<< Back to C-SHARP
C# enum Examples
Store named and magic constants with enums. Use enums in collections, if and switch.Enum. In the forest a flower grows. This flower has many attributes. It is blooming. It is purple (not blue or yellow). A gentle wind passes through the region.
With an enum, we can classify this plant. Consider that flowers come in all colors. It could use FlowerColor.Purple. Another one could be Blue.
Enum example. Here is an enum that expresses importance. An enum type internally contains an enumerator list. The values (like Trivial and Critical) are ints like 1 and 4.
Int: The underlying value of this enum is the default, which is int. When we use an Importance variable, we are using an int.
If: We test the enum value with an if-statement that includes an if-else block. The value is Critical, so "True" is printed.
C# program that uses enums
using System;
class Program
{
enum Importance
{
None,
Trivial,
Regular,
Important,
Critical
}
static void Main()
{
// ... An enum local variable.
Importance value = Importance.Critical;
// ... Test against known Importance values.
if (value == Importance.Trivial)
{
Console.WriteLine("Not true");
}
else if (value == Importance.Critical)
{
Console.WriteLine("True");
}
}
}
Output
True
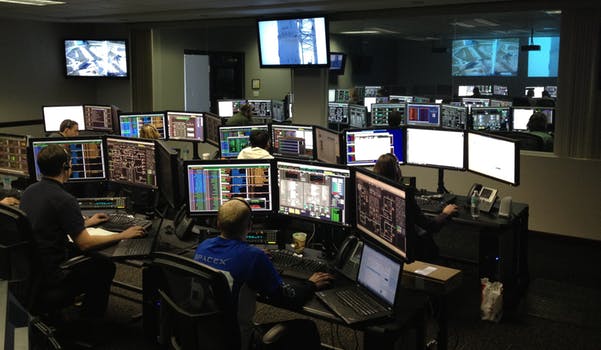
Enum advantages. With an enum, magic constants are separate. This modular design makes things easier to understand. Fewer bugs will be introduced.
Example: Consider this program—the color Blue is supposed to have the value 9001. We can store this in an enum to ease maintenance.
IntelliSense: Enums can be used with IntelliSense in Visual Studio. This feature will guess the value you are typing.
Press tab: We can simply press tab and select the enum type we want. This is an advantage to using enum types.
C# program that shows enum advantages
using System;
enum CaseColor
{
Uncolored = 0,
Red = 8001,
Blue = 9001
}
class Program
{
static void Main()
{
// This makes sense to read.
Console.WriteLine("COLOR: {0}, {1}",
CaseColor.Blue,
(int)CaseColor.Blue);
// This is more confusing to read.
Console.WriteLine("COLOR: {0}, {1}",
"Blue",
9001);
}
}
Output
COLOR: Blue, 9001
COLOR: Blue, 9001
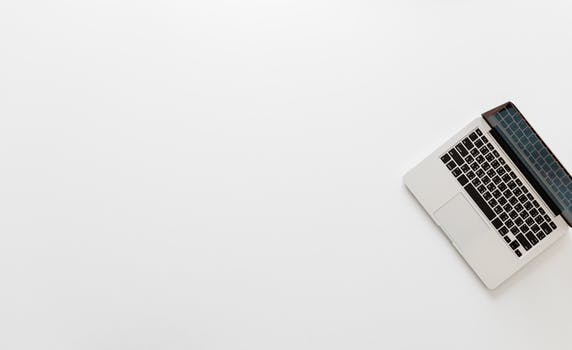
Debugger. We examine what enums look like in the Visual Studio debugger. We see that enums are strongly-typed. You cannot assign them to just any value.
Note: The debugger shows that "tagValue" is of type Program.TagType. Internally it is represented as an integer.
C# program that uses enums with Debugger
using System;
class Program
{
enum TagType
{
None,
BoldTag,
ItalicsTag,
HyperlinkTag,
}
static void Main()
{
// Specify a tag instance.
TagType tagValue = TagType.BoldTag;
if (tagValue == TagType.BoldTag)
{
// Will be printed.
Console.WriteLine("Bold");
}
if (tagValue == TagType.HyperlinkTag)
{
// This is not printed.
Console.WriteLine("Not true");
}
}
}
Output
Bold
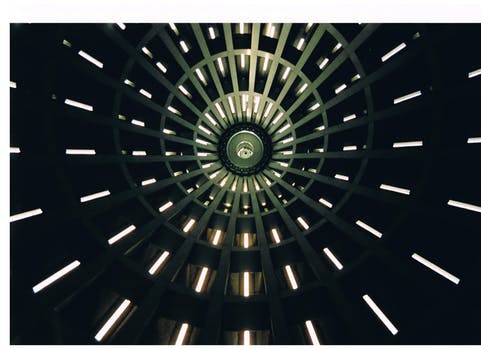
Strings. We convert enums to strings for display on the Console. Enum values always have a name, such as TagType.None (in the above example).
Tip: To print out the enum values, you can call ToString on the enum variable in a program.
Also: Another method such as Console.WriteLine can automatically call the ToString method.
ToString: Internally, ToString invokes methods that use reflection to acquire the string representation.
Enum ToStringC# program that uses enums with Console.WriteLine
class Program
{
enum Visibility
{
None,
Hidden = 2,
Visible = 4
}
enum AnimalType
{
None,
Cat = 1,
Dog = 2
}
static void Main()
{
// ... Two enum variables.
AnimalType animal = AnimalType.Dog;
Visibility visible = Visibility.Hidden;
// ... Use Console.WriteLine to print out heir values.
System.Console.WriteLine(animal);
System.Console.WriteLine(visible);
}
}
Output
Dog
Hidden
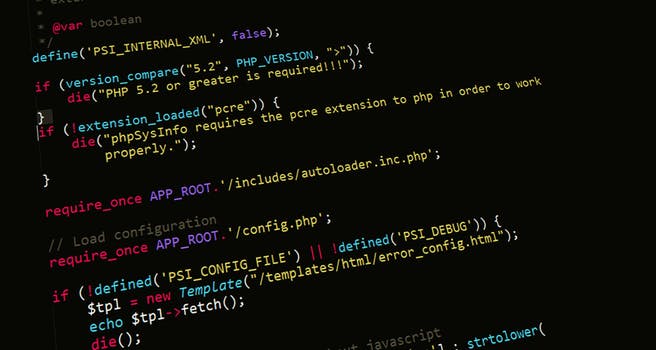
Switch. We often use if-statements with enums. But switch is another option. And switch is sometimes compiled to more efficient IL.
Switch EnumIsFormat: This method works as a filter that tells us something about sets of enum values—it contains a switch statement.
Logic: We can separate the logic here instead of repeating ourselves. This helps clarify the program logic.
C# program that uses enums in switch
using System;
class Program
{
enum FormatType
{
None,
BoldFormat, // Is a format value.
ItalicsFormat, // Is a format value.
Hyperlink // Not a format value.
}
static void Main()
{
// ... Test enum with switch method.
FormatType formatValue = FormatType.None;
if (IsFormat(formatValue))
{
// This is not reached, as None does not return a true value in IsFormat.
Console.WriteLine("Error");
}
// ... Test another enum with switch.
formatValue = FormatType.ItalicsFormat;
if (IsFormat(formatValue))
{
// This is printed, as we receive true from IsFormat.
Console.WriteLine("True");
}
}
/// <summary>
/// Returns true if the FormatType is Bold or Italics.
/// </summary>
static bool IsFormat(FormatType value)
{
switch (value)
{
case FormatType.BoldFormat:
case FormatType.ItalicsFormat:
{
// These 2 values are format values.
return true;
}
default:
{
// The argument is not a format value.
return false;
}
}
}
}
Output
True
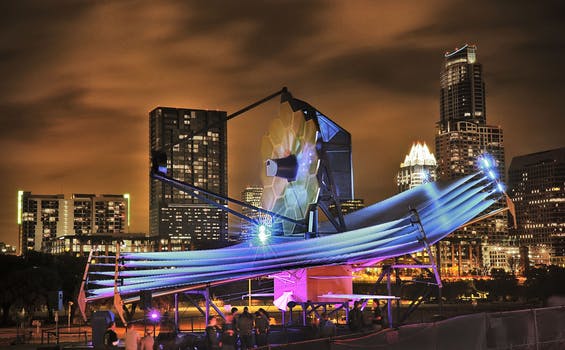
Default. Values are always initialized to zero when they are fields of a class. Upon class creation, an enum field will also be initialized to zero (and the equivalent value).
Tip: To make enums valid, always use the default value of zero. This way, we can test for the default value of fields.
Sometimes: This issue is not worth fixing. But it is often useful for verifying correctness.
Also: You can place a semicolon at the end of an enum block (but this is not required or helpful usually).
C# program that shows default enum value
using System;
enum CatFurColor
{
None,
Orange,
Grey
};
class Program
{
static CatFurColor _color;
static void Main()
{
// The enum is a field, so it has its default value of None.
Console.WriteLine("DEFAULT ENUM VALUE: {0}", _color);
}
}
Output
DEFAULT ENUM VALUE: None
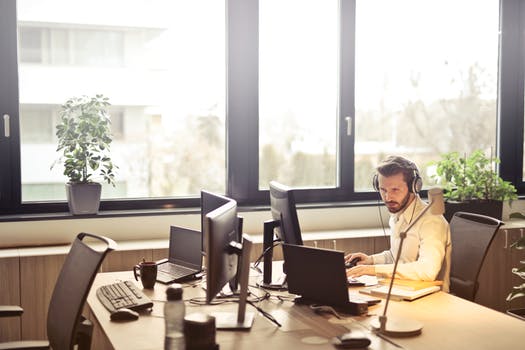
Collections. Here we apply the Stack collection in the .NET Framework. With Stack, we can develop a parser that keeps the most recently encountered enum value on the top.
Stack: The Stack here can only have TagType values added to it. This is an example of type checking and validation.
StackPop: With the Pop method we get the top element from the stack. This is of type TagType.ItalicsTag.
Result: In the execution of this program, the stack has two enums added and one removed.
C# program that uses enums with Stack
using System.Collections.Generic;
class Program
{
enum TagType
{
None, // integer value = 0
BoldTag, // 1
ItalicsTag, // 2
HyperlinkTag, // 3
}
static void Main()
{
// Create a Stack of enums.
var stack = new Stack<TagType>();
// ... Add enum values to our Stack.
stack.Push(TagType.BoldTag); // Add bold.
stack.Push(TagType.ItalicsTag); // Add italics.
// ... Get the top enum value.
TagType thisTag = stack.Pop(); // Get top tag.
System.Console.WriteLine("POP RESULT: " + thisTag);
// Peek at the top.
var peeked = stack.Peek();
System.Console.WriteLine("PEEK RESULT: " + peeked);
}
}
Output
POP RESULT: ItalicsTag
PEEK RESULT: BoldTag
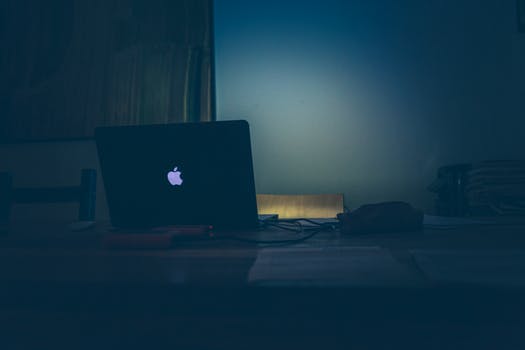
Type. An enum has an underlying type. Each time we use the enum, we are using the underlying type. The enum has syntactic sugar on top.
Int: Enums are by default an int type, but we can adjust this to a different numeric type.
Byte: Here we create an enum with a type of byte. This is sometimes useful on small enums. A byte can only contain 256 different values.
ByteMemory: The CoffeeSize enum will use memory equivalent to a byte. This can make classes more efficient and smaller.
C# program that uses underlying type
using System;
class Program
{
enum CoffeeSize : byte
{
None,
Tall,
Venti,
Grande
};
static void Main()
{
// ... Create a coffee size local.
CoffeeSize size = CoffeeSize.Venti;
Console.WriteLine(size);
}
}
Output
Venti
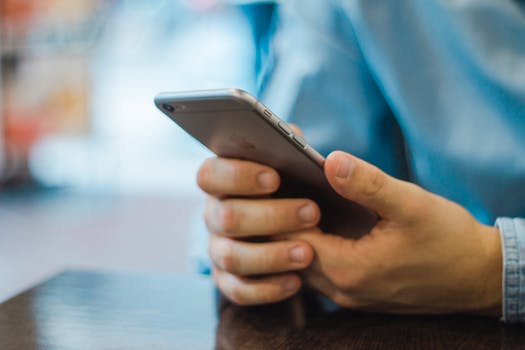
GetUnderlyingType. We can determine an enum's type (like int) at runtime. Enum.GetUnderlyingType, a static method, determines the underlying type.
StaticNext: We declare an enum Importance. For this example it uses an underlying type of byte.
Then: When the GetUnderlyingType method is called, the System.Byte type is returned.
C# program that uses GetUnderlyingType
using System;
class Program
{
enum Importance : byte
{
Low,
Medium,
High
};
static void Main()
{
// Determine the underlying type of the enum.
Type type = Enum.GetUnderlyingType(typeof(Importance));
Console.WriteLine(type);
}
}
Output
System.Byte
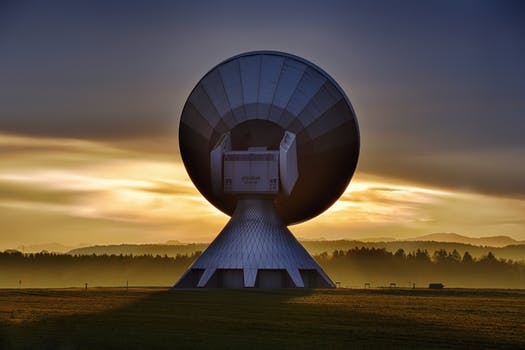
Null, none. An enum value cannot be null. It is a value type like an int. To avoid the "cannot convert null" error, use a special None constant as the first enum item.
C# program that causes null error
enum Color
{
None,
Blue,
Red
}
class Program
{
static void Main()
{
Color c = null;
}
}
Output
Error CS0037
Cannot convert null to 'Color' because it is a non-nullable value type
C# program that uses None enum
enum Color
{
None,
Blue,
Red
}
class Program
{
static void Main()
{
Color c = Color.None;
}
}
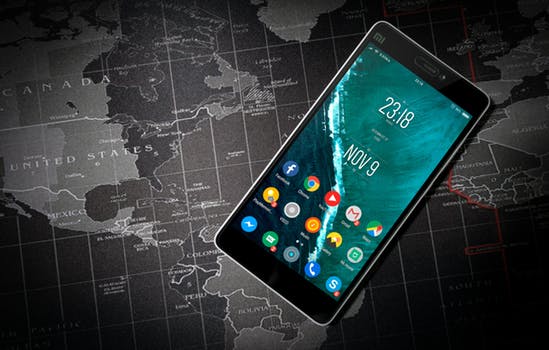
Flags. The C# language allows us to specify a Flags attribute on an enum. This enables the enum to be used as a bit field. We can use combinations of enum values this way.
Enum FlagsHere: We have an enum called DataInfo that has 4 different flag values. We set 2 of them at once in Main().
And: In the if-statement, we find that OptionA and OptionC are set to true, but OptionB is not set. This is the correct result.
C# program that shows enum flags
using System;
class Program
{
[Flags]
enum DataInfo
{
None = 0,
OptionA = 1,
OptionB = 2,
OptionC = 4,
}
static void Main()
{
var info = DataInfo.OptionA | DataInfo.OptionC;
// See if current flag is set.
if ((info & DataInfo.OptionA) == DataInfo.OptionA &&
(info & DataInfo.OptionB) != DataInfo.OptionB && // Not OptionB.
(info & DataInfo.OptionC) == DataInfo.OptionC)
{
Console.WriteLine("Has OptionA, OptionC, but not OptionB.");
}
}
}
Output
Has OptionA, OptionC, but not OptionB.
Benchmark, enum. Enums are fast. They are almost never a performance concern. They are just syntactic sugar on a type like int, which is also fast.
Version 1: This version of the code tests for an enum value. We run it in a tight loop for many iterations.
Version 2: This codes tests an int. By comparing these versions of the code, we can see any possible performance impact from enums.
Result: The enum test takes the same amount of time as the int test—the enum carries no performance penalty over int.
C# program that benchmarks enum, int
using System;
using System.Diagnostics;
class Program
{
enum TestType
{
None,
Valid,
Invalid
}
const int _max = 10000000;
static void Main()
{
// Version 1: use enum in if-statement.
var s1 = Stopwatch.StartNew();
var temp1 = TestType.Valid;
for (int i = 0; i < _max; i++)
{
if (temp1 == TestType.Invalid)
{
return;
}
}
s1.Stop();
// Version 2: use int in if-statement.
var s2 = Stopwatch.StartNew();
var temp2 = 0;
for (int i = 0; i < _max; i++)
{
if (temp2 == 2)
{
return;
}
}
s2.Stop();
Console.WriteLine(((double)(s1.Elapsed.TotalMilliseconds * 1000000) /
_max).ToString("0.00 ns"));
Console.WriteLine(((double)(s2.Elapsed.TotalMilliseconds * 1000000) /
_max).ToString("0.00 ns"));
}
}
Output
0.27 ns if enum
0.27 ns if int
IL, enum. We can determine the exact performance of enums by examining the IL (intermediate language) of a compiled C# program. Enums are loaded with ldc.i4.
And: This means "load constant integer of 4 bytes." So an enum of just a constant 4-byte integer, exactly like any other const int.
Program: This has an enum, 3 const ints, and 3 static readonly ints. The IL shows how the values are loaded onto the evaluation stack.
Results: Enums and const ints are loaded with ld.c, so they perform the same. But static ints are loaded with ldsfld, which is slower.
C# program that uses enum, const, fields
using System;
enum Test
{
Cat,
Dog,
Rabbit
}
class Program
{
const int _cat = 0;
const int _dog = 1;
const int _rabbit = 2;
static readonly int _cat2 = 0;
static readonly int _dog2 = 1;
static readonly int _rabbit2 = 2;
static void Main()
{
Method(Test.Cat);
Method(Test.Dog);
Method(Test.Rabbit);
Method(_cat);
Method(_dog);
Method(_rabbit);
Method(_cat2);
Method(_dog2);
Method(_rabbit2);
}
static void Method(Test test)
{
}
static void Method(int value)
{
}
}
Compiled Main method from program: IL
.method private hidebysig static void Main() cil managed
{
.entrypoint
.maxstack 1
L_0000: ldc.i4.0
L_0001: call void Program::Method(valuetype Test)
L_0006: ldc.i4.1
L_0007: call void Program::Method(valuetype Test)
L_000c: ldc.i4.2
L_000d: call void Program::Method(valuetype Test)
L_0012: ldc.i4.0
L_0013: call void Program::Method(int32)
L_0018: ldc.i4.1
L_0019: call void Program::Method(int32)
L_001e: ldc.i4.2
L_001f: call void Program::Method(int32)
L_0024: ldsfld int32 Program::_cat2
L_0029: call void Program::Method(int32)
L_002e: ldsfld int32 Program::_dog2
L_0033: call void Program::Method(int32)
L_0038: ldsfld int32 Program::_rabbit2
L_003d: call void Program::Method(int32)
L_0042: ret
}
Parse. Sometimes we have a string value that we want to convert to an equivalent enum. This could happen when accepting user input.
Tip: When using the .NET Framework, calling a built-in method to do conversions (where one exists) is usually best.
Enum.Parse: The tricky part of using this method involves typeof and casting. It is best to avoid this if possible.
Enum.Parse
GetName, Getnames. Built-in methods get strings that represent enums. With GetName, we can get the name for an enum value. With GetNames we get all the string representations at once.
Enum.GetName
Format enums. It is possible to format the values stored in enums in different ways. We can display an integer representation, or a hex representation.
Enum.FormatFxCop. This is a code analysis tool released by Microsoft. It helps us improve certain issues in code. It will tell us that "enums should have zero value."
Quote: A non-flags attributed enumeration should define a member with the value of zero so that the default value is a valid value of the enumeration. If appropriate, name the member None.
Enums should have zero value: microsoft.comArrays. Enums are values. We can use enums to index arrays. This approach is useful for some kinds of tables or data structures in programs.
Enum ArrayMemory. Suppose we develop a class that has an enum field. The underlying type of this enum contributes to how much memory the class will use.
Memory, continued. A smaller type (such as byte) will make class instances that hold the enum smaller. Thousands of class instances are required before this optimization is important.
A review. Enums enhance clarity and reduce the probability of invalid constants. We use them to represent constant values (such as integers) in a consistent way.
Related Links:
- C# Array Examples, String Arrays
- C# ArrayList Examples
- C# ArraySegment: Get Array Range, Offset and Count
- C# break Statement
- C# Buffer BlockCopy Example
- C# BufferedStream: Optimize Read and Write
- 404 Not Found
- C# 24 Hour Time Formats
- C# 2D Array Examples
- 7 Zip Command Line Examples
- C# 7 Zip Executable (Process.Start)
- C# All Method: All Elements Match a Condition
- C# Alphabetize String
- C# Alphanumeric Sorting
- C# Arithmetic Expression Optimization
- C# Array.AsReadOnly Method (ObjectModel)
- C# Array.BinarySearch Method
- C# Array.Clear Examples
- C# Array.IndexOf, LastIndexOf: Search Arrays
- C# async, await Examples
- C# Attribute Examples
- C# Average Method
- C# BackgroundWorker
- C# base Keyword
- C# String Between, Before, After
- C# Binary Representation int (Convert, toBase 2)
- C# BinarySearch List
- C# bool Array (Memory Usage, One Byte Per Element)
- C# bool.Parse, TryParse: Convert String to Bool
- C# bool Type
- C# Array Length Property, Get Size of Array
- C# Button Example
- C# Byte Array: Memory Usage, Read All Bytes
- C# Byte and sbyte Types
- C# Capacity for List, Dictionary
- C# Case Insensitive Dictionary
- C# case Example (Switch Case)
- C# Char Array
- C# Checked and Unchecked Keywords
- C# CheckedListBox: Windows Forms
- C# Color Table
- C# Color Examples: FromKnownColor, FromName
- C# ColorDialog Example
- C# Comment: Single Line and Multiline
- C# Concat Extension: Combine Lists and Arrays
- C# Conditional Attribute
- C# Console Color, Text and BackgroundColor
- C# String Clone() method
- C# Constructor Examples
- C# Contains Extension Method
- C# String GetTypeCode() method
- C# String ToLowerInvariant() method
- C# Customized Dialog Box
- C# DataColumn Example: Columns.Add
- C# DataGridView Add Rows
- DataGridView Columns, Edit Columns Dialog
- C# DataGridView Row Colors (Alternating)
- C# DataGridView Tutorial
- C# DataGridView
- C# DataRow Examples
- C# DataSet Examples
- C# DataSource Example
- C# DataTable Compare Rows
- C# DataTable foreach Loop
- C# DataTable RowChanged Example: AcceptChanges
- C# DataTable Select Example
- C# DataTable Examples
- C# DataView Examples
- C# String ToString() method
- C# String ToUpper() method
- C# Digit Separator
- C# DateTime.MinValue (Null DateTime)
- C# DateTime.Month Property
- C# DateTime.Parse: Convert String to DateTime
- C# DateTime Subtract Method
- C# Decompress GZIP
- C# Remove Duplicates From List
- C# dynamic Keyword
- C# ElementAt, ElementAtOrDefault Use
- C# Encapsulate Field
- C# Enum Array Example, Use Enum as Array Index
- C# enum Flags Attribute Examples
- C# Enum ToString: Convert Enum to String
- C# enum Examples
- C# Enumerable.Range, Repeat and Empty
- C# Environment Type
- C# EventLog Example
- C# Exception Handling
- C# explicit and implicit Keywords
- C# Factory Design Pattern
- C# File.Copy Examples
- C# typeof and nameof Operators
- C# String TrimEnd() method
- C# var Examples
- C# virtual Keyword
- C# void Method, Return No Value
- C# volatile Example
- C# WebBrowser Control (Navigate Method)
- C# WebClient: DownloadData, Headers
- C# Where Method and Keyword
- C# String TrimStart() method
- C# delegate Keyword
- C# descending, ascending Keywords
- C# while Loop Examples
- C# Whitespace Methods: Convert UNIX, Windows Newlines
- C# XmlReader, Parse XML File
- C# XmlTextReader
- C# XmlTextWriter
- C# XmlWriter, Create XML File
- C# XOR Operator (Bitwise)
- C# yield Example
- C# float Numbers
- FlowLayoutPanel Control
- C# Focused Property
- C# FolderBrowserDialog Control
- C# Font Type: FontFamily and FontStyle
- C# FontDialog Example
- C# for Loop Examples
- C# foreach Loop Examples
- ForeColor, BackColor: Windows Forms
- C# Form: Event Handlers
- C# Contains String Method
- C# ContainsValue Method (Value Exists in Dictionary)
- C# ContextMenuStrip Example
- C# continue Keyword
- C# Control: Windows Forms
- C# Windows Forms Controls
- C# Convert Char Array to String
- C# Convert Char to String
- C# Convert Days to Months
- C# Convert String to Byte Array
- C# String Format
- C# Func Object (Lambda That Returns a Value)
- C# GC.Collect Examples: CollectionCount, GetTotalMemory
- C# Path.GetDirectoryName (Remove File From Path)
- C# goto Examples
- C# HttpClient Example: System.Net.Http
- ASP.NET HttpContext Request Property
- IL Disassembler Tutorial
- C# Intermediate Language (IL)
- C# IndexOf Examples
- C# IndexOfAny Examples
- C# Initialize Array
- C# Initialize List
- C# InitializeComponent Method: Windows Forms
- C# Inline Optimization
- C# Dictionary Equals: If Contents Are the Same
- C# Dictionary Versus List Loop
- C# Dictionary Order, Use Keys Added Last
- C# Dictionary Size and Performance
- C# Dictionary Versus List Lookup Time
- C# Dictionary Examples
- C# Get Directory Size (Total Bytes in All Files)
- C# Directory Type
- C# Distinct Method, Get Unique Elements Only
- C# Divide by Powers of Two (Bitwise Shift)
- C# Divide Numbers (Cast Ints)
- C# DomainUpDown Control Example
- C# Double Type: double.MaxValue, double.Parse
- C# Remove Duplicate Chars
- C# IEqualityComparer
- C# If Preprocessing Directive: Elif and Endif
- C# If Versus Switch Performance
- C# if Statement
- C# int.MaxValue, MinValue (Get Lowest Number)
- C# Program to reverse number
- C# Int and uint Types
- C# Integer Append Optimization
- C# Keywords
- C# Label Example: Windows Forms
- C# Lambda Expressions
- C# LastIndexOf Examples
- C# Last, LastOrDefault Methods
- C# Mutex Example (OpenExisting)
- C# Named Parameters
- C# Let Keyword (Use Variable in Query Expression)
- C# Levenshtein Distance
- C# LinkLabel Example: Windows Forms
- C# LINQ
- C# List Add Method, Append Element to List
- C# List AddRange, InsertRange (Append Array to List)
- C# List Clear Example
- C# List Contains Method
- C# List Remove Examples
- C# List Examples
- C# ListBox Tutorial (DataSource, SelectedIndex)
- C# ListView Tutorial: Windows Forms
- C# Maze Pathfinding Algorithm
- C# Memoization
- C# Memory Usage for Arrays of Objects
- C# MessageBox.Show Examples
- C# Method Call Depth Performance
- C# Method Parameter Performance
- C# Method Size Optimization
- C# Multidimensional Array
- C# MultiMap Class (Dictionary of Lists)
- C# Optimization
- C# new Keyword
- C# NotifyIcon: Windows Forms
- C# NotImplementedException
- C# Null Array
- C# String GetType() method
- C# Null Coalescing and Null Conditional Operators
- C# Null List (NullReferenceException)
- C# Numeric Casts
- C# NumericUpDown Control: Windows Forms
- C# object.ReferenceEquals Method
- C# Object Examples
- C# Optional Parameters
- C# Prime Number
- C# OrderBy, OrderByDescending Examples
- C# Process Examples (Process.Start)
- Panel, Windows Forms (Create Group of Controls)
- C# Path Examples
- C# Get Percentage From Number With Format String
- ASP.NET PhysicalApplicationPath
- C# PictureBox: Windows Forms
- C# PNG Optimization
- C# Position Windows: Use WorkingArea and Location
- Visual Studio Post Build, Pre Build Macros
- C# ProfileOptimization
- C# ProgressBar Example
- C# Property Examples
- C# PropertyGrid: Windows Forms
- C# Protected and internal Keywords
- C# Public and private Methods
- C# Remove Punctuation From String
- C# Query Windows Forms (Controls.OfType)
- C# Queryable: IQueryable Interface and AsQueryable
- ASP.NET QueryString Examples
- C# Queue Collection: Enqueue
- C# RadioButton Use: Windows Forms
- C# ReadOnlyCollection Use (ObjectModel)
- C# Recursion Optimization
- C# Recursive File List: GetFiles With AllDirectories
- C# ref Keyword
- C# Reflection Examples
- C# Regex.Escape and Unescape Methods
- C# StringBuilder Examples
- C# StringComparison and StringComparer
- C# StringReader Class (Get Parts of Strings)
- C# String GetEnumerator() method
- C# String GetHashCode() method
- C# Regex Versus Loop: Use For Loop Instead of Regex
- C# Regex.Match Examples: Regular Expressions
- C# RemoveAll: Use Lambda to Delete From List
- C# Replace String Examples
- ASP.NET Response.BinaryWrite
- ASP.NET Response.Write
- C# Return Optimization: out Performance
- C# SaveFileDialog: Use ShowDialog and FileName
- C# Scraping HTML Links
- C# sealed Keyword
- C# Seek File Examples: ReadBytes
- C# select new Example: LINQ
- C# Select Method (Use Lambda to Modify Elements)
- C# Serialize List (Write to File With BinaryFormatter)
- C# Settings.settings in Visual Studio
- C# Shuffle Array: KeyValuePair and List
- C# Single and Double Types
- C# Single Instance Windows Form
- C# Snippet Examples
- C# Sort DateTime List
- C# Sort List With Lambda, Comparison Method
- C# Sort Number Strings
- C# Sort Examples: Arrays and Lists
- C# SortedDictionary
- C# SortedList
- C# SortedSet Examples
- C# Split String Examples
- C# String Copy() method
- C# SplitContainer: Windows Forms
- C# SqlClient Tutorial: SqlConnection, SqlCommand
- C# SqlCommand Example: SELECT TOP, ORDER BY
- C# SqlCommandBuilder Example: GetInsertCommand
- C# SqlConnection Example: Using, SqlCommand
- C# SqlDataAdapter Example
- C# SqlDataReader: GetInt32, GetString
- C# SqlParameter Example: Constructor, Add
- C# Stack Collection: Push, Pop
- C# Static List: Global List Variable
- C# Static Regex
- C# Static String
- C# static Keyword
- C# StatusStrip Example: Windows Forms
- C# String Chars (Get Char at Index)
- C# string.Concat Examples
- C# String Interpolation Examples
- C# string.Join Examples
- C# String Performance, Memory Usage Info
- C# String Property
- C# String Slice, Get Substring Between Indexes
- C# String Switch Examples
- C# String
- C# StringBuilder Append Performance
- C# StringBuilder Cache
- C# ToBase64String (Data URI Image)
- C# Struct Versus Class
- C# struct Examples
- C# Substring Examples
- C# Numeric Suffix Examples
- C# switch Examples
- C# String IsNormalized() method
- C# TabControl: Windows Forms
- TableLayoutPanel: Windows Forms
- C# Take and TakeWhile Examples
- C# Task Examples (Task.Run, ContinueWith and Wait)
- C# Ternary Operator
- C# Text Property: Windows Forms
- C# TextBox.AppendText Method
- C# TextBox Example
- C# TextChanged Event
- C# TextFieldParser Examples: Read CSV
- C# ThreadStart and ParameterizedThreadStart
- C# throw Keyword Examples
- C# Timer Examples
- C# TimeSpan Examples
- C# TrimEnd and TrimStart
- C# True and False
- C# Truncate String
- C# String ToLower() method
- C# String ToCharArray() method
- C# String ToUpperInvariant() method
- C# String Trim() method
- C# Assign Variables Optimization
- C# Array.Resize Examples
- C# Array.Sort: Keys, Values and Ranges
- C# Array.Reverse Example
- C# Array Slice, Get Elements Between Indexes
- C# Array.TrueForAll: Use Lambda to Test All Elements
- C# ArrayTypeMismatchException
- C# as: Cast Examples
- C# ASCII Table
- C# ASCII Transformation, Convert Char to Index
- C# AsEnumerable Method
- C# AsParallel Example
- ASP.NET AspLiteral
- C# BaseStream Property
- C# Console.Beep Example
- C# Benchmark
- C# BinaryReader Example (Use ReadInt32)
- C# BinaryWriter Type
- C# BitArray Examples
- C# BitConverter Examples
- C# Bitcount Examples
- C# Bool Methods, Return True and False
- C# bool Sort Examples (True to False)
- C# Caesar Cipher
- C# Cast Extension: System.Linq
- C# Cast to Int (Convert Double to Int)
- C# Cast Examples
- C# catch Examples
- C# Change Characters in String (ToCharArray, For Loop)
- C# Char Combine: Get String From Chars
- C# char.IsDigit (If Char Is Between 0 and 9)
- C# char.IsLower and IsUpper
- C# Character Literal (const char)
- C# Char Lowercase Optimization
- C# Char Test (If Char in String Equals a Value)
- C# char.ToLower and ToUpper
- C# char Examples
- C# abstract Keyword
- C# Action Object (Lambda That Returns Void)
- C# Aggregate: Use Lambda to Accumulate Value
- C# AggressiveInlining: MethodImpl Attribute
- C# Anagram Method
- C# And Bitwise Operator
- C# Anonymous Function (Delegate With No Name)
- C# Any Method, Returns True If Match Exists
- C# StringBuilder Append and AppendLine
- C# StringBuilder AppendFormat
- ASP.NET appSettings Example
- C# ArgumentException: Invalid Arguments
- C# Array.ConvertAll, Change Type of Elements
- C# Array.Copy Examples
- C# Array.CreateInstance Method
- C# Array and Dictionary Test, Integer Lookups
- C# Array.Exists Method, Search Arrays
- C# Array.Find Examples, Search Array With Lambda
- C# Array.ForEach: Use Lambda on Every Element
- C# Array Versus List Memory Usage
- C# Array Property, Return Empty Array
- C# CharEnumerator
- C# Chart, Windows Forms (Series and Points)
- C# CheckBox: Windows Forms
- C# class Examples
- C# Clear Dictionary: Remove All Keys
- C# Clone Examples: ICloneable Interface
- C# Closest Date (Find Dates Nearest in Time)
- C# Combine Arrays: List, Array.Copy and Buffer.BlockCopy
- C# Combine Dictionary Keys
- C# ComboBox: Windows Forms
- C# CompareTo Int Method
- C# Comparison Object, Used With Array.Sort
- C# Compress Data: GZIP
- C# Console.Read Method
- C# Console.ReadKey Example
- C# Console.ReadLine Example (While Loop)
- C# Console.SetOut and Console.SetIn
- C# Console.WindowHeight
- C# Console.Write, Append With No Newline
- C# Console.WriteLine (Print)
- C# const Example
- C# Constraint Puzzle Solver
- C# Count Characters in String
- C# Count, Dictionary (Get Number of Keys)
- C# Count Letter Frequencies
- C# Count Extension Method: Use Lambda to Count
- C# CSV Methods (Parse and Segment)
- C# DataRow Field Method: Cast DataTable Cell
- C# Get Day Count Elapsed From DateTime
- C# DateTime Format
- C# DateTime.Now (Current Time)
- C# DateTime.Today (Current Day With Zero Time)
- C# DateTime.TryParse and TryParseExact
- C# DateTime Examples
- C# DateTimePicker Example
- C# Debug.Write Examples
- C# Visual Studio Debugging Tutorial
- C# decimal Examples
- C# DayOfWeek
- C# Enum.Format Method (typeof Enum)
- C# Enum.GetName, GetNames: Get String Array From Enum
- C# Enum.Parse, TryParse: Convert String to Enum
- C# Error and Warning Directives
- C# ErrorProvider Control: Windows Forms
- C# event Examples
- C# Get Every Nth Element From List (Modulo)
- C# Excel Interop Example
- C# Except (Remove Elements From Collection)
- C# Extension Method
- C# extern alias Example
- C# Convert Feet, Inches
- C# File.Delete
- C# File Equals: Compare Files
- C# File.Exists Method
- C# try Keyword
- C# TryGetValue (Get Value From Dictionary)
- C# Tuple Examples
- C# Type Class: Returned by typeof, GetType
- C# TypeInitializationException
- C# Union: Combine and Remove Duplicate Elements
- C# Unreachable Code Detected
- C# Unsafe Keyword: Fixed, Pointers
- C# Uppercase First Letter
- C# Uri and UriBuilder Classes
- C# Using Alias Example
- C# using Statement: Dispose and IDisposable
- C# value Keyword
- C# ValueTuple Examples (System.ValueTuple, ToTuple)
- C# ValueType Examples
- C# Variable Initializer for Class Field
- C# Word Count
- C# Word Interop: Microsoft.Office.Interop.Word
- C# XElement Example (XElement.Load, XName)
- C# Zip Method (Use Lambda on Two Collections)
- C# File.Move Method, Rename File
- C# File.Open Examples
- C# File.ReadAllBytes, Get Byte Array From File
- C# File.ReadAllLines, Get String Array From File
- C# File.ReadAllText, Get String From File
- C# File.ReadLines, Use foreach Over Strings
- C# File.Replace Method
- C# FileInfo Length, Get File Size
- C# FileInfo Examples
- C# File Handling
- C# Filename With Date Example (DateTime.Now)
- C# FileNotFoundException (catch Example)
- C# FileStream Length, Get Byte Count From File
- C# FileStream Example, File.Create
- C# FileSystemWatcher Tutorial (Changed, e.Name)
- C# finally Keyword
- C# First Sentence
- C# FirstOrDefault (Get First Element If It Exists)
- C# Fisher Yates Shuffle: Generic Method
- C# fixed Keyword (unsafe)
- C# Flatten Array (Convert 2D to 1D)
- C# First Words in String
- C# First (Get Matching Element With Lambda)
- C# ContainsKey Method (Key Exists in Dictionary)
- C# Convert ArrayList to Array (Use ToArray)
- C# Convert ArrayList to List
- C# Convert Bool to Int
- C# Convert Bytes to Megabytes
- C# Convert Degrees Celsius to Fahrenheit
- C# Convert Dictionary to List
- C# Convert Dictionary to String (Write to File)
- C# Convert List to Array
- C# Convert List to DataTable (DataGridView)
- C# Convert List to String
- C# Convert Miles to Kilometers
- C# Convert Milliseconds, Seconds, Minutes
- C# Convert Nanoseconds, Microseconds, Milliseconds
- C# Convert String Array to String
- C# Convert TimeSpan to Long
- C# Convert Types
- C# Copy Dictionary
- C# Count Elements in Array and List
- C# FromOADate and Excel Dates
- C# Generic Class, Generic Method Examples
- C# GetEnumerator: While MoveNext, Get Current
- C# GetHashCode (String Method)
- C# Thumbnail Image With GetThumbnailImage
- C# GetType Method
- C# Global Variable Examples (Public Static Property)
- ASP.NET Global Variables Example
- C# Group By Operator: LINQ
- GroupBox: Windows Forms
- C# GroupBy Method: LINQ
- C# GroupJoin Method
- C# GZipStream Example (DeflateStream)
- C# HashSet Examples
- C# Hashtable Examples
- HelpProvider Control Use
- C# HTML and XML Handling
- C# HtmlEncode and HtmlDecode
- C# HtmlTextWriter Example
- C# HttpUtility.HtmlEncode Methods
- C# HybridDictionary
- C# default Operator
- C# DefaultIfEmpty Method
- C# Define and Undef Directives
- C# Destructor
- C# DialogResult: Windows Forms
- C# Dictionary, Read and Write Binary File
- C# Dictionary Memory
- C# Dictionary Optimization, Increase Capacity
- C# Dictionary Optimization, Test With ContainsKey
- C# DictionaryEntry Example (Hashtable)
- C# Directives
- C# Directory.CreateDirectory, Create New Folder
- C# Directory.GetFiles Example (Get List of Files)
- C# DivideByZeroException
- C# DllImport Attribute
- C# Do While Loop Example
- C# DriveInfo Examples
- C# DropDownItems Control
- C# IComparable Example, CompareTo: Sort Objects
- C# IDictionary Generic Interface
- C# IEnumerable Examples
- C# IList Generic Interface: List and Array
- C# Image Type
- C# ImageList Use: Windows Forms
- C# Increment String That Contains a Number
- C# Increment, Preincrement and Decrement Ints
- Dot Net Perls
- C# Indexer Examples (This Keyword, get and set)
- C# IndexOutOfRangeException
- C# Inheritance
- C# Insert String Examples
- C# int Array
- C# Interface Examples
- C# Interlocked Examples: Add, CompareExchange
- C# Intersect: Get Common Elements
- C# InvalidCastException
- C# InvalidOperationException: Collection Was Modified
- C# IOException Type: File, Directory Not Found
- C# IOrderedEnumerable (Query Expression With orderby)
- C# is: Cast Examples
- C# IsFixedSize, IsReadOnly and IsSynchronized Arrays
- C# string.IsNullOrEmpty, IsNullOrWhiteSpace
- C# IsSorted Method: If Array Is Already Sorted
- C# Jagged Array Examples
- C# join Examples (LINQ)
- C# KeyCode Property and KeyDown
- C# KeyNotFoundException: Key Not Present in Dictionary
- C# KeyValuePair Examples
- C# Line Count for File
- C# Line Directive
- C# LinkedList
- C# List CopyTo (Copy List Elements to Array)
- C# List Equals (If Elements Are the Same)
- C# List Find and Exists Examples
- C# List Insert Performance
- ASP.NET LiteralControl Example
- C# Locality Optimizations (Memory Hierarchy)
- C# lock Keyword
- C# Long and ulong Types
- C# Loop Over String Chars: Foreach, For
- C# Loop Over String Array
- C# Main args Examples
- C# Map Example
- ASP.NET MapPath: Virtual and Physical Paths
- C# Mask Optimization
- C# MaskedTextBox Example
- C# Math.Abs: Absolute Value
- C# Math.Ceiling Usage
- C# Math.Floor Method
- C# Math.Max and Math.Min Examples
- C# Math.Pow Method, Exponents
- C# Math.Round Examples: MidpointRounding
- C# Math Type
- C# Max and Min: Get Highest or Lowest Element
- C# MemoryFailPoint and InsufficientMemoryException
- C# MemoryStream: Use Stream on Byte Array
- C# MenuStrip: Windows Forms
- C# Modulo Operator: Get Remainder From Division
- C# MonthCalendar Control: Windows Forms
- C# Multiple Return Values
- C# Multiply Numbers
- C# namespace Keyword
- C# NameValueCollection Usage
- C# Nested Lists: Create 2D List or Jagged List
- C# Nested Switch Statement
- C# Environment.NewLine
- C# Normalize, IsNormalized Methods
- C# Null String Example
- C# null Keyword
- C# Nullable Examples
- C# NullReferenceException and Null Parameter
- C# Object Array
- C# Obsolete Attribute
- C# OfType Examples
- C# OpenFileDialog Example
- C# operator Keyword
- C# Odd and Even Numbers
- C# Bitwise Or
- C# orderby Query Keyword
- C# out Parameter
- C# OutOfMemoryException
- C# OverflowException
- C# Overload Method
- C# Override Method
- C# PadRight and PadLeft: String Columns
- C# Get Paragraph From HTML With Regex
- C# Parallel.For Example (Benchmark)
- C# Parallel.Invoke: Run Methods on Separate Threads
- C# Parameter Optimization
- C# Parameter Passing, ref and out
- C# params Keyword
- C# int.Parse: Convert Strings to Integers
- C# partial Keyword
- C# Path.ChangeExtension
- C# Path Exists Example
- C# Path.GetExtension: File Extension
- C# Path.GetRandomFileName Method
- C# Pragma Directive
- C# Predicate (Lambda That Returns True or False)
- C# Pretty Date Format (Hours or Minutes Ago)
- C# PreviewKeyDown Event
- C# Random Lowercase Letter
- C# Random Paragraphs and Sentences
- C# Random String
- C# Random Number Examples
- C# StreamReader ReadLine, ReadLineAsync Examples
- C# readonly Keyword
- C# Recursion Example
- C# Regex, Read and Match File Lines
- C# Regex Groups, Named Group Example
- C# Regex.Matches Quote Example
- C# Regex.Matches Method: foreach Match, Capture
- C# Regex.Replace, Matching End of String
- C# Regex.Replace, Remove Numbers From String
- C# Regex.Replace, Merge Multiple Spaces
- C# Regex.Replace Examples: MatchEvaluator
- C# Regex.Split, Get Numbers From String
- C# Regex.Split Examples
- C# Regex Trim, Remove Start and End Spaces
- C# RegexOptions.Compiled
- C# RegexOptions.IgnoreCase Example
- C# RegexOptions.Multiline
- C# Region and endregion
- C# Remove Char From String at Index
- C# Remove Element
- C# Remove HTML Tags
- C# Remove String
- C# Reserved Filenames
- C# return Keyword
- C# Reverse String
- C# Reverse Words
- C# Reverse Extension Method
- C# RichTextBox Example
- C# Right String Part
- C# RNGCryptoServiceProvider Example
- C# ROT13 Method, Char Lookup Table
- C# SelectMany Example: LINQ
- C# Sentinel Optimization
- C# SequenceEqual Method (If Two Arrays Are Equal)
- C# Shift Operators (Bitwise)
- C# Short and ushort Types
- C# Single Method: Get Element If Only One Matches
- C# SingleOrDefault
- C# Singleton Pattern Versus Static Class
- C# Singleton Class
- C# sizeof Keyword
- C# Skip and SkipWhile Examples
- C# Sleep Method (Pause)
- C# Sort Dictionary: Keys and Values
- C# Sort by File Size
- C# Sort, Ignore Leading Chars
- C# Sort KeyValuePair List: CompareTo
- C# Sort Strings by Length
- C# Thread.SpinWait Example
- C# Math.Sqrt Method
- C# stackalloc Operator
- C# StackOverflowException
- C# StartsWith and EndsWith String Methods
- C# Static Array
- C# Static Dictionary
- C# Stopwatch Examples
- C# Stream
- C# StreamReader ReadToEnd Example (Read Entire File)
- C# StreamReader ReadToEndAsync Example (Performance)
- C# StreamReader Examples
- C# StreamWriter Examples
- C# String Append (Add Strings)
- C# String Compare and CompareTo Methods
- C# String Constructor (new string)
- C# string.Copy Method
- C# CopyTo String Method: Put Chars in Array
- C# Empty String Examples
- C# String Equals Examples
- C# String For Loop, Count Spaces in String
- C# string.Intern and IsInterned
- C# String IsUpper, IsLower
- C# String Length Property: Get Character Count
- C# String Literal: Newline and Quote Examples
- C# StringBuilder Capacity
- C# StringBuilder Clear (Set Length to Zero)
- C# StringBuilder Data Types
- C# StringBuilder Performance
- C# StringBuilder Equals (If Chars Are Equal)
- C# StringBuilder Memory
- C# StringBuilder ToString: Get String From StringBuilder
- C# StringWriter Class
- C# Sum Method: Add up All Numbers
- C# Switch Char, Test Chars With Cases
- C# Switch Enum
- C# System (using System namespace)
- C# Tag Property: Windows Forms
- C# TextInfo Examples
- C# TextReader, Returned by File.OpenText
- C# TextWriter, Returned by File.CreateText
- C# this Keyword
- C# ThreadPool
- C# Thread Join Method (Join Array of Threads)
- C# ThreadPool.SetMinThreads Method
- C# TimeZone Examples
- C# Get Title From HTML With Regex
- C# ToArray Extension Method
- C# ToCharArray: Convert String to Array
- C# ToDictionary Method
- C# Token
- C# ToList Extension Method
- C# ToLookup Method (Get ILookup)
- C# ToLower and ToUpper: Uppercase and Lowercase Strings
- ToolStripContainer Control: Dock, Properties
- C# ToolTip: Windows Forms
- C# ToString Integer Optimization
- C# ToString: Get String From Object
- C# ToTitleCase Method
- C# TrackBar: Windows Forms
- C# Tree and Nodes Example: Directed Acyclic Word Graph
- C# TreeView Tutorial
- C# Trim Strings
- C# Thread Methods
- C# History
- C# Features
- C# Variables
- C# Data Types
- C# Operators
- C# Keywords
- C# New Features | C# Version Features
- C# Programs
- C# Program to swap numbers without third variable
- C# Program to convert Decimal to Binary
- C# Program to Convert Number in Characters
- C# Program to Print Alphabet Triangle
- C# Program to print Number Triangle
- C# Program to generate Fibonacci Triangle
- C# String Compare() method
- C# String CompareOrdinal() method
- C# String CompareTo() method
- C# String Concat() method
- C# String Contains() method
- C# String CopyTo() method
- C# String EndsWith() method
- C# String Equals() method
- C# String Format() method
- C# String IndexOf() method
- C# String Insert() method
- C# String Intern(String str) method
- C# String IsInterned() method
- C# String Normalize() method
- C# String IsNullOrEmpty() method
- C# String IsNullOrWhiteSpace() method
- C# String Join() method
- C# String LastIndexOf() method
- C# String LastIndexOfAny() method
- C# String PadLeft() method
- C# String PadRight() method
- C# Nullable
- C# String Remove() method
- C# String Replace() method
- C# String Split() method
- C# String StartsWith() method
- C# String SubString() method
- C# Partial Types
- C# Iterators
- C# Delegate Covariance
- C# Delegate Inference
- C# Anonymous Types
- C# Extension Methods
- C# Query Expression
- C# Partial Method
- C# Implicitly Typed Local Variable
- C# Object and Collection Initializer
- C# Auto Implemented Properties
- C# Dynamic Binding
- C# Named and Optional Arguments
- C# Asynchronous Methods
- C# Caller Info Attributes
- C# Using Static Directive
- C# Exception Filters
- C# Await in Catch Finally Blocks
- C# Default Values for Getter Only Properties
- C# Expression Bodied Members
- C# Null Propagator
- C# String Interpolation
- C# nameof operator
- C# Dictionary Initializer
- C# Pattern Matching
- C# Tuples
- C# Deconstruction
- C# Local Functions
- C# Binary Literals
- C# Ref Returns and Locals
- C# Expression Bodied Constructors and Finalizers
- C# Expression Bodied Getters and Setters
- C# Async Main
- C# Default Expression
Related Links
Adjectives
Ado
Ai
Android
Angular
Antonyms
Apache
Articles
Asp
Autocad
Automata
Aws
Azure
Basic
Binary
Bitcoin
Blockchain
C
Cassandra
Change
Coa
Computer
Control
Cpp
Create
Creating
C-Sharp
Cyber
Daa
Data
Dbms
Deletion
Devops
Difference
Discrete
Es6
Ethical
Examples
Features
Firebase
Flutter
Fs
Git
Go
Hbase
History
Hive
Hiveql
How
Html
Idioms
Insertion
Installing
Ios
Java
Joomla
Js
Kafka
Kali
Laravel
Logical
Machine
Matlab
Matrix
Mongodb
Mysql
One
Opencv
Oracle
Ordering
Os
Pandas
Php
Pig
Pl
Postgresql
Powershell
Prepositions
Program
Python
React
Ruby
Scala
Selecting
Selenium
Sentence
Seo
Sharepoint
Software
Spellings
Spotting
Spring
Sql
Sqlite
Sqoop
Svn
Swift
Synonyms
Talend
Testng
Types
Uml
Unity
Vbnet
Verbal
Webdriver
What
Wpf