable Examples (Public Static Property)
- ASP.NET Global Variables Example
- C# Group By Operator: LINQ
- GroupBox: Windows Forms
- C# GroupBy Method: LINQ
- C# GroupJoin Method
- C# GZipStream Example (DeflateStream)
- C# HashSet Examples
- C# Hashtable Examples
- HelpProvider Control Use
- C# HTML and XML Handling
- C# HtmlEncode and HtmlDecode
- C# HtmlTextWriter Example
- C# HttpUtility.HtmlEncode Methods
- C# HybridDictionary
- C# default Operator
- C# DefaultIfEmpty Method
- C# Define and Undef Directives
- C# Destructor
- C# DialogResult: Windows Forms
- C# Dictionary, Read and Write Binary File
- C# Dictionary Memory
- C# Dictionary Optimization, Increase Capacity
- C# Dictionary Optimization, Test With ContainsKey
- C# DictionaryEntry Example (Hashtable)
- C# Directives
- C# Directory.CreateDirectory, Create New Folder
- C# Directory.GetFiles Example (Get List of Files)
- C# DivideByZeroException
- C# DllImport Attribute
- C# Do While Loop Example
- C# DriveInfo Examples
- C# DropDownItems Control
- C# IComparable Example, CompareTo: Sort Objects
- C# IDictionary Generic Interface
- C# IEnumerable Examples
- C# IList Generic Interface: List and Array
- C# Image Type
- C# ImageList Use: Windows Forms
- C# Increment String That Contains a Number
- C# Increment, Preincrement and Decrement Ints
- Dot Net Perls
- C# Indexer Examples (This Keyword, get and set)
- C# IndexOutOfRangeException
- C# Inheritance
- C# Insert String Examples
- C# int Array
- C# Interface Examples
- C# Interlocked Examples: Add, CompareExchange
- C# Intersect: Get Common Elements
- C# InvalidCastException
- C# InvalidOperationException: Collection Was Modified
- C# IOException Type: File, Directory Not Found
- C# IOrderedEnumerable (Query Expression With orderby)
- C# is: Cast Examples
- C# IsFixedSize, IsReadOnly and IsSynchronized Arrays
- C# string.IsNullOrEmpty, IsNullOrWhiteSpace
- C# IsSorted Method: If Array Is Already Sorted
- C# Jagged Array Examples
- C# join Examples (LINQ)
- C# KeyCode Property and KeyDown
- C# KeyNotFoundException: Key Not Present in Dictionary
- C# KeyValuePair Examples
- C# Line Count for File
- C# Line Directive
- C# LinkedList
- C# List CopyTo (Copy List Elements to Array)
- C# List Equals (If Elements Are the Same)
- C# List Find and Exists Examples
- C# List Insert Performance
- ASP.NET LiteralControl Example
- C# Locality Optimizations (Memory Hierarchy)
- C# lock Keyword
- C# Long and ulong Types
- C# Loop Over String Chars: Foreach, For
- C# Loop Over String Array
- C# Main args Examples
- C# Map Example
- ASP.NET MapPath: Virtual and Physical Paths
- C# Mask Optimization
- C# MaskedTextBox Example
- C# Math.Abs: Absolute Value
- C# Math.Ceiling Usage
- C# Math.Floor Method
- C# Math.Max and Math.Min Examples
- C# Math.Pow Method, Exponents
- C# Math.Round Examples: MidpointRounding
- C# Math Type
- C# Max and Min: Get Highest or Lowest Element
- C# MemoryFailPoint and InsufficientMemoryException
- C# MemoryStream: Use Stream on Byte Array
- C# MenuStrip: Windows Forms
- C# Modulo Operator: Get Remainder From Division
- C# MonthCalendar Control: Windows Forms
- C# Multiple Return Values
- C# Multiply Numbers
- C# namespace Keyword
- C# NameValueCollection Usage
- C# Nested Lists: Create 2D List or Jagged List
- C# Nested Switch Statement
- C# Environment.NewLine
- C# Normalize, IsNormalized Methods
- C# Null String Example
- C# null Keyword
- C# Nullable Examples
- C# NullReferenceException and Null Parameter
- C# Object Array
- C# Obsolete Attribute
- C# OfType Examples
- C# OpenFileDialog Example
- C# operator Keyword
- C# Odd and Even Numbers
- C# Bitwise Or
- C# orderby Query Keyword
- C# out Parameter
- C# OutOfMemoryException
- C# OverflowException
- C# Overload Method
- C# Override Method
- C# PadRight and PadLeft: String Columns
- C# Get Paragraph From HTML With Regex
- C# Parallel.For Example (Benchmark)
- C# Parallel.Invoke: Run Methods on Separate Threads
- C# Parameter Optimization
- C# Parameter Passing, ref and out
- C# params Keyword
- C# int.Parse: Convert Strings to Integers
- C# partial Keyword
- C# Path.ChangeExtension
- C# Path Exists Example
- C# Path.GetExtension: File Extension
- C# Path.GetRandomFileName Method
- C# Pragma Directive
- C# Predicate (Lambda That Returns True or False)
- C# Pretty Date Format (Hours or Minutes Ago)
- C# PreviewKeyDown Event
- C# Random Lowercase Letter
- C# Random Paragraphs and Sentences
- C# Random String
- C# Random Number Examples
- C# StreamReader ReadLine, ReadLineAsync Examples
- C# readonly Keyword
- C# Recursion Example
- C# Regex, Read and Match File Lines
- C# Regex Groups, Named Group Example
- C# Regex.Matches Quote Example
- C# Regex.Matches Method: foreach Match, Capture
- C# Regex.Replace, Matching End of String
- C# Regex.Replace, Remove Numbers From String
- C# Regex.Replace, Merge Multiple Spaces
- C# Regex.Replace Examples: MatchEvaluator
- C# Regex.Split, Get Numbers From String
- C# Regex.Split Examples
- C# Regex Trim, Remove Start and End Spaces
- C# RegexOptions.Compiled
- C# RegexOptions.IgnoreCase Example
- C# RegexOptions.Multiline
- C# Region and endregion
- C# Remove Char From String at Index
- C# Remove Element
- C# Remove HTML Tags
- C# Remove String
- C# Reserved Filenames
- C# return Keyword
- C# Reverse String
- C# Reverse Words
- C# Reverse Extension Method
- C# RichTextBox Example
- C# Right String Part
- C# RNGCryptoServiceProvider Example
- C# ROT13 Method, Char Lookup Table
- C# SelectMany Example: LINQ
- C# Sentinel Optimization
- C# SequenceEqual Method (If Two Arrays Are Equal)
- C# Shift Operators (Bitwise)
- C# Short and ushort Types
- C# Single Method: Get Element If Only One Matches
- C# SingleOrDefault
- C# Singleton Pattern Versus Static Class
- C# Singleton Class
- C# sizeof Keyword
- C# Skip and SkipWhile Examples
- C# Sleep Method (Pause)
- C# Sort Dictionary: Keys and Values
- C# Sort by File Size
- C# Sort, Ignore Leading Chars
- C# Sort KeyValuePair List: CompareTo
- C# Sort Strings by Length
- C# Thread.SpinWait Example
- C# Math.Sqrt Method
- C# stackalloc Operator
- C# StackOverflowException
- C# StartsWith and EndsWith String Methods
- C# Static Array
- C# Static Dictionary
- C# Stopwatch Examples
- C# Stream
- C# StreamReader ReadToEnd Example (Read Entire File)
- C# StreamReader ReadToEndAsync Example (Performance)
- C# StreamReader Examples
- C# StreamWriter Examples
- C# String Append (Add Strings)
- C# String Compare and CompareTo Methods
- C# String Constructor (new string)
- C# string.Copy Method
- C# CopyTo String Method: Put Chars in Array
- C# Empty String Examples
- C# String Equals Examples
- C# String For Loop, Count Spaces in String
- C# string.Intern and IsInterned
- C# String IsUpper, IsLower
- C# String Length Property: Get Character Count
- C# String Literal: Newline and Quote Examples
- C# StringBuilder Capacity
- C# StringBuilder Clear (Set Length to Zero)
- C# StringBuilder Data Types
- C# StringBuilder Performance
- C# StringBuilder Equals (If Chars Are Equal)
- C# StringBuilder Memory
- C# StringBuilder ToString: Get String From StringBuilder
- C# StringWriter Class
- C# Sum Method: Add up All Numbers
- C# Switch Char, Test Chars With Cases
- C# Switch Enum
- C# System (using System namespace)
- C# Tag Property: Windows Forms
- C# TextInfo Examples
- C# TextReader, Returned by File.OpenText
- C# TextWriter, Returned by File.CreateText
- C# this Keyword
- C# ThreadPool
- C# Thread Join Method (Join Array of Threads)
- C# ThreadPool.SetMinThreads Method
- C# TimeZone Examples
- C# Get Title From HTML With Regex
- C# ToArray Extension Method
- C# ToCharArray: Convert String to Array
- C# ToDictionary Method
- C# Token
- C# ToList Extension Method
- C# ToLookup Method (Get ILookup)
- C# ToLower and ToUpper: Uppercase and Lowercase Strings
- ToolStripContainer Control: Dock, Properties
- C# ToolTip: Windows Forms
- C# ToString Integer Optimization
- C# ToString: Get String From Object
- C# ToTitleCase Method
- C# TrackBar: Windows Forms
- C# Tree and Nodes Example: Directed Acyclic Word Graph
- C# TreeView Tutorial
- C# Trim Strings
- C# Thread Methods
- C# History
- C# Features
- C# Variables
- C# Data Types
- C# Operators
- C# Keywords
- C# New Features | C# Version Features
- C# Programs
- C# Program to swap numbers without third variable
- C# Program to convert Decimal to Binary
- C# Program to Convert Number in Characters
- C# Program to Print Alphabet Triangle
- C# Program to print Number Triangle
- C# Program to generate Fibonacci Triangle
- C# String Compare() method
- C# String CompareOrdinal() method
- C# String CompareTo() method
- C# String Concat() method
- C# String Contains() method
- C# String CopyTo() method
- C# String EndsWith() method
- C# String Equals() method
- C# String Format() method
- C# String IndexOf() method
- C# String Insert() method
- C# String Intern(String str) method
- C# String IsInterned() method
- C# String Normalize() method
- C# String IsNullOrEmpty() method
- C# String IsNullOrWhiteSpace() method
- C# String Join() method
- C# String LastIndexOf() method
- C# String LastIndexOfAny() method
- C# String PadLeft() method
- C# String PadRight() method
- C# Nullable
- C# String Remove() method
- C# String Replace() method
- C# String Split() method
- C# String StartsWith() method
- C# String SubString() method
- C# Partial Types
- C# Iterators
- C# Delegate Covariance
- C# Delegate Inference
- C# Anonymous Types
- C# Extension Methods
- C# Query Expression
- C# Partial Method
- C# Implicitly Typed Local Variable
- C# Object and Collection Initializer
- C# Auto Implemented Properties
- C# Dynamic Binding
- C# Named and Optional Arguments
- C# Asynchronous Methods
- C# Caller Info Attributes
- C# Using Static Directive
- C# Exception Filters
- C# Await in Catch Finally Blocks
- C# Default Values for Getter Only Properties
- C# Expression Bodied Members
- C# Null Propagator
- C# String Interpolation
- C# nameof operator
- C# Dictionary Initializer
- C# Pattern Matching
- C# Tuples
- C# Deconstruction
- C# Local Functions
- C# Binary Literals
- C# Ref Returns and Locals
- C# Expression Bodied Constructors and Finalizers
- C# Expression Bodied Getters and Setters
- C# Async Main
- C# Default Expression
Related Links
Adjectives
Ado
Ai
Android
Angular
Antonyms
Apache
Articles
Asp
Autocad
Automata
Aws
Azure
Basic
Binary
Bitcoin
Blockchain
C
Cassandra
Change
Coa
Computer
Control
Cpp
Create
Creating
C-Sharp
Cyber
Daa
Data
Dbms
Deletion
Devops
Difference
Discrete
Es6
Ethical
Examples
Features
Firebase
Flutter
Fs
Git
Go
Hbase
History
Hive
Hiveql
How
Html
Idioms
Insertion
Installing
Ios
Java
Joomla
Js
Kafka
Kali
Laravel
Logical
Machine
Matlab
Matrix
Mongodb
Mysql
One
Opencv
Oracle
Ordering
Os
Pandas
Php
Pig
Pl
Postgresql
Powershell
Prepositions
Program
Python
React
Ruby
Scala
Selecting
Selenium
Sentence
Seo
Sharepoint
Software
Spellings
Spotting
Spring
Sql
Sqlite
Sqoop
Svn
Swift
Synonyms
Talend
Testng
Types
Uml
Unity
Vbnet
Verbal
Webdriver
What
Wpf